How Can You Make a Variable Global in Python?
In the world of programming, the ability to manage variables effectively can make or break the functionality of your code. Python, known for its simplicity and readability, offers various ways to define and manipulate variables. However, when you find yourself needing to access a variable across different functions or scopes, the concept of global variables comes into play. Understanding how to make a variable global in Python is essential for any developer looking to streamline their code and enhance its modularity.
At its core, a global variable is one that is accessible from any part of your code, regardless of where it is defined. This can be particularly useful when you want to maintain a single source of truth for a value that multiple functions need to reference or modify. However, the use of global variables can introduce complexity and potential pitfalls, such as unintended side effects or difficulties in debugging. Therefore, it’s crucial to grasp the nuances of how global variables work in Python, including the syntax and best practices for their implementation.
As we delve deeper into the mechanics of global variables, we’ll explore various scenarios where they can be beneficial, as well as the potential drawbacks of relying too heavily on them. By the end of this article, you’ll have a clearer understanding of how to effectively utilize global variables in your Python projects, empowering you to write
Understanding Variable Scope in Python
In Python, the scope of a variable refers to the context within which that variable can be accessed or modified. Variables defined within a function are local to that function, meaning they cannot be accessed from outside it. Conversely, global variables are defined outside of any function and can be accessed throughout the entire code.
To make a variable global within a function, the `global` keyword is used. This keyword tells Python that you want to use the global variable rather than creating a new local variable.
Using the Global Keyword
To declare a variable as global within a function, follow these steps:
- Define the variable outside of any function.
- Inside the function, use the `global` keyword followed by the variable name.
- Modify or access the global variable as needed.
Here’s a basic example:
python
x = 10 # Global variable
def modify_global():
global x # Declare x as global
x = 20 # Modify the global variable
modify_global()
print(x) # Output: 20
In this example, the global variable `x` is modified within the function `modify_global`. Without the `global` keyword, Python would treat `x` as a local variable, leading to an error if you attempted to modify it.
Global Variables in Multiple Functions
When working with multiple functions that need access to the same global variable, the same principles apply. Each function must declare the variable as global if it intends to modify it.
Consider the following example:
python
y = 5 # Global variable
def function_one():
global y
y += 5
def function_two():
global y
y *= 2
function_one()
function_two()
print(y) # Output: 20
In this case, `function_one` adds 5 to `y`, and `function_two` multiplies `y` by 2. After calling both functions, the global variable `y` reflects the cumulative changes.
Best Practices for Global Variables
While global variables can be useful, they should be used judiciously. Here are some best practices:
- Limit Usage: Minimize the use of global variables to reduce side effects and make code easier to understand.
- Naming Convention: Use uppercase letters for global variables to distinguish them from local variables (e.g., `GLOBAL_VAR`).
- Documentation: Clearly document the purpose of global variables to ensure maintainability.
Comparison of Local and Global Variables
The following table summarizes the key differences between local and global variables:
Feature | Local Variable | Global Variable |
---|---|---|
Definition Location | Inside a function | Outside any function |
Scope | Limited to the function | Accessible throughout the code |
Declaration | Automatically local | Requires `global` keyword to modify |
By following these guidelines and understanding variable scope, developers can effectively manage global variables in Python, leading to cleaner and more maintainable code.
Understanding Global Variables in Python
In Python, a global variable is defined outside of any function and can be accessed throughout the program, including inside functions. However, to modify a global variable from within a function, specific rules must be followed.
Defining a Global Variable
To declare a global variable, simply define it outside of any function. For example:
python
x = 10 # Global variable
This variable `x` can be accessed anywhere in the code after its declaration.
Accessing Global Variables Inside Functions
You can directly access a global variable within a function without any special declarations. For instance:
python
def print_x():
print(x)
print_x() # Output: 10
Modifying Global Variables Inside Functions
To modify a global variable within a function, use the `global` keyword. This informs Python that you intend to use the global variable rather than creating a local one. Here’s how to do it:
python
def modify_x():
global x
x = 20 # Modifying the global variable
modify_x()
print(x) # Output: 20
Best Practices for Using Global Variables
While global variables can be useful, they can also lead to code that is difficult to debug and maintain. Consider the following best practices:
- Limit Usage: Use global variables sparingly. Prefer passing parameters to functions when possible.
