How Can You Effectively Organize Your Python Project for Success?
In the ever-evolving world of software development, the importance of a well-organized project cannot be overstated. For Python developers, effective project organization is not just a matter of aesthetics; it’s a crucial factor that can significantly enhance productivity, maintainability, and collaboration. Whether you are embarking on a solo venture or collaborating with a team, establishing a clear structure from the outset can save you countless hours of debugging and confusion down the line. In this article, we will explore the essential principles and best practices for organizing your Python projects, ensuring that your code is not only functional but also easy to navigate and understand.
When it comes to organizing a Python project, the first step is to establish a logical directory structure. This involves categorizing your files in a way that reflects their purpose and functionality, making it easier for you and others to locate specific components. From separating your source code from tests and documentation to implementing version control, a thoughtful approach to organization lays the groundwork for a successful project. Additionally, adhering to established conventions, such as those outlined in PEP 8, can further enhance the clarity and consistency of your codebase.
Moreover, effective project organization extends beyond mere file structure. It encompasses the use of virtual environments, dependency management, and documentation practices
Directory Structure
A well-organized directory structure is fundamental for maintaining clarity in a Python project. The standard practice is to adopt a hierarchical structure that separates different components of the project, facilitating easier navigation and management.
Here is a typical directory structure for a Python project:
“`
project_name/
│
├── src/ Source code
│ ├── module1/ Submodule 1
│ ├── module2/ Submodule 2
│ └── __init__.py Makes src a package
│
├── tests/ Test cases
│ ├── test_module1.py
│ └── test_module2.py
│
├── docs/ Documentation
│ └── index.md
│
├── requirements.txt Dependencies
├── setup.py Installation script
└── README.md Project overview
“`
In this structure, the `src` directory contains the main application code, while `tests` holds the test cases to validate the functionality of the modules. The `docs` folder is reserved for documentation, ensuring that all relevant information is easily accessible.
Use of Virtual Environments
Utilizing virtual environments is essential for isolating project dependencies, ensuring that each project can maintain its own package versions without interference from other projects. This can prevent conflicts and make project management more straightforward.
To create a virtual environment, use the following commands:
“`bash
Create a virtual environment
python -m venv env
Activate the virtual environment
On Windows
.\env\Scripts\activate
On macOS/Linux
source env/bin/activate
“`
Dependency Management
Managing project dependencies is critical for ensuring that all necessary packages are installed and compatible with the project code. The most common way to handle dependencies in Python is through a `requirements.txt` file.
To create a `requirements.txt` file, you can use the following command while your virtual environment is activated:
“`bash
pip freeze > requirements.txt
“`
This command generates a list of all installed packages and their versions, allowing for easy installation in different environments using:
“`bash
pip install -r requirements.txt
“`
Code Documentation
Proper documentation enhances code readability and maintainability. It is advisable to use docstrings in Python to document modules, classes, and functions. This practice helps other developers understand the code’s purpose and usage.
Example of a function with a docstring:
“`python
def add(a, b):
“””
Add two numbers and return the result.
Parameters:
a (int): The first number.
b (int): The second number.
Returns:
int: The sum of a and b.
“””
return a + b
“`
Version Control
Implementing version control systems, such as Git, is vital for tracking changes and collaborating with others. A `.gitignore` file should be included to specify files and directories that should not be tracked, such as:
- Compiled code (e.g., `*.pyc`)
- Virtual environment directories (e.g., `env/`)
- IDE configuration files (e.g., `.vscode/`)
Here’s an example of a `.gitignore` file:
“`
env/
__pycache__/
*.pyc
*.pyo
.idea/
.vscode/
“`
Testing Strategy
Establishing a robust testing strategy is crucial for maintaining code quality. The following types of tests should be considered:
- Unit Tests: Validate individual components in isolation.
- Integration Tests: Ensure that different components work together as expected.
- End-to-End Tests: Test the entire application flow from start to finish.
A well-structured testing framework, like `pytest` or `unittest`, can streamline the testing process. The tests should be organized in the `tests` directory, mirroring the structure of the `src` directory for consistency.
Type of Test | Description |
---|---|
Unit Test | Tests individual functions or methods. |
Integration Test | Tests combined parts of an application. |
End-to-End Test | Tests the complete application workflow. |
Project Structure
A well-organized project structure enhances readability and maintainability. A typical Python project structure may look like this:
“`
project_name/
│
├── README.md
├── setup.py
├── requirements.txt
├── src/
│ ├── __init__.py
│ ├── module1.py
│ └── module2.py
│
├── tests/
│ ├── __init__.py
│ ├── test_module1.py
│ └── test_module2.py
│
└── docs/
└── index.rst
“`
- README.md: Contains project description, installation instructions, usage examples, and contribution guidelines.
- setup.py: Defines the package metadata and dependencies for distribution.
- requirements.txt: Lists external libraries needed for the project, useful for setting up virtual environments.
- src/: Main source code directory, containing the application code organized in modules.
- tests/: Contains unit tests, ensuring the code behaves as expected.
- docs/: Documentation files, providing an overview of the project, usage, and developer guides.
Environment Management
Using virtual environments is essential for dependency management. Tools like `venv` or `conda` help create isolated environments, preventing version conflicts.
