How Can You Multiply Matrices in Python Effectively?
In the realm of data science, machine learning, and scientific computing, matrix operations stand as fundamental building blocks. Among these operations, matrix multiplication is particularly critical, serving as the backbone for various algorithms and computations. Whether you’re working with datasets, transforming images, or optimizing complex systems, understanding how to multiply matrices in Python can significantly enhance your programming toolkit. This article will guide you through the essentials of matrix multiplication, exploring the techniques and libraries that make these operations both efficient and intuitive.
Matrix multiplication is not just a mathematical concept; it’s a powerful operation that allows us to combine and manipulate data in meaningful ways. In Python, there are several methods to achieve this, ranging from basic loops to using specialized libraries like NumPy. Each approach has its own use cases and advantages, making it essential to understand the context in which you’re working. As we delve deeper, you’ll discover how to perform matrix multiplication effectively, whether you’re handling small matrices or large datasets.
Moreover, the flexibility and efficiency of Python make it an ideal language for matrix operations. With the right tools and techniques, you can perform complex calculations with ease, paving the way for advanced data analysis and machine learning tasks. As we explore the intricacies of matrix multiplication in Python, you’ll gain the confidence to tackle various
Matrix Multiplication Using NumPy
To perform matrix multiplication in Python, one of the most efficient and widely-used libraries is NumPy. This library provides a powerful array object and functions to perform mathematical operations easily. To multiply matrices using NumPy, follow these steps:
- Install NumPy: If you haven’t already installed NumPy, you can do so using pip:
“`bash
pip install numpy
“`
- Import NumPy: Start by importing the library in your Python script.
“`python
import numpy as np
“`
- Define Matrices: Create the matrices you want to multiply. In NumPy, you can define matrices using the `np.array()` function.
“`python
A = np.array([[1, 2], [3, 4]])
B = np.array([[5, 6], [7, 8]])
“`
- Multiply Matrices: Use the `np.dot()` function or the `@` operator for matrix multiplication.
“`python
C = np.dot(A, B)
or
C = A @ B
“`
- Output the Result: To view the resultant matrix, simply print it.
“`python
print(C)
“`
The resulting matrix `C` will be:
“`
[[19 22]
[43 50]]
“`
Matrix Multiplication Using Nested Loops
Although using NumPy is the recommended approach due to its efficiency, you can also multiply matrices using nested loops. This method is less efficient for large matrices but is useful for understanding the mechanics of matrix multiplication.
Here’s how you can do it:
- Define the Function: Create a function to multiply two matrices.
“`python
def multiply_matrices(A, B):
Get the dimensions of A and B
rows_A, cols_A = len(A), len(A[0])
rows_B, cols_B = len(B), len(B[0])
Initialize the result matrix with zeros
result = [[0 for _ in range(cols_B)] for _ in range(rows_A)]
Perform multiplication
for i in range(rows_A):
for j in range(cols_B):
for k in range(cols_A):
result[i][j] += A[i][k] * B[k][j]
return result
“`
- Define Matrices: Similar to the previous example, define the matrices you want to multiply.
“`python
A = [[1, 2], [3, 4]]
B = [[5, 6], [7, 8]]
“`
- Call the Function: Pass the matrices to the function and print the result.
“`python
C = multiply_matrices(A, B)
print(C)
“`
The output will be the same:
“`
[[19, 22], [43, 50]]
“`
Comparison of Methods
Using NumPy for matrix multiplication is highly recommended due to its optimized performance and simplicity. Below is a comparison table that highlights the differences between the two methods:
Aspect | NumPy Method | Nested Loops |
---|---|---|
Performance | High (optimized for large matrices) | Low (slower for large matrices) |
Code Complexity | Low (simple and concise) | High (requires more lines of code) |
Readability | High (easy to understand) | Medium (more complex logic) |
In summary, while both methods achieve the same result, utilizing NumPy is preferable for practical applications, especially when dealing with larger datasets or matrices.
Matrix Multiplication Basics
Matrix multiplication involves taking two matrices and producing a third matrix. For two matrices A and B to be multiplicative, the number of columns in A must equal the number of rows in B. The resultant matrix C will have the dimensions defined by the number of rows in A and the number of columns in B.
To perform matrix multiplication, consider the following:
- If A is an m x n matrix and B is an n x p matrix, then:
- C will be an m x p matrix.
- Each element of C, denoted as C[i][j], is computed as the dot product of the i-th row of A and the j-th column of B.
