How Can You Execute Specific Lines of Code in Python?
In the world of programming, efficiency and precision are paramount. As developers, we often find ourselves in situations where we need to test specific parts of our code without running the entire script. Whether you’re debugging, experimenting with new features, or simply trying to understand a complex function, knowing how to selectively execute certain lines in Python can save you time and enhance your workflow. This article will explore various techniques that allow you to run only the lines of code you need, empowering you to streamline your coding process and focus on what truly matters.
When working with Python, there are several methods to execute only specific lines of code. One common approach is to use interactive environments like Jupyter Notebooks or Python’s built-in REPL, which allow for line-by-line execution. This flexibility enables you to run snippets of code in isolation, making it easier to test and debug without the overhead of executing the entire program. Additionally, leveraging comments and conditional statements can help you control the flow of execution, allowing you to bypass sections of code that are not relevant at the moment.
Moreover, utilizing functions and modular programming can significantly enhance your ability to run specific lines. By encapsulating code within functions, you can call only the parts you need, reducing redundancy and improving readability. This modular approach
Using Functions to Execute Specific Lines
One effective way to run specific lines of code in Python is by encapsulating them within functions. By defining functions for different segments of your code, you can selectively execute only the lines contained within those functions. This approach not only organizes your code better but also enhances reusability.
For instance, consider the following example:
“`python
def task_one():
print(“Executing Task One”)
Additional lines of code for Task One
def task_two():
print(“Executing Task Two”)
Additional lines of code for Task Two
“`
To execute only `task_one`, you would call the function directly:
“`python
task_one()
“`
This runs only the lines defined within `task_one`, allowing for precise control over execution.
Commenting Out Lines
Another straightforward method to prevent certain lines from executing is by commenting them out. In Python, you can comment a line by placing a “ at the beginning. This is useful for temporarily disabling code without deleting it.
Example:
“`python
print(“This line will execute”)
print(“This line will not execute”)
“`
In this snippet, the second `print` statement will not run because it has been commented out.
Using Interactive Python Environments
In interactive environments like Jupyter Notebook or IPython, you can execute specific lines or blocks of code interactively. This is particularly useful for testing and debugging.
- Cell-based execution: In Jupyter, you can run entire cells independently. By splitting your code into different cells, you can execute only the parts you wish to test.
- Line execution: In IPython, you can execute single lines by simply typing them into the prompt.
Conditional Execution with If Statements
You can also control which lines run based on certain conditions using `if` statements. This allows for dynamic execution of code based on variables or user input.
Example:
“`python
run_task = True
if run_task:
print(“Task is running”)
else:
print(“Task is skipped”)
“`
In this scenario, the print statement will only execute if `run_task` is `True`.
Using a Loop for Selective Execution
You can also use loops to run specific lines based on conditions or iterations. This is particularly useful when dealing with collections of data.
Example:
“`python
tasks = [‘task_one’, ‘task_two’, ‘task_three’]
for task in tasks:
if task == ‘task_one’:
print(“Executing Task One”)
Task Two is skipped
Task Three is not executed
“`
This loop allows you to selectively execute code based on the task being processed.
Table of Execution Methods
Method | Description | Use Case |
---|---|---|
Functions | Encapsulate code in reusable units. | Organizing code and reusability. |
Commenting | Disable lines by commenting. | Temporarily skipping code. |
Interactive Environments | Run code in blocks or lines interactively. | Testing and debugging. |
If Statements | Conditionally execute lines. | Dynamic control based on conditions. |
Loops | Iterate through tasks with selective execution. | Handling collections of data. |
Using the Python Interpreter
Running specific lines of Python code can be efficiently accomplished using the Python interpreter. This allows for interactive execution of commands, making it ideal for testing snippets of code without running an entire script.
– **Launch the Python Interpreter**: You can start the interpreter by simply typing `python` or `python3` in your terminal.
– **Input Lines Directly**: Once in the interpreter, you can type in the lines of code you wish to execute one at a time. Each line can be executed immediately after inputting it.
Example:
“`python
>>> x = 10
>>> print(x)
10
“`
Using a Python Script with Conditional Execution
Another approach to run specific lines of a script involves using conditional statements or functions. This method is particularly useful when you want to control the execution flow based on certain conditions.
- Define Functions: By encapsulating code in functions, you can call only the lines you need.
Example:
“`python
def main():
print(“This is the main function.”)
def secondary_function():
print(“This is a secondary function.”)
Call only the function you want to execute
secondary_function()
“`
- Conditional Statements: You can use conditions to control which parts of the code run.
Example:
“`python
run_secondary = True
if run_secondary:
secondary_function()
“`
Using Jupyter Notebooks
Jupyter Notebooks provide a user-friendly interface for executing specific lines or blocks of code without running the entire file. This is particularly useful for data analysis and visualization tasks.
