How Can You Create Tables in Python Effectively?
Creating tables in Python is a fundamental skill that can significantly enhance your data organization and presentation capabilities. Whether you’re working on data analysis, web development, or even game design, the ability to construct tables efficiently can streamline your workflow and improve the readability of your output. In a world where data is king, mastering tables can empower you to convey information clearly and effectively, making it an essential tool in any Python programmer’s toolkit.
In Python, there are several libraries and methods available for creating tables, each catering to different needs and preferences. From simple text-based tables to complex, interactive data frames, the options are vast. Libraries like Pandas provide powerful tools for data manipulation and analysis, while others like PrettyTable and tabulate focus on creating visually appealing outputs for console applications. Understanding the strengths and limitations of each approach will help you choose the right one for your project.
Moreover, tables can serve various purposes, from displaying results in a user-friendly format to organizing data for further analysis. As you delve deeper into the world of Python tables, you’ll discover how to customize their appearance, manage data efficiently, and even export them to different formats. With the right techniques at your disposal, you can transform raw data into structured, meaningful insights that are easy to interpret and share.
Creating Tables Using Pandas
Pandas is a powerful library in Python that provides extensive capabilities for data manipulation and analysis, including easy-to-use functions for creating and managing tables. To create a table in Pandas, you typically start by importing the library and defining your data in a structured format, such as a dictionary or a list of lists.
Here’s a basic example of how to create a DataFrame, which is essentially a table in Pandas:
“`python
import pandas as pd
data = {
‘Name’: [‘Alice’, ‘Bob’, ‘Charlie’],
‘Age’: [25, 30, 35],
‘City’: [‘New York’, ‘Los Angeles’, ‘Chicago’]
}
df = pd.DataFrame(data)
print(df)
“`
This code snippet will produce the following table:
Name | Age | City |
---|---|---|
Alice | 25 | New York |
Bob | 30 | Los Angeles |
Charlie | 35 | Chicago |
Pandas also allows for various operations on the data frame, such as filtering, sorting, and aggregating. Here are some common functionalities:
- Filtering Rows: You can filter rows based on specific conditions.
- Sorting Data: Data can be sorted based on one or more columns.
- Group By: You can group data and perform aggregate operations.
Using PrettyTable for Simple Tables
If you require a straightforward way to create tables for console output, the PrettyTable library provides an easy-to-use interface. It is particularly useful for generating text-based tables.
First, install PrettyTable if it is not already available in your environment:
“`bash
pip install prettytable
“`
Here’s an example of how to use PrettyTable:
“`python
from prettytable import PrettyTable
table = PrettyTable()
Adding columns
table.field_names = [“Name”, “Age”, “City”]
table.add_row([“Alice”, 25, “New York”])
table.add_row([“Bob”, 30, “Los Angeles”])
table.add_row([“Charlie”, 35, “Chicago”])
print(table)
“`
This will produce a neatly formatted table in the console:
“`
+———+—–+————-+
Name | Age | City |
---|
+———+—–+————-+
Alice | 25 | New York |
---|---|---|
Bob | 30 | Los Angeles |
Charlie | 35 | Chicago |
+———+—–+————-+
“`
PrettyTable allows customization of the table’s appearance, such as alignment, border styles, and more.
Using Matplotlib for Visual Tables
For visual representation of data, Matplotlib can be utilized to create tables within plots. This is particularly useful when you want to display data as part of a figure.
Here’s an example of creating a simple table in a Matplotlib plot:
“`python
import matplotlib.pyplot as plt
Create a figure and axis
fig, ax = plt.subplots()
Hide axes
ax.axis(‘tight’)
ax.axis(‘off’)
Define data for the table
data = [
[‘Alice’, 25, ‘New York’],
[‘Bob’, 30, ‘Los Angeles’],
[‘Charlie’, 35, ‘Chicago’],
]
Create the table
table = ax.table(cellText=data, colLabels=[“Name”, “Age”, “City”], cellLoc = ‘center’, loc=’center’)
plt.show()
“`
This code will render a table as part of a Matplotlib figure, allowing for integration with other visual elements in your data presentation.
Using Pandas to Create Tables
Pandas is a powerful library in Python, widely used for data manipulation and analysis. To create tables, you typically work with DataFrames, which are two-dimensional labeled data structures.
- Installation: If Pandas is not already installed, you can install it using pip:
“`bash
pip install pandas
“`
- Creating a DataFrame: You can create a DataFrame from various data structures like lists, dictionaries, or even CSV files. Here’s an example of creating a DataFrame from a dictionary:
“`python
import pandas as pd
data = {
‘Name’: [‘Alice’, ‘Bob’, ‘Charlie’],
‘Age’: [25, 30, 35],
‘City’: [‘New York’, ‘Los Angeles’, ‘Chicago’]
}
df = pd.DataFrame(data)
print(df)
“`
- Output:
“`
Name Age City
0 Alice 25 New York
1 Bob 30 Los Angeles
2 Charlie 35 Chicago
“`
Displaying Tables with PrettyTable
PrettyTable is another library that allows for easy creation and display of ASCII tables in the terminal.
- Installation:
“`bash
pip install prettytable
“`
- Creating a Table:
“`python
from prettytable import PrettyTable
table = PrettyTable()
table.field_names = [“Name”, “Age”, “City”]
table.add_row([“Alice”, 25, “New York”])
table.add_row([“Bob”, 30, “Los Angeles”])
table.add_row([“Charlie”, 35, “Chicago”])
print(table)
“`
- Output:
“`
+———+—–+————-+
Name | Age | City |
---|
+———+—–+————-+
Alice | 25 | New York |
---|---|---|
Bob | 30 | Los Angeles |
Charlie | 35 | Chicago |
+———+—–+————-+
“`
Creating Tables with Matplotlib
Matplotlib is primarily a plotting library, but it also allows for table creation within figures.
