How Can You Create a Directed Graph in Python for LeetCode Challenges?
Creating directed graphs is a fundamental skill in computer science, particularly for solving complex problems on platforms like LeetCode. Whether you’re tackling graph traversal, pathfinding, or optimizing network flows, understanding how to represent and manipulate directed graphs in Python can significantly enhance your problem-solving toolkit. In this article, we’ll explore the nuances of constructing directed graphs using Python, providing you with the insights needed to excel in coding challenges and interviews.
Directed graphs, or digraphs, are structures where edges have a direction, indicating a one-way relationship between nodes. This characteristic makes them particularly useful for modeling scenarios such as web page links, task scheduling, and social networks. In Python, there are several approaches to represent these graphs, including adjacency lists and matrices, each offering unique advantages depending on the problem at hand. As we delve deeper, you’ll discover how to effectively implement these representations and utilize them to solve various LeetCode problems.
Moreover, understanding directed graphs paves the way for mastering key algorithms such as Depth-First Search (DFS) and Breadth-First Search (BFS), which are essential for traversing and analyzing graph structures. By the end of this article, you will not only be equipped with the technical know-how to create directed graphs in Python but also gain the confidence to
Creating a Directed Graph Using Adjacency List
One common way to represent a directed graph in Python is through an adjacency list. This method utilizes a dictionary where each key corresponds to a vertex, and the associated value is a list of vertices that the key vertex points to.
To create a directed graph using an adjacency list, follow these steps:
- Initialize an empty dictionary.
- For each directed edge, update the dictionary by appending the target vertex to the list of the source vertex.
Here’s an example implementation:
python
class DirectedGraph:
def __init__(self):
self.graph = {}
def add_edge(self, u, v):
if u not in self.graph:
self.graph[u] = []
self.graph[u].append(v)
def display(self):
for vertex in self.graph:
print(f”{vertex} -> {‘, ‘.join(map(str, self.graph[vertex]))}”)
In this code:
- The `add_edge` method adds a directed edge from vertex `u` to vertex `v`.
- The `display` method prints the graph in a readable format.
Using NetworkX for Directed Graphs
For more complex graph operations, the NetworkX library is a powerful tool in Python. It provides built-in functions to create, manipulate, and analyze graphs.
To create a directed graph using NetworkX, follow these steps:
- Install NetworkX via pip:
bash
pip install networkx
- Use the following code to create a directed graph:
python
import networkx as nx
# Create a directed graph
G = nx.DiGraph()
# Add edges
G.add_edges_from([(1, 2), (1, 3), (2, 4), (3, 4)])
# Display the graph
print(“Directed Graph:”)
print(G.edges())
In this example:
- A directed graph `G` is created using `nx.DiGraph()`.
- Edges are added using the `add_edges_from` method, which takes a list of tuples, each representing a directed edge.
Visualizing a Directed Graph
Visualization of directed graphs can greatly aid in understanding their structure. Using NetworkX in combination with Matplotlib allows for straightforward graph visualization.
To visualize a directed graph, add the following code snippet:
python
import matplotlib.pyplot as plt
# Draw the directed graph
pos = nx.spring_layout(G) # positions for all nodes
nx.draw(G, pos, with_labels=True, arrows=True)
plt.show()
This code snippet:
- Uses the `spring_layout` for positioning nodes.
- Draws the graph and displays it with arrows indicating the direction of edges.
Performance Considerations
When implementing a directed graph, consider the following performance factors:
Operation | Adjacency List | NetworkX |
---|---|---|
Space Complexity | O(V + E) | O(V + E) |
Add Edge | O(1) | O(1) |
Remove Edge | O(V) | O(1) |
Check for Edge | O(V) | O(1) |
- Adjacency List: Efficient for sparse graphs and allows for quick edge additions.
- NetworkX: Provides additional functionalities like algorithms for shortest paths, connectivity, and more, but may be less efficient for very large graphs.
Utilizing the appropriate structure and libraries based on your specific needs can significantly enhance the performance and usability of your directed graphs in Python.
Creating a Directed Graph in Python for LeetCode Problems
To create a directed graph in Python, especially for algorithmic challenges on platforms like LeetCode, you can utilize various data structures. The most common methods include using an adjacency list or an adjacency matrix. Below are steps and examples for both approaches.
Using an Adjacency List
An adjacency list is a space-efficient way to represent a graph. It consists of a dictionary where each key is a node, and the corresponding value is a list of nodes to which it is directed.
Steps:
- Initialize an empty dictionary.
- For each directed edge, add the destination node to the source node’s list.
Example:
python
from collections import defaultdict
def create_graph(edges):
graph = defaultdict(list)
for src, dest in edges:
graph[src].append(dest)
return graph
# Example edges
edges = [(0, 1), (0, 2), (1, 2), (2, 0), (2, 3)]
graph = create_graph(edges)
print(graph)
Output:
defaultdict(
Using an Adjacency Matrix
An adjacency matrix is a 2D array where the element at row `i` and column `j` indicates if there is a directed edge from node `i` to node `j`. This method is less space-efficient for sparse graphs but can be beneficial for dense graphs.
Steps:
- Create a 2D list initialized to `0`.
