How Can You Create an Empty List in Python?
In the world of programming, lists are one of the most versatile and widely used data structures, especially in Python. Whether you’re a seasoned developer or a beginner just starting your coding journey, understanding how to create and manipulate lists is fundamental to harnessing the full power of Python. Among the various operations you can perform with lists, knowing how to make an empty list is a crucial first step that opens the door to a myriad of possibilities.
Creating an empty list in Python is a straightforward task, yet it lays the groundwork for more complex data handling. An empty list serves as a blank canvas, allowing you to dynamically add, remove, or modify elements as your program evolves. This flexibility is particularly useful in scenarios where the size of your data is not predetermined, such as when collecting user input or processing data from external sources.
In this article, we will explore the different methods to create an empty list in Python, discuss their practical applications, and highlight best practices for using lists effectively. By mastering this simple yet essential skill, you’ll be better equipped to tackle a variety of programming challenges and enhance your coding proficiency. So, let’s dive in and unlock the potential of empty lists in Python!
Creating an Empty List in Python
In Python, creating an empty list is straightforward and can be accomplished in two primary ways. Understanding these methods is essential for effective list manipulation in your code.
Using Square Brackets
The most common method to create an empty list is by using square brackets. This syntax is simple and widely recognized in the Python community.
“`python
empty_list = []
“`
This line of code initializes a variable named `empty_list` with an empty list. You can then append elements to this list using methods such as `append()` or `extend()`.
Using the list() Constructor
Another method to create an empty list is by utilizing the built-in `list()` constructor. This approach can be particularly useful for those who prefer a more explicit or functional style.
“`python
empty_list = list()
“`
This line achieves the same result as the previous method. The choice between using square brackets and the `list()` constructor often comes down to personal or project-specific coding style preferences.
Comparative Overview
To better understand these methods, the following table summarizes their characteristics:
Method | Syntax | Notes |
---|---|---|
Square Brackets | empty_list = [] | Commonly used; concise and clear. |
list() Constructor | empty_list = list() | More explicit; may be preferred for readability. |
Adding Elements to an Empty List
Once you have created an empty list, you may want to add elements to it. Here are a few methods to do so:
- append(): Adds a single element to the end of the list.
“`python
empty_list.append(1)
“`
- extend(): Adds multiple elements (from an iterable) to the end of the list.
“`python
empty_list.extend([2, 3])
“`
- insert(): Inserts an element at a specified position in the list.
“`python
empty_list.insert(0, 0) Inserts 0 at the beginning
“`
Utilizing these methods allows for dynamic manipulation of lists, making them versatile for various programming needs.
Creating an Empty List in Python
In Python, creating an empty list can be accomplished in two primary ways. Both methods are straightforward and widely used in programming practices.
Using Square Brackets
The most common way to create an empty list is by using square brackets. This method is concise and clear, making it a preferred choice among Python developers.
“`python
empty_list = []
“`
This statement initializes `empty_list` as an empty list. You can then add elements to this list using methods such as `.append()` or list concatenation.
Using the list() Constructor
Another method to create an empty list is by using the built-in `list()` constructor. This approach is equally valid and can be useful in scenarios where you want to emphasize that you are creating a list.
“`python
empty_list = list()
“`
This code snippet also creates an empty list that can be populated later. The choice between using square brackets and the `list()` constructor often comes down to personal or team coding style preferences.
Examples of Adding Elements
Once you have created an empty list, you can easily add elements to it. Here are some examples:
- Using append() method:
“`python
empty_list.append(1)
empty_list.append(2)
“`
- Using extend() method:
“`python
empty_list.extend([3, 4])
“`
- Using list concatenation:
“`python
empty_list += [5, 6]
“`
After executing the above examples, `empty_list` will contain the elements `[1, 2, 3, 4, 5, 6]`.
Best Practices
When creating and managing empty lists, consider the following best practices:
- Initialization: Always initialize lists before using them to avoid `NameError`.
- Clarity: Use square brackets for simplicity when initializing an empty list.
- Avoid Mutability Issues: Be cautious when using mutable lists as default arguments in functions.
Common Use Cases
Empty lists are often used in various scenarios, including:
Use Case | Description |
---|---|
Collecting user input | Initialize a list to gather inputs in loops. |
Storing results | Create an empty list to store results from computations. |
Building data structures | Use empty lists as initial placeholders in complex data structures. |
These examples illustrate the versatility of empty lists in Python programming, allowing developers to structure their code efficiently and effectively.
Expert Insights on Creating Empty Lists in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Creating an empty list in Python is straightforward and can be accomplished using either the list constructor `list()` or by using square brackets `[]`. Both methods are equally valid, but using `[]` is more commonly preferred for its simplicity and readability.”
Mark Thompson (Software Engineer, CodeCraft Solutions). “When initializing an empty list in Python, it is crucial to understand its mutability. Lists are mutable data structures, meaning you can modify them after creation. This feature allows developers to dynamically add elements as needed, making lists an essential tool in Python programming.”
Linda Martinez (Python Educator, LearnPython Academy). “For beginners, I always recommend starting with the square brackets method to create an empty list. It not only helps in memorizing the syntax but also aligns with Python’s philosophy of simplicity and clarity in code.”
Frequently Asked Questions (FAQs)
How do I create an empty list in Python?
You can create an empty list in Python by using either the list constructor `list()` or by using square brackets `[]`. For example, `empty_list = []` or `empty_list = list()` will both result in an empty list.
What is the difference between using `[]` and `list()` to create an empty list?
There is no functional difference between using `[]` and `list()` to create an empty list. However, `[]` is generally preferred for its brevity and readability.
Can I add items to an empty list after creating it?
Yes, you can add items to an empty list using methods such as `append()`, `extend()`, or `insert()`. For instance, `empty_list.append(1)` will add the integer `1` to the list.
Is it possible to create a list with a specific size but no elements?
Yes, you can create a list with a specific size filled with `None` or any default value by using list comprehension. For example, `empty_list = [None] * 5` creates a list of size 5 with all elements set to `None`.
What are some common use cases for empty lists in Python?
Empty lists are commonly used as placeholders for data that will be populated later, for accumulating results in loops, or for initializing data structures before processing.
Can I check if a list is empty in Python?
Yes, you can check if a list is empty by using a simple conditional statement. For example, `if not my_list:` will evaluate to `True` if `my_list` is empty.
Creating an empty list in Python is a fundamental task that can be accomplished in a couple of straightforward ways. The most common method is by using empty square brackets, denoted as `my_list = []`. This method is simple and efficient, making it the preferred choice for many developers. Another approach is to use the built-in `list()` function, which can be invoked as `my_list = list()`. Both methods yield the same result, allowing you to initialize a list that can be populated with elements later in your code.
Understanding how to create an empty list is essential for effective programming in Python. Lists are versatile data structures that can store multiple items, making them invaluable for various applications, including data manipulation and algorithm implementation. By starting with an empty list, developers can dynamically add, remove, or modify elements as needed, which enhances the flexibility of their code.
In summary, the creation of an empty list in Python can be achieved through either the use of square brackets or the `list()` function. Both methods are equally valid and serve the same purpose. By mastering this foundational concept, programmers can leverage the full potential of lists in their projects, leading to more organized and efficient code.
Author Profile
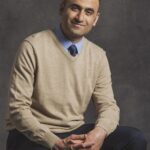
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?