How Can You Create Stunning Animations in Python?
How to Make a Captivating Animation in Python
In today’s digital landscape, animation has become an essential tool for storytelling, education, and entertainment. Whether you’re a budding programmer, a seasoned developer, or an artist looking to bring your creations to life, Python offers a versatile and accessible platform for crafting stunning animations. With its rich ecosystem of libraries and frameworks, you can transform your ideas into dynamic visual experiences that captivate and engage your audience.
Creating animations in Python is not just about the technical execution; it’s about harnessing creativity and innovation to convey messages effectively. From simple geometric shapes to intricate character movements, Python’s capabilities allow you to explore a wide range of animation styles and techniques. Libraries like Pygame, Matplotlib, and Manim provide the tools you need to start your animation journey, whether you’re aiming for a playful game, an educational video, or a data visualization project.
As you delve into the world of Python animations, you’ll discover that the process is both rewarding and enlightening. You’ll learn how to manipulate graphics, control timing, and synchronize movements, all while gaining a deeper understanding of programming concepts. So, if you’re ready to embark on this creative adventure, let’s explore the exciting possibilities that await you in the realm of Python animation!
Choosing the Right Library
When creating animations in Python, selecting the appropriate library is crucial. Several libraries cater to different needs, ranging from simple 2D animations to complex 3D visualizations. Here are some popular options:
- Matplotlib: Primarily used for plotting, it has basic animation capabilities.
- Pygame: Excellent for game development, offering extensive tools for 2D animations.
- Manim: Designed for mathematical animations, it provides a high level of control and precision.
- Blender (with Python API): Best suited for 3D animations, allowing for intricate designs and animations.
Each library has its strengths, depending on your specific animation requirements.
Basic Animation with Matplotlib
Matplotlib is a versatile library that can be used to create simple animations. It utilizes the `FuncAnimation` class from the `animation` module. Below is an example of how to create a basic sine wave animation.
“`python
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
x = np.linspace(0, 2 * np.pi, 100)
fig, ax = plt.subplots()
line, = ax.plot(x, np.sin(x))
def update(frame):
line.set_ydata(np.sin(x + frame / 10.0))
return line,
ani = FuncAnimation(fig, update, frames=100, blit=True)
plt.show()
“`
This code initializes a sine wave and updates its position over time, creating a smooth animation.
Creating Animation with Pygame
Pygame offers a more interactive approach to animations, making it ideal for game development. Below is a simple example of moving a rectangle across the screen.
“`python
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((800, 600))
clock = pygame.time.Clock()
x = 50
y = 50
width = 64
height = 64
velocity = 5
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
x -= velocity
if keys[pygame.K_RIGHT]:
x += velocity
screen.fill((0, 0, 0))
pygame.draw.rect(screen, (0, 128, 255), (x, y, width, height))
pygame.display.flip()
clock.tick(60)
“`
In this example, a rectangle moves left and right based on keyboard input, illustrating how to create interactive animations.
Advanced Animation Techniques
For more sophisticated animations, consider using libraries like Manim or Blender. These libraries allow you to create detailed animations with ease. Below is a comparative table highlighting features of different libraries for animation in Python.
Library | Type | Best Use Case |
---|---|---|
Matplotlib | 2D Plotting | Graphs and Scientific Data |
Pygame | 2D Game Development | Interactive Games |
Manim | Mathematical Animation | Educational Videos |
Blender | 3D Animation | Complex Visual Effects |
By understanding the capabilities and optimal use cases of each library, you can make informed choices that enhance your animation projects in Python.
Choosing the Right Libraries for Animation
When creating animations in Python, selecting the appropriate libraries is crucial for achieving the desired effects and ease of use. Below are some popular libraries that can be employed for animation tasks:
- Matplotlib: Primarily used for plotting, it also offers basic animation capabilities.
- Pygame: A set of Python modules designed for writing video games; it can be effectively used for 2D animations.
- Manim: A powerful library for mathematical animations; ideal for educational content.
- Pillow: A library for image processing that can create simple frame-based animations.
Library | Use Case | Pros | Cons |
---|---|---|---|
Matplotlib | Data visualization and simple animations | Built-in support for various plots | Limited animation features |
Pygame | Game development and 2D animations | High performance and extensive documentation | Steeper learning curve |
Manim | Educational and mathematical animations | High-quality output and flexibility | Complex setup |
Pillow | Image manipulation and frame animation | Easy to use for basic animations | Limited to frame-based animations |
Creating a Simple Animation Using Matplotlib
To create a basic animation using Matplotlib, follow these steps:
- Import Necessary Libraries:
“`python
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
“`
- Set Up the Figure:
“`python
fig, ax = plt.subplots()
xdata = np.linspace(0, 2*np.pi, 100)
ydata = np.sin(xdata)
line, = ax.plot(xdata, ydata)
“`
- Define Update Function:
“`python
def update(frame):
line.set_ydata(np.sin(xdata + frame / 10)) Update the data.
return line,
“`
- Create Animation:
“`python
ani = FuncAnimation(fig, update, frames=np.arange(0, 100), blit=True)
plt.show()
“`
This code generates a simple animation of a sine wave oscillating.
