How Can You Create a Class in Python?
### Introduction
In the world of programming, the ability to create and manipulate classes is a fundamental skill that can elevate your coding prowess to new heights. Python, known for its simplicity and readability, offers a powerful object-oriented programming paradigm that allows developers to model real-world entities through classes. Whether you’re building a complex application or just starting your programming journey, understanding how to make a class in Python is essential. This article will guide you through the process, unlocking the potential of encapsulation, inheritance, and polymorphism in your projects.
Creating a class in Python is not just about defining a blueprint for objects; it’s about structuring your code in a way that enhances reusability and maintainability. By encapsulating data and functionality within classes, you can create more organized and efficient programs. This overview will introduce the basic concepts of classes, including attributes and methods, and explain how they contribute to the overall architecture of your code.
As we delve deeper into the topic, you will discover the nuances of class creation, from defining attributes to implementing methods that bring your objects to life. We will explore key principles of object-oriented programming, enabling you to harness the full power of Python’s class system. Whether you’re a novice eager to learn or an experienced programmer looking to refine your skills, this
Defining a Class
To create a class in Python, you use the `class` keyword followed by the class name and a colon. The class name should follow the CamelCase naming convention. Here’s a basic structure:
python
class ClassName:
pass
The `pass` statement is a placeholder indicating that the class does not contain any content yet. Once defined, you can add attributes and methods to the class.
Attributes and Methods
Attributes are variables that hold data about the class, while methods are functions that define the behaviors of the class. You typically initialize attributes in the `__init__` method, which is the constructor for the class. Here’s an example:
python
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
def bark(self):
return “Woof!”
In this example:
- `name` and `age` are attributes initialized via the constructor.
- `bark` is a method that returns a string.
Using the `self` Keyword
The `self` keyword is crucial in class definitions. It refers to the instance of the class itself, allowing you to access variables and methods associated with the instance.
Here’s how you can create an instance of the class and call its methods:
python
my_dog = Dog(“Buddy”, 3)
print(my_dog.name) # Output: Buddy
print(my_dog.bark()) # Output: Woof!
Class Inheritance
Inheritance allows one class to inherit attributes and methods from another class. This promotes code reuse and establishes a hierarchy. To create a subclass, simply define a new class that specifies the parent class in parentheses.
python
class Puppy(Dog):
def wag_tail(self):
return “Wagging tail!”
The `Puppy` class inherits from the `Dog` class and can use its methods and attributes while also introducing new behaviors.
Access Modifiers
Python does not have strict access modifiers like some other languages but uses naming conventions to indicate the intended level of access:
- Public: Attributes and methods that can be accessed from outside the class (e.g., `self.name`).
- Protected: Attributes that should not be accessed directly outside the class (e.g., `_protected_attr`).
- Private: Attributes that cannot be accessed directly outside the class (e.g., `__private_attr`).
Class Example Overview
Here’s a summary of the class creation elements:
Element | Description |
---|---|
Class Definition | Defines a new class using the `class` keyword. |
Constructor (`__init__`) | Initializes attributes for the class instance. |
Methods | Defines behaviors for the class. |
Inheritance | Allows a class to inherit attributes and methods from another class. |
By following these guidelines, you can effectively create and utilize classes in Python, leveraging the principles of object-oriented programming to build robust applications.
Defining a Class
In Python, a class is defined using the `class` keyword followed by the class name and a colon. The class body contains attributes and methods that define the behavior and properties of the objects created from the class.
python
class ClassName:
# class attributes
attribute_name = value
def method_name(self):
# method body
pass
Creating Attributes
Attributes are variables that hold data related to a class. They can be defined in two primary ways: class attributes and instance attributes.
- Class Attributes: Shared across all instances of the class.
- Instance Attributes: Specific to each instance, typically defined within the `__init__` method.
Example of defining both types:
python
class Dog:
species = “Canis familiaris” # Class attribute
def __init__(self, name, age):
self.name = name # Instance attribute
self.age = age # Instance attribute
Methods in a Class
Methods are functions defined within a class that operate on its attributes. The first parameter of a method is typically `self`, which refers to the instance of the class.
- Instance Methods: Operate on instance attributes.
- Class Methods: Use the `@classmethod` decorator and take `cls` as the first parameter.
- Static Methods: Use the `@staticmethod` decorator and do not take `self` or `cls` as a parameter.
Example of different method types:
python
class Dog:
def __init__(self, name):
self.name = name
def bark(self): # Instance method
return f”{self.name} says woof!”
@classmethod
def species_info(cls): # Class method
return f”All dogs are of the species {cls.species}.”
@staticmethod
def common_breed(): # Static method
return “Labrador is a common breed.”
