How Can You Create a Media Player Using Python?
In an age where digital media consumption is at an all-time high, the ability to create your own media player can be both a rewarding and practical endeavor. Imagine having a personalized application that caters to your specific audio and video playback needs, all while honing your programming skills. Python, with its simplicity and robust libraries, offers an excellent platform for building a media player that can handle various formats and features. Whether you’re a novice coder eager to learn or a seasoned developer looking to expand your toolkit, this guide will illuminate the path to creating a functional media player in Python.
Creating a media player in Python involves understanding the fundamental components of audio and video playback, as well as the libraries that facilitate these processes. You’ll explore how to leverage popular libraries like Pygame, PyQt, or VLC bindings to manage media files, control playback, and even implement user interfaces. Each library provides unique capabilities and functionalities, allowing you to tailor your media player to your preferences and requirements.
As you embark on this journey, you’ll not only gain insights into multimedia programming but also develop problem-solving skills that are essential for any software development project. From handling user input to managing playback controls, the process of building a media player is an excellent way to deepen your understanding of Python and its capabilities.
Setting Up Your Environment
To create a media player in Python, you need to set up your development environment properly. This involves installing the necessary libraries and ensuring that your system meets the requirements for media playback.
- Python Installation: Ensure you have Python installed on your system. You can download it from the official Python website.
- Library Installation: Common libraries to facilitate media playback include:
- `pygame`: For handling audio and video.
- `tkinter`: For building the graphical user interface (GUI).
- `ffmpeg`: For handling various media formats.
You can install these libraries using pip:
“`bash
pip install pygame tk ffmpeg-python
“`
Creating the Basic GUI
The graphical user interface (GUI) is crucial for user interaction in your media player. Using `tkinter`, you can create a simple window with buttons for play, pause, and stop functionalities.
“`python
import tkinter as tk
from tkinter import filedialog
class MediaPlayer:
def __init__(self, root):
self.root = root
self.root.title(“Simple Media Player”)
self.create_widgets()
def create_widgets(self):
self.play_button = tk.Button(self.root, text=”Play”, command=self.play_media)
self.play_button.pack()
self.pause_button = tk.Button(self.root, text=”Pause”, command=self.pause_media)
self.pause_button.pack()
self.stop_button = tk.Button(self.root, text=”Stop”, command=self.stop_media)
self.stop_button.pack()
def play_media(self):
pass Implement play functionality
def pause_media(self):
pass Implement pause functionality
def stop_media(self):
pass Implement stop functionality
if __name__ == “__main__”:
root = tk.Tk()
player = MediaPlayer(root)
root.mainloop()
“`
Handling Media Playback
To manage the playback of media files, you can utilize `pygame` to handle audio and video playback. Here’s how you can integrate basic media handling functions:
- Load Media: Use `pygame.mixer` to load audio files.
- Play/Pause/Stop Functionality: Control playback using the mixer functions.
Here is an expanded example to include playback functionality:
“`python
import pygame
class MediaPlayer:
def __init__(self, root):
self.root = root
self.root.title(“Simple Media Player”)
self.create_widgets()
pygame.init()
pygame.mixer.init()
def create_widgets(self):
Same as above…
def play_media(self):
file_path = filedialog.askopenfilename()
if file_path:
pygame.mixer.music.load(file_path)
pygame.mixer.music.play()
def pause_media(self):
if pygame.mixer.music.get_busy():
pygame.mixer.music.pause()
def stop_media(self):
pygame.mixer.music.stop()
“`
Supported Formats and Compatibility
When developing a media player, it is important to be aware of the media formats you intend to support. Here’s a brief overview of common audio formats:
Format | Extension | Compatibility |
---|---|---|
MP3 | .mp3 | Widely Supported |
WAV | .wav | Widely Supported |
OGG | .ogg | Widely Supported |
FLAC | .flac | Limited Support |
It is advisable to test your player with various formats to ensure compatibility and smooth playback experiences. By using libraries like `ffmpeg`, you can enhance the format support of your media player significantly.
Choosing a Library for Media Playback
When developing a media player in Python, selecting the right library is crucial. The most commonly used libraries for audio and video playback include:
- Pygame: Best suited for basic audio functionalities and simple games.
- Pydub: Excellent for audio manipulation and conversion.
- vlc: A wrapper for the VLC media player, allowing extensive media format support.
- PyQt5: Useful for creating a GUI and includes support for multimedia content through `QMediaPlayer`.
Setting Up Your Environment
To begin, ensure that you have Python installed on your system. Next, you will need to install the chosen library. For instance, if you opt for `vlc`, execute the following command in your terminal:
“`bash
pip install python-vlc
“`
For `Pygame`, use:
“`bash
pip install pygame
“`
Ensure all dependencies are met based on the library documentation.
Basic Media Player with VLC
Here’s a straightforward implementation of a media player using the `vlc` library.
“`python
import vlc
import time
Create an instance of the VLC player
player = vlc.MediaPlayer(“path/to/your/media/file.mp3”)
Play the media
player.play()
Keep the program running to allow playback
try:
while True:
time.sleep(1)
except KeyboardInterrupt:
player.stop()
“`
In this example, replace `”path/to/your/media/file.mp3″` with the actual file path.
