How Can You Make a Copy of a List in Python?
In the world of Python programming, lists are a fundamental data structure that allows developers to store and manipulate collections of items. Whether you’re managing a simple list of names or handling complex data sets, knowing how to create a copy of a list is crucial for effective coding. Copying lists can help prevent unintended changes to your original data, ensuring that your programs run smoothly and as intended. In this article, we will explore various methods to make copies of lists in Python, empowering you with the knowledge to manage your data efficiently and safely.
When working with lists in Python, it’s essential to understand the difference between copying a list and merely referencing it. A shallow copy creates a new list object containing references to the original list’s elements, while a deep copy duplicates the entire structure, including nested objects. This distinction can have significant implications for your code, especially when dealing with mutable objects. By mastering these concepts, you’ll be better equipped to handle data manipulation without the risk of unintentional side effects.
Throughout this article, we will delve into the various techniques available for copying lists in Python, from simple methods to more advanced strategies. By the end, you’ll have a comprehensive understanding of how to create copies of lists effectively, allowing you to write cleaner, more reliable
Methods to Copy a List in Python
In Python, there are several methods to create a copy of a list, each with its unique characteristics and use cases. Understanding these methods is crucial for managing data effectively and avoiding unintended side effects, particularly when dealing with mutable objects.
Using the `copy()` Method
The `copy()` method is a straightforward way to create a shallow copy of a list. It returns a new list containing the elements of the original list, but it does not copy nested objects.
“`python
original_list = [1, 2, 3, 4]
copied_list = original_list.copy()
“`
This method is particularly useful when you want to maintain the integrity of the original list while making modifications to the copy.
Using Slicing
Another common technique for copying a list in Python is through slicing. This method effectively creates a shallow copy by using the slice notation.
“`python
copied_list = original_list[:]
“`
This approach is concise and efficient, making it a popular choice among Python developers.
Using the `list()` Constructor
The `list()` constructor can also be employed to create a new list from an existing iterable, including another list.
“`python
copied_list = list(original_list)
“`
This method is beneficial for converting other iterable types, such as tuples or sets, into a list format.
Using List Comprehension
List comprehension provides a flexible way to copy a list while allowing for modifications to the elements during the copying process.
“`python
copied_list = [item for item in original_list]
“`
This method is advantageous when you need to apply transformations to the elements while copying.
Deep Copying with the `copy` Module
For complex lists containing nested objects, a shallow copy may not suffice. In such cases, using the `copy` module’s `deepcopy()` function ensures that all levels of nested objects are duplicated.
“`python
import copy
original_list = [[1, 2, 3], [4, 5, 6]]
deep_copied_list = copy.deepcopy(original_list)
“`
This method is essential when dealing with mutable objects within lists, as it prevents changes in the copied list from affecting the original list.
Comparison of List Copying Methods
The following table summarizes the different methods of copying lists in Python, highlighting their characteristics:
Method | Type of Copy | Use Case |
---|---|---|
copy() | Shallow | Simple list copying |
Slicing | Shallow | Concise and efficient copying |
list() | Shallow | Copying from various iterables |
List Comprehension | Shallow | Transformative copying |
deepcopy() | Deep | Complex lists with nested objects |
Each method has its appropriate application depending on the structure of the list and the desired outcome. Selecting the right copying method is essential for effective data manipulation in Python.
Methods to Create a Copy of a List in Python
In Python, there are several effective techniques for creating a copy of a list. Each method has its own use cases and performance implications. Below are the most common approaches.
Using the `list()` Constructor
The `list()` constructor can be utilized to create a shallow copy of a list. This method is straightforward and efficient for most scenarios.
“`python
original_list = [1, 2, 3, 4]
copied_list = list(original_list)
“`
Using the `copy()` Method
The `copy()` method is another intuitive approach to copy a list. It returns a shallow copy of the list, similar to the `list()` constructor.
“`python
original_list = [1, 2, 3, 4]
copied_list = original_list.copy()
“`
Using Slicing
Slicing is a Pythonic way to create a copy of a list. By specifying the entire range of the list, a new list is generated.
“`python
original_list = [1, 2, 3, 4]
copied_list = original_list[:]
“`
Using List Comprehension
List comprehension can also be employed to create a copy, although it is typically used for generating modified lists. Still, it serves as an effective method to copy a list.