Creating an Instance
An instance (or object) of a class is created by calling the class as if it were a function. This invokes the `__init__` method to initialize the object.
Example of creating an instance and accessing its attributes and methods:
python
my_dog = Dog(“Buddy”)
print(my_dog.bark()) # Outputs: Buddy says woof!
print(Dog.species_info()) # Outputs: All dogs are of the species Canis familiaris.
Inheritance
Inheritance allows a class (child) to inherit attributes and methods from another class (parent). This promotes code reuse and establishes a hierarchical relationship.
Example of inheritance:
python
class Puppy(Dog): # Puppy inherits from Dog
def play(self):
return f”{self.name} is playing!”
my_puppy = Puppy(“Charlie”)
print(my_puppy.bark()) # Inherited method
print(my_puppy.play()) # Outputs: Charlie is playing!
Encapsulation
Encapsulation is the concept of restricting access to certain components of an object. This is done by using private attributes and methods by prefixing them with an underscore.
Example of encapsulation:
python
class BankAccount:
def __init__(self, balance):
self.__balance = balance # Private attribute
def deposit(self, amount):
self.__balance += amount
def get_balance(self):
return self.__balance # Public method to access private attribute
Polymorphism
Polymorphism allows methods to do different things based on the object that it is acting upon. It can be achieved through method overriding in derived classes.
Example of polymorphism:
python
class Cat(Dog): # Cat inherits from Dog
def bark(self): # Method overriding
return f”{self.name} says meow!”
my_cat = Cat(“Whiskers”)
print(my_cat.bark()) # Outputs: Whiskers says meow!
This structured approach to creating classes in Python establishes a foundation for building more complex and organized code.
Expert Insights on Creating Classes in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). Creating a class in Python is fundamental to object-oriented programming. It allows for encapsulation of data and functions, enabling more organized and reusable code. When defining a class, it is essential to understand the role of the `__init__` method, which serves as the constructor to initialize object attributes.
Michael Chen (Lead Python Developer, CodeMasters). To effectively create a class in Python, one must grasp the concepts of inheritance and polymorphism. These principles enhance code flexibility and maintainability. By leveraging these features, developers can create a hierarchy of classes that share common functionality while allowing for specific implementations.
Sarah Johnson (Python Educator, LearnPython Academy). When teaching how to make a class in Python, I emphasize the importance of clear naming conventions and documentation. A well-defined class should not only encapsulate data but also provide methods that are intuitive and easy to understand. This practice not only aids in code readability but also fosters collaboration among developers.
Frequently Asked Questions (FAQs)
How do I define a class in Python?
To define a class in Python, use the `class` keyword followed by the class name and a colon. For example:
python
class MyClass:
pass
What is the purpose of the `__init__` method in a class?
The `__init__` method is a special method called a constructor. It initializes the object’s attributes when an instance of the class is created. It allows you to set initial values for the object.
How do I create an instance of a class?
To create an instance of a class, call the class name followed by parentheses. For example:
python
my_instance = MyClass()
Can a class in Python inherit from another class?
Yes, a class can inherit from another class in Python. This is done by specifying the parent class in parentheses. For example:
python
class ChildClass(ParentClass):
pass
What are class attributes and instance attributes?
Class attributes are variables that belong to the class itself and are shared among all instances. Instance attributes are specific to each object and are defined within the `__init__` method.
How do I define methods within a class?
Methods are defined within a class using the `def` keyword, similar to functions. The first parameter should typically be `self`, which refers to the instance of the class. For example:
python
class MyClass:
def my_method(self):
pass
In Python, creating a class is a fundamental aspect of object-oriented programming that allows for the encapsulation of data and behavior. A class serves as a blueprint for creating objects, defining the properties (attributes) and methods (functions) that characterize the objects instantiated from it. The basic syntax involves using the `class` keyword followed by the class name, with an optional inheritance from a parent class. Within the class, attributes can be defined in the `__init__` method, which acts as the constructor, initializing the object’s state when it is created.
Furthermore, classes in Python can include various methods that define the actions that can be performed on the objects. These methods can manipulate the object’s attributes and provide functionality. In addition to instance methods, Python supports class methods and static methods, which offer different scopes of access to the class and its attributes. By utilizing encapsulation, inheritance, and polymorphism, Python classes promote code reusability and modular design, making it easier to manage complex programs.
In summary, mastering the creation and utilization of classes in Python is essential for any developer looking to leverage the power of object-oriented programming. By understanding the structure and functionality of classes, developers can create more organized, efficient, and
Author Profile
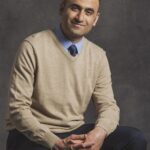
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?