How Do You Create a Python Script from Scratch?
In today’s digital age, the ability to automate tasks and solve problems with code is more valuable than ever. Python, renowned for its simplicity and versatility, has emerged as a favorite among both beginners and seasoned developers alike. Whether you’re looking to streamline your workflow, analyze data, or create a web application, learning how to make a Python script can open up a world of possibilities. This article will guide you through the essentials of scripting in Python, empowering you to harness the power of this programming language to bring your ideas to life.
Creating a Python script is not just about writing lines of code; it’s about understanding the logic behind programming and how to apply it to real-world scenarios. From setting up your development environment to writing your first lines of code, the process is both accessible and rewarding. With Python’s extensive libraries and frameworks, you can tackle a wide range of projects, whether you’re interested in web development, data analysis, or even game design.
As you embark on this journey, you’ll discover the fundamental concepts that underpin Python scripting, including variables, control structures, and functions. Each of these components plays a crucial role in building efficient and effective scripts. By the end of this article, you’ll not only have the knowledge to create your own Python scripts but also the
Setting Up Your Environment
To create a Python script, you first need to set up your development environment. This typically involves installing Python and a code editor. Here are the steps:
- Install Python: Download the latest version from the official [Python website](https://www.python.org/downloads/). Follow the installation instructions for your operating system.
- Choose a Code Editor: Options include:
- Visual Studio Code
- PyCharm
- Atom
- Sublime Text
After installation, ensure that Python is added to your system’s PATH environment variable, allowing you to run Python from the command line.
Creating Your First Script
Once your environment is set up, you can create your first Python script. Here’s how:
- Open your code editor.
- Create a new file and save it with a `.py` extension (e.g., `hello.py`).
- Write a simple Python program. For example:
“`python
print(“Hello, World!”)
“`
- Save the file.
Running the Script
To execute your Python script, follow these steps:
- Open a command line or terminal window.
- Navigate to the directory where your script is saved using the `cd` command.
- Run the script by typing:
“`
python hello.py
“`
This command will invoke the Python interpreter to execute your script, and you should see the output “Hello, World!” in the terminal.
Understanding the Script Structure
A Python script can include various components, such as:
- Comments: Lines that are not executed. They start with “.
- Variables: Used to store data.
- Functions: Blocks of reusable code.
- Control Structures: Such as loops (`for`, `while`) and conditionals (`if`, `else`).
Here is a simple table summarizing these components:
Component | Description |
---|---|
Comments | Used for documentation and explanation. |
Variables | Store data values. |
Functions | Reusable blocks of code that perform a specific task. |
Control Structures | Direct the flow of execution in the script. |
Debugging Your Script
Debugging is an essential part of script development. Common methods include:
- Print Statements: Insert print statements to track variable values and flow.
- Using a Debugger: Most code editors come with built-in debugging tools. Set breakpoints and inspect variables step-by-step.
- Error Messages: Pay attention to error messages in the terminal to identify issues in your code.
By following these steps and understanding these concepts, you can effectively create and run Python scripts tailored to your needs.
Choosing the Right Environment
Selecting the appropriate environment for your Python script is crucial for a smooth development process. Common options include:
- Local Development: Use an Integrated Development Environment (IDE) such as:
- PyCharm
- Visual Studio Code
- Jupyter Notebook (ideal for data analysis)
- Online IDEs: Useful for quick scripts or collaboration. Options include:
- Repl.it
- Google Colab (great for data science)
- Command Line Interface: You can also write scripts using a simple text editor (e.g., Notepad, Vim) and execute them via the terminal.
Setting Up the Python Environment
To begin writing your Python script, ensure that Python is installed on your system. Follow these steps:
- Download and Install Python:
- Visit the official Python website.
- Download the installer for your operating system.
- Follow the installation instructions, ensuring to check the box to add Python to your PATH.
- Verify Installation:
- Open your command line interface.
- Type `python –version` or `python3 –version` to confirm installation.
- Install Required Packages:
- Use `pip`, Python’s package manager, to install any additional libraries:
“`
pip install package_name
“`
Writing Your First Script
Creating a Python script involves a few simple steps. Here’s how to create a basic script:
- Create a New File:
- Use your chosen IDE or text editor to create a new file, naming it with a `.py` extension (e.g., `my_script.py`).
- Write Your Code:
- Start with a simple “Hello, World!” example:
“`python
print(“Hello, World!”)
“`
- Save Your File.
