How Can You Create Two Plots Side by Side in Python?
Creating visual representations of data is an essential aspect of data analysis and presentation in Python. Among the myriad of tools available, the ability to display two plots side by side can significantly enhance the clarity and impact of your findings. Whether you’re comparing datasets, showcasing different visualizations of the same data, or simply looking to create a more organized presentation, mastering the technique of side-by-side plotting is a valuable skill for any data enthusiast or professional.
In the world of Python, libraries like Matplotlib and Seaborn provide powerful functionalities to create stunning visualizations with ease. By leveraging these libraries, you can not only create individual plots but also arrange them in a grid-like structure, allowing for seamless comparisons and a more cohesive storytelling approach. This method is particularly useful in exploratory data analysis, where visualizing multiple aspects of the data simultaneously can lead to deeper insights.
As you delve into the nuances of side-by-side plotting, you’ll discover various techniques to customize the appearance and layout of your visualizations. From adjusting figure sizes to fine-tuning axes and labels, the possibilities are vast. Whether you’re a beginner eager to learn or an experienced programmer looking to refine your skills, understanding how to make two plots side by side will undoubtedly elevate your data visualization game. Prepare to unlock the full potential of your
Using Matplotlib for Side-by-Side Plots
Matplotlib is one of the most widely used libraries in Python for creating static, animated, and interactive visualizations. To create two plots side by side, you can utilize the `subplots` function, which allows you to arrange multiple plots in a grid.
To create side-by-side plots, follow these steps:
- Import the necessary libraries:
“`python
import matplotlib.pyplot as plt
import numpy as np
“`
- Prepare your data:
For example, you can generate some sample data using NumPy:
“`python
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
“`
- Create subplots:
Use `plt.subplots()` to create a figure and axes for your plots. Specify the number of rows and columns to arrange your plots:
“`python
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(10, 5))
“`
- Plot your data on the respective axes:
“`python
ax1.plot(x, y1, label=’Sine Wave’, color=’blue’)
ax1.set_title(‘Sine Function’)
ax1.set_xlabel(‘X-axis’)
ax1.set_ylabel(‘Y-axis’)
ax1.legend()
ax2.plot(x, y2, label=’Cosine Wave’, color=’orange’)
ax2.set_title(‘Cosine Function’)
ax2.set_xlabel(‘X-axis’)
ax2.set_ylabel(‘Y-axis’)
ax2.legend()
“`
- Display the plots:
Finally, use `plt.tight_layout()` for better spacing and `plt.show()` to display the plots:
“`python
plt.tight_layout()
plt.show()
“`
This code will produce two plots side by side, each with its own title, labels, and legends, providing a clear comparison between the sine and cosine functions.
Customizing Plot Layouts
To further customize the appearance of your side-by-side plots, you can adjust various parameters:
- Figure Size: Change the `figsize` parameter in `plt.subplots()` to modify the overall size of the plot.
- Gridlines: Use `ax.grid()` to add gridlines to your plots for better readability.
- Colors and Styles: Customize the line colors and styles using additional parameters in the `plot` function.
Here’s an example of a customized layout:
“`python
fig, (ax1, ax2) = plt.subplots(1, 2, figsize=(12, 6))
ax1.plot(x, y1, label=’Sine Wave’, color=’blue’, linestyle=’–‘, linewidth=2)
ax2.plot(x, y2, label=’Cosine Wave’, color=’orange’, linestyle=’-‘, linewidth=2)
for ax in (ax1, ax2):
ax.grid(True)
ax.legend()
“`
Table of Common Customization Options
Customization | Parameter | Example |
---|---|---|
Figure Size | figsize | (12, 6) |
Line Color | color | ‘blue’ |
Line Style | linestyle | ‘–‘ |
Line Width | linewidth | 2 |
By leveraging these customization options, you can create visually appealing and informative side-by-side plots that enhance the clarity of your data presentation.
Using Matplotlib for Side-by-Side Plots
To create two plots side by side in Python, the Matplotlib library is one of the most commonly used tools. By utilizing the `subplots` function, you can effectively manage the layout of multiple plots within a single figure.
- Basic Example:
“`python
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
fig, (ax1, ax2) = plt.subplots(1, 2) 1 row, 2 columns
ax1.plot(x, y1)
ax1.set_title(‘Sine Wave’)
ax2.plot(x, y2)
ax2.set_title(‘Cosine Wave’)
plt.show()
“`
The `subplots` function takes two parameters to define the number of rows and columns. In this case, `1, 2` indicates one row and two columns, allowing for side-by-side plots.
Adjusting the Layout
To enhance the appearance and spacing of the plots, you can use the `tight_layout()` function or the `fig.subplots_adjust()` method. This helps to minimize overlapping titles and labels.
- Using `tight_layout()`:
“`python
plt.tight_layout()
“`
- Using `subplots_adjust()`:
“`python
fig.subplots_adjust(wspace=0.3) Adjusts the width space between plots
“`
Creating Side-by-Side Plots with Seaborn
Seaborn, built on top of Matplotlib, offers a more straightforward syntax for creating visually appealing statistical graphics. You can also generate side-by-side plots using the `FacetGrid` function.
