How Can You Efficiently Loop Through a List in Python?
### Introduction
In the world of programming, loops are an essential tool that can transform the way we interact with data. Python, known for its simplicity and readability, offers a variety of ways to loop through lists, making it a favorite among both beginners and seasoned developers. Whether you’re processing data, automating tasks, or simply trying to understand how to manipulate collections of items, mastering the art of looping through lists is a fundamental skill that can significantly enhance your coding efficiency.
When it comes to looping through lists in Python, there are several techniques at your disposal, each with its own unique advantages. From the straightforward `for` loop to more advanced methods like list comprehensions, Python provides a flexible framework that caters to different programming styles and use cases. Understanding these methods not only helps in writing cleaner code but also in optimizing performance for larger datasets.
As you delve deeper into this topic, you’ll discover the nuances of each looping technique, including how to access elements, modify items on the fly, and even filter data based on specific criteria. By the end of this exploration, you’ll be equipped with the knowledge and skills to effectively navigate lists in Python, empowering you to tackle a wide range of programming challenges with confidence.
Using a For Loop
The most common way to loop through a list in Python is by utilizing a `for` loop. This approach allows you to iterate over each element in the list, performing operations or calculations as needed. The syntax is straightforward:
python
for item in my_list:
# Perform operations with item
This method is efficient and enhances readability, making it clear that you are processing each element sequentially.
Using a While Loop
Another method to loop through a list is by using a `while` loop. This is less common for this purpose, but it can be useful in specific scenarios. The syntax requires maintaining an index to access list elements:
python
index = 0
while index < len(my_list):
item = my_list[index]
# Perform operations with item
index += 1
While this provides more control over the iteration process, it can be more error-prone due to the manual management of the index.
Using List Comprehensions
List comprehensions offer a concise way to create lists by iterating over an existing list. They allow for more compact code while still being readable:
python
squared_list = [x**2 for x in my_list]
This example generates a new list containing the squares of the original list’s elements. List comprehensions can also include conditional statements to filter elements during iteration.
Using the Enumerate Function
When you need both the index and the value of the elements while looping through a list, `enumerate` is particularly useful. This built-in function provides a counter alongside the elements:
python
for index, item in enumerate(my_list):
# Perform operations with item and index
This approach is beneficial when tracking the position of each element within the list.
Iterating Over Nested Lists
When dealing with nested lists (lists within lists), you can use nested loops to iterate through each element:
python
for sublist in my_list:
for item in sublist:
# Perform operations with item
This structure allows you to access every item within the nested lists systematically.
Performance Considerations
When selecting a looping method, consider the following factors:
- Readability: Choose a method that is easy to understand.
- Performance: For very large lists, performance differences may be significant.
- Functionality: Ensure the method meets the specific needs of your task.
Method | Readability | Performance | Use Case |
---|---|---|---|
For Loop | High | Good | General iteration |
While Loop | Medium | Good | When index manipulation is needed |
List Comprehension | High | Very Good | Creating new lists |
Enumerate | High | Good | When index is needed |
Nested Loops | Medium | Variable | Iterating through nested lists |
Choosing the appropriate looping method can significantly affect the clarity and efficiency of your code.
Iterating Through a List Using a For Loop
The most common method to loop through a list in Python is by using a `for` loop. This approach is straightforward and allows for easy access to each element in the list.
python
my_list = [1, 2, 3, 4, 5]
for item in my_list:
print(item)
In this example, `item` takes on each value in `my_list` sequentially, and the `print` function outputs each value.
Using the Range Function with Indexing
Another approach to loop through a list is using the `range()` function along with indexing. This method is useful when you need to access the index of each element.
python
my_list = [‘a’, ‘b’, ‘c’, ‘d’]
for i in range(len(my_list)):
print(f’Index {i}: {my_list[i]}’)
Here, `len(my_list)` determines the number of elements, and `i` serves as the index to access each item.
List Comprehensions for Efficient Iteration
List comprehensions provide a compact way to process all items in a list and can replace the need for a loop in many situations. This method is not only concise but also efficient.
python
squared_list = [x**2 for x in range(10)]
print(squared_list)
This example generates a list of squares for numbers from 0 to 9, demonstrating how to loop through a range in a single line.
