How Can You Effectively Normalize Data in Python?
In the age of big data, the ability to glean insights from vast datasets has become a cornerstone of modern analytics. However, raw data often comes in various scales and formats, which can lead to misleading results if not handled properly. Enter data normalization—a crucial preprocessing step that ensures your datasets are on a comparable scale, allowing for more accurate analysis and modeling. If you’ve ever wondered how to normalize data in Python, you’re in the right place. This article will guide you through the essential techniques and libraries that make data normalization not just manageable, but efficient.
Data normalization is the process of adjusting values in a dataset to a common scale without distorting differences in the ranges of values. This technique is particularly important when working with machine learning algorithms, as many of them are sensitive to the scale of input data. By normalizing your data, you can improve the performance of your models, enhance convergence speed, and mitigate the risk of biased predictions. In Python, a variety of libraries, such as NumPy and scikit-learn, provide powerful tools to streamline this process.
As we delve deeper into the methods and best practices for normalizing data in Python, you’ll discover how to implement techniques like Min-Max scaling and Z-score normalization. Whether you’re a seasoned data scientist or just starting your journey
Understanding Data Normalization
Data normalization is a crucial preprocessing step in data analysis and machine learning. It involves adjusting the values in a dataset to a common scale without distorting differences in the ranges of values. This process is especially important for algorithms that are sensitive to the scale of input data, such as k-means clustering or gradient descent.
There are several normalization techniques, each with its own advantages and use cases:
- Min-Max Normalization: Scales the data to a fixed range, typically [0, 1].
- Z-Score Normalization (Standardization): Centers the data around the mean with a standard deviation of 1.
- Robust Scaler: Uses the median and the interquartile range for scaling, making it robust to outliers.
Min-Max Normalization in Python
Min-Max normalization can be implemented easily using libraries such as NumPy and pandas. Here’s an example of how to perform Min-Max normalization:
“`python
import pandas as pd
Sample data
data = {‘A’: [1, 2, 3, 4, 5],
‘B’: [10, 20, 30, 40, 50]}
df = pd.DataFrame(data)
Min-Max Normalization
df_normalized = (df – df.min()) / (df.max() – df.min())
print(df_normalized)
“`
This code snippet normalizes the columns ‘A’ and ‘B’ to the range [0, 1].
Z-Score Normalization in Python
Z-score normalization can be implemented using the `StandardScaler` from the `sklearn.preprocessing` module. Here’s how to do it:
“`python
from sklearn.preprocessing import StandardScaler
import pandas as pd
Sample data
data = {‘A’: [1, 2, 3, 4, 5],
‘B’: [10, 20, 30, 40, 50]}
df = pd.DataFrame(data)
Z-Score Normalization
scaler = StandardScaler()
df_normalized = scaler.fit_transform(df)
print(df_normalized)
“`
In this example, the data is transformed such that each feature will have a mean of 0 and a standard deviation of 1.
Robust Scaling in Python
For datasets that contain outliers, the Robust Scaler is more appropriate. It scales features using statistics that are robust to outliers. Here’s an example:
“`python
from sklearn.preprocessing import RobustScaler
import pandas as pd
Sample data
data = {‘A’: [1, 2, 3, 4, 100],
‘B’: [10, 20, 30, 40, 500]}
df = pd.DataFrame(data)
Robust Scaling
scaler = RobustScaler()
df_normalized = scaler.fit_transform(df)
print(df_normalized)
“`
This code will scale the dataset based on the median and interquartile range.
Comparison of Normalization Techniques
The choice of normalization technique can significantly affect the performance of machine learning models. Below is a comparison table of the different methods:
Normalization Technique | Scale | Robust to Outliers | Use Cases |
---|---|---|---|
Min-Max Normalization | [0, 1] | No | Neural Networks, k-NN |
Z-Score Normalization | Mean = 0, Std Dev = 1 | No | Algorithms assuming Gaussian distribution |
Robust Scaler | Centered around Median | Yes | Data with outliers |
Selecting the right normalization technique is essential based on the nature of the data and the specific requirements of the analysis or machine learning task.
Methods to Normalize Data in Python
Normalization is a critical preprocessing step in data analysis and machine learning, ensuring that features contribute equally to the distance calculations and model training processes. Python provides several libraries and methods to achieve this.
Using Scikit-Learn
The Scikit-Learn library offers built-in functions for normalization that are easy to implement. The two most common normalization techniques are Min-Max Scaling and Z-score Standardization.
Min-Max Scaling
Min-Max scaling transforms features to a fixed range, usually [0, 1]. This method is useful when the data is uniformly distributed.
“`python
from sklearn.preprocessing import MinMaxScaler
import numpy as np
data = np.array([[1, 2], [2, 3], [4, 5]])
scaler = MinMaxScaler()
normalized_data = scaler.fit_transform(data)
“`
Z-score Standardization
Z-score standardization rescales data to have a mean of 0 and a standard deviation of 1. This is particularly useful for normally distributed data.