– **Creating a virtual environment**:
- Using `venv`:
“`bash
python -m venv venv
source venv/bin/activate On Unix or MacOS
venv\Scripts\activate On Windows
“`
- Using `conda`:
“`bash
conda create –name env_name python=3.9
conda activate env_name
“`
– **Managing dependencies**:
- Install required libraries with pip:
“`bash
pip install library_name
“`
- Freeze current environment packages:
“`bash
pip freeze > requirements.txt
“`
Code Organization
Organizing code into modules and packages promotes reusability and clarity. Follow these guidelines:
- Use descriptive names: Name modules and functions clearly to reflect their purpose.
- Group related functions: Keep similar functionalities within the same module.
- Limit module size: Aim to keep modules under 200–300 lines of code for better manageability.
- Separate concerns: Use different modules for different features or components of the application.
Version Control
Implementing version control is crucial for collaborative development and tracking changes. Use Git for managing project versions:
- Initialize a Git repository:
“`bash
git init
“`
- Create a .gitignore file: Exclude files and directories that should not be tracked, such as:
- `venv/`
- `__pycache__/`
- `.DS_Store`
- Regular commits: Make small, frequent commits with clear messages to document progress.
Documentation Practices
Well-documented code and project resources improve usability and facilitate onboarding new contributors.
- Docstrings: Use docstrings for modules, classes, and functions to describe their purpose and usage.
- Sphinx for documentation: Consider using Sphinx to generate HTML documentation from reStructuredText files.
- Update documentation: Ensure documentation is kept up-to-date with code changes, reflecting the latest features and usage.
Testing Strategy
A robust testing strategy ensures code reliability and helps identify issues early.
- Unit tests: Write unit tests for individual functions using frameworks like `unittest` or `pytest`.
- Integration tests: Test how different modules work together.
- Continuous Integration (CI): Implement CI tools like GitHub Actions or Travis CI to automate testing on code changes.
This structured approach to organizing a Python project lays a solid foundation for efficient development, collaboration, and maintenance.
Expert Insights on Organizing Python Projects
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “A well-structured Python project should follow a clear directory hierarchy, separating source code, tests, and documentation. Utilizing a standard layout, such as the one suggested by the Python Packaging Authority, can significantly enhance maintainability and collaboration.”
Michael Chen (Lead Developer, CodeCraft Solutions). “In my experience, adopting a modular approach is crucial when organizing a Python project. Each module should encapsulate specific functionality, allowing for easier testing and reuse. Additionally, leveraging virtual environments can help manage dependencies effectively.”
Sarah Johnson (Technical Consultant, Agile Dev Strategies). “Documentation is often overlooked, yet it is essential for any Python project. I recommend including a README file at the root of your project, outlining the purpose, setup instructions, and usage examples. This practice not only aids new developers but also enhances the overall project clarity.”
Frequently Asked Questions (FAQs)
What are the best practices for structuring a Python project?
A well-structured Python project typically includes a clear directory layout, such as separating source code, tests, and documentation. Common directories include `src/`, `tests/`, and `docs/`, along with a root-level `README.md` and `requirements.txt` for dependencies.
How should I name my Python files and directories?
Use lowercase letters and underscores for file and directory names. This convention enhances readability and maintains consistency across the project. For example, use `my_module.py` instead of `MyModule.py`.
What is the purpose of a virtual environment in a Python project?
A virtual environment isolates project dependencies, ensuring that packages required for one project do not interfere with others. This practice helps maintain compatibility and prevents version conflicts.
How can I manage dependencies in my Python project?
Utilize a `requirements.txt` file or `Pipfile` to specify project dependencies. This allows for easy installation and management of packages using pip, ensuring that all team members have the same environment setup.
What role does documentation play in organizing a Python project?
Documentation is crucial for understanding the project’s purpose, structure, and usage. Including a `README.md`, code comments, and detailed API documentation helps both current and future developers navigate and maintain the project effectively.
How can I ensure code quality in my organized Python project?
Implement code quality tools such as linters (e.g., flake8) and formatters (e.g., black) to enforce coding standards. Additionally, writing unit tests and integrating continuous integration (CI) practices will help maintain high code quality throughout the project lifecycle.
Organizing a Python project is essential for maintaining clarity, scalability, and efficiency throughout the development process. A well-structured project allows developers to navigate the codebase easily, facilitates collaboration among team members, and enhances code readability. Key elements of a well-organized Python project include a clear directory structure, the use of virtual environments, and adherence to coding conventions such as PEP 8. Additionally, incorporating documentation and tests into the project structure can significantly improve the overall quality and maintainability of the code.
One of the primary takeaways from the discussion on organizing Python projects is the importance of a logical directory structure. This typically involves separating the source code, tests, and documentation into distinct folders. By following a conventional layout, such as placing the main application code in a ‘src’ or ‘app’ directory and tests in a ‘tests’ directory, developers can ensure that the project remains manageable as it grows. Furthermore, using a virtual environment is crucial for isolating dependencies and maintaining a clean workspace, which prevents conflicts between different projects.
Another critical insight is the value of documentation and testing in project organization. Well-documented code not only aids in understanding the functionality but also serves as a guide for future developers. Implementing a
Author Profile
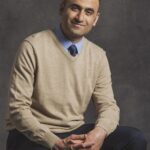
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?