How Can You Create Your First Python Script?
Introduction
In the digital age, the ability to automate tasks and manipulate data through programming has become an invaluable skill. Among the myriad of programming languages available, Python stands out for its simplicity, versatility, and robust community support. Whether you’re a seasoned developer or a curious beginner, learning how to make a Python script can empower you to streamline processes, analyze data, and even create engaging applications. This article will guide you through the essential steps and concepts needed to embark on your Python scripting journey, unlocking a world of possibilities.
Creating a Python script is not just about writing lines of code; it’s about understanding the logic and structure that underpins programming. At its core, a script is a sequence of instructions that the Python interpreter executes to perform specific tasks. From automating mundane chores like file management to developing complex algorithms for data analysis, Python scripts can significantly enhance productivity and creativity.
As you delve deeper into the world of Python scripting, you’ll discover the importance of libraries and frameworks that can extend the capabilities of your code, making it more efficient and powerful. With a focus on best practices and real-world applications, this article will equip you with the foundational knowledge to start crafting your own scripts, paving the way for more advanced programming endeavors in the future.
Setting Up Your Python Environment
To create a Python script, you must first ensure that your Python environment is correctly set up. This involves installing Python on your system and selecting a suitable code editor. Here are the essential steps:
- Install Python:
- Download the latest version of Python from the official website (python.org).
- Follow the installation instructions relevant to your operating system (Windows, macOS, or Linux).
- During installation, make sure to check the option to add Python to your system’s PATH.
- Choose a Code Editor:
- Options include:
- Visual Studio Code
- PyCharm
- Sublime Text
- Atom
- These editors provide syntax highlighting, debugging tools, and other features that enhance the coding experience.
Creating Your First Python Script
Once your environment is set up, you can create your first Python script. A Python script is essentially a text file containing Python code, which typically has a `.py` extension. Follow these steps:
- Open your code editor.
- Create a new file and save it with a `.py` extension (e.g., `hello_world.py`).
- Write your code. For instance, a simple script could look like this:
python
print(“Hello, World!”)
- Save the file.
Running Your Python Script
To execute your Python script, you need to use the command line (or terminal). Here’s how to run your script:
- Open Command Line or Terminal:
- On Windows, search for `cmd` or `PowerShell`.
- On macOS or Linux, open the Terminal application.
- Navigate to the directory where your script is located using the `cd` command. For example:
bash
cd path\to\your\script
- Run the script by entering the following command:
bash
python hello_world.py
This command should output:
Hello, World!
Understanding Python Script Structure
A Python script can consist of various components. Here’s a breakdown of common elements found in Python scripts:
Component | Description |
---|---|
Comments | Lines that start with `#` are ignored by the interpreter and are used for documentation. |
Variables | Used to store data values. For example, `x = 5` assigns the value 5 to the variable x. |
Functions | Reusable pieces of code that perform specific tasks. Defined using the `def` keyword. |
Control Structures | Includes loops (`for`, `while`) and conditionals (`if`, `else`) that control the flow of execution. |
Understanding these components will help you structure your script effectively and enhance its functionality.
Choosing an Environment
Selecting the right environment for your Python script is crucial for a smooth development process. Here are common options:
- Text Editors: Lightweight options like Notepad++, Sublime Text, or Visual Studio Code.
- Integrated Development Environments (IDEs): Comprehensive tools like PyCharm or Anaconda that provide debugging and testing features.
- Jupyter Notebooks: Ideal for data analysis, allowing you to write code in chunks and see immediate results.
Setting Up Python
Before writing a Python script, ensure that Python is installed on your machine. Follow these steps:
- Download Python from the official website (https://www.python.org/downloads/).
- Follow the installation prompts, ensuring to check the box that says “Add Python to PATH.”
- Verify the installation by opening a command line or terminal and typing `python –version`.
Writing Your First Script
To create a Python script, follow these steps:
- Open your chosen text editor or IDE.
- Create a new file and save it with a `.py` extension, for example, `hello.py`.
- Write your Python code in the file. Here’s a simple example:
python
print(“Hello, World!”)
- Save the file after writing the code.
Running Your Script
Executing your Python script can be done through various methods:
- Command Line:
- Open a command prompt or terminal.
- Navigate to the directory containing your script using `cd path_to_your_script`.
- Execute the script by typing `python hello.py` and pressing Enter.
- From an IDE: Most IDEs have a run button or an option in the menu that allows you to execute the script directly.
