How Can You Efficiently Loop Through a String in Python?
In the world of programming, strings are one of the most fundamental data types, serving as the backbone for text manipulation and processing. Whether you’re developing a simple script or a complex application, understanding how to loop through a string in Python can unlock a wealth of possibilities. This seemingly straightforward task can lead to powerful outcomes, from analyzing text data to crafting intricate algorithms. If you’ve ever wondered how to efficiently traverse each character of a string and harness its potential, you’re in the right place!
Looping through a string in Python is not just about accessing each character; it’s about leveraging the language’s elegant syntax and built-in functions to make your code more efficient and readable. Python offers several methods to iterate over strings, each with its own advantages and use cases. From traditional loops to more advanced techniques, mastering these approaches can enhance your programming skills and streamline your projects.
As we delve deeper into the various methods of looping through strings, you’ll discover how to apply these techniques in practical scenarios. Whether you’re looking to perform operations on individual characters, extract substrings, or even manipulate data for analysis, understanding how to loop through a string will empower you to write cleaner, more effective Python code. Get ready to unlock the secrets of string iteration!
Using For Loop to Iterate Through a String
The most common method for looping through a string in Python is by using a `for` loop. This allows you to access each character of the string sequentially. Here’s an example of how to implement this:
“`python
my_string = “Hello, World!”
for char in my_string:
print(char)
“`
In this example, the loop iterates over each character in `my_string`, printing them one by one. This method is simple and effective for tasks requiring direct access to each character.
Using While Loop to Iterate Through a String
An alternative approach to looping through a string is using a `while` loop. This method provides more control over the iteration process by explicitly managing the index:
“`python
my_string = “Hello, World!”
index = 0
while index < len(my_string):
print(my_string[index])
index += 1
```
In this code, a variable `index` is initialized to zero. The loop continues until `index` is equal to the length of the string, incrementing `index` with each iteration to access each character.
Accessing Characters by Index
Strings in Python are indexed, meaning you can access characters directly using their position in the string. The first character has an index of 0, the second an index of 1, and so forth. Here’s a demonstration:
“`python
my_string = “Python”
print(my_string[0]) Output: P
print(my_string[1]) Output: y
“`
Negative indexing can also be used to access characters from the end of the string. For instance, `my_string[-1]` returns the last character.
Looping with Enumerate
For scenarios where both the character and its index are needed, the `enumerate()` function is particularly useful. It allows for iteration while tracking the index automatically:
“`python
my_string = “Hello”
for index, char in enumerate(my_string):
print(f”Index: {index}, Character: {char}”)
“`
This method provides a clean and efficient way to access both the character and its index within the loop.
Table of Looping Techniques
Method | Code Example | Use Case |
---|---|---|
For Loop | for char in my_string: |
Simple character access |
While Loop | while index < len(my_string): |
When index control is needed |
Enumerate | for index, char in enumerate(my_string): |
Access both character and index |
Each of these methods has its advantages and can be chosen based on specific requirements of the task at hand.
Using a For Loop
One of the most straightforward methods to loop through a string in Python is by using a `for` loop. This approach iterates over each character in the string, allowing for easy access and manipulation.
```python
my_string = "Hello, World!"
for char in my_string:
print(char)
```
In this example, each character of the string `my_string` is printed on a new line. The `for` loop automatically handles the iteration over the string, making it simple and efficient.
Using a While Loop
Another method is to utilize a `while` loop, which can be beneficial when you need more control over the iteration process, such as modifying the index manually.
```python
my_string = "Hello, World!"
index = 0
while index < len(my_string):
print(my_string[index])
index += 1
```
This code snippet demonstrates how to loop through a string using an index variable. The loop continues until the index reaches the length of the string, accessing each character through indexing.
Using String Enumeration
Python provides a built-in function called `enumerate()` that can be particularly useful when you need both the index and the character of the string during iteration.
```python
my_string = "Hello, World!"
for index, char in enumerate(my_string):
print(f"Index {index}: {char}")
```
This code will output both the index and the corresponding character, allowing for more complex operations that may depend on the position of the character within the string.
Looping with List Comprehensions
For more compact code, list comprehensions can be employed to loop through a string and create a list based on the characters.