“`python
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
standardized_data = scaler.fit_transform(data)
“`
Using Pandas
Pandas is another powerful library that facilitates data normalization through simple DataFrame operations.
Min-Max Scaling with Pandas
“`python
import pandas as pd
df = pd.DataFrame(data, columns=[‘Feature1’, ‘Feature2’])
normalized_df = (df – df.min()) / (df.max() – df.min())
“`
Z-score Standardization with Pandas
“`python
standardized_df = (df – df.mean()) / df.std()
“`
Custom Normalization Functions
For scenarios where built-in methods are insufficient, you can create custom normalization functions.
“`python
def min_max_scaling(x):
return (x – np.min(x)) / (np.max(x) – np.min(x))
def z_score_standardization(x):
return (x – np.mean(x)) / np.std(x)
data_scaled = min_max_scaling(data)
data_standardized = z_score_standardization(data)
“`
Comparison of Normalization Techniques
Technique | When to Use | Output Range |
---|---|---|
Min-Max Scaling | When features have different ranges and distributions | [0, 1] |
Z-score Standardization | When data is normally distributed | Mean = 0, Std = 1 |
Selecting the appropriate normalization technique depends on the dataset characteristics and the requirements of the machine learning algorithms. By leveraging libraries like Scikit-Learn and Pandas, normalization can be efficiently implemented in Python, enhancing model performance and accuracy.
Expert Insights on Normalizing Data in Python
Dr. Emily Chen (Data Scientist, Tech Innovations Inc.). “Normalizing data in Python is essential for ensuring that your machine learning models perform optimally. Techniques such as Min-Max scaling and Z-score normalization are widely used, and libraries like Scikit-learn offer built-in functions to streamline this process.”
Michael Thompson (Senior Data Analyst, Analytics Hub). “When normalizing data in Python, it is crucial to understand the context of your dataset. Different normalization methods can yield varying results, and selecting the appropriate one can significantly impact the accuracy of your analysis.”
Sarah Patel (Machine Learning Engineer, AI Solutions Group). “I recommend using the Pandas library for data manipulation before normalization. It allows for easy handling of missing values and outliers, which can distort your normalization efforts if not addressed properly.”
Frequently Asked Questions (FAQs)
What is data normalization?
Data normalization is the process of scaling individual data points to a common scale, typically to improve the performance of machine learning algorithms. It helps in reducing bias and ensuring that each feature contributes equally to the analysis.
Why is normalization important in data processing?
Normalization is crucial because it prevents features with larger ranges from dominating the model training process. It enhances the convergence speed of optimization algorithms and improves the overall accuracy of the model.
How can I normalize data in Python?
You can normalize data in Python using libraries like `scikit-learn`. The `MinMaxScaler` or `StandardScaler` can be employed to scale your data to a specified range or to standardize it to have a mean of zero and a standard deviation of one.
What are the common methods for normalizing data?
Common methods include Min-Max Scaling, which rescales data to a range of [0, 1], and Z-score Normalization, which standardizes data based on the mean and standard deviation. Each method serves different purposes depending on the dataset characteristics.
Can normalization be applied to categorical data?
Normalization is typically not applied to categorical data directly. However, categorical variables can be encoded using techniques such as one-hot encoding or label encoding before normalization of numerical features.
What libraries in Python are best for data normalization?
The most widely used libraries for data normalization in Python include `scikit-learn`, `pandas`, and `numpy`. These libraries provide various functions and methods to efficiently scale and preprocess your data.
Normalizing data in Python is a crucial step in data preprocessing, particularly in machine learning and statistical analysis. The primary objective of normalization is to adjust the values in the dataset to a common scale without distorting differences in the ranges of values. Common techniques for normalization include min-max scaling, z-score standardization, and robust scaling. Each method has its own advantages and is suited for different types of data distributions and modeling requirements.
Python offers several libraries that facilitate data normalization, with popular choices being NumPy, Pandas, and Scikit-learn. These libraries provide built-in functions that simplify the implementation of normalization techniques. For instance, Scikit-learn’s `MinMaxScaler` and `StandardScaler` are widely used for min-max scaling and z-score normalization, respectively. By leveraging these libraries, practitioners can efficiently preprocess their datasets, ensuring that the models they build are more robust and perform better.
understanding how to normalize data effectively is essential for anyone working with data in Python. By applying the appropriate normalization technique, one can enhance the quality of the data, leading to improved model accuracy and performance. It is important to consider the nature of the data and the specific requirements of the analysis when selecting a normalization method
Author Profile
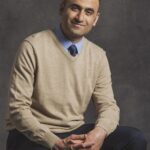
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?