How Can You Effectively Loop Through a List in Python?
In the world of programming, efficiency and simplicity are key, especially when it comes to handling collections of data. One of the fundamental tasks that every Python programmer encounters is the need to loop through lists. Whether you’re processing user inputs, managing data sets, or performing calculations, mastering the art of iterating over lists is essential. This powerful capability not only enhances your coding skills but also opens the door to more complex programming concepts.
Looping through lists in Python is a straightforward yet versatile process that can be accomplished in several ways. From traditional `for` loops to more advanced techniques like list comprehensions and the `enumerate` function, Python provides a rich toolkit for developers. Each method offers unique advantages, making it crucial to understand the best approach for your specific needs. As you delve deeper into the nuances of list iteration, you’ll discover how these techniques can streamline your code and improve its readability.
In this article, we will explore various methods to loop through lists in Python, highlighting their syntax and use cases. By the end, you’ll not only be equipped with practical skills but also gain insights into optimizing your code for better performance. So, whether you’re a novice programmer or an experienced developer looking to refine your skills, get ready to unlock the full potential of Python’s list iteration
Using a For Loop
The most common method to iterate through a list in Python is using a `for` loop. This approach allows you to access each element in the list sequentially.
“`python
my_list = [1, 2, 3, 4, 5]
for item in my_list:
print(item)
“`
In this example, the variable `item` takes the value of each element in `my_list` one at a time, and the `print` function outputs each value.
Using List Comprehensions
List comprehensions provide a concise way to create lists and can also be used for looping through a list. This method is especially useful for applying an operation to each element and generating a new list.
“`python
squared = [x**2 for x in my_list]
“`
This line creates a new list, `squared`, which contains the square of each element in `my_list`.
Using the While Loop
While loops can also be employed to iterate through a list, though this method is less common than `for` loops. You must manually manage the index variable.
“`python
index = 0
while index < len(my_list):
print(my_list[index])
index += 1
```
Here, the loop continues until the index reaches the length of the list, allowing access to each element.
Using the Enumerate Function
The `enumerate()` function is particularly useful when you need both the index and the value of each element. It adds a counter to the list during iteration.
“`python
for index, value in enumerate(my_list):
print(index, value)
“`
This produces output that includes both the index and the value:
Index | Value |
---|---|
0 | 1 |
1 | 2 |
2 | 3 |
3 | 4 |
4 | 5 |
Iterating with List Indexing
Another approach is to use list indexing directly within a loop. This method allows for more complex manipulations or conditions during iteration.
“`python
for i in range(len(my_list)):
print(f”Element at index {i} is {my_list[i]}”)
“`
This technique is beneficial when you need to modify the list while iterating or when the iteration logic is more complex.
Using Functional Programming Tools
Python includes several functional programming tools like `map()` and `filter()` that can operate on lists.
- Map applies a function to every item in the list:
“`python
def double(x):
return x * 2
doubled_list = list(map(double, my_list))
“`
- Filter creates a new list from those elements that satisfy a condition:
“`python
even_list = list(filter(lambda x: x % 2 == 0, my_list))
“`
These functional programming methods can lead to more readable and concise code, especially for operations that apply uniformly to list elements.
Methods to Loop Through a List in Python
Looping through a list in Python can be accomplished using several methods, each suitable for different scenarios. Below are the most commonly used techniques.
Using a For Loop
The `for` loop is the most straightforward way to iterate through a list. This method allows you to access each element directly.
“`python
my_list = [1, 2, 3, 4, 5]
for item in my_list:
print(item)
“`
In this example, each element in `my_list` is printed sequentially.
Using List Comprehension
List comprehension provides a concise way to create lists based on existing lists. It can also be used for iteration and transformation.
“`python
squared = [x**2 for x in my_list]
print(squared)
“`
This example creates a new list of squared values from `my_list`.
Using the enumerate() Function
The `enumerate()` function adds a counter to the iteration, allowing you to retrieve both the index and the value of each element.
“`python
for index, value in enumerate(my_list):
print(f”Index: {index}, Value: {value}”)
“`
This method is particularly useful when the position of elements is significant.
Using a While Loop
A `while` loop can be used to iterate through a list by maintaining an index variable. This method provides more control over the iteration process.
“`python
index = 0
while index < len(my_list):
print(my_list[index])
index += 1
```
This approach allows for conditional breaks or modifications to the index during the loop.
