How Can You Create a Simple Password Program in Python?
In an age where digital security is paramount, the importance of creating strong, reliable passwords cannot be overstated. Whether you’re safeguarding personal information, protecting sensitive data, or simply trying to keep your online accounts secure, understanding how to create a simple password program in Python can be an invaluable skill. This not only enhances your coding abilities but also equips you with the tools to fortify your digital presence against potential threats.
Developing a password program in Python is a fantastic way to dive into the world of programming while addressing a real-world need. With just a few lines of code, you can create a basic application that generates secure passwords, checks their strength, and even stores them safely. This project serves as an excellent to Python’s capabilities, allowing you to explore concepts like string manipulation, user input, and basic data structures.
As you embark on this journey, you’ll gain insights into the principles of good password creation, such as length, complexity, and unpredictability. By the end of the process, not only will you have a functional program, but you’ll also have a deeper understanding of how programming can be applied to enhance security in our increasingly digital lives. Get ready to unlock the potential of Python and take a significant step toward better online security!
Basic Structure of a Password Program
To create a simple password program in Python, you will need to establish a basic structure that includes user input, password validation, and feedback mechanisms. The program can prompt users to create a password, check its strength, and provide suggestions for improvement. Below is a sample outline of the program structure.
“`python
def get_password():
password = input(“Enter your password: “)
return password
def validate_password(password):
Check for minimum length
if len(password) < 8:
return , "Password must be at least 8 characters long."
Check for numeric characters
if not any(char.isdigit() for char in password):
return , "Password must contain at least one number."
Check for uppercase letters
if not any(char.isupper() for char in password):
return , "Password must contain at least one uppercase letter."
Check for special characters
if not any(char in "!@$%^&*()-_=+[]{};:,.<>?/” for char in password):
return , “Password must contain at least one special character.”
return True, “Password is strong.”
def main():
password = get_password()
is_valid, message = validate_password(password)
print(message)
if __name__ == “__main__”:
main()
“`
Password Validation Criteria
Implementing password validation is crucial for enhancing security. The following criteria ensure that the password is robust and less susceptible to attacks.
- Minimum Length: At least 8 characters.
- Numeric Characters: Inclusion of at least one digit (0-9).
- Uppercase Letters: Inclusion of at least one uppercase letter (A-Z).
- Special Characters: Inclusion of at least one special character such as `!@$%^&*()`.
The table below summarizes these criteria:
Criteria | Description |
---|---|
Minimum Length | At least 8 characters |
Numeric Character | At least one digit (0-9) |
Uppercase Letter | At least one uppercase letter (A-Z) |
Special Character | At least one special character (e.g., !@$%^&*) |
Running the Password Program
Once the program is structured and the validation criteria are set, you can execute the program. The user will be prompted to enter a password, and the program will evaluate its strength based on the specified criteria. If the password does not meet the requirements, the program will provide specific feedback indicating what needs to be improved.
This approach not only guides users in creating secure passwords but also enhances their understanding of password strength. By following best practices in password creation, users can significantly reduce the risk of unauthorized access to their accounts.
The program can also be expanded with additional features, such as password suggestions or an option to change an existing password, to further aid users in maintaining security.
Understanding the Basics of Password Generation
A simple password program in Python can help users create secure passwords. Passwords should generally meet certain criteria to enhance security:
- Length: At least 8 characters.
- Complexity: A mix of uppercase letters, lowercase letters, numbers, and special characters.
- Unpredictability: Avoid common words or sequences.
Setting Up the Environment
To create a password program, ensure you have Python installed on your system. You can download the latest version from the official Python website. Additionally, a code editor like Visual Studio Code, PyCharm, or even a simple text editor will suffice.
Creating a Simple Password Generator
The following Python code snippet demonstrates how to create a basic password generator:
“`python
import random
import string
def generate_password(length=12):
Define the character pool
characters = string.ascii_letters + string.digits + string.punctuation
Generate a random password
password = ”.join(random.choice(characters) for _ in range(length))
return password
Example usage
if __name__ == “__main__”:
password_length = int(input(“Enter desired password length: “))
print(“Generated Password:”, generate_password(password_length))
“`
Code Explanation
- Imports:
- `random`: To generate random choices.
- `string`: To access predefined string constants (letters, digits, punctuation).
- Function `generate_password`:
- Accepts a `length` parameter, defaulting to 12.
- Combines uppercase, lowercase, digits, and punctuation into a character pool.
