How Can You Create a Circle in Python? A Step-by-Step Guide!
Creating shapes and graphics in Python can be a fun and rewarding experience, especially when it comes to drawing circles. Whether you’re a budding programmer looking to enhance your skills or an experienced developer exploring new libraries, understanding how to make a circle in Python opens up a world of possibilities. From simple visualizations to complex graphical applications, the ability to draw circles can serve as a fundamental building block for your projects.
In this article, we will explore various methods to create circles in Python, utilizing popular libraries such as Matplotlib, Pygame, and Turtle. Each of these libraries offers unique features and capabilities, allowing you to choose the best tool for your specific needs. We will delve into the basics of setting up your environment and provide step-by-step guidance on how to draw circles, including customizing their properties like color, size, and position.
By the end of this exploration, you will not only have a solid understanding of how to create circles in Python but also gain insights into how these shapes can be integrated into larger graphical projects. Whether you aim to create engaging visualizations, interactive games, or educational tools, mastering the art of drawing circles is an essential skill that will enhance your programming toolkit. Let’s get started on this exciting journey into the world of Python graphics!
Using the Turtle Graphics Library
The Turtle graphics library is a popular way to create drawings and shapes in Python. It is particularly user-friendly for beginners and allows for an intuitive way to create a circle through simple commands. To draw a circle using Turtle, you can follow these steps:
- Import the Turtle module: This is essential to access the Turtle graphics functions.
- Create a Turtle object: This object will control the drawing.
- Use the `circle()` method: This built-in method simplifies the process of drawing a circle.
Here is a sample code snippet that demonstrates how to create a circle using Turtle:
“`python
import turtle
Create a turtle object
my_turtle = turtle.Turtle()
Draw a circle with a specified radius
my_turtle.circle(100)
Finish drawing
turtle.done()
“`
In this example, a circle with a radius of 100 units is created. The `turtle.done()` function ensures that the window stays open until closed by the user.
Using Matplotlib to Plot a Circle
Matplotlib is a comprehensive library used for creating static, animated, and interactive visualizations in Python. You can plot a circle using Matplotlib by employing the `Circle` patch from the `matplotlib.patches` module. The following steps detail how to create a circle using Matplotlib:
- Import the necessary libraries: You’ll need to import `matplotlib.pyplot` and `matplotlib.patches`.
- Create a figure and an axis: This sets up the area where the circle will be drawn.
- Add a Circle patch to the axis: Specify the center and radius of the circle.
Here’s how you can implement this:
“`python
import matplotlib.pyplot as plt
import matplotlib.patches as patches
Create a figure and an axis
fig, ax = plt.subplots()
Create a Circle patch
circle = patches.Circle((0.5, 0.5), 0.3, color=’blue’, alpha=0.5)
Add the circle to the axes
ax.add_patch(circle)
Set limits and aspect
ax.set_xlim(0, 1)
ax.set_ylim(0, 1)
ax.set_aspect(‘equal’, adjustable=’box’)
Show the plot
plt.show()
“`
This code will generate a blue circle with a radius of 0.3, centered at the point (0.5, 0.5) within a unit square.
Creating a Circle Using Pygame
Pygame is a versatile library used for game development in Python. It allows for creating a variety of shapes, including circles. To draw a circle in Pygame, follow these steps:
- Initialize Pygame: This is necessary to set up the environment.
- Create a game window: Define the dimensions of the display.
- Use the `draw.circle()` method: This method draws a circle on the screen.
Below is an example demonstrating how to draw a circle with Pygame:
“`python
import pygame
import sys
Initialize Pygame
pygame.init()
Set up the display
window_size = (400, 400)
screen = pygame.display.set_mode(window_size)
Define the color
color = (255, 0, 0) Red
Main loop
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
Clear the screen
screen.fill((255, 255, 255)) White
Draw a circle
pygame.draw.circle(screen, color, (200, 200), 50) Center (200, 200), radius 50
Update the display
pygame.display.flip()
“`
In this example, a red circle with a radius of 50 pixels is drawn at the center of a 400×400 window. The loop continues until the window is closed by the user.
Library | Method to Draw Circle | Example Code Snippet |
---|---|---|
Turtle | my_turtle.circle(radius) | my_turtle.circle(100) |
Matplotlib | patches.Circle(center, radius) | patches.Circle((0.5, 0.5), 0.3) |
Pygame | pygame.draw.circle(surface, color, center, radius) | pygame.draw.circle(screen, (255, 0, 0), (200, 200), 50) |
Using Turtle Graphics to Draw a Circle
Python’s Turtle graphics module is an excellent way to create simple graphics, including circles. The following steps outline how to draw a circle using this module.
- Install Turtle Module: The Turtle module comes pre-installed with Python, so you usually don’t need to install it separately.
- Basic Circle Drawing Code:
“`python
import turtle
Create a turtle object
my_turtle = turtle.Turtle()
Draw a circle with a radius of 100
my_turtle.circle(100)
Finish
turtle.done()
“`
This code snippet initializes a turtle graphics window, creates a turtle object, and commands it to draw a circle with a specified radius.
Using Matplotlib to Plot a Circle
Matplotlib is a widely used library for creating static, animated, and interactive visualizations in Python. To plot a circle, follow these steps:
- Installation: Ensure you have Matplotlib installed. You can install it via pip:
“`bash
pip install matplotlib
“`
- Circle Plotting Code:
“`python
import matplotlib.pyplot as plt
import numpy as np
Create an array of angles
theta = np.linspace(0, 2*np.pi, 100)
Circle coordinates
x = np.cos(theta)
y = np.sin(theta)
Plotting the circle
plt.figure(figsize=(6,6))
plt.plot(x, y)
plt.xlim(-1.5, 1.5)
plt.ylim(-1.5, 1.5)
plt.gca().set_aspect(‘equal’)
plt.title(‘Circle with radius 1’)
plt.grid()
plt.show()
“`
This code creates a circle centered at the origin with a radius of 1 by using parametric equations.