Using NumPy for Matrix Multiplication
The NumPy library in Python is a powerful tool for numerical computations, including matrix operations. It allows for efficient manipulation and multiplication of matrices. To multiply matrices using NumPy, follow these steps:
- Install NumPy, if not already installed:
“`bash
pip install numpy
“`
- Import the library:
“`python
import numpy as np
“`
- Define the matrices:
“`python
A = np.array([[1, 2], [3, 4]])
B = np.array([[5, 6], [7, 8]])
“`
- Perform the multiplication:
“`python
C = np.dot(A, B) or C = A @ B
“`
- Display the result:
“`python
print(C)
“`
Example of Matrix Multiplication
Consider the following example with matrices A and B:
A | B | |
---|---|---|
1 2 | 5 6 | |
3 4 | 7 8 |
The product C will be calculated as follows:
- C[0][0] = (1*5 + 2*7) = 19
- C[0][1] = (1*6 + 2*8) = 22
- C[1][0] = (3*5 + 4*7) = 43
- C[1][1] = (3*6 + 4*8) = 50
Thus, the resultant matrix C will be:
C |
---|
19 22 |
43 50 |
Using the `@` Operator for Multiplication
In addition to `np.dot()`, the `@` operator can be used for matrix multiplication, enhancing code readability. Here’s how to use it:
“`python
C = A @ B
print(C)
“`
This will yield the same result as using `np.dot()`, effectively simplifying the syntax.
Multiplying Higher-Dimensional Matrices
NumPy also supports multiplication of higher-dimensional arrays (tensors). When multiplying tensors, ensure that the dimensions align appropriately, following similar rules as 2D matrices.
Example for 3D tensors:
“`python
A = np.random.rand(2, 3, 4) 2x3x4 tensor
B = np.random.rand(2, 4, 5) 2x4x5 tensor
C = np.matmul(A, B) Result will be a 2x3x5 tensor
“`
Utilizing `np.matmul()` or the `@` operator allows for efficient computation across multiple dimensions, adhering to the same rules of alignment.
Conclusion on Best Practices
- Use NumPy for efficient and simplified matrix operations.
- Leverage the `@` operator for clearer syntax.
- Ensure matrices are properly aligned before multiplication to avoid runtime errors.
- Explore additional NumPy functions for more complex operations, such as `np.linalg` for linear algebra tasks.
Expert Insights on Multiplying Matrices in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Multiplying matrices in Python can be efficiently achieved using the NumPy library, which provides a straightforward interface for matrix operations. By utilizing the `numpy.dot()` function or the `@` operator, one can perform matrix multiplication with optimal performance and minimal code.”
Michael Chen (Software Engineer, AI Solutions Group). “For those new to Python, understanding the fundamental concepts of matrix multiplication is crucial. I recommend starting with basic lists and nested loops before transitioning to libraries like NumPy or TensorFlow, which abstract away the complexities and enhance computational efficiency.”
Dr. Sarah Patel (Mathematics Educator, Online Learning Hub). “While libraries such as NumPy are excellent for practical applications, I emphasize the importance of grasping the mathematical principles behind matrix multiplication. This understanding will not only aid in coding but also in applying these concepts to real-world problems effectively.”
Frequently Asked Questions (FAQs)
How do I multiply two matrices in Python?
You can multiply two matrices in Python using the NumPy library. First, import NumPy, then use the `numpy.dot()` function or the `@` operator to perform matrix multiplication.
What is the difference between element-wise multiplication and matrix multiplication?
Element-wise multiplication multiplies corresponding elements of two matrices, while matrix multiplication involves the dot product of rows and columns, adhering to specific mathematical rules.
Can I multiply matrices of different sizes in Python?
No, you cannot multiply matrices of incompatible sizes. The number of columns in the first matrix must equal the number of rows in the second matrix for matrix multiplication to be valid.
How do I handle matrix multiplication with NumPy?
To handle matrix multiplication with NumPy, ensure you have the library installed, create your matrices as NumPy arrays, and then use either the `numpy.dot()` function or the `@` operator for multiplication.
What should I do if I encounter a shape mismatch error while multiplying matrices?
A shape mismatch error indicates that the matrices do not conform to the multiplication rules. Verify the dimensions of both matrices and ensure that the number of columns in the first matrix matches the number of rows in the second matrix.
Are there any alternatives to NumPy for matrix multiplication in Python?
Yes, alternatives include using the `matrix` class from the `numpy` library, or libraries such as `pandas` for DataFrame operations, or even pure Python implementations using nested loops, though these are less efficient.
Multiplying matrices in Python can be efficiently performed using various libraries, with NumPy being the most popular choice due to its powerful array manipulation capabilities. To multiply two matrices, one can utilize the `numpy.dot()` function or the `@` operator, both of which are designed to handle matrix operations seamlessly. Additionally, it is crucial to ensure that the dimensions of the matrices are compatible for multiplication; specifically, the number of columns in the first matrix must equal the number of rows in the second matrix.
Another important aspect to consider when multiplying matrices in Python is the performance implications of using different methods. While NumPy provides optimized functions for matrix multiplication, using Python’s built-in lists for such operations can lead to significantly slower performance, especially with larger matrices. Therefore, leveraging libraries that are optimized for numerical computations is highly recommended for efficiency.
In summary, understanding how to multiply matrices in Python involves familiarizing oneself with the appropriate libraries, ensuring dimensional compatibility, and recognizing the performance benefits of using optimized functions. By following these guidelines, users can effectively perform matrix multiplication and leverage Python’s capabilities for mathematical computations.
Author Profile
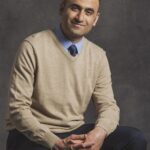
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?