How Can You Create a Tic Tac Toe Game in Python?
Tic Tac Toe, a classic game that has entertained generations, is not just a simple pastime; it’s also a fantastic project for budding programmers. Whether you’re a novice looking to dip your toes into the world of coding or an experienced developer seeking a fun challenge, creating a Tic Tac Toe game in Python is an excellent way to hone your skills. This project combines logic, user interaction, and basic programming concepts, making it an ideal starting point for anyone eager to learn.
In this article, we will explore the fundamentals of building a Tic Tac Toe game using Python. You’ll discover how to set up the game board, manage player turns, and implement win conditions. The beauty of this project lies in its simplicity, yet it offers ample opportunities to expand and customize your game, allowing you to experiment with different features and enhancements. By the end of this journey, you will not only have a fully functional game but also a deeper understanding of programming principles that can be applied to more complex projects.
Join us as we unravel the steps to create your own Tic Tac Toe game in Python, turning a beloved childhood game into a hands-on coding experience. Whether you’re looking to impress friends with your programming prowess or simply enjoy the satisfaction of building something from scratch, this guide
Setting Up the Game Board
To create a Tic Tac Toe game in Python, you first need to set up the game board. This can be accomplished using a 2D list, which will represent the grid. The board will consist of three rows and three columns, initialized with empty strings or placeholders.
“`python
board = [[‘ ‘ for _ in range(3)] for _ in range(3)]
“`
This code snippet creates a 3×3 grid filled with spaces. Each space will later hold either ‘X’ or ‘O’ depending on the player’s move.
Displaying the Board
An essential feature of the game is the ability to display the current state of the board. You can define a function that prints the board in a user-friendly format. The function iterates through the rows of the board, printing each row with dividers.
“`python
def print_board(board):
for row in board:
print(‘|’.join(row))
print(‘-‘ * 5)
“`
This function outputs the game board with vertical dividers between columns and horizontal lines separating the rows, making it visually clear.
Handling Player Input
To allow players to make moves, you need to create a function that takes input for the row and column where they want to place their marker. Input validation is crucial to ensure players do not overwrite existing moves or choose invalid positions.
“`python
def player_move(board, player):
while True:
try:
row = int(input(f”Player {player}, enter row (0-2): “))
col = int(input(f”Player {player}, enter column (0-2): “))
if board[row][col] == ‘ ‘:
board[row][col] = player
break
else:
print(“Invalid move. Try again.”)
except (ValueError, IndexError):
print(“Invalid input. Please enter numbers between 0 and 2.”)
“`
This function prompts the player for their move and checks if the selected position is valid.
Checking for a Winner
To determine the outcome of the game, implement a function that checks for a winner after each move. The function should check all possible winning combinations: rows, columns, and diagonals.
“`python
def check_winner(board):
for row in board:
if row[0] == row[1] == row[2] != ‘ ‘:
return row[0]
for col in range(3):
if board[0][col] == board[1][col] == board[2][col] != ‘ ‘:
return board[0][col]
if board[0][0] == board[1][1] == board[2][2] != ‘ ‘:
return board[0][0]
if board[0][2] == board[1][1] == board[2][0] != ‘ ‘:
return board[0][2]
return None
“`
This function returns the player who has won or `None` if there is no winner yet.
Putting It All Together
Finally, the main game loop coordinates the flow of the game. Players alternate turns until there is a winner or the board is full.
“`python
def tic_tac_toe():
board = [[‘ ‘ for _ in range(3)] for _ in range(3)]
current_player = ‘X’
for _ in range(9):
print_board(board)
player_move(board, current_player)
if check_winner(board):
print_board(board)
print(f”Player {current_player} wins!”)
return
current_player = ‘O’ if current_player == ‘X’ else ‘X’
print_board(board)
print(“It’s a draw!”)
“`
This function initializes the game, handles player turns, and checks for a winner after each move.
Function | Description |
---|---|
print_board | Displays the current state of the game board. |
player_move | Handles the player’s input and updates the board. |
check_winner | Checks the board for a winning condition. |
tic_tac_toe | Main loop to manage the game’s flow. |
Setting Up the Environment
To create a Tic Tac Toe game in Python, you first need to ensure your development environment is ready. Follow these steps:
- Install Python: Download and install the latest version of Python from the official website.
- Text Editor or IDE: Choose a text editor (such as Visual Studio Code, Sublime Text) or an Integrated Development Environment (IDE) like PyCharm to write your code.
- Terminal/Command Line: Familiarize yourself with using the terminal or command line for running your Python scripts.
Creating the Game Board
The game board can be represented as a list of lists in Python. This structure enables easy manipulation of the board’s state. Here is how you can define it:
“`python
def initialize_board():
return [[‘ ‘ for _ in range(3)] for _ in range(3)]
“`
- Each element represents a cell on the board.
- The initial state is filled with spaces to indicate empty cells.
Displaying the Board
To visualize the game board in the console, create a function to print the current state:
“`python
def display_board(board):
for row in board:
print(‘|’.join(row))
print(‘-‘ * 5)
“`
- This function iterates through each row, joining the elements with a vertical bar and adding horizontal dividers for clarity.