Creating Circles with Pygame
Pygame is a set of Python modules designed for writing video games but can also be used for graphical applications. Here’s how to create a circle using Pygame:
- Installation: Install Pygame with pip:
“`bash
pip install pygame
“`
- Circle Drawing Code:
“`python
import pygame
import sys
Initialize Pygame
pygame.init()
Set up display
screen = pygame.display.set_mode((400, 400))
Color
white = (255, 255, 255)
blue = (0, 0, 255)
Main loop
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
Fill background
screen.fill(white)
Draw a circle
pygame.draw.circle(screen, blue, (200, 200), 100)
Update display
pygame.display.flip()
“`
This code initializes a Pygame window and draws a blue circle at the center.
Circle Drawing Using NumPy and Matplotlib
For more mathematical approaches, NumPy can be combined with Matplotlib to draw circles based on polar coordinates.
- Circle Drawing Code:
“`python
import numpy as np
import matplotlib.pyplot as plt
Circle parameters
radius = 1
theta = np.linspace(0, 2 * np.pi, 100)
Circle coordinates
x = radius * np.cos(theta)
y = radius * np.sin(theta)
Plotting the circle
plt.plot(x, y)
plt.axis(‘equal’)
plt.title(‘Circle with radius 1’)
plt.grid()
plt.show()
“`
Using NumPy allows for efficient calculations and is particularly useful for handling large datasets or complex mathematical shapes.
Summary of Circle Drawing Methods
Method | Library | Code Complexity | Use Case |
---|---|---|---|
Turtle | Turtle | Simple | Educational graphics |
Matplotlib | Matplotlib | Moderate | Data visualization |
Pygame | Pygame | Moderate | Game development |
NumPy + Matplotlib | NumPy & Matplotlib | Moderate | Mathematical modeling and visualizations |
Expert Insights on Creating Circles in Python
Dr. Emily Carter (Computer Graphics Specialist, Tech Innovations Journal). “Creating circles in Python can be efficiently accomplished using libraries like Matplotlib and Pygame. These libraries provide built-in functions that simplify the drawing process, allowing developers to focus on the creative aspects of their projects rather than the underlying mathematics.”
James Lin (Software Engineer, Data Visualization Expert). “When working with Python, utilizing the Turtle graphics library is a great way to create circles interactively. The simplicity of Turtle commands makes it an excellent choice for beginners looking to understand basic programming concepts while visualizing geometric shapes.”
Dr. Sarah Thompson (Educational Technology Consultant, Code for Kids). “For educational purposes, teaching students how to draw circles in Python using the Pygame library not only introduces them to game development but also reinforces their understanding of coordinates and shapes. This hands-on approach can significantly enhance learning outcomes in programming classes.”
Frequently Asked Questions (FAQs)
How can I draw a circle using the Turtle module in Python?
You can draw a circle using the Turtle module by creating a Turtle object and calling the `circle()` method. For example:
“`python
import turtle
t = turtle.Turtle()
t.circle(50)
turtle.done()
“`
What libraries can I use to create circles in Python?
Common libraries for creating circles in Python include Turtle, Matplotlib, and Pygame. Each library offers different functionalities and is suited for various applications, such as graphics, data visualization, or game development.
How do I create a filled circle in Matplotlib?
To create a filled circle in Matplotlib, use the `Circle` class from the `matplotlib.patches` module and add it to an Axes object. Example:
“`python
import matplotlib.pyplot as plt
import matplotlib.patches as patches
fig, ax = plt.subplots()
circle = patches.Circle((0.5, 0.5), 0.1, color=’blue’)
ax.add_patch(circle)
plt.xlim(0, 1)
plt.ylim(0, 1)
plt.gca().set_aspect(‘equal’)
plt.show()
“`
Can I create a circle using Pygame?
Yes, you can create a circle in Pygame using the `draw.circle()` function. Specify the surface, color, center coordinates, and radius. Example:
“`python
import pygame
pygame.init()
screen = pygame.display.set_mode((400, 400))
pygame.draw.circle(screen, (255, 0, 0), (200, 200), 50)
pygame.display.flip()
“`
What parameters do I need to specify to draw a circle in Python?
When drawing a circle, you typically need to specify the center coordinates (x, y), the radius of the circle, and optionally the color and fill style depending on the library used.
Is it possible to animate a circle in Python?
Yes, you can animate a circle in Python using libraries like Pygame or Matplotlib. You can update the circle’s position or properties in a loop to create an animation effect.
In Python, creating a circle can be accomplished using various libraries, each suited for different applications. The most common libraries include Matplotlib for plotting, Pygame for game development, and Turtle for educational purposes. Each library provides unique methods and functionalities to draw circles, allowing developers to choose the one that best fits their project requirements.
When using Matplotlib, the `Circle` class from the `patches` module is a straightforward way to create and display a circle on a plot. Pygame, on the other hand, allows for dynamic circle rendering in a game environment using the `draw.circle()` method. Turtle graphics is particularly user-friendly for beginners, enabling simple commands to draw shapes, including circles, with ease. Understanding the context and requirements of your project will guide you in selecting the most appropriate library.
In summary, making a circle in Python is a versatile task that can be achieved through multiple libraries, each offering distinct advantages. Familiarity with these libraries not only enhances your programming skills but also broadens your ability to visualize and interact with graphical content effectively. By leveraging the right tools, you can create visually appealing graphics and applications that incorporate circles seamlessly.
Author Profile
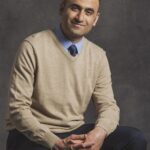
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?