How Can You Create a Table in Python? A Step-by-Step Guide!
Creating tables in Python is an essential skill for anyone looking to manipulate and present data effectively. Whether you’re a data analyst, a software developer, or a hobbyist programmer, understanding how to construct and manage tables can significantly enhance your ability to organize information and draw insightful conclusions. With Python’s rich ecosystem of libraries, you can easily create tables that are not only functional but also visually appealing, making your data more accessible and understandable.
In Python, there are several approaches to creating tables, each suited for different tasks and requirements. From simple text-based tables that can be printed in the console to more complex data structures that can be displayed in graphical user interfaces or web applications, the possibilities are vast. Libraries such as Pandas, PrettyTable, and Tabulate provide powerful tools for building tables, allowing you to focus on the data rather than the underlying code.
Moreover, the versatility of Python means that you can integrate tables into various applications, whether you’re working with data analysis, reporting, or even web development. As you delve deeper into the world of Python tables, you’ll discover techniques for customizing layouts, formatting data, and even exporting tables to different file formats. This article will guide you through the myriad ways to create tables in Python, equipping you with the knowledge to enhance your projects
Using Pandas to Create Tables
Pandas is a powerful data manipulation library in Python that provides easy methods for creating and managing tables, referred to as DataFrames. To begin using Pandas, you need to install it, which can be done using pip:
“`bash
pip install pandas
“`
Once installed, you can create a DataFrame in several ways, including from a dictionary, a list of lists, or a CSV file. Here’s a quick overview:
- From a Dictionary: Each key-value pair will represent a column in the DataFrame.
- From a List of Lists: Each inner list will represent a row in the DataFrame.
- From a CSV File: Load data directly from a CSV file into a DataFrame.
Here’s an example of creating a DataFrame from a dictionary:
“`python
import pandas as pd
data = {
‘Name’: [‘Alice’, ‘Bob’, ‘Charlie’],
‘Age’: [25, 30, 35],
‘City’: [‘New York’, ‘Los Angeles’, ‘Chicago’]
}
df = pd.DataFrame(data)
print(df)
“`
This will produce the following output:
“`
Name Age City
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 Chicago
“`
Creating Tables with PrettyTable
Another approach to creating tables in Python is by using the PrettyTable library, which is designed for printing ASCII tables in a visually appealing format. To install PrettyTable, use the following command:
“`bash
pip install prettytable
“`
After installation, you can create tables as follows:
“`python
from prettytable import PrettyTable
table = PrettyTable()
Adding columns
table.field_names = [“Name”, “Age”, “City”]
table.add_row([“Alice”, 25, “New York”])
table.add_row([“Bob”, 30, “Los Angeles”])
table.add_row([“Charlie”, 35, “Chicago”])
print(table)
“`
The output will look like this:
“`
+———+—–+————-+
Name | Age | City |
---|
+———+—–+————-+
Alice | 25 | New York |
---|---|---|
Bob | 30 | Los Angeles |
Charlie | 35 | Chicago |
+———+—–+————-+
“`
Creating HTML Tables Using Pandas
Pandas can also export DataFrames to HTML tables, making it easy to integrate with web applications. You can use the `to_html` method to convert your DataFrame to an HTML table format.
“`python
html_table = df.to_html()
print(html_table)
“`
This will generate an HTML representation of the DataFrame:
“`html
Name | Age | City | |
---|---|---|---|
0 | Alice | 25 | New York |
1 | Bob | 30 | Los Angeles |
2 | Charlie | 35 | Chicago |
“`
Utilizing these methods allows for flexible and efficient table creation in Python, accommodating various use cases from data analysis to web application development.
Creating Tables with Pandas
Using the Pandas library is one of the most efficient ways to create and manipulate tables in Python. Pandas provides a flexible data structure called a DataFrame, which is ideal for handling tabular data.
To create a DataFrame, you can use the following methods:
- From a Dictionary:
“`python
import pandas as pd
data = {
‘Name’: [‘Alice’, ‘Bob’, ‘Charlie’],
‘Age’: [25, 30, 35],
‘City’: [‘New York’, ‘Los Angeles’, ‘Chicago’]
}
df = pd.DataFrame(data)
“`
- From a List of Lists:
“`python
data = [
[‘Alice’, 25, ‘New York’],
[‘Bob’, 30, ‘Los Angeles’],
[‘Charlie’, 35, ‘Chicago’]
]
df = pd.DataFrame(data, columns=[‘Name’, ‘Age’, ‘City’])
“`
- From a CSV File:
“`python
df = pd.read_csv(‘file.csv’)
“`
The DataFrame can be displayed in a Jupyter notebook or printed in the console.
Using PrettyTable for Console Output
PrettyTable is a Python library designed for displaying tabular data in a visually appealing way in the console. To use PrettyTable, first install it via pip:
“`bash
pip install prettytable
“`
Here’s how to create and display a table using PrettyTable:
“`python
from prettytable import PrettyTable
Create a PrettyTable instance
table = PrettyTable()
Define the columns
table.field_names = [“Name”, “Age”, “City”]
Add rows
table.add_row([“Alice”, 25, “New York”])
table.add_row([“Bob”, 30, “Los Angeles”])
table.add_row([“Charlie”, 35, “Chicago”])
Print the table
print(table)
“`
This will render a neatly formatted table in the console.
