How Can You Create a Class in Python?
In the world of programming, the ability to create and utilize classes is a fundamental skill that can elevate your coding from basic scripts to sophisticated, object-oriented applications. Python, known for its simplicity and readability, offers a powerful yet intuitive approach to defining classes. Whether you’re a novice eager to learn the ropes or an experienced developer looking to refine your skills, understanding how to make a class in Python is an essential step in your programming journey. This article will guide you through the core concepts and practical applications of classes, empowering you to harness the full potential of object-oriented programming.
Overview
At its core, a class in Python serves as a blueprint for creating objects, encapsulating data and behaviors that define the characteristics and functionalities of those objects. By grouping related properties and methods, classes allow for organized and reusable code, making your programs easier to maintain and expand. This encapsulation not only promotes code clarity but also fosters a modular approach to software development, where complex systems can be broken down into manageable components.
Creating a class involves defining its attributes and methods, which dictate how the class behaves and interacts with other parts of your program. As you delve deeper into the world of classes, you’ll discover concepts such as inheritance, encapsulation, and polymorphism, which further enhance
Defining a Class in Python
To create a class in Python, the `class` keyword is utilized followed by the name of the class, adhering to the naming conventions (typically CamelCase). The class can include attributes (variables) and methods (functions) that define the behaviors and properties of the objects instantiated from it. Below is a simple example:
“`python
class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
def bark(self):
return “Woof!”
“`
In this example, the `Dog` class has an initializer method `__init__`, which sets the initial state of the object with the attributes `name` and `age`. The method `bark` is an instance method that defines a behavior of the `Dog` class.
Creating an Object
Once a class is defined, you can create objects (instances) of that class. This is done by calling the class name as if it were a function. Here’s how you can instantiate the `Dog` class:
“`python
my_dog = Dog(“Buddy”, 3)
“`
Now, `my_dog` is an object of the `Dog` class with the attributes `name` set to “Buddy” and `age` set to 3.
Class Attributes and Instance Attributes
Attributes in a class can be classified as either class attributes or instance attributes. Class attributes are shared across all instances of the class, while instance attributes are unique to each instance.
- Class Attributes: Defined directly within the class definition, outside any methods.
- Instance Attributes: Defined within methods using `self`.
Example:
“`python
class Dog:
species = “Canis familiaris” Class attribute
def __init__(self, name, age):
self.name = name Instance attribute
self.age = age Instance attribute
“`
In this case, `species` is a class attribute that is common to all `Dog` instances.
Methods in a Class
Methods are functions defined within a class that operate on instances of that class. The first parameter is always `self`, which refers to the instance itself. Here’s a breakdown of different types of methods:
Method Type | Description |
---|---|
Instance Method | Operates on an instance of the class. |
Class Method | Operates on the class itself, defined with `@classmethod`. |
Static Method | Does not operate on an instance or class, defined with `@staticmethod`. |
Example of a class method and static method:
“`python
class Dog:
species = “Canis familiaris”
@classmethod
def get_species(cls):
return cls.species
@staticmethod
def info():
return “Dogs are domesticated mammals.”
“`
Inheritance in Classes
Inheritance allows a class to inherit attributes and methods from another class. The class that inherits is called the child class, and the class it inherits from is called the parent class. This promotes code reusability.
Example of inheritance:
“`python
class Animal:
def speak(self):
return “Animal sound”
class Dog(Animal):
def bark(self):
return “Woof!”
“`
In this example, the `Dog` class inherits from the `Animal` class, allowing it to use the `speak` method.
Encapsulation and Access Modifiers
Encapsulation restricts access to certain components of an object. In Python, this is typically done using naming conventions:
- Public: Attributes and methods that are accessible from outside the class (default).
- Protected: Attributes and methods that should not be accessed from outside the class, indicated by a single underscore (e.g., `_attribute`).
- Private: Attributes and methods that are not accessible from outside the class, indicated by a double underscore (e.g., `__attribute`).
This encapsulation allows for better control over how data is accessed and modified, enhancing security and integrity.
Defining a Class in Python
In Python, a class is defined using the `class` keyword followed by the class name and a colon. The body of the class contains attributes and methods, which define the properties and behaviors of the class.
“`python
class ClassName:
Class attributes
attribute1 = value1
attribute2 = value2
def method_name(self):
Method body
pass
“`
Creating an Instance of a Class
An instance of a class is created by calling the class name as if it were a function. This instantiation allows access to the class’s attributes and methods.
“`python
instance = ClassName()
“`
To access attributes and methods, use the dot notation:
“`python
instance.attribute1
instance.method_name()
“`
Understanding the Constructor Method
The constructor method, `__init__`, is a special method that initializes new objects. It is called automatically when a new instance of the class is created.
“`python
class ClassName:
def __init__(self, param1, param2):
self.attribute1 = param1
self.attribute2 = param2
“`
Here, `self` refers to the instance being created and allows the instance to access its own attributes.