- Utilizes a list comprehension to select characters randomly from the pool and join them into a single string.
- Main Block:
- Prompts the user for the desired password length.
- Calls the `generate_password` function and prints the result.
Enhancing Security Features
To further improve the password generator, consider implementing the following features:
- User-defined Complexity: Allow users to specify requirements for character types.
- Avoiding Similar Characters: Exclude ambiguous characters such as ‘l’, ‘1’, ‘O’, and ‘0’.
- Password Strength Evaluation: Integrate a function to evaluate the strength of the generated password.
Sample Code with Enhanced Features
Here is an extended version of the password generator that includes user-defined complexity and avoids similar characters:
“`python
def generate_strong_password(length=12, use_special=True, use_numbers=True):
characters = string.ascii_letters
if use_special:
characters += string.punctuation
if use_numbers:
characters += string.digits
Avoid similar characters
characters = characters.replace(‘l’, ”).replace(‘1’, ”).replace(‘O’, ”).replace(‘0’, ”)
password = ”.join(random.choice(characters) for _ in range(length))
return password
Example usage
if __name__ == “__main__”:
length = int(input(“Enter desired password length: “))
special = input(“Include special characters? (y/n): “).lower() == ‘y’
numbers = input(“Include numbers? (y/n): “).lower() == ‘y’
print(“Generated Strong Password:”, generate_strong_password(length, special, numbers))
“`
This version allows users to toggle the inclusion of special characters and numbers, while also avoiding similar-looking characters for improved readability.
Building a Simple Password Program in Python: Expert Insights
Dr. Emily Carter (Software Engineer, Cybersecurity Innovations). “Creating a simple password program in Python is an excellent way to understand basic programming concepts. I recommend starting with user input for the password, followed by implementing validation checks to ensure it meets security standards, such as length and complexity.”
James Liu (Lead Developer, SecureTech Solutions). “When developing a password program, it’s crucial to incorporate hashing for storing passwords securely. Using libraries like hashlib in Python can help you achieve this, ensuring that even if the data is compromised, the actual passwords remain protected.”
Sarah Thompson (Python Instructor, Code Academy). “For beginners, I suggest breaking down the project into smaller tasks. Start with writing functions for password creation, validation, and storage. This modular approach will not only simplify the coding process but also enhance your understanding of function-based programming in Python.”
Frequently Asked Questions (FAQs)
What is a simple password program in Python?
A simple password program in Python is a basic application that allows users to create, store, and validate passwords. It typically includes functionalities for user input, password strength checking, and secure storage.
How can I start writing a password program in Python?
Begin by setting up a Python environment. Use an integrated development environment (IDE) or a text editor to write your code. Import necessary libraries like `getpass` for secure password input and `re` for regular expressions to validate password strength.
What features should I include in a basic password program?
Essential features include user registration, password creation, password strength validation, and user login functionality. You may also consider adding password recovery options and secure password storage techniques.
How do I validate password strength in Python?
You can validate password strength by checking for minimum length, the inclusion of uppercase and lowercase letters, numbers, and special characters. Use regular expressions to enforce these rules effectively.
What is the best way to store passwords securely in Python?
The best practice for storing passwords is to hash them using libraries like `bcrypt` or `hashlib`. This ensures that even if the data is compromised, the original passwords remain secure and unreadable.
Can I implement user authentication in my password program?
Yes, you can implement user authentication by comparing the hashed version of the entered password with the stored hash. This process ensures that only users with the correct credentials can access the system.
Creating a simple password program in Python involves understanding the basic principles of user input, string manipulation, and conditional statements. The program typically prompts the user to enter a password, checks its validity based on predefined criteria, and provides feedback. This foundational exercise not only reinforces programming skills but also introduces concepts of security and user authentication.
Key components of a simple password program include defining password requirements such as length, the inclusion of uppercase and lowercase letters, numbers, and special characters. Utilizing Python’s built-in functions and libraries can streamline the process of validating these criteria. Additionally, implementing loops and conditionals allows for a user-friendly experience, ensuring that users can attempt to create a valid password until they succeed.
In summary, a simple password program serves as an excellent starting point for beginners in Python programming. It highlights the importance of security in software development and encourages best practices in password management. By mastering this simple project, programmers can build a solid foundation for more complex applications that require user authentication and data protection.
Author Profile
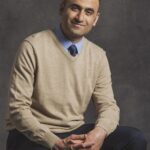
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?