How Can You Effectively Merge Dictionaries in Python?
In the world of Python programming, dictionaries stand out as one of the most versatile and powerful data structures. They allow developers to store and manipulate data in key-value pairs, making it easy to access and organize information efficiently. However, as projects grow in complexity, the need to combine multiple dictionaries often arises. Whether you’re aggregating data from different sources, merging configurations, or simply looking to streamline your code, understanding how to merge dictionaries effectively is essential for any Python programmer.
Merging dictionaries in Python is a straightforward yet crucial task that can enhance your coding efficiency and clarity. With various methods available, from using built-in functions to leveraging the latest syntax introduced in recent Python versions, developers have multiple options at their disposal. Each method offers unique advantages, whether it’s for maintaining data integrity, ensuring readability, or optimizing performance.
As you delve deeper into the nuances of merging dictionaries, you’ll discover not only the mechanics of each approach but also best practices that can elevate your programming skills. From simple merges to more complex scenarios involving nested dictionaries, mastering this skill will empower you to handle data with confidence and precision in your Python projects. Get ready to unlock the full potential of dictionaries and streamline your coding experience!
Using the Update Method
The `update()` method allows you to merge two dictionaries by adding the key-value pairs of one dictionary into another. If there are overlapping keys, the values from the second dictionary will overwrite those in the first. This method modifies the original dictionary in place.
“`python
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘b’: 3, ‘c’: 4}
dict1.update(dict2)
print(dict1) Output: {‘a’: 1, ‘b’: 3, ‘c’: 4}
“`
Using the Merge Operator (Python 3.9 and above)
Starting from Python 3.9, you can use the merge operator (`|`) to combine dictionaries. This operator creates a new dictionary that includes the keys and values from both dictionaries, prioritizing the second dictionary in case of key collisions.
“`python
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘b’: 3, ‘c’: 4}
merged_dict = dict1 | dict2
print(merged_dict) Output: {‘a’: 1, ‘b’: 3, ‘c’: 4}
“`
Using Dictionary Comprehension
Another flexible way to merge dictionaries is through dictionary comprehension. This method can be particularly useful for applying conditions or transformations while merging.
“`python
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘b’: 3, ‘c’: 4}
merged_dict = {k: v for d in (dict1, dict2) for k, v in d.items()}
print(merged_dict) Output: {‘a’: 1, ‘b’: 3, ‘c’: 4}
“`
Using the ChainMap Class from Collections
The `ChainMap` class from the `collections` module provides a way to group multiple dictionaries together. It doesn’t create a new merged dictionary but allows you to treat multiple dictionaries as a single unit.
“`python
from collections import ChainMap
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘b’: 3, ‘c’: 4}
merged_dict = ChainMap(dict2, dict1)
print(merged_dict[‘b’]) Output: 3
print(dict(merged_dict)) Output: {‘b’: 3, ‘c’: 4, ‘a’: 1}
“`
Comparison of Dictionary Merging Methods
The following table summarizes the different methods for merging dictionaries, including their characteristics:
Method | Python Version | Returns New Dictionary | Overwrites Values |
---|---|---|---|
update() | All | No | Yes |
Merge Operator (|) | 3.9+ | Yes | Yes |
Dictionary Comprehension | All | Yes | Yes |
ChainMap | All | No | No |
Each method has its own advantages and use cases, allowing developers to choose the one that best fits their needs.
Merging Dictionaries in Python
In Python, merging dictionaries can be accomplished using various methods depending on the version of Python being utilized. Below are the most common approaches to merge dictionaries effectively.
Using the `update()` Method
The `update()` method allows you to merge one dictionary into another. The original dictionary will be modified, and any overlapping keys will have their values updated with the values from the second dictionary.
“`python
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘b’: 3, ‘c’: 4}
dict1.update(dict2)
print(dict1) Output: {‘a’: 1, ‘b’: 3, ‘c’: 4}
“`
Using the `|` Operator (Python 3.9 and later)
Python 3.9 introduced the `|` operator, which allows for a more concise syntax to merge dictionaries. This approach creates a new dictionary without modifying the originals.
“`python
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘b’: 3, ‘c’: 4}
merged_dict = dict1 | dict2
print(merged_dict) Output: {‘a’: 1, ‘b’: 3, ‘c’: 4}
“`
Using Dictionary Comprehension
Dictionary comprehension provides a flexible way to merge dictionaries, allowing for custom logic during the merge process.