Running Your Script
Once your script is written, you can execute it using the command line:
- Navigate to the Script Location:
- Use the `cd` command to change directories to where your script is saved:
“`
cd path_to_your_script
“`
- Run the Script:
- Execute the script by typing:
“`
python my_script.py
“`
- For Python 3 specifically, use:
“`
python3 my_script.py
“`
Debugging Your Script
Debugging is an essential part of the coding process. Here are strategies for identifying and fixing errors:
- Print Statements: Use `print()` to output variable values at different points in the code.
- Error Messages: Read error messages carefully; they often indicate where the problem occurred.
- Python Debugger (pdb):
- Insert `import pdb; pdb.set_trace()` in your script to start an interactive debugging session.
Best Practices for Python Scripting
Adhering to best practices will enhance the quality and maintainability of your scripts:
- Code Organization:
- Use functions to encapsulate code blocks.
- Organize code logically and use comments to explain complex sections.
- Version Control:
- Use Git for tracking changes and collaborating with others.
- Documentation:
- Write docstrings for functions and modules to describe their purpose and usage.
- Testing:
- Implement unit tests to validate functionality using frameworks like `unittest` or `pytest`.
By following these guidelines, you can efficiently create and manage Python scripts that are functional and reliable.
Expert Insights on Creating Python Scripts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When creating a Python script, it is essential to start with a clear understanding of the problem you are trying to solve. This involves defining the requirements and breaking down the task into smaller, manageable components. A well-structured approach not only simplifies the coding process but also enhances code readability and maintainability.”
James Liu (Lead Python Developer, CodeCrafters). “Utilizing libraries and frameworks is a game-changer when developing Python scripts. By leveraging existing modules like NumPy or Pandas, you can significantly reduce development time and improve functionality. Always ensure to check the documentation for best practices and integration techniques.”
Sarah Thompson (Python Instructor, Code Academy). “For beginners, I recommend starting with simple scripts that automate everyday tasks. This hands-on experience is invaluable. Additionally, using version control systems like Git from the outset can help you track changes and collaborate effectively, which is crucial as your projects grow in complexity.”
Frequently Asked Questions (FAQs)
What are the basic steps to create a Python script?
To create a Python script, start by installing Python on your system. Next, open a text editor or an Integrated Development Environment (IDE) like PyCharm or VSCode. Write your Python code in the editor and save the file with a `.py` extension. Finally, run the script using the command line or the IDE’s built-in terminal.
How do I run a Python script from the command line?
To run a Python script from the command line, open your terminal or command prompt. Navigate to the directory where your script is saved using the `cd` command. Then, execute the script by typing `python script_name.py` or `python3 script_name.py`, depending on your Python installation.
What is the difference between a Python script and a Python module?
A Python script is a file containing Python code that is intended to be executed, while a Python module is a file that can be imported into other Python scripts. Modules typically contain functions, classes, and variables that can be reused across different scripts.
Can I create a Python script without any programming experience?
Yes, you can create a simple Python script without prior programming experience. Python’s syntax is designed to be intuitive and easy to learn. Numerous online resources, tutorials, and documentation are available to help beginners get started with writing scripts.
What are some common uses for Python scripts?
Python scripts are commonly used for automating tasks, data analysis, web scraping, creating web applications, and developing machine learning models. Their versatility makes them suitable for a wide range of applications across different industries.
How do I debug a Python script?
To debug a Python script, you can use print statements to check variable values and flow control. Alternatively, you can employ debugging tools like Python’s built-in `pdb` module or IDE features that allow step-by-step execution and inspection of code.
Creating a Python script involves several essential steps that enable developers to automate tasks, analyze data, or build applications. The process begins with setting up the Python environment, which includes installing Python and any necessary libraries or frameworks. Understanding the basic syntax and structure of Python is crucial, as it allows for writing clear and efficient code. Furthermore, utilizing an Integrated Development Environment (IDE) or a code editor can significantly enhance the coding experience by providing features like syntax highlighting, debugging tools, and code completion.
Once the environment is established, the next step is to define the purpose of the script. This involves identifying the problem to be solved or the task to be automated. Writing pseudocode can be beneficial at this stage, as it helps outline the logic and flow of the script without getting bogged down in syntax. After drafting the pseudocode, the actual coding can begin, where the developer translates the logic into Python code, ensuring to incorporate functions, loops, and conditionals as needed to achieve the desired functionality.
Testing and debugging are critical components of script development. After the initial coding, running the script to identify any errors or unexpected behavior is essential. Utilizing debugging tools and print statements can help isolate issues. Once the script runs successfully, documenting
Author Profile
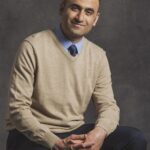
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?