Player Input and Move Validation
Capture player moves and ensure they are valid. Here’s a function to handle player input:
“`python
def get_player_move(board, player):
while True:
try:
move = int(input(f”Player {player}, enter your move (1-9): “)) – 1
row, col = divmod(move, 3)
if board[row][col] == ‘ ‘:
board[row][col] = player
break
else:
print(“Cell is already taken. Try again.”)
except (ValueError, IndexError):
print(“Invalid move. Please enter a number between 1 and 9.”)
“`
- This function checks if the chosen cell is empty and handles invalid inputs gracefully.
Win Condition Check
To determine if a player has won the game, implement a function to check for winning conditions:
“`python
def check_winner(board):
Check rows, columns, and diagonals
for i in range(3):
if board[i][0] == board[i][1] == board[i][2] != ‘ ‘:
return board[i][0]
if board[0][i] == board[1][i] == board[2][i] != ‘ ‘:
return board[0][i]
if board[0][0] == board[1][1] == board[2][2] != ‘ ‘:
return board[0][0]
if board[0][2] == board[1][1] == board[2][0] != ‘ ‘:
return board[0][2]
return None
“`
- This function checks all possible winning combinations on the board.
Game Loop
The main game loop coordinates player turns and checks for victory conditions:
“`python
def play_game():
board = initialize_board()
current_player = ‘X’
for turn in range(9): Maximum of 9 moves
display_board(board)
get_player_move(board, current_player)
if check_winner(board):
display_board(board)
print(f”Player {current_player} wins!”)
return
current_player = ‘O’ if current_player == ‘X’ else ‘X’
display_board(board)
print(“It’s a tie!”)
“`
- The game alternates between players and displays the board after each move.
Running the Game
To run the game, simply call the `play_game()` function:
“`python
if __name__ == “__main__”:
play_game()
“`
- This starts the Tic Tac Toe game in the console, allowing two players to take turns.
Expert Insights on Creating Tic Tac Toe in Python
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “When developing a Tic Tac Toe game in Python, it’s essential to focus on modular code design. This approach not only enhances readability but also simplifies debugging and future feature additions.”
Mark Thompson (Lead Game Developer, PixelPlay Studios). “Implementing a user-friendly interface is crucial for engaging players. Utilizing libraries like Pygame can significantly enhance the visual appeal and interactivity of your Tic Tac Toe game.”
Linda Zhang (Computer Science Educator, TechEd Academy). “It’s beneficial to incorporate artificial intelligence into your Tic Tac Toe project. By implementing a simple minimax algorithm, you can create a challenging opponent that enhances the learning experience for beginners.”
Frequently Asked Questions (FAQs)
How do I start coding a Tic Tac Toe game in Python?
Begin by setting up a basic structure using a list to represent the game board. Initialize the board with empty strings or spaces and create functions to display the board, check for a win, and switch turns between players.
What libraries do I need to create a Tic Tac Toe game in Python?
You can create a simple console-based Tic Tac Toe game using only Python’s built-in capabilities. For graphical interfaces, consider using libraries like Tkinter or Pygame.
How can I check for a win in Tic Tac Toe using Python?
To check for a win, create a function that evaluates all possible winning combinations (rows, columns, and diagonals) and returns true if any combination is fulfilled by the same player’s marker.
What is the best way to handle user input in a Tic Tac Toe game?
Use the `input()` function to capture user moves and validate the input to ensure it corresponds to an empty cell on the board. Implement error handling to manage invalid inputs gracefully.
How can I implement a computer player in my Tic Tac Toe game?
You can implement a simple algorithm for the computer player, such as random move selection or a more advanced strategy like the Minimax algorithm for optimal play.
Is it possible to add a graphical user interface (GUI) to my Tic Tac Toe game?
Yes, you can use libraries like Tkinter or Pygame to create a GUI for your Tic Tac Toe game, allowing for a more interactive and visually appealing experience for users.
creating a Tic Tac Toe game in Python involves understanding the fundamental concepts of programming, including the use of functions, loops, and conditionals. The game can be implemented in a console-based format, allowing for user input and displaying the game board after each move. A simple yet effective approach is to represent the board using a list or a 2D array, which enables easy tracking of player moves and checking for win conditions.
Key takeaways from the discussion include the importance of structuring the code for clarity and maintainability. Implementing functions for tasks such as displaying the board, checking for a win, and handling player input can significantly enhance the organization of the code. Additionally, incorporating error handling ensures that the game runs smoothly, preventing invalid moves and maintaining a positive user experience.
Furthermore, expanding the basic version of Tic Tac Toe can lead to valuable learning opportunities. For instance, adding features such as a graphical user interface (GUI) using libraries like Tkinter or Pygame can provide a more engaging experience. Exploring artificial intelligence to allow the computer to play against the user can also deepen one’s understanding of algorithms and game logic.
Author Profile
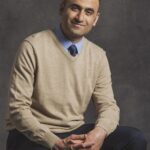
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?