Building an Animation with Pygame
Pygame provides a more interactive approach for animations, especially suited for game development. Here’s how to set up a basic animation:
- Initialize Pygame:
“`python
import pygame
pygame.init()
“`
- Set Up the Display:
“`python
screen = pygame.display.set_mode((800, 600))
“`
- Load Images or Create Shapes:
“`python
color = (255, 0, 0) Red color
“`
- Main Loop:
“`python
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running =
screen.fill((255, 255, 255)) Fill background with white
pygame.draw.circle(screen, color, (400, 300), 50) Draw a circle
pygame.display.flip() Update the display
pygame.time.delay(100) Delay to control animation speed
pygame.quit()
“`
This example illustrates a simple animation where a circle is drawn on the screen.
Advanced Animations with Manim
Manim enables the creation of high-quality mathematical animations. Here’s a brief example of how to animate a mathematical object:
- Install Manim:
Use pip to install:
“`bash
pip install manim
“`
- Create a Scene:
“`python
from manim import *
class SineWave(Scene):
def construct(self):
axes = Axes(x_range=[-3, 3], y_range=[-2, 2], axis_config={“color”: BLUE})
sine_wave = axes.plot(lambda x: np.sin(x), color=YELLOW)
self.play(Create(axes), Create(sine_wave))
“`
- Render the Animation:
Use the command line to run the script:
“`bash
manim -pql your_script.py SineWave
“`
This will generate a sine wave animation, showcasing Manim’s capabilities for creating complex visualizations.
Expert Insights on Creating Animations in Python
Dr. Emily Carter (Computer Graphics Researcher, Tech Innovations Journal). “To create effective animations in Python, one must leverage libraries such as Pygame or Matplotlib. These tools provide robust frameworks for rendering graphics and handling user interactions, which are essential for dynamic animations.”
Michael Chen (Senior Software Engineer, Animation Studio Inc.). “Understanding the principles of animation, such as timing and easing, is crucial when programming animations in Python. By combining these principles with the capabilities of libraries like Manim, developers can produce visually appealing and smooth animations.”
Sarah Johnson (Educational Technology Specialist, LearnPython.org). “For beginners looking to make animations in Python, I recommend starting with simple projects using Turtle graphics. It offers an intuitive way to grasp the basics of animation while allowing for creativity and experimentation.”
Frequently Asked Questions (FAQs)
How can I create a simple animation in Python?
You can create a simple animation in Python using libraries like Pygame or Matplotlib. Pygame allows for real-time graphics and is suitable for game development, while Matplotlib can be used for plotting and animating data visually.
What libraries are recommended for making animations in Python?
Recommended libraries include Pygame for game-like animations, Matplotlib for data visualizations, and Manim for mathematical animations. Each library has its strengths depending on the type of animation you want to create.
Is it possible to create animations using only built-in Python libraries?
While Python’s standard library does not include specific animation tools, you can use the Turtle module for basic animations. However, for more complex animations, external libraries are advisable.
Can I use Python to create animations for web applications?
Yes, you can use libraries such as Plotly or Bokeh to create interactive animations for web applications. These libraries generate HTML and JavaScript, allowing for seamless integration into web environments.
What are the steps to animate a plot using Matplotlib?
To animate a plot using Matplotlib, you should import the necessary modules, create a figure and axes, define an update function for the animation, and then use the `FuncAnimation` class to generate the animation.
Are there any online resources or tutorials for learning animation in Python?
Yes, several online platforms offer tutorials for animation in Python, including official documentation for libraries like Pygame and Matplotlib, as well as video tutorials on platforms like YouTube and educational sites like Coursera or Udemy.
Creating animations in Python can be a rewarding endeavor, leveraging libraries such as Matplotlib, Pygame, or Manim to bring visual concepts to life. Each library offers unique functionalities, catering to different needs, whether for scientific visualization, game development, or mathematical demonstrations. Understanding the specific capabilities of these libraries is crucial for selecting the right tool for your animation project.
For instance, Matplotlib is particularly effective for creating 2D plots and animations, allowing users to visualize data dynamically. Pygame, on the other hand, is tailored for game development and provides extensive features for creating interactive animations. Manim, designed for mathematical animations, excels in producing high-quality educational videos. Familiarizing oneself with the syntax and functionality of these libraries is essential for efficient animation creation.
Additionally, mastering the principles of animation, such as timing, easing, and keyframes, can significantly enhance the quality of the final product. By combining technical skills with a solid understanding of animation principles, developers can create engaging and visually appealing animations. As Python continues to evolve, the community around these libraries grows, providing ample resources and support for both beginners and experienced developers alike.
Author Profile
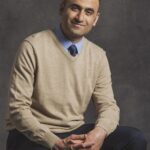
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?