“`python
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘b’: 3, ‘c’: 4}
merged_dict = {k: v for d in (dict1, dict2) for k, v in d.items()}
print(merged_dict) Output: {‘a’: 1, ‘b’: 3, ‘c’: 4}
“`
Using the `**` Unpacking Operator
The unpacking operator `**` can be used to merge dictionaries in a concise manner. This approach works in Python 3.5 and later.
“`python
dict1 = {‘a’: 1, ‘b’: 2}
dict2 = {‘b’: 3, ‘c’: 4}
merged_dict = {dict1, dict2}
print(merged_dict) Output: {‘a’: 1, ‘b’: 3, ‘c’: 4}
“`
Handling Key Conflicts
When merging dictionaries, it is essential to consider how to handle key conflicts. The methods above will always take the value from the latter dictionary when keys overlap. Here are some strategies to manage conflicts:
- Keep the first value:
“`python
merged_dict = {k: dict1.get(k, dict2.get(k)) for k in set(dict1) | set(dict2)}
“`
- Combine values into a list:
“`python
merged_dict = {}
for d in (dict1, dict2):
for k, v in d.items():
if k in merged_dict:
merged_dict[k] = [merged_dict[k], v] if not isinstance(merged_dict[k], list) else merged_dict[k] + [v]
else:
merged_dict[k] = v
“`
By employing these methods and strategies, you can effectively merge dictionaries in Python, tailoring the approach to fit specific requirements based on your data and application needs.
Expert Insights on Merging Dictionaries in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Group). “Merging dictionaries in Python can be efficiently accomplished using the `update()` method or the `**` unpacking operator. The choice between these methods often depends on the specific requirements of the application, such as whether you need to preserve the original dictionaries or not.”
James Liu (Data Scientist, Tech Innovations Inc.). “In Python 3.9 and later, the `|` operator provides a very intuitive way to merge dictionaries. This not only enhances code readability but also simplifies the merging process, making it a preferred option for many developers in contemporary coding practices.”
Sarah Thompson (Python Educator, Code Academy). “When teaching how to merge dictionaries, I emphasize the importance of understanding the implications of key collisions. Using methods like `ChainMap` from the `collections` module can provide a more controlled approach to merging, especially in complex applications where data integrity is paramount.”
Frequently Asked Questions (FAQs)
How can I merge two dictionaries in Python?
You can merge two dictionaries in Python using the `update()` method, which updates the first dictionary with the key-value pairs from the second dictionary. Alternatively, you can use the `**` unpacking operator in Python 3.5 and later, or the `dict()` constructor.
What is the syntax for merging dictionaries using the `update()` method?
The syntax is `dict1.update(dict2)`, where `dict1` is the dictionary to be updated and `dict2` is the dictionary whose items will be added to `dict1`.
Can I merge dictionaries with overlapping keys?
Yes, when merging dictionaries with overlapping keys, the values from the second dictionary will overwrite those in the first dictionary.
Is there a way to merge dictionaries without modifying the original ones?
Yes, you can create a new dictionary using the `` unpacking operator like this: `merged_dict = {dict1, **dict2}`, which creates a new dictionary containing the merged items.
What is the method to merge dictionaries in Python 3.9 and later?
In Python 3.9 and later, you can use the `|` operator to merge dictionaries. The syntax is `merged_dict = dict1 | dict2`.
Are there any performance considerations when merging large dictionaries?
Yes, merging large dictionaries can be memory-intensive and may affect performance. It is advisable to consider the method used for merging and optimize for efficiency, especially in cases involving large datasets.
Merging dictionaries in Python is a common task that can be accomplished through various methods, each suited to different use cases. The most straightforward approach is using the `update()` method, which modifies the original dictionary by adding key-value pairs from another dictionary. However, this method does not create a new dictionary and can overwrite existing keys without warning.
Another efficient way to merge dictionaries is by using the unpacking operator (`**`). This method allows you to create a new dictionary that combines the contents of multiple dictionaries while preserving the original ones. Starting from Python 3.5, this technique has become increasingly popular due to its simplicity and readability. Furthermore, Python 3.9 introduced the `|` operator, which provides a more intuitive syntax for merging dictionaries, allowing for a clear and concise expression of intent.
When merging dictionaries, it is essential to consider how to handle duplicate keys. The last value encountered for a duplicate key will typically overwrite any previous values. Therefore, understanding the implications of the chosen merging method is crucial for maintaining data integrity. Additionally, developers should be aware of the performance implications of each method, especially when dealing with large datasets.
Python offers multiple methods for merging dictionaries, each with its
Author Profile
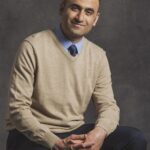
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?