Defining Methods in a Class
Methods are functions defined inside a class. They can manipulate the object’s state and perform actions. Every method in a class must have at least one parameter, `self`, which represents the instance calling the method.
“`python
class ClassName:
def method1(self):
Perform an action
pass
def method2(self, param):
Use the parameter
return self.attribute1 + param
“`
Class Inheritance
Inheritance allows a class to inherit attributes and methods from another class, promoting code reuse. The derived class can override or extend the functionality of the base class.
“`python
class BaseClass:
def base_method(self):
return “This is the base method.”
class DerivedClass(BaseClass):
def derived_method(self):
return “This is the derived method.”
“`
Class Attributes vs. Instance Attributes
Attributes can be classified into class attributes and instance attributes. Class attributes are shared among all instances, while instance attributes are unique to each instance.
Attribute Type | Definition | Example |
---|---|---|
Class Attribute | Shared across all instances | `ClassName.class_attr = 0` |
Instance Attribute | Unique to each instance | `self.instance_attr = 1` |
Encapsulation in Classes
Encapsulation is a fundamental principle of object-oriented programming that restricts direct access to some of an object’s components. This is typically achieved through private and protected attributes.
“`python
class ClassName:
def __init__(self):
self.__private_attr = “I am private”
def get_private_attr(self):
return self.__private_attr
“`
In this example, `__private_attr` is not accessible from outside the class, promoting data protection.
Polymorphism in Classes
Polymorphism allows methods to do different things based on the object it is acting upon, enhancing flexibility and the ability to extend functionality.
“`python
class BaseClass:
def speak(self):
return “Base class speaking”
class DerivedClass(BaseClass):
def speak(self):
return “Derived class speaking”
def make_speak(instance):
print(instance.speak())
make_speak(BaseClass()) Output: Base class speaking
make_speak(DerivedClass()) Output: Derived class speaking
“`
This demonstrates how different classes can define methods with the same name but with different implementations.
Expert Insights on Creating Classes in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When creating a class in Python, it is essential to understand the principles of object-oriented programming. Start by defining the class using the ‘class’ keyword, followed by the class name and a colon. This establishes the blueprint for your objects, allowing you to encapsulate data and functions effectively.”
Mark Thompson (Lead Python Developer, CodeMaster Solutions). “A well-structured class in Python should include an initializer method, known as ‘__init__’, which allows you to set initial values for object attributes. This method is crucial for ensuring that your objects are created with the necessary state and behavior right from the start.”
Lisa Nguyen (Python Programming Instructor, Online Learning Academy). “In addition to defining attributes and methods, it is important to implement proper inheritance and polymorphism when creating classes in Python. These concepts enhance code reusability and flexibility, allowing you to build more complex systems with less redundancy.”
Frequently Asked Questions (FAQs)
How do I define a class in Python?
To define a class in Python, use the `class` keyword followed by the class name and a colon. For example:
“`python
class MyClass:
pass
“`
What is the purpose of the `__init__` method in a Python class?
The `__init__` method is a special method that initializes a new object of the class. It is called automatically when a new instance is created, allowing you to set initial values for attributes.
How can I create attributes for a class in Python?
Attributes can be created within the `__init__` method using the `self` keyword. For example:
“`python
class MyClass:
def __init__(self, value):
self.attribute = value
“`
What is inheritance in Python classes?
Inheritance allows a class to inherit attributes and methods from another class, known as the parent or base class. This promotes code reuse and establishes a hierarchical relationship between classes.
How do I create methods in a Python class?
Methods are defined within a class using the `def` keyword, just like regular functions. They must include `self` as the first parameter to access instance attributes. For example:
“`python
class MyClass:
def my_method(self):
print(“Hello, World!”)
“`
Can I create class-level attributes in Python?
Yes, class-level attributes are defined directly within the class body, outside of any methods. They are shared among all instances of the class. For example:
“`python
class MyClass:
class_attribute = “I am a class attribute”
“`
In summary, creating a class in Python involves defining a blueprint for objects that encapsulates data and functions. The process begins with the use of the ‘class’ keyword, followed by the class name and a colon. Within the class, attributes can be initialized using the ‘__init__’ method, which serves as the constructor. This method allows for the setting of initial values for object properties, enabling the creation of instances with specific characteristics.
Furthermore, classes can include methods that define the behaviors of the objects. These methods are functions defined within the class that can manipulate the object’s attributes or perform actions relevant to the object. Inheritance can also be utilized to create subclasses that extend the functionality of existing classes, promoting code reuse and organization.
Key takeaways include the importance of understanding the principles of object-oriented programming, such as encapsulation, inheritance, and polymorphism. Mastery of these concepts enhances the ability to design robust and scalable applications. Additionally, leveraging Python’s built-in features, such as decorators and properties, can further enrich class functionality and improve code maintainability.
Author Profile
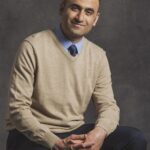
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?