How Can You Efficiently Loop Over a Struct in Golang?
In the world of Go programming, structs are one of the fundamental building blocks that allow developers to create complex data types. Whether you’re building a simple application or a large-scale system, understanding how to effectively manipulate and iterate over structs is crucial for efficient data handling. If you’ve ever found yourself wondering how to loop over structs in Go, you’re not alone. This article will guide you through the intricacies of struct iteration, providing you with the tools you need to harness the full potential of this powerful feature.
Looping over structs in Go is not just a matter of syntax; it involves understanding the underlying principles of data organization and memory management that Go employs. As you delve into this topic, you’ll discover various techniques to access and manipulate the fields of structs, whether you’re working with a single instance or a collection of them. From using slices to leveraging the `range` keyword, the possibilities are vast, and the methods can vary based on your specific use case.
Moreover, the ability to loop over structs can significantly enhance your code’s readability and maintainability. By mastering this skill, you’ll be able to write cleaner, more efficient code that can adapt to changing requirements. As we explore the different approaches to iterating through structs, you’ll gain insights into best practices and common pitfalls to avoid,
Looping Over Structs in Go
In Go, looping over structs can be accomplished in various ways depending on the requirements. The most common methods involve using slices or arrays of structs, which allow you to iterate through each element efficiently.
To demonstrate, consider the following struct definition:
“`go
type Person struct {
Name string
Age int
}
“`
To loop over a slice of `Person` structs, you can use the `for` loop. Here’s how you can do it:
“`go
people := []Person{
{“Alice”, 30},
{“Bob”, 25},
{“Charlie”, 35},
}
for _, person := range people {
fmt.Printf(“Name: %s, Age: %d\n”, person.Name, person.Age)
}
“`
In this example, the `range` clause provides both the index and the value of each element. Since the index is not needed, it’s replaced with an underscore (`_`).
Using a For Loop with Index
Alternatively, you can also use a traditional `for` loop with an index to iterate over the slice:
“`go
for i := 0; i < len(people); i++ {
fmt.Printf("Name: %s, Age: %d\n", people[i].Name, people[i].Age)
}
```
This approach can be useful when you need the index for further operations or comparisons.
Looping Over Struct Fields
If you want to loop over the fields of a struct, you will need to use the `reflect` package. This is particularly useful when you need to deal with dynamic struct fields. Here’s a quick example:
“`go
import (
“fmt”
“reflect”
)
func printStructFields(s interface{}) {
v := reflect.ValueOf(s)
t := v.Type()
for i := 0; i < v.NumField(); i++ { fmt.Printf("%s: %v\n", t.Field(i).Name, v.Field(i).Interface()) } } person := Person{"Alice", 30} printStructFields(person) ``` This function will print each field name and value of the `Person` struct.
Summary of Looping Techniques
Technique | Description |
---|---|
For Range Loop | Simplest way to iterate through a slice of structs. |
Traditional For Loop | Useful when the index is required. |
Reflect Package | Allows iterating over struct fields dynamically. |
When working with structs in Go, understanding these methods allows for flexibility and control over how you handle data structures. Each method has its use case, and selecting the appropriate one depends on the specific needs of your application.
Looping Over Structs in Go
In Go, looping over a struct involves iterating through its fields, which can be achieved using the `reflect` package. This package allows you to inspect the type and value of an object at runtime, making it possible to work with structs generically.
Using the Reflect Package
The `reflect` package provides functions to obtain information about types and values. To loop over a struct, follow these steps:
- Import the `reflect` package.
- Create an instance of the struct.
- Use `reflect.TypeOf()` to get the type of the struct.
- Use `reflect.ValueOf()` to get the value of the struct.
- Iterate through the fields using a loop.
Here is a sample implementation:
“`go
package main
import (
“fmt”
“reflect”
)
type Person struct {
Name string
Age int
}
func main() {
p := Person{Name: “Alice”, Age: 30}
val := reflect.ValueOf(p)
for i := 0; i < val.Type().NumField(); i++ { field := val.Type().Field(i) value := val.Field(i) fmt.Printf("%s: %v\n", field.Name, value) } } ``` Explanation of Code
- `reflect.ValueOf(p)`: Retrieves the value of the struct instance.
- `val.Type().NumField()`: Returns the number of fields in the struct.
- `val.Type().Field(i)`: Accesses the field information at index `i`.
- `val.Field(i)`: Retrieves the value of the field at index `i`.
