How Can You Create a Global Variable in Python?
In the world of programming, the ability to manage data effectively is crucial for creating robust applications. One of the fundamental concepts in Python that every aspiring developer should grasp is the use of global variables. These variables, which can be accessed from any part of your code, offer a unique way to share information across different functions and modules. Whether you’re building a simple script or a complex software application, understanding how to implement global variables can enhance your coding efficiency and organization.
Global variables serve as a powerful tool for managing state and sharing data, but they come with their own set of challenges. In Python, declaring a global variable allows you to define a variable outside of any function, making it accessible throughout your program. This can simplify data handling in certain scenarios, especially when multiple functions need to read or modify the same information. However, the use of global variables can also lead to unintended side effects, such as making your code harder to debug and maintain, if not used judiciously.
As you delve deeper into the nuances of global variables in Python, you’ll discover best practices for their implementation, as well as potential pitfalls to avoid. From understanding the scope of variables to learning how to effectively manage state, mastering global variables will empower you to write cleaner, more efficient code. Join
Understanding Global Variables in Python
In Python, a global variable is a variable that is declared outside of any function and can be accessed throughout the entire program. This means that any function can read or modify the global variable, making it a useful tool for sharing information across different parts of your code.
Declaring a Global Variable
To create a global variable, simply declare it outside of any function. Here’s how you can do it:
“`python
global_var = “I am a global variable”
def my_function():
print(global_var)
my_function() Output: I am a global variable
“`
In this example, `global_var` is defined at the top level of the script, making it accessible to `my_function()`.
Modifying a Global Variable
If you need to modify a global variable within a function, you must declare it as global inside that function. This informs Python that you intend to use the global variable rather than creating a new local variable. Here’s an illustration:
“`python
global_var = 10
def modify_global():
global global_var Declare the variable as global
global_var += 5
modify_global()
print(global_var) Output: 15
“`
By using the `global` keyword, you can change the value of `global_var` inside `modify_global()`.
When to Use Global Variables
Global variables can be beneficial but should be used judiciously. Here are some guidelines:
- Use when necessary: If multiple functions need to share a state or configuration, a global variable may be appropriate.
- Avoid overuse: Excessive reliance on global variables can make debugging and understanding code more challenging.
- Consider alternatives: For many cases, passing parameters to functions or using classes can be more effective.
Best Practices for Global Variables
To ensure that your use of global variables is effective and manageable, consider the following best practices:
- Naming Conventions: Use uppercase letters to differentiate global variables from local variables.
- Limit Scope: Keep the number of global variables to a minimum to reduce complexity.
- Documentation: Clearly document the purpose of each global variable to aid readability.
Best Practice | Description |
---|---|
Naming Conventions | Use uppercase letters to identify global variables. |
Limit Scope | Reduce the number of global variables to maintain simplicity. |
Documentation | Clearly comment on the purpose of global variables. |
By adhering to these guidelines, you can effectively utilize global variables in Python while maintaining clean and efficient code.
Defining Global Variables
In Python, a global variable is defined outside of any function and can be accessed throughout the entire code file. To create a global variable, simply declare it at the top level of your script. For example:
“`python
my_global_var = 10
“`
This variable can now be accessed from any function within the same file.
Accessing Global Variables Within Functions
To use a global variable inside a function, you can reference it directly. However, if you need to modify its value, you must declare it as `global` within the function. Here’s how to do it:
“`python
my_global_var = 10
def my_function():
global my_global_var
my_global_var += 5
my_function()
print(my_global_var) Output: 15
“`
In this example, the `global` keyword allows the function to modify the global variable.
Best Practices for Global Variables
Using global variables can lead to code that is difficult to understand and maintain. To ensure clarity, consider the following best practices:
- Limit Usage: Use global variables sparingly and only when necessary.
- Clear Naming: Choose descriptive names to indicate the variable’s purpose.