Using the map() Function
The `map()` function applies a specified function to every item in the list. This is useful for transformation without explicit loops.
“`python
def square(x):
return x ** 2
squared = list(map(square, my_list))
print(squared)
“`
The `map()` function is efficient for applying operations to lists.
Using List Iterators
Python provides iterators that allow you to traverse through a list without the need for indexing.
“`python
iterator = iter(my_list)
for item in iterator:
print(item)
“`
Using iterators can be more memory efficient, especially for large datasets.
Comparison of Looping Methods
Method | Description | Use Case |
---|---|---|
For Loop | Direct iteration over list elements | General-purpose iteration |
List Comprehension | Create new lists via concise syntax | Transforming data |
Enumerate | Access index and value simultaneously | When index matters |
While Loop | Manual index control | More complex iteration logic |
Map | Functional approach to apply a function | Applying transformations |
Iterators | Memory-efficient traversal | Large datasets |
Each method has its advantages depending on the context in which it is used. Selecting the appropriate technique enhances code readability and efficiency.
Expert Insights on Looping Through Lists in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “Looping through lists in Python is fundamental for data manipulation. Utilizing a for loop is the most straightforward method, allowing for easy access to each element. Additionally, list comprehensions provide a concise way to iterate and transform lists in a single line, enhancing both readability and performance.”
Michael Chen (Software Engineer, CodeCraft Solutions). “When it comes to looping through lists in Python, understanding the nuances of different loop constructs is crucial. While the for loop is commonly used, employing the enumerate function can be particularly beneficial when you need both the index and the value of the elements in the list, which can simplify many coding tasks.”
Sarah Patel (Python Instructor, LearnPython Academy). “In my experience teaching Python, I emphasize the importance of mastering loops for effective programming. Beyond the basic for loop, I encourage my students to explore the use of the while loop and the itertools module for more advanced looping techniques, which can greatly enhance their coding toolkit.”
Frequently Asked Questions (FAQs)
How can I loop through a list in Python?
You can loop through a list in Python using a `for` loop. For example:
“`python
my_list = [1, 2, 3]
for item in my_list:
print(item)
“`
What are the different methods to iterate over a list in Python?
Common methods to iterate over a list include using a `for` loop, a `while` loop, and list comprehensions. Each method serves different use cases and can be chosen based on the requirements of your code.
Can I use the `enumerate()` function while looping through a list?
Yes, the `enumerate()` function allows you to loop through a list while keeping track of the index of each item. For example:
“`python
for index, value in enumerate(my_list):
print(index, value)
“`
Is it possible to modify a list while looping through it?
Modifying a list while iterating over it can lead to unexpected behavior. It is generally recommended to create a copy of the list or use list comprehensions to avoid issues.
What is a list comprehension and how can it be used to loop through a list?
A list comprehension is a concise way to create lists. It allows you to loop through a list and apply an expression to each item in a single line. For example:
“`python
squared = [x**2 for x in my_list]
“`
Can I loop through a list of dictionaries in Python?
Yes, you can loop through a list of dictionaries using a `for` loop. You can access each dictionary’s keys and values within the loop. For example:
“`python
dict_list = [{‘a’: 1}, {‘b’: 2}]
for d in dict_list:
for key, value in d.items():
print(key, value)
“`
Looping through a list in Python is a fundamental skill that allows developers to efficiently access and manipulate each element within the list. Python provides several methods to iterate over lists, including the traditional `for` loop, list comprehensions, and the `enumerate()` function. Each method offers unique advantages, depending on the specific requirements of the task at hand.
The traditional `for` loop is the most straightforward approach, allowing developers to execute a block of code for each item in the list. This method is particularly useful for scenarios where additional operations or conditions need to be applied to each element. On the other hand, list comprehensions provide a more concise syntax, enabling the creation of new lists by applying an expression to each item in an existing list. This method is not only cleaner but also enhances code readability and performance in many cases.
Furthermore, the `enumerate()` function is invaluable when both the index and the value of each item are needed during iteration. This function simplifies the process of tracking the position of elements within the list, making it easier to perform operations that depend on the index. Overall, understanding these various methods of looping through lists in Python equips developers with the tools necessary to write efficient and effective code.
Author Profile
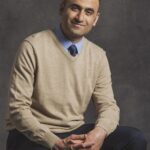
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?