- Cell Execution: Each block of code is contained in a cell. You can run individual cells by selecting them and pressing `Shift + Enter`.
- Code Cells vs. Markdown Cells: Differentiate between code cells (where you write Python code) and markdown cells (for documentation). This structure enhances clarity and organization.
Utilizing Debugging Tools
Debugging tools such as `pdb` (Python Debugger) can be employed to execute specific lines within your code during a debugging session.
- Set Breakpoints: You can set breakpoints in your code where you want execution to pause. This allows you to inspect the current state and run subsequent lines interactively.
Example:
“`python
import pdb
def test_function():
x = 5
pdb.set_trace() Execution will pause here
y = x + 10
print(y)
test_function()
“`
- Step Through Code: Use commands like `n` (next) to run the next line, and `c` (continue) to resume execution until the next breakpoint.
Leveraging Integrated Development Environments (IDEs)
Many IDEs, such as PyCharm, Visual Studio Code, and Spyder, have built-in features that facilitate the execution of specific lines of code.
- Run Selection: Highlight the lines you want to run and use a shortcut (commonly `Shift + Enter`) to execute only the selected lines.
- Debugging Features: Use the debugging tools within the IDE to set breakpoints and step through code execution.
IDE | Shortcut for Run Selection |
---|---|
PyCharm | Shift + Enter |
Visual Studio Code | Shift + Enter |
Spyder | F9 |
Using the `exec()` Function
The `exec()` function allows for dynamic execution of Python code contained within strings. This provides flexibility to run specific lines or blocks of code programmatically.
Example:
“`python
code = “””
x = 10
print(x)
“””
exec(code) Executes the code in the string
“`
This method is generally less common for routine tasks but can be powerful in certain dynamic contexts where code needs to be generated or modified at runtime.
Strategies for Selectively Executing Python Code
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “To run specific lines of code in Python, you can utilize functions to encapsulate the lines you want to execute. By calling the function, you can control which parts of your code run without executing the entire script.”
James Liu (Lead Software Engineer, CodeCraft Solutions). “Using an interactive Python shell or Jupyter Notebook allows you to execute individual lines or blocks of code easily. This is particularly useful for testing and debugging specific segments of your code without running the whole program.”
Sarah Thompson (Python Educator, LearnPython Academy). “Another effective method is to use conditional statements or comments to control the execution flow. By commenting out lines or wrapping them in conditions, you can selectively execute the desired parts of your script.”
Frequently Asked Questions (FAQs)
How can I run specific lines of code in a Python script?
You can run specific lines of code in a Python script by using an interactive environment like Jupyter Notebook or IPython, where you can execute individual cells or lines. Alternatively, you can comment out the lines you do not want to execute in a standard Python script.
Is there a way to execute specific lines using a Python IDE?
Yes, most Python IDEs, such as PyCharm and Visual Studio Code, allow you to select specific lines of code and run them directly. Look for options like “Run Selection” or similar commands in the context menu.
Can I use the Python debugger to run certain lines?
Yes, you can use the Python debugger (pdb) to step through your code line by line. By setting breakpoints and using commands like ‘n’ (next) or ‘c’ (continue), you can control the execution flow and run specific lines.
What is the purpose of using the `exec()` function in Python?
The `exec()` function allows you to execute Python code dynamically, including specific lines or blocks of code stored as strings. This can be useful for running code generated at runtime.
Are there any command-line tools to run specific lines in Python scripts?
Yes, you can use command-line tools like `python -c` to execute specific lines of code directly from the command line. However, this is best suited for small snippets rather than entire scripts.
How can I isolate and test specific lines in a Python script?
You can isolate and test specific lines by creating a separate test script or using a testing framework like pytest. This allows you to run only the desired portions of your code in a controlled environment.
In Python, executing only certain lines of code can be achieved through various methods, depending on the context and the desired outcome. One common approach is to utilize functions, which allow you to encapsulate specific lines of code and call them as needed. This modularity not only enhances code organization but also promotes reusability, making it easier to run specific segments without executing the entire script.
Another effective method is the use of conditional statements. By incorporating `if` statements, you can control the flow of execution based on certain conditions, thereby allowing only the desired lines to run when specific criteria are met. This technique is particularly useful for debugging or when you want to isolate parts of your code for testing purposes.
Additionally, interactive environments such as Jupyter Notebooks provide a user-friendly way to execute individual cells of code. This feature allows developers to run specific lines or blocks of code independently, facilitating a more iterative and exploratory coding experience. Leveraging these tools can significantly streamline the development process and enhance productivity.
In summary, Python offers multiple strategies for executing only certain lines of code, including the use of functions, conditional statements, and interactive environments. Understanding and utilizing these techniques can lead to more efficient coding practices and improved
Author Profile
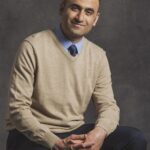
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?