- Encapsulation: Prefer passing variables to functions as arguments instead of relying on globals.
- Document Changes: Clearly document any modifications made to global variables within functions.
Example of Global Variable Usage
The following example illustrates the use of global variables in a simple program:
“`python
counter = 0 Global variable
def increment():
global counter
counter += 1
def reset():
global counter
counter = 0
increment()
increment()
print(counter) Output: 2
reset()
print(counter) Output: 0
“`
This code demonstrates how to increment and reset a global variable through function calls.
Global Variables in Modules
When working with multiple modules, global variables can be defined in one module and accessed in others. For example:
“`python
module_a.py
global_var = “Hello, World!”
module_b.py
from module_a import global_var
print(global_var) Output: Hello, World!
“`
This approach allows for a centralized definition of global variables, promoting reuse across multiple files.
Considerations for Thread Safety
In multi-threaded applications, global variables can lead to race conditions. To manage this, consider:
- Using Locks: Implement threading locks to ensure that only one thread can modify a global variable at a time.
- Immutable Structures: Use immutable data types (like tuples) or thread-local storage to avoid shared state issues.
By adhering to these guidelines, you can effectively manage global variables in Python while maintaining code clarity and safety.
Expert Insights on Creating Global Variables in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Creating global variables in Python is straightforward; you simply define a variable outside of any function. However, to modify that variable within a function, you must declare it as global using the ‘global’ keyword. This practice helps maintain clarity in your code, especially in larger projects.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “While global variables can be useful, they should be used sparingly. They can lead to code that is difficult to debug and maintain. Instead, consider using function parameters or return values to manage state more effectively.”
Sarah Thompson (Python Educator, LearnPython.org). “Understanding the scope of variables is crucial when working with global variables in Python. By defining a variable globally, you allow it to be accessed across different functions, but this can introduce risks of unintended side effects. Always document your global variables clearly to avoid confusion.”
Frequently Asked Questions (FAQs)
What is a global variable in Python?
A global variable in Python is a variable that is declared outside of any function or class and can be accessed throughout the entire program, including within functions.
How do I declare a global variable in Python?
To declare a global variable, simply define it outside of any function. For example: `my_variable = 10`. This variable can then be accessed anywhere in the code.
How can I modify a global variable inside a function?
To modify a global variable within a function, use the `global` keyword followed by the variable name. For example:
“`python
def my_function():
global my_variable
my_variable = 20
“`
Can I create a global variable inside a function?
No, you cannot create a global variable inside a function without using the `global` keyword. If you assign a value to a variable inside a function without declaring it global, it will be treated as a local variable.
What are the best practices for using global variables in Python?
Best practices include minimizing the use of global variables to reduce potential side effects, encapsulating variables within classes or modules, and using them only when necessary for shared state.
Are there any alternatives to global variables in Python?
Yes, alternatives include using function parameters and return values, utilizing class attributes, or employing a configuration object to manage shared state without relying on global variables.
In Python, global variables are defined outside of functions and can be accessed throughout the entire module. To create a global variable, you simply declare it at the top level of your script, outside any function definitions. This allows all functions within the module to read the variable without any additional declarations. However, if you need to modify a global variable within a function, you must use the `global` keyword to indicate that you are referring to the global variable, rather than creating a new local variable.
It is important to use global variables judiciously, as they can lead to code that is difficult to debug and maintain. Over-reliance on global variables can introduce side effects that complicate the flow of data within a program. Instead, consider using function parameters and return values to manage data flow more effectively. This approach promotes better encapsulation and reduces the risk of unintended interactions between different parts of your code.
In summary, while global variables can be useful for sharing state across functions, they should be used with caution. Understanding when and how to use the `global` keyword is crucial for maintaining clarity in your code. By prioritizing local variables and clear function interfaces, you can create more robust and maintainable Python programs.
Author Profile
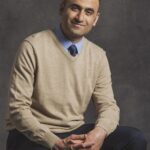
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?