- Installation:
“`bash
pip install matplotlib
“`
- Creating a Table:
“`python
import matplotlib.pyplot as plt
data = [[“Name”, “Age”, “City”],
[“Alice”, 25, “New York”],
[“Bob”, 30, “Los Angeles”],
[“Charlie”, 35, “Chicago”]]
fig, ax = plt.subplots()
ax.axis(‘tight’)
ax.axis(‘off’)
ax.table(cellText=data, cellLoc=’center’, loc=’center’)
plt.show()
“`
This code snippet generates a visual table embedded in a Matplotlib figure.
Using SQLAlchemy for Database Tables
SQLAlchemy is an ORM (Object Relational Mapper) that allows Python developers to interact with databases easily.
- Installation:
“`bash
pip install sqlalchemy
“`
- Creating a Table:
“`python
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
Base = declarative_base()
class User(Base):
__tablename__ = ‘users’
id = Column(Integer, primary_key=True)
name = Column(String)
age = Column(Integer)
city = Column(String)
engine = create_engine(‘sqlite:///:memory:’)
Base.metadata.create_all(engine)
Session = sessionmaker(bind=engine)
session = Session()
session.add(User(name=’Alice’, age=25, city=’New York’))
session.commit()
“`
This code snippet creates an in-memory SQLite database with a `users` table.
Formatting Tables for Output
When displaying tables, formatting can enhance readability. You can format tables in various ways, such as adjusting column width, aligning text, and using colors. Libraries such as `tabulate` can help achieve this.
- Installation:
“`bash
pip install tabulate
“`
- Using Tabulate:
“`python
from tabulate import tabulate
table = [[“Name”, “Age”, “City”],
[“Alice”, 25, “New York”],
[“Bob”, 30, “Los Angeles”],
[“Charlie”, 35, “Chicago”]]
print(tabulate(table, headers=’firstrow’, tablefmt=’grid’))
“`
- Output:
“`
+———+—–+————-+
Name | Age | City |
---|
+———+—–+————-+
Alice | 25 | New York |
---|---|---|
Bob | 30 | Los Angeles |
Charlie | 35 | Chicago |
+———+—–+————-+
“`
Expert Insights on Creating Tables in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Utilizing libraries such as Pandas is essential for efficiently creating and manipulating tables in Python. The DataFrame structure allows for intuitive data handling and is widely adopted in the industry for data analysis tasks.”
Michael Chen (Software Engineer, CodeCraft Solutions). “For developers looking to present data in a more visual format, libraries like Matplotlib and Seaborn can be integrated to enhance tables with graphical representations. This approach not only improves readability but also aids in data interpretation.”
Sarah Thompson (Python Instructor, LearnPython Academy). “When teaching newcomers how to make tables in Python, I emphasize the importance of understanding both the data structure and the libraries available. Mastery of NumPy and Pandas will empower learners to create dynamic tables efficiently.”
Frequently Asked Questions (FAQs)
How can I create a simple table in Python using lists?
You can create a simple table by using nested lists or tuples. For example, define a list of lists, where each inner list represents a row. You can then print the table using a loop.
What libraries can I use to create tables in Python?
Popular libraries for creating tables in Python include Pandas for data manipulation, PrettyTable for ASCII tables, and Tabulate for formatted output. Each library has its own features and use cases.
How do I create a DataFrame table using Pandas?
To create a DataFrame, import the Pandas library and use the `pd.DataFrame()` function, passing in a dictionary or a list of lists. This allows you to structure your data in a tabular format.
Can I format tables in Python for better readability?
Yes, you can format tables using libraries like PrettyTable or Tabulate, which provide options for aligning text, setting column widths, and adding borders to enhance readability.
Is it possible to export tables created in Python to Excel or CSV formats?
Yes, using Pandas, you can easily export DataFrames to Excel using the `to_excel()` method or to CSV using the `to_csv()` method. This facilitates data sharing and reporting.
How can I visualize tables in Python?
You can visualize tables using libraries like Matplotlib or Seaborn. These libraries allow you to create graphical representations of your data, making it easier to analyze and interpret.
Creating tables in Python can be accomplished through various libraries and methods, each suited for different needs and contexts. Popular libraries such as Pandas, PrettyTable, and Tabulate offer distinct functionalities that cater to data manipulation, display, and output formatting. Pandas is particularly powerful for handling large datasets and performing complex data analysis, while PrettyTable and Tabulate excel in generating well-formatted tables for console output or reports.
When using Pandas, the DataFrame structure allows for easy creation and manipulation of tabular data. Users can import data from various sources, such as CSV files or databases, and perform operations like filtering, sorting, and aggregating. In contrast, PrettyTable and Tabulate are more focused on formatting and presenting data in a visually appealing manner, making them ideal for quick outputs in terminal applications.
the choice of method to create tables in Python largely depends on the specific requirements of the task at hand. For data analysis and manipulation, Pandas is the go-to library, while PrettyTable and Tabulate are excellent for generating readable outputs. Understanding the strengths and limitations of each library will enable users to select the most appropriate tool for their needs, ultimately enhancing their productivity and efficiency in data handling.
Author Profile
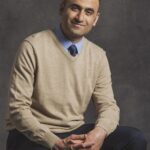
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?