“`python
original_list = [1, 2, 3, 4]
copied_list = [item for item in original_list]
“`
Using the `copy` Module for Deep Copies
When dealing with nested lists, a shallow copy might not suffice. The `copy` module provides a method for creating deep copies, which ensure that all elements and sub-elements are duplicated.
“`python
import copy
original_list = [[1, 2], [3, 4]]
copied_list = copy.deepcopy(original_list)
“`
Comparison of Methods
Method | Shallow Copy | Deep Copy | Performance | Use Case |
---|---|---|---|---|
`list()` Constructor | Yes | No | Fast | Basic list copying |
`copy()` Method | Yes | No | Fast | Basic list copying |
Slicing | Yes | No | Fast | Basic list copying |
List Comprehension | Yes | No | Fast | Copying while potentially modifying |
`copy.deepcopy()` | No | Yes | Slower | Nested lists or complex objects |
Each method serves different needs depending on the structure of the data being copied. When performance is a critical factor, prefer the simpler methods. For nested data structures, opt for `copy.deepcopy()` to ensure the integrity of the copied data.
Expert Insights on Copying Lists in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Creating a copy of a list in Python can be efficiently achieved using the list slicing method. By using `new_list = old_list[:]`, you create a shallow copy that is independent of the original list, which is crucial for maintaining data integrity in complex applications.”
Michael Chen (Software Engineer, Data Solutions Corp.). “For those who require a deep copy of a list—especially when dealing with nested lists—utilizing the `copy` module with `import copy` and `new_list = copy.deepcopy(old_list)` is essential. This ensures that all levels of the list are duplicated, preventing unintended modifications to the original list.”
Sarah Thompson (Python Educator, Code Academy). “Understanding the difference between shallow and deep copies is fundamental for Python programmers. While methods like `list()` or `copy()` can create shallow copies, it is vital to choose the appropriate method based on the data structure’s complexity to avoid issues with mutable objects.”
Frequently Asked Questions (FAQs)
How can I create a shallow copy of a list in Python?
You can create a shallow copy of a list in Python using the `list()` constructor or slicing. For example, `new_list = list(original_list)` or `new_list = original_list[:]` will create a shallow copy.
What is the difference between a shallow copy and a deep copy in Python?
A shallow copy creates a new list object but inserts references to the original objects. In contrast, a deep copy creates a new list and recursively copies all objects found in the original list, resulting in completely independent objects.
How do I make a deep copy of a list in Python?
To create a deep copy of a list, use the `copy` module’s `deepcopy()` function. For example, `import copy; new_list = copy.deepcopy(original_list)` will create a deep copy of the original list.
Can I use the `copy()` method to duplicate a list?
Yes, the `copy()` method can be used to create a shallow copy of a list. For example, `new_list = original_list.copy()` achieves the same result as slicing or using the `list()` constructor.
What happens if I modify a shallow copied list?
Modifying a shallow copied list will not affect the original list if you change the list structure itself (e.g., adding or removing elements). However, if you modify mutable objects within the copied list, those changes will reflect in the original list since they reference the same objects.
Is it necessary to use `copy()` or `deepcopy()` for copying lists?
Using `copy()` or `deepcopy()` is not strictly necessary for copying lists, but it is recommended for clarity and to avoid unintended side effects, especially when dealing with mutable objects.
In Python, creating a copy of a list is a fundamental operation that can be accomplished using several methods. The most common techniques include using the `list()` function, the slicing method, and the `copy()` method available in the list class. Each of these methods effectively creates a new list that is a duplicate of the original, ensuring that modifications to the new list do not affect the original list.
Using the `list()` function is straightforward; it takes an iterable and returns a new list. The slicing method, which involves using the syntax `original_list[:]`, is another efficient way to create a shallow copy. Additionally, the `copy()` method, introduced in Python 3.3, provides a clear and explicit way to create a copy of a list. It is important to note that these methods create shallow copies, meaning that if the list contains mutable objects, changes to those objects will reflect in both lists.
Understanding the differences between shallow and deep copies is crucial when working with lists that contain nested structures. For situations requiring a complete duplication of nested lists, the `copy` module’s `deepcopy()` function should be utilized. This function ensures that all levels of the list are copied, preventing any unintended side effects from
Author Profile
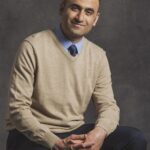
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?