Using the Enumerate Function
The `enumerate()` function is a built-in Python function that allows you to loop through a list while keeping track of the index. This is particularly useful when both the index and the value are needed.
python
my_list = [‘apple’, ‘banana’, ‘cherry’]
for index, value in enumerate(my_list):
print(f’Index {index}: {value}’)
This approach provides a clear and readable way to access both the index and the value of each item in the list.
Looping Through a List of Dictionaries
When dealing with lists that contain dictionaries, you can still utilize the same looping techniques. This is beneficial for accessing specific values within the dictionaries.
python
list_of_dicts = [{‘name’: ‘Alice’, ‘age’: 30}, {‘name’: ‘Bob’, ‘age’: 25}]
for person in list_of_dicts:
print(f”{person[‘name’]} is {person[‘age’]} years old.”)
This code snippet iterates over each dictionary in the list and retrieves the values associated with the keys `name` and `age`.
Using While Loops for Custom Iteration
While loops can also be used to iterate through lists, especially when more complex conditions are involved. This method requires careful management of the index variable.
python
my_list = [10, 20, 30, 40]
index = 0
while index < len(my_list):
print(my_list[index])
index += 1
In this case, the loop continues until the index exceeds the length of the list, incrementing the index with each iteration.
Handling Nested Lists
For lists that contain other lists (nested lists), you can use nested loops to access elements at different levels.
python
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
for sublist in nested_list:
for item in sublist:
print(item)
This example demonstrates how to iterate through each sublist and then through each item within those sublists.
Expert Insights on Looping Through Lists in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Looping through lists in Python can be efficiently accomplished using a variety of methods such as for-loops, while-loops, and list comprehensions. Each method has its own advantages, and understanding when to use each can significantly enhance code performance and readability.”
Michael Tran (Data Scientist, Analytics Hub). “In Python, the most common way to iterate through a list is by using the for-loop. This method allows for straightforward access to each element, making it ideal for data manipulation tasks where clarity and simplicity are paramount.”
Sarah Lee (Software Engineer, CodeCraft Solutions). “Utilizing list comprehensions for looping through lists not only simplifies the code but also improves execution speed. This approach is particularly useful when transforming lists, as it condenses multiple lines of code into a single, expressive line.”
Frequently Asked Questions (FAQs)
How do I loop through a list in Python?
You can loop through a list in Python using a `for` loop. For example:
python
my_list = [1, 2, 3]
for item in my_list:
print(item)
What is the difference between a for loop and a while loop when looping through a list?
A `for` loop iterates over each element in a list directly, while a `while` loop continues until a specified condition is met. Using a `for` loop is generally more concise and easier for iterating through lists.
Can I use list comprehensions to loop through a list?
Yes, list comprehensions provide a concise way to create lists by looping through an existing list. For example:
python
squared = [x**2 for x in my_list]
Is it possible to modify elements of a list while looping through it?
Yes, you can modify elements of a list while looping through it, but it is recommended to use the `enumerate()` function to avoid issues with indexing. For example:
python
for index, value in enumerate(my_list):
my_list[index] = value * 2
What are some common pitfalls when looping through lists in Python?
Common pitfalls include modifying the list while iterating over it, which can lead to unexpected behavior, and using the wrong loop type for the task. Always ensure that the loop structure fits the intended operation.
Can I loop through a list in reverse order?
Yes, you can loop through a list in reverse order using the `reversed()` function or by specifying a negative step in slicing. For example:
python
for item in reversed(my_list):
print(item)
Looping through a list in Python is a fundamental skill that enables developers to efficiently access and manipulate data. Python provides several methods for iterating over lists, including the use of the `for` loop, list comprehensions, and the `enumerate()` function. Each of these methods offers unique advantages, allowing for flexibility depending on the specific requirements of the task at hand.
The most common approach is the `for` loop, which allows for straightforward iteration over each element in the list. This method is particularly useful for executing operations on each item, such as printing values or applying transformations. Additionally, list comprehensions offer a concise way to create new lists by applying an expression to each element, thereby enhancing code readability and efficiency.
Moreover, the `enumerate()` function is invaluable when both the index and the value of the list elements are needed. This function simplifies the process of tracking the position of elements while iterating, making it easier to perform operations that depend on the index. Understanding these various looping techniques is essential for effective programming in Python, as they contribute to writing cleaner, more efficient code.
Author Profile
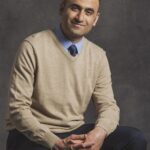
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?