- Example:
“`python
import seaborn as sns
import pandas as pd
Sample data
data = pd.DataFrame({
‘x’: np.linspace(0, 10, 100),
‘y1’: np.sin(np.linspace(0, 10, 100)),
‘y2′: np.cos(np.linspace(0, 10, 100))
})
g = sns.FacetGrid(data, col=’variable’, hue=’variable’, height=5)
g.map(sns.lineplot, ‘x’, ‘y1’)
g.map(sns.lineplot, ‘x’, ‘y2’)
plt.show()
“`
In this example, `FacetGrid` creates a grid of plots based on the categorical variable you specify.
Using Plotly for Interactive Side-by-Side Plots
For interactive visualizations, Plotly provides an intuitive way to create side-by-side plots using `make_subplots`.
- Example:
“`python
import plotly.graph_objects as go
from plotly.subplots import make_subplots
fig = make_subplots(rows=1, cols=2)
fig.add_trace(go.Scatter(x=x, y=y1, mode=’lines’, name=’Sine Wave’), row=1, col=1)
fig.add_trace(go.Scatter(x=x, y=y2, mode=’lines’, name=’Cosine Wave’), row=1, col=2)
fig.update_layout(title_text=’Side-by-Side Plots’)
fig.show()
“`
The `make_subplots` function allows you to specify the number of rows and columns, similar to Matplotlib, while enabling interactive features.
Summary of Key Functions
Library | Function | Description |
---|---|---|
Matplotlib | `plt.subplots()` | Create a grid of subplots |
Matplotlib | `plt.tight_layout()` | Adjust layout to minimize overlaps |
Seaborn | `sns.FacetGrid()` | Create a grid of plots based on categories |
Plotly | `make_subplots()` | Create interactive grid of subplots |
This structured approach to creating side-by-side plots across different libraries allows for flexibility depending on the requirements of your data visualization tasks.
Expert Insights on Creating Side-by-Side Plots in Python
Dr. Emily Chen (Data Visualization Specialist, Tech Data Insights). “To effectively create two plots side by side in Python, utilizing the Matplotlib library is highly recommended. By employing the `subplots` function, users can easily specify the number of rows and columns for their plots, allowing for a clear and organized presentation of data.”
Mark Thompson (Senior Data Scientist, Analytics Pro). “When aiming to compare datasets visually, using the `plt.subplots()` method in Matplotlib not only provides flexibility in layout but also enhances readability. This approach allows for individual customization of each subplot, which is crucial for detailed analysis.”
Lisa Patel (Python Programming Educator, Code Academy). “For beginners, I recommend starting with the `subplot` function for creating side-by-side plots. It simplifies the process of arranging multiple visualizations and can be easily adapted for more complex layouts as users become more proficient in Python.”
Frequently Asked Questions (FAQs)
How can I create two plots side by side using Matplotlib?
You can use the `subplot` function in Matplotlib to create two plots side by side. For example, use `plt.subplot(1, 2, 1)` for the first plot and `plt.subplot(1, 2, 2)` for the second plot.
What is the difference between `subplot` and `subplots` in Matplotlib?
`subplot` creates a single subplot in a specified grid, while `subplots` creates an entire grid of subplots at once and returns both the figure and axes objects, making it easier to manage multiple plots.
Can I adjust the spacing between the side-by-side plots?
Yes, you can adjust spacing using `plt.subplots_adjust(left, right, top, bottom, wspace, hspace)` where `wspace` controls the width space between subplots.
Is it possible to plot different types of charts side by side?
Yes, you can plot different types of charts side by side by specifying different plotting functions for each subplot, such as `plt.bar()` for a bar chart and `plt.plot()` for a line chart.
What libraries can I use besides Matplotlib for side-by-side plots?
You can use libraries like Seaborn and Plotly, which provide functions to create side-by-side plots with ease. Seaborn’s `FacetGrid` and Plotly’s subplots feature are particularly useful.
How do I save the figure with side-by-side plots?
You can save the figure using `plt.savefig(‘filename.png’)` after creating your plots. Ensure to call this function before `plt.show()` to save the figure correctly.
Creating two plots side by side in Python is a common task that can be accomplished using various libraries, with Matplotlib being one of the most popular choices. By utilizing the `subplots` function, users can easily define a grid layout for their plots, allowing for effective comparison and visualization of data. This function provides flexibility in configuring the number of rows and columns for the subplots, making it straightforward to arrange multiple visualizations in a single figure.
In addition to Matplotlib, other libraries such as Seaborn and Plotly also offer functionalities for side-by-side plotting. Seaborn, built on top of Matplotlib, simplifies the process of creating aesthetically pleasing plots, while Plotly provides interactive capabilities that enhance user engagement. Depending on the specific requirements of the project—such as the need for interactivity or advanced statistical visualizations—users can choose the library that best fits their needs.
Furthermore, it is essential to consider the layout and aesthetics of the plots when displaying them side by side. Adjusting parameters such as figure size, spacing, and axis labels can significantly improve the clarity and impact of the visualizations. By paying attention to these details, users can create professional-quality figures that effectively communicate their data insights.
Author Profile
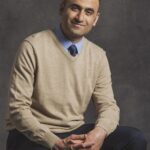
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?