How Can You Create a Timer in Python?
Introduction
In our fast-paced world, time management is more crucial than ever, and what better way to enhance your productivity than by creating your own timer? Whether you’re a student looking to manage study sessions, a professional aiming to optimize work intervals, or a developer wanting to build a unique application, mastering the art of creating a timer in Python can be both a practical skill and a fun project. Python, with its simplicity and versatility, offers a straightforward approach to implement timers, making it accessible for beginners and experienced programmers alike.
Creating a timer in Python involves understanding basic programming concepts and leveraging built-in libraries that make time manipulation seamless. From simple countdowns to more complex features like user input and alerts, the possibilities are endless. This article will guide you through the essential steps to build a timer, ensuring you grasp the foundational elements while also inspiring you to explore further functionalities.
As you delve into the world of Python timers, you’ll discover how to utilize various techniques, such as threading and the time module, to enhance the functionality of your timer. This exploration not only reinforces your programming skills but also opens up avenues for creating more sophisticated applications. So, whether you’re looking to improve your focus with a Pomodoro timer or develop a countdown for an event, let’s embark on
Basic Timer Implementation
To create a simple timer in Python, you can utilize the built-in `time` module. This approach allows you to track elapsed time easily. Here’s a straightforward example of how to implement a countdown timer:
python
import time
def countdown_timer(seconds):
while seconds:
mins, secs = divmod(seconds, 60)
timer = ‘{:02d}:{:02d}’.format(mins, secs)
print(timer, end=’\r’)
time.sleep(1)
seconds -= 1
print(“Time’s up!”)
# Example usage
countdown_timer(10)
This function takes an integer input representing the number of seconds and counts down to zero, updating the display every second.
Using the Threading Module
For more complex applications, especially where you need a timer running in the background, the `threading` module can be beneficial. This allows the timer to operate independently of the main program flow.
python
import threading
import time
def timer_function(seconds):
time.sleep(seconds)
print(“Timer finished!”)
# Example usage
timer_thread = threading.Thread(target=timer_function, args=(5,))
timer_thread.start()
print(“Timer started, doing other tasks.”)
In this example, the timer runs in a separate thread, allowing the main program to continue executing other tasks while waiting for the timer to finish.
Creating a GUI Timer with Tkinter
For a graphical user interface (GUI) timer, you can use the `Tkinter` library, which is included with Python. Here’s how to create a simple countdown timer application:
python
import tkinter as tk
def countdown(seconds):
if seconds > 0:
mins, secs = divmod(seconds, 60)
timer = ‘{:02d}:{:02d}’.format(mins, secs)
label.config(text=timer)
window.update()
window.after(1000, countdown, seconds – 1)
else:
label.config(text=”Time’s up!”)
window = tk.Tk()
window.title(“Countdown Timer”)
label = tk.Label(window, font=(‘Helvetica’, 48), fg=’red’)
label.pack()
countdown(10) # Set the countdown time in seconds
window.mainloop()
This code snippet creates a window displaying the countdown timer, and it updates the label every second until the timer reaches zero.
Timer with Input Functionality
To allow users to set the timer duration dynamically, you can integrate input functionality within your GUI application:
python
def start_timer():
try:
seconds = int(entry.get())
countdown(seconds)
except ValueError:
label.config(text=”Please enter a valid number.”)
entry = tk.Entry(window, font=(‘Helvetica’, 24))
entry.pack()
start_button = tk.Button(window, text=”Start Timer”, command=start_timer)
start_button.pack()
This enhancement allows users to input the desired countdown duration before starting the timer.
Functionality | Description |
---|---|
Basic Timer | Counts down from a specified number of seconds in the console. |
Threaded Timer | Runs a timer in a separate thread, allowing other tasks to execute concurrently. |
GUI Timer | Displays a countdown timer in a graphical window using Tkinter. |
User Input Timer | Allows users to set the timer duration through an input field in the GUI. |
Creating a Simple Timer
To create a basic timer in Python, you can use the built-in `time` module, which provides various time-related functions. Here’s how to set up a simple countdown timer:
python
import time
def countdown_timer(seconds):
while seconds:
mins, secs = divmod(seconds, 60)
timer = ‘{:02d}:{:02d}’.format(mins, secs)
print(timer, end=’\r’)
time.sleep(1)
seconds -= 1
print(“Time’s up!”)
# Example usage
countdown_timer(10)
### Explanation of the Code:
- Importing Time Module: The `time` module is imported to enable the use of time-related functions.
- Countdown Function: The `countdown_timer` function accepts a parameter `seconds`, which defines the countdown duration.
- Looping through Seconds: A `while` loop continues until `seconds` is zero. The `divmod` function calculates minutes and seconds.
- Formatting Time: The formatted time is displayed in `MM:SS` format using string formatting.
- Sleeping for One Second: The `time.sleep(1)` call ensures the loop pauses for one second between updates.