Using Tabulate for Advanced Formatting
The Tabulate library offers even more formatting options than PrettyTable. To install Tabulate, use:
“`bash
pip install tabulate
“`
To create a table, you can use the following code:
“`python
from tabulate import tabulate
data = [
[“Alice”, 25, “New York”],
[“Bob”, 30, “Los Angeles”],
[“Charlie”, 35, “Chicago”]
]
Print table using tabulate
print(tabulate(data, headers=[“Name”, “Age”, “City”], tablefmt=”grid”))
“`
This will produce a well-structured table with grid lines.
Creating Tables with Matplotlib
For visual representation, Matplotlib can be used to create tables within plots. Here’s a simple example:
“`python
import matplotlib.pyplot as plt
data = [[‘Alice’, 25, ‘New York’],
[‘Bob’, 30, ‘Los Angeles’],
[‘Charlie’, 35, ‘Chicago’]]
Create a figure and axis
fig, ax = plt.subplots()
Hide axes
ax.axis(‘tight’)
ax.axis(‘off’)
Create the table
table = ax.table(cellText=data, colLabels=[“Name”, “Age”, “City”], cellLoc=’center’, loc=’center’)
Show the plot
plt.show()
“`
This code snippet will display a table within a Matplotlib plot, suitable for presentations and reports.
Summary of Libraries for Table Creation
Library | Use Case | Installation Command |
---|---|---|
Pandas | Data manipulation and analysis | `pip install pandas` |
PrettyTable | Console output | `pip install prettytable` |
Tabulate | Advanced console formatting | `pip install tabulate` |
Matplotlib | Visual representation in plots | `pip install matplotlib` |
Utilizing these libraries allows for versatile handling and presentation of tabular data in Python.
Expert Insights on Creating Tables in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “Creating tables in Python can be efficiently accomplished using libraries such as Pandas and PrettyTable. Pandas, in particular, offers powerful data manipulation capabilities, allowing users to create, modify, and visualize data tables with ease.”
Mark Thompson (Software Engineer, CodeCraft Solutions). “For those looking to create simple text-based tables, the PrettyTable library is an excellent choice. It provides a straightforward interface for generating ASCII tables that are both readable and customizable, making it ideal for console applications.”
Lisa Chen (Python Developer, DataViz Experts). “Using Jupyter Notebooks for Python programming allows for seamless integration of tables using the Pandas library. The ability to visualize DataFrames directly in the notebook enhances the understanding of data structures and facilitates better data analysis.”
Frequently Asked Questions (FAQs)
How can I create a simple table in Python using lists?
You can create a simple table by using lists of lists. Each inner list represents a row, and you can print the table using a loop. For example:
“`python
table = [[‘Name’, ‘Age’], [‘Alice’, 30], [‘Bob’, 25]]
for row in table:
print(‘\t’.join(map(str, row)))
“`
What libraries can I use to create tables in Python?
Several libraries facilitate table creation in Python, including `pandas`, `PrettyTable`, and `tabulate`. `pandas` is particularly useful for data manipulation and analysis, while `PrettyTable` and `tabulate` are great for formatting console output.
How do I create a table using pandas?
To create a table using `pandas`, first import the library and then create a DataFrame. For example:
“`python
import pandas as pd
data = {‘Name’: [‘Alice’, ‘Bob’], ‘Age’: [30, 25]}
df = pd.DataFrame(data)
print(df)
“`
Can I export a table created in Python to a CSV file?
Yes, you can easily export a table to a CSV file using the `to_csv()` method in `pandas`. For example:
“`python
df.to_csv(‘output.csv’, index=)
“`
How do I format a table for better readability in the console?
You can use the `PrettyTable` library to format tables for better readability in the console. After installing it, you can create a table as follows:
“`python
from prettytable import PrettyTable
table = PrettyTable()
table.field_names = [“Name”, “Age”]
table.add_row([“Alice”, 30])
table.add_row([“Bob”, 25])
print(table)
“`
Is it possible to create a table with dynamic data in Python?
Yes, you can create a table with dynamic data by collecting input from users or reading from a database or file. You can then populate a DataFrame or a list of lists with this data and display it accordingly.
Creating tables in Python can be accomplished through various libraries, each offering unique features and functionalities. The most commonly used libraries for this purpose include Pandas, PrettyTable, and tabulate. Pandas is particularly powerful for data manipulation and analysis, allowing users to create DataFrames that function as tables, complete with advanced indexing and data handling capabilities. PrettyTable and tabulate, on the other hand, are more focused on formatting tables for display in console applications, providing aesthetically pleasing outputs with minimal coding effort.
When utilizing Pandas, users can create tables by importing data from various sources such as CSV files, Excel spreadsheets, or even SQL databases. The library supports a wide range of operations, including filtering, grouping, and aggregating data, making it ideal for complex data analysis tasks. In contrast, PrettyTable and tabulate are best suited for simpler use cases where the emphasis is on presenting data rather than manipulating it. These libraries allow for easy customization of table styles, making them suitable for generating reports or displaying results in a user-friendly manner.
In summary, the choice of library depends on the specific needs of the user. For data analysis and manipulation, Pandas is the preferred choice due to its extensive functionality. For generating formatted tables
Author Profile
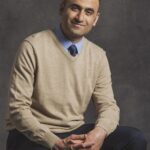
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?