How Can You Easily Open a TXT File in Python?
In the world of programming, the ability to manipulate files is a fundamental skill that every developer should master. Among the various file types, text files (.txt) are the simplest yet most versatile, serving as a common medium for data storage and information exchange. Whether you’re a beginner embarking on your coding journey or a seasoned programmer looking to refresh your skills, understanding how to open and interact with text files in Python is essential. This article will guide you through the process, equipping you with the knowledge to read, write, and manage text files effectively.
When it comes to opening a text file in Python, the language provides a straightforward and intuitive approach. Python’s built-in functions allow you to access file content with ease, making it an ideal choice for tasks ranging from simple data logging to complex data analysis. With just a few lines of code, you can unlock the information stored within a text file, enabling you to read its contents, modify them, or even create new files from scratch.
As we delve deeper into the topic, we will explore various methods for opening text files, discussing best practices and common pitfalls to avoid. Whether you need to handle large datasets or simply want to read a configuration file, understanding the nuances of file handling in Python will empower
Opening a Text File
To open a text file in Python, you can utilize the built-in `open()` function. This function allows you to specify the file’s path and the mode in which you wish to open it. The most common modes include:
- `’r’`: Read (default mode) – Opens a file for reading.
- `’w’`: Write – Opens a file for writing, truncating the file first if it exists.
- `’a’`: Append – Opens a file for appending new content.
- `’b’`: Binary – Opens a file in binary mode, which is useful for non-text files.
- `’x’`: Exclusive creation – Fails if the file already exists.
Here is a basic example of how to open a text file for reading:
“`python
file = open(‘example.txt’, ‘r’)
content = file.read()
file.close()
print(content)
“`
This code snippet opens `example.txt`, reads its content, and then closes the file to free up system resources.
Using the `with` Statement
A more efficient way to handle files in Python is by using the `with` statement. This method automatically manages file closing, even if an error occurs. Here’s how you can open a text file using the `with` statement:
“`python
with open(‘example.txt’, ‘r’) as file:
content = file.read()
print(content)
“`
This approach is recommended as it ensures that the file is properly closed after its suite finishes, enhancing code readability and reliability.
Reading Lines from a File
In addition to reading the entire content of a file, you can read it line by line. This can be done using the `readline()` method or by iterating through the file object directly. Here’s an example using both methods:
“`python
Using readline()
with open(‘example.txt’, ‘r’) as file:
line = file.readline()
while line:
print(line.strip()) Print each line without leading/trailing whitespace
line = file.readline()
Using for loop
with open(‘example.txt’, ‘r’) as file:
for line in file:
print(line.strip())
“`
Common File Operations
When working with text files, you may need to perform various operations. Below is a summary of common actions along with their respective code snippets.
Operation | Code Snippet |
---|---|
Writing to a file |
|
Appending to a file |
|
Reading all lines |
|
Utilizing these methods effectively allows you to handle text files in Python seamlessly, ensuring robust and efficient file operations.
Opening a Text File in Python
To open a text file in Python, the built-in `open()` function is utilized. This function is versatile and allows for various modes of file handling, which include reading, writing, and appending. Below are the steps and considerations for effectively opening a text file.
Using the `open()` Function
The syntax for the `open()` function is as follows:
“`python
file_object = open(file_name, mode)
“`
- file_name: The name of the file you wish to open, including its path if it is not in the current working directory.
- mode: A string that specifies the mode in which the file is opened. Common modes include:
- `’r’`: Read mode (default). Opens a file for reading.
- `’w’`: Write mode. Opens a file for writing (overwrites the existing file).
- `’a’`: Append mode. Opens a file for appending data.
- `’b’`: Binary mode. Used for non-text files.
- `’t’`: Text mode (default). Opens a file for text data.
Example of Opening a Text File
Here is a simple example demonstrating how to open a text file for reading:
“`python
Open a text file for reading
file = open(‘example.txt’, ‘r’)
Read the contents of the file
content = file.read()
Print the contents
print(content)
Close the file
file.close()
“`
In this example:
- The file `example.txt` is opened in read mode.
- The entire content of the file is read and printed.
- It is important to close the file after the operations are completed to free up system resources.