Building a Timer with User Input
To enhance the timer, you can allow user input for specifying the duration. This can be accomplished as follows:
python
def user_input_timer():
seconds = int(input(“Enter the time in seconds: “))
countdown_timer(seconds)
user_input_timer()
### Key Components:
- User Input: The `input` function captures user input, converting it to an integer for the countdown.
- Function Call: The `user_input_timer` function invokes the `countdown_timer` with the specified duration.
Creating a Stopwatch
A stopwatch can be implemented using the following approach, which involves measuring elapsed time:
python
import time
def stopwatch():
input(“Press Enter to start the stopwatch”)
start_time = time.time()
input(“Press Enter to stop the stopwatch”)
end_time = time.time()
elapsed_time = end_time – start_time
print(f”Elapsed Time: {elapsed_time:.2f} seconds”)
# Example usage
stopwatch()
### Explanation of Stopwatch Functionality:
- Starting the Stopwatch: The first `input` waits for the user to press Enter, signaling the start.
- Recording Time: `time.time()` captures the current time in seconds since the epoch.
- Stopping the Stopwatch: The second `input` pauses execution until the user stops the timer.
- Calculating Elapsed Time: The difference between the start and end times gives the total elapsed time, which is displayed in seconds.
Using Threading for a Non-blocking Timer
For a more advanced timer that allows other tasks to run simultaneously, you can use the `threading` module:
python
import threading
import time
def countdown_timer(seconds):
while seconds:
mins, secs = divmod(seconds, 60)
timer = ‘{:02d}:{:02d}’.format(mins, secs)
print(timer, end=’\r’)
time.sleep(1)
seconds -= 1
print(“Time’s up!”)
def start_timer(seconds):
timer_thread = threading.Thread(target=countdown_timer, args=(seconds,))
timer_thread.start()
# Example usage
start_timer(10)
### Advantages of Using Threading:
- Non-blocking Behavior: The main program can continue to run while the timer counts down.
- Concurrency: Allows the execution of multiple functions without waiting for one to finish, enhancing user experience in applications.
By implementing these techniques, you can create various timer functionalities in Python, ranging from simple countdowns to sophisticated, non-blocking timers.
Expert Insights on Creating Timers in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Creating a timer in Python can be accomplished using the built-in `time` and `threading` modules. By leveraging these tools, developers can implement precise timing functions that cater to various applications, from simple countdowns to complex scheduling tasks.”
Michael Chen (Python Developer and Author, CodeMaster Publications). “Utilizing the `time.sleep()` function is a straightforward approach for creating timers in Python. However, for more advanced features, such as asynchronous timers, the `asyncio` library provides a robust framework that allows for non-blocking timer implementations, enhancing the efficiency of your code.”
Sarah Thompson (Lead Data Scientist, AI Solutions Group). “When designing a timer in Python, it is crucial to consider the user interface. For applications with a graphical user interface (GUI), integrating timers with libraries like Tkinter or PyQt can significantly improve user experience, allowing for real-time updates and interactions.”
Frequently Asked Questions (FAQs)
How do I create a simple countdown timer in Python?
To create a simple countdown timer in Python, you can use the `time` module. Implement a loop that decrements the timer and calls `time.sleep(1)` to pause for one second between updates.
Can I use the `threading` module to create a timer in Python?
Yes, the `threading` module can be used to run a timer in a separate thread. Use `threading.Timer` to schedule a function to be executed after a specified interval.
How can I format the timer output in hours, minutes, and seconds?
You can format the timer output by calculating hours, minutes, and seconds from the total seconds remaining. Use integer division and the modulus operator to extract each component.
Is it possible to create a GUI timer in Python?
Yes, you can create a GUI timer using libraries such as Tkinter or PyQt. These libraries provide widgets to display the timer and handle user interactions.
What is the best way to handle user input for setting a timer duration?
You can use the `input()` function to receive user input for the timer duration. Validate the input to ensure it is a positive integer before starting the timer.
Can I pause and resume a timer in Python?
Yes, you can implement pause and resume functionality by maintaining the state of the timer and using flags to control the execution flow. Store the remaining time when paused and resume from that point.
Creating a timer in Python is a straightforward process that can be accomplished using various methods, depending on the specific requirements of the application. The most common approach involves using the built-in `time` module, which provides functions to handle time-related tasks effectively. A simple countdown timer can be implemented using a loop that decrements a specified time interval while utilizing the `sleep` function to create pauses between updates.
For more advanced functionality, developers can explore the `threading` module, which allows timers to run in the background without blocking the main program execution. This is particularly useful for applications that require simultaneous operations or user interactions while the timer is active. Additionally, the `datetime` module can be employed for more precise time calculations, enabling the creation of timers that can work with specific date and time formats.
In summary, Python offers multiple avenues for implementing timers, ranging from simple countdowns to more complex, multi-threaded solutions. Understanding the available modules and their capabilities is essential for selecting the most appropriate method for a given project. By leveraging these tools, developers can create efficient and effective timers tailored to their specific needs.
Author Profile
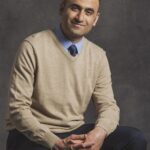
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?