Iterating Over Structs with Tags
Struct tags can be utilized to provide metadata about struct fields. When iterating, you can also access these tags. Here’s an example:
“`go
package main
import (
“fmt”
“reflect”
)
type Person struct {
Name string `json:”name”`
Age int `json:”age”`
}
func main() {
p := Person{Name: “Bob”, Age: 25}
val := reflect.ValueOf(p)
for i := 0; i < val.Type().NumField(); i++ { field := val.Type().Field(i) value := val.Field(i) tag := field.Tag.Get("json") fmt.Printf("%s (%s): %v\n", field.Name, tag, value) } } ``` Key Points
- Use `field.Tag.Get(“json”)` to retrieve the tag for JSON serialization.
- This method allows you to dynamically handle field metadata.
Limitations and Considerations
When working with reflection, consider the following:
- Performance: Reflection can be slower than direct access due to the additional overhead.
- Safety: Reflection bypasses compile-time checks; ensure you validate types and values to avoid runtime panics.
- Complexity: Code using reflection can be harder to read and maintain.
Aspect | Reflection | Direct Access |
---|---|---|
Performance | Slower | Faster |
Type Safety | Less safe | More safe |
Readability | Less readable | More readable |
By understanding how to effectively loop over structs using the `reflect` package, you can manipulate and access struct data dynamically, enabling more flexible and generic programming patterns in Go.
Expert Insights on Looping Over Structs in Go
Dr. Emily Carter (Senior Software Engineer, GoLang Innovations). “Looping over structs in Go can be efficiently achieved using the `range` keyword. This allows developers to iterate over the fields of a struct, providing both the field name and value. However, it is crucial to understand that structs are value types, so modifications to the fields within the loop will not affect the original struct unless a pointer receiver is used.”
Michael Chen (Go Language Advocate, TechWrite Publications). “When working with slices of structs, the `for` loop combined with `range` is the most idiomatic approach in Go. It not only enhances readability but also ensures that the loop operates efficiently without unnecessary memory overhead. Developers should also be mindful of the zero value of structs when initializing them in a loop.”
Sarah Johnson (Go Developer and Educator, CodeMaster Academy). “For those new to Go, understanding how to loop over structs is essential for effective data manipulation. Utilizing the `reflect` package can offer deeper insights into struct types and their fields, allowing for dynamic iteration. However, this should be done judiciously, as it may introduce performance overhead compared to direct field access.”
Frequently Asked Questions (FAQs)
How do I define a struct in Go?
To define a struct in Go, use the `type` keyword followed by the struct name and the `struct` keyword. For example:
“`go
type Person struct {
Name string
Age int
}
“`
What is the syntax to create an instance of a struct?
You can create an instance of a struct by using the struct name followed by curly braces containing the field values. For example:
“`go
p := Person{Name: “Alice”, Age: 30}
“`
How can I loop over the fields of a struct in Go?
Go does not provide a direct way to loop over struct fields. However, you can use reflection to achieve this. Here’s a basic example:
“`go
import “reflect”
func loopOverStruct(s interface{}) {
v := reflect.ValueOf(s)
for i := 0; i < v.NumField(); i++ {
field := v.Type().Field(i)
value := v.Field(i)
fmt.Printf("%s: %v\n", field.Name, value.Interface())
}
}
```
Can I loop over a slice of structs in Go?
Yes, you can loop over a slice of structs using a `for` loop. For example:
“`go
people := []Person{{“Alice”, 30}, {“Bob”, 25}}
for _, person := range people {
fmt.Println(person.Name, person.Age)
}
“`
What is the difference between a pointer to a struct and a struct value in Go?
A pointer to a struct allows you to modify the original struct when passing it to functions, while a struct value creates a copy. Using pointers can improve performance when dealing with large structs.
Is it possible to use method receivers with structs in Go?
Yes, you can define methods with receivers on structs. This allows you to associate behavior with your struct. For example:
“`go
func (p Person) Greet() string {
return “Hello, my name is ” + p.Name
}
“`
Looping over a struct in Go (Golang) involves utilizing the reflect package, as Go does not support direct iteration over structs like it does with slices or maps. The reflect package allows developers to inspect the type and value of struct fields dynamically. By leveraging the reflect.Value type, one can iterate through the struct’s fields and access their names and values, which is particularly useful for tasks such as serialization, logging, or implementing generic functions.
Another common approach to loop over a struct is to define methods on the struct that return slices or maps of the data you wish to iterate. This method provides a more type-safe way to access struct data without relying on reflection, which can be slower and less efficient. By encapsulating iteration logic within methods, developers can maintain clearer and more maintainable code while still achieving the desired functionality.
In summary, while Go does not natively support direct looping over structs, the use of the reflect package or custom methods provides effective alternatives. Understanding these techniques is essential for Go developers, as they enhance the ability to manipulate and interact with struct data flexibly and efficiently.
Author Profile
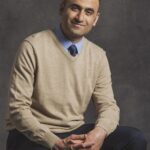
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?