```python
my_string = "Hello, World!"
char_list = [char for char in my_string]
print(char_list)
```
This approach creates a list of characters from the string and is particularly useful for transforming or filtering characters in a concise manner.
Accessing Characters with Indices
If you need to access specific characters or ranges of characters, indexing is a powerful tool. Python strings support negative indexing, which allows you to access characters from the end of the string.
```python
my_string = "Hello, World!"
first_char = my_string[0] 'H'
last_char = my_string[-1] '!'
substring = my_string[0:5] 'Hello'
```
This method allows for targeted access to characters, enabling both simple retrieval and slicing of portions of the string.
Iterating with Conditions
When looping through a string, it may be necessary to apply conditions to filter or process certain characters.
```python
my_string = "Hello, World!"
for char in my_string:
if char.isalpha(): Check if the character is a letter
print(char)
```
In this example, only alphabetic characters are printed, demonstrating how to incorporate conditions into your string iteration.
Using the `join()` Method
The `join()` method can be used in conjunction with a loop to concatenate characters or strings together efficiently.
```python
my_string = "Hello, World!"
modified_string = ''.join(char for char in my_string if char.isalpha())
print(modified_string) Output: HelloWorld
```
This snippet constructs a new string consisting only of alphabetic characters, showcasing how to filter and join characters in a single expression.
Python provides multiple methods for looping through strings, each with its own advantages depending on the use case. Selecting the appropriate method enhances code clarity and efficiency.
Expert Insights on Looping Through Strings in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). "When looping through a string in Python, it is essential to utilize the `for` loop construct effectively. This allows for clean and readable code, enabling developers to iterate over each character seamlessly."
Michael Chen (Lead Software Engineer, CodeCraft Solutions). "Using the `enumerate()` function while looping through a string can be particularly beneficial. It provides both the index and the character, which is useful for scenarios where you need to track the position of each character in the string."
Sarah Patel (Python Educator, LearnPythonNow). "For beginners, employing a simple `for` loop is the best approach to understand string iteration. This foundational method lays the groundwork for more advanced techniques, such as list comprehensions and generator expressions."
Frequently Asked Questions (FAQs)
How can I loop through a string in Python?
You can loop through a string in Python using a `for` loop. For example:
```python
my_string = "Hello"
for char in my_string:
print(char)
```
This will print each character in the string on a new line.
What is the purpose of using a loop with a string?
Using a loop with a string allows you to access and manipulate each character individually. This is essential for tasks such as searching, modifying, or analyzing the content of the string.
Can I use a while loop to iterate through a string?
Yes, you can use a `while` loop to iterate through a string. You would typically use an index to access each character. For example:
```python
my_string = "Hello"
index = 0
while index < len(my_string):
print(my_string[index])
index += 1
```
What happens if I try to modify a string while looping through it?
Strings in Python are immutable, meaning you cannot change them directly. If you attempt to modify a string while looping, you will need to create a new string or use a list to store modifications before converting it back to a string.
Is there a way to loop through a string using list comprehension?
Yes, you can use list comprehension to create a list of characters from a string. For example:
```python
my_string = "Hello"
char_list = [char for char in my_string]
```
This creates a list containing each character from the string.
How can I loop through a string in reverse order?
You can loop through a string in reverse order by using slicing. For example:
```python
my_string = "Hello"
for char in my_string[::-1]:
print(char)
```
This will print the characters of the string from the last to the first.
Looping through a string in Python is a fundamental operation that allows developers to access and manipulate each character within the string. Python provides various methods to achieve this, including the use of simple for loops, while loops, and even list comprehensions. The most common approach is to utilize a for loop, which iterates over each character in the string, enabling easy access to individual elements for processing or analysis.
Another method to loop through a string is by using the built-in functions such as enumerate(), which provides both the index and the character during iteration. This can be particularly useful when the position of the character is relevant to the task at hand. Additionally, Python's slicing capabilities allow for more complex manipulations, enabling developers to extract substrings or modify parts of the string while iterating.
Key takeaways from the discussion on looping through strings in Python include the versatility of the for loop as the primary method for iteration, the utility of enumerate() for index-based access, and the power of string slicing for advanced operations. Understanding these techniques is essential for effective string manipulation and is a crucial skill for any Python programmer.
Author Profile
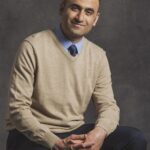
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?