- Naming Convention: Use a consistent naming convention (e.g., prefixing with `g_`) to indicate that a variable is global.
- Encapsulation: Encapsulate global variables within classes or modules to limit their scope and enhance readability.
Global Variables in Different Scopes
Understanding variable scope is crucial for effective programming. Below is a summary of variable scopes in Python:
Scope Type | Description |
---|---|
Local | Variables defined within a function. |
Enclosing | Variables in the local scope of enclosing functions. |
Global | Variables defined at the top level of a script/module. |
Built-in | Names preassigned in the built-in names module. |
Using Global Variables in Modules
When working with modules, a variable declared in one module can be accessed in another module. To do this, follow these steps:
- Create a Module: Define your global variable in a separate Python file (e.g., `config.py`).
python
# config.py
global_var = “Hello, World!”
- Import the Module: Access the global variable in another file.
python
# main.py
import config
print(config.global_var) # Output: Hello, World!
By adhering to these practices and understanding the nuances of global variables, you can effectively manage their use in your Python programs.
Strategies for Making Variables Global in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To make a variable global in Python, you can declare it outside of any function. This allows you to access and modify it within functions by using the ‘global’ keyword, which explicitly tells Python that you want to use the global variable instead of creating a local one.”
Michael Chen (Python Developer, CodeCraft Solutions). “Using the ‘global’ keyword is essential when you need to update a global variable inside a function. Without it, Python will treat the variable as a local one, leading to potential confusion and bugs in your code.”
Sarah Patel (Lead Data Scientist, Data Insights Group). “While making variables global can be useful, it is important to consider the design of your code. Overusing global variables can lead to tightly coupled code and make debugging difficult. It’s often better to pass variables as parameters or use classes to encapsulate state.”
Frequently Asked Questions (FAQs)
What does it mean to make a variable global in Python?
Making a variable global in Python means that the variable can be accessed and modified from any function within the same module, rather than being limited to the scope of a single function.
How do you declare a global variable inside a function?
To declare a global variable inside a function, use the `global` keyword followed by the variable name. This informs Python that you want to use the global instance of the variable, not a local one.
Can you give an example of making a variable global?
Certainly.
python
x = 10 # Global variable
def modify_variable():
global x
x = 20 # This modifies the global variable
modify_variable()
print(x) # Output: 20
Are there any limitations to using global variables?
Yes, excessive use of global variables can lead to code that is difficult to debug and maintain. It can also introduce unintended side effects, as changes to global variables can affect multiple parts of the program.
Is it a good practice to use global variables?
Using global variables is generally discouraged unless necessary. It is better to use function parameters and return values to maintain clean and modular code, enhancing readability and maintainability.
How can you access a global variable from a nested function?
You can access a global variable from a nested function directly without declaring it as global. However, if you want to modify it, you must declare it as global in the enclosing function. For example:
python
x = 5
def outer_function():
def inner_function():
global x
x += 1
inner_function()
outer_function()
print(x) # Output: 6
In Python, making a variable global involves using the `global` keyword within a function. This keyword allows you to modify a variable that exists in the global scope, rather than creating a new local variable with the same name. When you declare a variable as global, any changes made to it within the function will affect its value outside the function as well.
It is important to note that global variables should be used judiciously. While they can facilitate data sharing across functions, excessive reliance on global variables can lead to code that is difficult to understand and maintain. Proper encapsulation and the use of function parameters are often preferable to ensure that functions remain modular and their interactions are clear.
Additionally, Python also supports the use of global variables in nested functions. In such cases, if a variable in the outer function is to be modified, the `nonlocal` keyword is used instead of `global`. This distinction is crucial for managing variable scopes effectively within nested structures.
understanding how to make a variable global in Python is essential for effective programming. However, developers should strive for a balanced approach that minimizes the use of global variables to maintain code clarity and integrity.
Author Profile
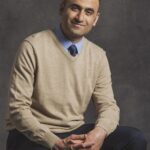
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?