How Can You Create Your Own GPS Tracker Using Python?
In an age where connectivity and location awareness have become integral to our daily lives, the ability to track and monitor movement has never been more relevant. Whether you’re looking to keep tabs on your belongings, enhance your outdoor adventures, or simply explore the fascinating world of geolocation technology, creating your own GPS tracker with Python presents an exciting challenge. This article will guide you through the essential concepts and tools needed to embark on this innovative project, merging the realms of programming and real-time tracking.
GPS tracking technology has evolved significantly, making it accessible to hobbyists and developers alike. By leveraging Python, a versatile and user-friendly programming language, you can tap into various libraries and APIs that simplify the process of gathering and interpreting location data. From understanding the basics of GPS signals to integrating hardware components, this project will not only bolster your coding skills but also provide you with a practical application of technology in everyday life.
As we delve deeper into the intricacies of building a GPS tracker, you’ll discover the essential components required, including GPS modules, microcontrollers, and the software necessary to process and display location data. With a blend of creativity and technical know-how, you’ll be well on your way to developing a functional GPS tracker that can be customized to meet your specific needs.
Setting Up the Environment
To begin creating a GPS tracker with Python, you will need to set up your development environment. This includes installing the necessary libraries and tools. The following steps will guide you through this process:
- Install Python: Ensure you have Python installed on your system. You can download it from [python.org](https://www.python.org/).
- Install Required Libraries: Use pip to install libraries such as `gps3`, `Flask`, and `requests`. Run the following commands in your terminal or command prompt:
“`
pip install gps3 Flask requests
“`
- Set Up a Virtual Environment (optional): It’s often a good idea to create a virtual environment for your project to avoid conflicts with other projects. You can do this with:
“`
python -m venv gps_tracker_env
source gps_tracker_env/bin/activate On Windows use gps_tracker_env\Scripts\activate
“`
Connecting to the GPS Module
To track GPS data, you’ll need to connect to a GPS module. Many use the `gps3` library for this purpose. The following is a sample code snippet to establish a connection with the GPS device:
“`python
from gps3 import GPS3
gps_socket = GPS3()
data_stream = gps_socket.stream()
gps_socket.start()
while True:
data = data_stream.next()
if data:
print(data)
“`
This code initializes the GPS connection and continuously reads data, printing it to the console.
Processing GPS Data
Once you have established a connection, the next step is to process the GPS data. The data typically includes latitude, longitude, speed, and timestamp. Here’s how you can extract and process this data:
- Extracting Data: You can access specific GPS data fields as follows:
“`python
if ‘lat’ in data and ‘lon’ in data:
latitude = data[‘lat’]
longitude = data[‘lon’]
print(f”Latitude: {latitude}, Longitude: {longitude}”)
“`
- Storing Data: You may want to store the GPS data in a database or a file for later analysis. Consider using SQLite for simplicity:
“`python
import sqlite3
Create a database connection
conn = sqlite3.connect(‘gps_data.db’)
c = conn.cursor()
c.execute(‘CREATE TABLE IF NOT EXISTS location (latitude REAL, longitude REAL, timestamp DATETIME DEFAULT CURRENT_TIMESTAMP)’)
Insert data
c.execute(‘INSERT INTO location (latitude, longitude) VALUES (?, ?)’, (latitude, longitude))
conn.commit()
“`
Creating a Web Interface
To visualize the GPS data, you can create a web interface using Flask. Below is a simple setup to display the data on a web page:
- Flask Application Setup:
“`python
from flask import Flask, render_template
app = Flask(__name__)
@app.route(‘/’)
def index():
Fetch the latest GPS data from your database or source
c.execute(‘SELECT * FROM location ORDER BY timestamp DESC LIMIT 1’)
latest_location = c.fetchone()
return render_template(‘index.html’, location=latest_location)
if __name__ == “__main__”:
app.run(debug=True)
“`
- HTML Template (`index.html`):
“`html
Latest GPS Location
{% if location %}
Latitude: {{ location[0] }}
Longitude: {{ location[1] }}
Timestamp: {{ location[2] }}
{% else %}
No data available
{% endif %}
“`
Data Management and Visualization
For effective data management and visualization, consider the following options:
- Data Visualization Libraries: Use libraries such as `matplotlib` or `folium` to create maps or graphs to visualize the tracked locations.
- Periodic Updates: Implement a mechanism to periodically update the GPS data, ensuring you always have the latest information.
- Error Handling: Incorporate error handling to manage cases where the GPS signal is lost or data retrieval fails.
Feature | Description |
---|---|
Real-time Tracking | Continuously fetch and display GPS data. |
Data Storage | Store GPS data for historical analysis. |
Web Interface | Visualize location data through a web application. |
Essential Components for a GPS Tracker
To create a GPS tracker using Python, several key components are required. These components can either be hardware elements or software libraries.
- Hardware:
- GPS Module (e.g., Neo-6M, SIM808)
- Microcontroller (e.g., Raspberry Pi, Arduino)
- Power Supply (battery or USB)
- SIM Card (for cellular-based tracking)
- Optional: Enclosure for protection
- Software Libraries:
- `gpsd`: For handling GPS data
- `requests`: For HTTP requests (if sending data to a server)
- `Flask`: To create a web server for tracking data
Setting Up the Hardware
Connect the GPS module to your microcontroller. The following steps outline a typical setup using a Raspberry Pi:
- Wiring:
- Connect the GPS module’s TX pin to the RX pin on the Raspberry Pi.
- Connect the GPS module’s RX pin to the TX pin on the Raspberry Pi.
- Connect power and ground pins appropriately.