Understanding Python Syntax
Familiarity with basic Python syntax is essential for effective scripting. Key components include:
Component | Description |
---|---|
Variables | Used to store data, e.g., `name = “Alice”` |
Data Types | Common types: integers, floats, strings, lists, dicts |
Functions | Blocks of code that perform specific tasks, defined with `def`, e.g., `def my_function():` |
Control Flow | Structures like `if`, `for`, and `while` for decision making and looping |
Debugging Your Script
Debugging is a critical step in script development. Consider the following methods:
- Print Statements: Insert `print()` statements to check variable values at different points.
- Using a Debugger: IDEs often come with debugging tools that allow you to step through code line-by-line.
- Error Messages: Pay attention to error messages in the console; they provide clues to issues in your code.
Adding Libraries and Modules
Enhancing your script with additional functionality can be achieved by importing libraries and modules. Commonly used libraries include:
- NumPy: For numerical computations.
- Pandas: For data manipulation and analysis.
- Requests: For making HTTP requests.
To install a library, use pip:
bash
pip install library_name
After installation, import it in your script:
python
import numpy as np
Best Practices
Implementing best practices ensures your script is efficient and maintainable:
- Code Comments: Use `#` to add comments that explain your code.
- Consistent Naming Conventions: Use descriptive variable names, following `snake_case` or `CamelCase`.
- Version Control: Utilize tools like Git for tracking changes and collaboration.
- Testing: Write tests for your functions to ensure they behave as expected.
Expert Guidance on Crafting a Python Script
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “To create an effective Python script, one must first clearly define the problem to be solved. A well-structured approach includes outlining the script’s purpose, breaking it down into manageable functions, and utilizing libraries that enhance functionality.”
Mark Thompson (Lead Python Developer, CodeMasters). “It is essential to adhere to Python’s syntax and conventions when scripting. Leveraging tools such as IDEs for debugging and version control systems can significantly improve the development process and ensure code quality.”
Sarah Patel (Data Scientist, Analytics World). “When writing a Python script, consider the scalability and efficiency of your code. Utilizing built-in functions and libraries not only speeds up development but also enhances performance, especially when dealing with large datasets.”
Frequently Asked Questions (FAQs)
How do I start writing a Python script?
To begin writing a Python script, open a text editor or an Integrated Development Environment (IDE) such as PyCharm or Visual Studio Code. Create a new file with a `.py` extension, and start coding using Python syntax.
What is the basic structure of a Python script?
A basic Python script typically includes import statements for libraries, function definitions, and executable code. The script may start with a shebang line (`#!/usr/bin/env python3`) to specify the interpreter, followed by comments and code.
How do I run a Python script?
To run a Python script, open a terminal or command prompt, navigate to the directory containing the script, and execute the command `python script_name.py`, replacing `script_name.py` with your actual script file name.
What are common errors to look out for when writing a Python script?
Common errors include syntax errors, indentation errors, and runtime errors. Syntax errors occur when the code does not conform to Python’s grammar, while indentation errors arise from inconsistent use of spaces or tabs.
How can I debug my Python script?
You can debug a Python script using built-in tools like `print()` statements to check variable values, or by using a debugger available in IDEs such as PyCharm. Python also includes the `pdb` module for more advanced debugging capabilities.
What libraries should I consider using in my Python scripts?
Consider using libraries such as NumPy for numerical computations, Pandas for data manipulation, Requests for HTTP requests, and Flask or Django for web development, depending on the specific needs of your project.
Creating a Python script involves several fundamental steps that are essential for both beginners and experienced programmers. First, it is important to have Python installed on your system, which can be done by downloading it from the official Python website. Once installed, you can choose an integrated development environment (IDE) or a simple text editor to write your script. Popular options include PyCharm, VS Code, and Jupyter Notebook, each providing unique features that enhance the coding experience.
After setting up your environment, you can start writing your script. Python uses a straightforward syntax, making it accessible for newcomers. It is advisable to begin with a clear understanding of the problem you want to solve, breaking it down into manageable parts. This approach allows for structured coding, making it easier to debug and maintain your script. Additionally, incorporating comments within your code can significantly enhance its readability and provide context for future reference.
Once your script is complete, testing and debugging are crucial steps to ensure functionality. Running your script in a terminal or through your chosen IDE will help identify any errors or areas for improvement. Utilizing Python’s built-in debugging tools can aid in this process. Finally, once you are satisfied with your script, consider sharing it with the community or using version control
Author Profile
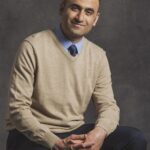
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?