- For each directed edge, set the corresponding position to `1`.
Example:
python
def create_adjacency_matrix(num_nodes, edges):
matrix = [[0] * num_nodes for _ in range(num_nodes)]
for src, dest in edges:
matrix[src][dest] = 1
return matrix
# Example edges and number of nodes
edges = [(0, 1), (0, 2), (1, 2), (2, 0), (2, 3)]
num_nodes = 4
adj_matrix = create_adjacency_matrix(num_nodes, edges)
for row in adj_matrix:
print(row)
Output:
[0, 1, 1, 0]
[0, 0, 1, 0]
[1, 0, 0, 1]
[0, 0, 0, 0]
Graph Traversal Techniques
Once the directed graph is created, you may need to traverse it for problems such as finding paths or detecting cycles. Common traversal algorithms include:
- Depth-First Search (DFS): Explores as far as possible along each branch before backtracking.
- Breadth-First Search (BFS): Explores all neighbors at the present depth prior to moving on to nodes at the next depth level.
DFS Example:
python
def dfs(graph, node, visited):
if node not in visited:
visited.add(node)
for neighbor in graph[node]:
dfs(graph, neighbor, visited)
visited = set()
dfs(graph, 0, visited)
print(visited)
BFS Example:
python
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
while queue:
node = queue.popleft()
if node not in visited:
visited.add(node)
queue.extend(graph[node])
return visited
bfs_result = bfs(graph, 0)
print(bfs_result)
Utilizing these structures and techniques allows efficient handling of directed graphs and can significantly aid in solving graph-related problems on LeetCode.
Creating Directed Graphs in Python: Expert Insights
Dr. Emily Chen (Data Structures Specialist, Tech Innovations Institute). “When constructing directed graphs in Python, utilizing libraries such as NetworkX can significantly streamline the process. This library provides built-in functions that allow for easy manipulation and visualization of graphs, which is essential for solving problems on platforms like LeetCode.”
Michael Thompson (Software Engineer, Algorithmic Solutions Inc.). “To effectively implement directed graphs for LeetCode challenges, it is crucial to understand the underlying data structures. Using adjacency lists is often more efficient in terms of space complexity, especially for sparse graphs, compared to adjacency matrices.”
Sarah Patel (Computer Science Educator, Code Academy). “I recommend starting with simple examples when learning how to create directed graphs in Python. By practicing with basic graph traversal algorithms such as Depth-First Search (DFS) or Breadth-First Search (BFS), learners can build a solid foundation before tackling more complex LeetCode problems.”
Frequently Asked Questions (FAQs)
How can I represent a directed graph in Python?
You can represent a directed graph in Python using various data structures, such as an adjacency list, adjacency matrix, or edge list. The adjacency list is commonly implemented using a dictionary where keys are nodes and values are lists of adjacent nodes.
What libraries can I use to create and manipulate directed graphs in Python?
Popular libraries for creating and manipulating directed graphs include NetworkX, Graph-tool, and igraph. NetworkX is particularly user-friendly and widely used in the LeetCode community for graph problems.
How do I implement a directed graph using an adjacency list in Python?
To implement a directed graph using an adjacency list, initialize a dictionary. For each directed edge from node `u` to node `v`, append `v` to the list of `u`. For example:
python
graph = {}
graph[u] = graph.get(u, []) + [v]
What is the time complexity of traversing a directed graph?
The time complexity of traversing a directed graph using Depth-First Search (DFS) or Breadth-First Search (BFS) is O(V + E), where V is the number of vertices and E is the number of edges in the graph.
How can I check for cycles in a directed graph using Python?
You can detect cycles in a directed graph using DFS with a recursion stack or by using Kahn’s algorithm for topological sorting. If you encounter a node that is already in the recursion stack during DFS, a cycle exists.
What are some common LeetCode problems involving directed graphs?
Common LeetCode problems involving directed graphs include Course Schedule, Course Schedule II, and Number of Islands. These problems often require knowledge of topological sorting, cycle detection, and graph traversal techniques.
Creating a directed graph in Python, especially in the context of solving problems on platforms like LeetCode, involves understanding both the data structures and algorithms that can effectively represent and manipulate such graphs. A directed graph, or digraph, consists of vertices connected by edges that have a direction, indicating a one-way relationship between nodes. In Python, directed graphs can be implemented using various data structures, with adjacency lists and adjacency matrices being the most common approaches.
When implementing a directed graph, the adjacency list is often preferred due to its efficient space utilization, particularly for sparse graphs. This structure allows for quick access to the neighbors of any given vertex, which is essential for performing graph traversal algorithms such as Depth-First Search (DFS) or Breadth-First Search (BFS). On LeetCode, understanding how to construct and manipulate these graphs is crucial for solving problems that involve topological sorting, cycle detection, or shortest path algorithms.
In addition to the basic implementation, Python’s libraries, such as NetworkX, can also facilitate graph creation and manipulation, providing built-in functions for common graph operations. However, for interview preparation or competitive programming, it is often beneficial to implement these structures from scratch to demonstrate a solid understanding of graph
Author Profile
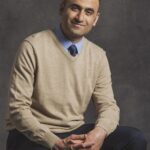
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?