- Install Required Software:
“`bash
sudo apt-get update
sudo apt-get install gpsd gpsd-clients python-gps
“`
- Configure gpsd:
Edit the `/etc/default/gpsd` file to set the GPS device path (e.g., `/dev/ttyAMA0`).
Writing the Python Code
The following Python code snippet demonstrates how to read data from the GPS module and send it to a server.
“`python
import gps
import requests
import time
Initialize GPS
session = gps.gps(mode=gps.WATCH_ENABLE)
Define your server URL
server_url = “http://yourserver.com/track”
while True:
session.next() Wait for a new GPS reading
if session.fix.mode >= 2: Check if GPS has a fix
latitude = session.fix.latitude
longitude = session.fix.longitude
print(f”Latitude: {latitude}, Longitude: {longitude}”)
Send data to server
data = {‘latitude’: latitude, ‘longitude’: longitude}
response = requests.post(server_url, json=data)
print(f”Data sent, server responded with: {response.status_code}”)
time.sleep(1) Delay for a second
“`
Data Storage and Visualization
For storing and visualizing the GPS data, you can use a database and a web application. Here are some common solutions:
- Database Options:
- SQLite: For lightweight storage
- PostgreSQL: For advanced querying and scalability
- Web Frameworks:
- Flask or Django: To create a web interface to visualize the data on maps using libraries like Leaflet or Google Maps API.
Testing and Validation
After setting up the hardware and writing the code, testing is crucial. Follow these steps:
- Test GPS Signal:
- Ensure the GPS module has a clear view of the sky for optimal signal reception.
- Run the Script:
- Execute the Python script and monitor the output for GPS coordinates.
- Check Server Logs:
- Verify that the data is being received correctly on the server.
- Visualize Data:
- Create a simple web page to display the captured GPS coordinates on a map.
Enhancements and Additional Features
Once the basic tracker is functional, consider implementing these enhancements:
- Geofencing:
- Trigger alerts when entering or exiting predefined geographical boundaries.
- Battery Management:
- Implement power-saving features for extended battery life.
- Mobile Application:
- Develop a mobile app to receive real-time updates from the GPS tracker.
- Data Encryption:
- Secure GPS data transmission to protect user privacy.
By following these guidelines, you can effectively create a functional GPS tracker using Python and associated technologies.
Expert Insights on Creating a GPS Tracker with Python
Dr. Emily Carter (Lead Software Engineer, GeoTech Innovations). “Building a GPS tracker with Python is an excellent way to leverage the language’s simplicity and versatility. Utilizing libraries such as `geopy` for geolocation and `Flask` for creating a web interface can significantly streamline the development process.”
Mark Thompson (IoT Specialist, Smart Devices Inc.). “Incorporating Python into GPS tracking systems allows for rapid prototyping and testing. By using Raspberry Pi or Arduino with Python, developers can create affordable and efficient tracking solutions that can be scaled easily.”
Linda Chen (Data Scientist, Urban Mobility Research). “When developing a GPS tracker with Python, it’s crucial to consider data privacy and security. Implementing encryption and secure data transmission protocols will ensure that user location data remains confidential and protected.”
Frequently Asked Questions (FAQs)
What components are needed to make a GPS tracker with Python?
To create a GPS tracker with Python, you will need a GPS module (like the Neo-6M), a microcontroller (such as Arduino or Raspberry Pi), a power source, and a method for data transmission (like GSM or Wi-Fi). Additionally, you will require Python libraries such as `serial` for communication and `requests` for sending data to a server.
How do I connect a GPS module to a microcontroller?
Connect the GPS module to the microcontroller using the appropriate pins. Typically, the GPS module will have a TX (transmit) pin connected to the RX (receive) pin of the microcontroller and vice versa. Ensure that the power supply voltage matches the specifications of the GPS module.
What Python libraries are essential for developing a GPS tracker?
Key Python libraries for developing a GPS tracker include `pyserial` for serial communication with the GPS module, `requests` for making HTTP requests to send location data, and optionally `Flask` for creating a web server to display the GPS data.
How can I transmit GPS data to a server using Python?
You can transmit GPS data to a server by using the `requests` library to send HTTP POST requests containing the GPS coordinates. Ensure that your server is set up to receive and process the incoming data correctly.
What are some common applications for a GPS tracker built with Python?
Common applications include vehicle tracking, asset tracking, personal safety devices, and wildlife monitoring. These trackers can provide real-time location data and historical tracking information.
Is it possible to visualize GPS data on a map using Python?
Yes, you can visualize GPS data on a map using libraries such as `Folium` or `Matplotlib`. These libraries allow you to create interactive maps and plot the GPS coordinates for better analysis and presentation.
creating a GPS tracker with Python involves a combination of hardware and software components. The essential hardware typically includes a GPS module, a microcontroller (such as Raspberry Pi or Arduino), and a communication module (like GSM or Wi-Fi) to transmit location data. On the software side, Python libraries such as PySerial for serial communication, and Flask or Django for web frameworks can be utilized to develop the tracking application. By integrating these elements, users can effectively track and monitor locations in real-time.
Moreover, implementing a GPS tracker with Python allows for customization and scalability. Developers can add features such as geofencing, historical data logging, and alerts for specific locations. The flexibility of Python enables the integration of various APIs and services, enhancing the functionality of the tracker. This adaptability makes it suitable for diverse applications, from personal tracking to fleet management.
Key takeaways from the discussion include the importance of selecting the right hardware components, understanding the communication protocols, and leveraging Python’s extensive libraries for development. Additionally, thorough testing and validation of the tracking system are crucial to ensure accuracy and reliability. By following these guidelines, individuals can successfully create a robust GPS tracking solution using Python.
Author Profile
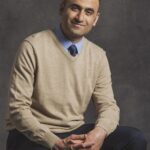
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?