Using Context Managers
Using context managers is a preferred method for file operations in Python. The `with` statement automatically handles file closing, even if an error occurs. Here’s how to use it:
“`python
with open(‘example.txt’, ‘r’) as file:
content = file.read()
print(content)
“`
In this approach:
- The file is opened and automatically closed at the end of the `with` block.
- This method reduces the risk of leaving files open, which can lead to memory leaks or file corruption.
Reading Different Parts of the File
Python provides several methods to read the contents of a file:
- `read(size)`: Reads up to `size` bytes from the file. If `size` is omitted, it reads until the end of the file.
- `readline()`: Reads the next line from the file. Each call reads one line.
- `readlines()`: Reads all lines into a list, where each line is an element of the list.
Example of reading lines:
“`python
with open(‘example.txt’, ‘r’) as file:
lines = file.readlines()
for line in lines:
print(line.strip()) strip() removes leading/trailing whitespace
“`
Error Handling
When opening files, it is prudent to implement error handling to manage potential issues, such as missing files or permission errors. This can be done using a try-except block:
“`python
try:
with open(‘example.txt’, ‘r’) as file:
content = file.read()
except FileNotFoundError:
print(“The file was not found.”)
except PermissionError:
print(“You do not have permission to access this file.”)
“`
This structure ensures that your program can handle errors gracefully, providing informative feedback without crashing.
Expert Insights on Opening TXT Files in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Opening a TXT file in Python is straightforward, primarily utilizing the built-in `open()` function. This function allows for various modes such as reading, writing, and appending, making it versatile for different use cases.”
Mark Thompson (Data Scientist, Analytics Group). “When working with large TXT files, it’s essential to consider memory efficiency. Using the `with` statement in Python not only simplifies file handling but also ensures that files are properly closed after their contents are processed.”
Linda Nguyen (Python Developer, CodeCraft Solutions). “For those new to Python, I recommend starting with the `read()` method for smaller files, while `readline()` or `readlines()` can be more effective for larger datasets, allowing for line-by-line processing.”
Frequently Asked Questions (FAQs)
How do I open a .txt file in Python?
To open a .txt file in Python, use the built-in `open()` function. For example, `file = open(‘filename.txt’, ‘r’)` opens the file in read mode.
What modes can I use when opening a text file in Python?
You can use several modes such as `’r’` for reading, `’w’` for writing (which overwrites the file), `’a’` for appending, and `’r+’` for both reading and writing.
How do I read the contents of a text file once it is opened?
You can read the contents using methods like `file.read()`, `file.readline()`, or `file.readlines()`, depending on whether you want to read all at once, line by line, or as a list of lines.
What should I do after I finish working with a text file?
Always close the file using `file.close()` to free up system resources. Alternatively, you can use a `with` statement to automatically close the file after the block of code is executed.
Can I open a text file in a specific encoding in Python?
Yes, you can specify the encoding by adding the `encoding` parameter in the `open()` function, such as `open(‘filename.txt’, ‘r’, encoding=’utf-8′)`.
What happens if the file I try to open does not exist?
If the file does not exist and you attempt to open it in read mode (`’r’`), Python raises a `FileNotFoundError`. To avoid this, check if the file exists before attempting to open it.
Opening a text file in Python is a straightforward process that can be accomplished using the built-in `open()` function. This function allows users to specify the file path and the mode in which they want to open the file, such as read (‘r’), write (‘w’), or append (‘a’). By default, the `open()` function opens files in read mode, making it ideal for accessing and processing text data.
Once the file is opened, it is essential to handle the file object properly to avoid resource leaks. This can be achieved using a context manager, which automatically closes the file after its block of code has been executed. The `with` statement is commonly employed for this purpose, ensuring that the file is closed even if an error occurs during file operations. This practice enhances code reliability and maintainability.
Additionally, reading the content of a text file can be accomplished using various methods, such as `read()`, `readline()`, or `readlines()`. Each method serves a different purpose, allowing users to read the entire file at once, read it line by line, or retrieve all lines as a list, respectively. Understanding these methods enables developers to choose the most efficient approach based on their specific needs.
Author Profile
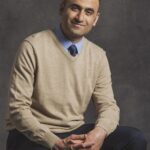
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?