Implementing a GUI with PyQt5
For a more user-friendly experience, integrating a graphical user interface (GUI) is recommended. Below is a basic example using PyQt5 alongside the `vlc` library.
“`python
import sys
import vlc
from PyQt5.QtWidgets import QApplication, QWidget, QPushButton, QVBoxLayout
class MediaPlayer(QWidget):
def __init__(self):
super().__init__()
self.initUI()
self.player = vlc.MediaPlayer()
def initUI(self):
self.setWindowTitle(‘Simple Media Player’)
self.layout = QVBoxLayout()
self.play_button = QPushButton(‘Play’)
self.play_button.clicked.connect(self.play_media)
self.layout.addWidget(self.play_button)
self.setLayout(self.layout)
def play_media(self):
self.player.set_media(vlc.Media(“path/to/your/media/file.mp3”))
self.player.play()
if __name__ == ‘__main__’:
app = QApplication(sys.argv)
player = MediaPlayer()
player.show()
sys.exit(app.exec_())
“`
This creates a simple window with a “Play” button that starts the media playback.
Adding Functionality
To enhance your media player, consider implementing additional features:
- Pause and Resume: Add buttons to control playback.
- Stop Functionality: Allow users to stop playback.
- Volume Control: Adjust volume using a slider.
- Track Duration: Display current playback time and total track duration.
Example for adding pause and stop:
“`python
self.pause_button = QPushButton(‘Pause’)
self.pause_button.clicked.connect(self.pause_media)
self.layout.addWidget(self.pause_button)
self.stop_button = QPushButton(‘Stop’)
self.stop_button.clicked.connect(self.stop_media)
self.layout.addWidget(self.stop_button)
def pause_media(self):
self.player.pause()
def stop_media(self):
self.player.stop()
“`
These enhancements will provide users with a more complete media playback experience.
Testing Your Media Player
After implementing your media player, it is essential to conduct thorough testing:
- Play Various Formats: Ensure compatibility with different media formats.
- Test on Different OS: Validate functionality across Windows, macOS, and Linux.
- User Feedback: Gather input from users to identify areas for improvement.
By following these guidelines, you can create a robust media player tailored to your needs.
Expert Insights on Creating a Media Player in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Building a media player in Python requires a solid understanding of libraries such as Pygame or PyQt. These libraries provide essential functionalities for audio and video playback, enabling developers to create a user-friendly interface and manage media files effectively.”
James Liu (Lead Developer, Open Source Media Project). “When designing a media player, consider the importance of modularity. By structuring your code into distinct components for playback, user interface, and file management, you enhance maintainability and scalability, allowing for future enhancements without major overhauls.”
Maria Gonzalez (Python Programming Instructor, Code Academy). “It’s crucial to incorporate error handling in your media player. Users may encounter issues such as unsupported file formats or missing codecs. Implementing robust error handling ensures a smoother user experience and improves the overall reliability of your application.”
Frequently Asked Questions (FAQs)
What libraries are commonly used to create a media player in Python?
Popular libraries for building a media player in Python include Pygame, PyQt, and VLC Python bindings. Pygame is suitable for simple audio and video playback, while PyQt offers a more comprehensive GUI framework. VLC Python bindings provide extensive media format support.
How do I install the necessary libraries for a media player in Python?
You can install libraries using pip. For example, to install Pygame, use the command `pip install pygame`. For VLC, install the bindings with `pip install python-vlc`. Ensure you have the required dependencies for each library.
Can I play both audio and video files in my Python media player?
Yes, you can play both audio and video files. Libraries like VLC Python bindings support a wide range of formats, allowing you to implement functionality for various media types in your player.
How do I create a basic GUI for my media player in Python?
You can create a basic GUI using Tkinter or PyQt. Define the main window, add buttons for play, pause, and stop, and link these buttons to their respective functions that control media playback.
What are some common features I should implement in my media player?
Common features include play, pause, stop, volume control, track progress bar, playlist management, and support for various file formats. Implementing these features enhances user experience and functionality.
Is it possible to stream media content in my Python media player?
Yes, it is possible to stream media content. You can utilize libraries like VLC or requests to handle streaming protocols. Ensure you manage buffering and playback controls effectively to provide a smooth streaming experience.
Creating a media player in Python involves several key components, including selecting the right libraries, designing the user interface, and implementing playback functionality. Popular libraries such as Pygame, PyQt, or Tkinter can be utilized for building the interface, while libraries like VLC or PyMedia can handle audio and video playback. Understanding the capabilities of these libraries is crucial for developing a robust media player that meets user needs.
Another important aspect is ensuring compatibility with various media formats. By leveraging libraries that support a wide range of codecs, developers can enhance the usability of their media player. Additionally, implementing features such as playlists, volume control, and playback controls can significantly improve the user experience. Testing the media player across different platforms can also help identify any compatibility issues that may arise.
In summary, building a media player in Python requires careful planning and consideration of the tools and libraries available. By focusing on user experience and ensuring broad media format support, developers can create a functional and appealing media player. Continuous learning and adaptation to new technologies will further enhance the capabilities and performance of the media player over time.
Author Profile
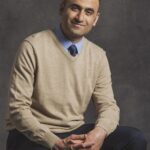
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?