How Can You Easily Host Python Code on a Server?
In today’s digital landscape, deploying Python applications to a server is an essential skill for developers and tech enthusiasts alike. Whether you’re building a web application, automating tasks, or creating data-driven solutions, knowing how to host your Python code effectively can make all the difference. With the rise of cloud computing and various hosting services, the process has become more accessible than ever, yet it still presents unique challenges and considerations that every programmer should be aware of.
Hosting Python code on a server involves several key steps, including selecting the right hosting environment, setting up the server, and deploying your application. Each of these steps requires an understanding of both the technical aspects and the tools available to streamline the process. From choosing between traditional web hosting and cloud platforms to configuring your server for optimal performance, there’s a wealth of information to navigate. Additionally, ensuring that your application is secure and scalable is paramount, especially as user demand grows.
As you delve deeper into this topic, you’ll discover various methods and best practices for deploying your Python code, including using frameworks like Flask or Django, containerization with Docker, and leveraging platforms such as Heroku or AWS. By mastering these techniques, you can transform your local projects into fully functional applications accessible to users around the globe. Get ready to unlock the potential
Choosing the Right Deployment Method
When considering how to host Python code on a server, it’s vital to select the appropriate deployment method based on your application type, scalability requirements, and available resources. Here are some common methods:
- Virtual Private Server (VPS): A VPS offers a dedicated environment on a physical server. It provides more control and is suitable for applications needing specific configurations.
- Platform as a Service (PaaS): Services like Heroku, Google App Engine, or AWS Elastic Beanstalk allow you to deploy applications without managing the underlying infrastructure.
- Containerization: Using Docker allows you to package your application and its dependencies into containers, ensuring consistency across different environments.
Setting Up Your Server Environment
After choosing a deployment method, the next step is setting up the server environment. This includes installing necessary software and dependencies that your Python application requires. Below is a checklist to guide you through the setup process:
- Operating System: Choose an OS that supports your application (e.g., Ubuntu, CentOS).
- Python Version: Ensure the Python version matches your development environment.
- Web Server: Install a web server like Nginx or Apache to serve your application.
- Database: Set up a database system, such as PostgreSQL or MySQL, if your application requires it.
Component | Installation Command |
---|---|
Python 3 | sudo apt-get install python3 |
Pip (Python package manager) | sudo apt-get install python3-pip |
Nginx | sudo apt-get install nginx |
PostgreSQL | sudo apt-get install postgresql |
Deploying Your Code
Once your environment is configured, you can proceed to deploy your Python code. This process typically involves several steps:
- Transfer Files: Use tools like `scp`, `rsync`, or FTP to transfer your Python files to the server.
- Set Up Virtual Environment: Create a virtual environment to manage dependencies for your application.
bash
python3 -m venv myenv
source myenv/bin/activate
- Install Dependencies: Use `pip` to install the required packages from your `requirements.txt` file.
bash
pip install -r requirements.txt
- Configure Web Server: Adjust the configuration of your web server (Nginx/Apache) to direct traffic to your Python application, often using a WSGI server like Gunicorn or uWSGI.
Testing and Monitoring Your Application
After deployment, it’s crucial to test your application thoroughly to ensure it operates as expected. Conduct tests for various scenarios, including load testing to assess performance under heavy traffic.
For ongoing management, consider the following monitoring tools:
- Prometheus: For metrics collection and monitoring.
- Grafana: For visualizing application performance metrics.
- Sentry: For error tracking and monitoring.
Establishing a continuous integration/continuous deployment (CI/CD) pipeline can streamline future updates and ensure that your code is always up to date and functioning correctly.
Deploying Python Code to a Server
Deploying Python applications involves several steps to ensure that the code runs effectively on a server environment. Below are key methods and considerations for deploying Python code.
Choosing the Right Server
The first step in deployment is selecting the appropriate server. The options typically include:
- Virtual Private Servers (VPS): Offers flexibility and control over the environment.
- Cloud Services: Providers like AWS, Google Cloud, and Azure offer scalable solutions.
- Platform as a Service (PaaS): Services like Heroku and PythonAnywhere simplify deployment.
Preparing Your Code for Deployment
Before deploying your Python application, ensure that it is production-ready:
– **Environment Configuration**: Use environment variables to manage sensitive data like API keys.
– **Dependencies**: List all dependencies in a `requirements.txt` file. Use the command:
pip freeze > requirements.txt
- Configuration Files: Create a configuration file for different environments (development, testing, production).
Deployment Steps
The deployment process can vary based on the chosen server type but generally follows these steps:
- Access the Server:
- Use SSH to connect to your server:
ssh username@server_ip_address
- Transfer Files:
- Use `scp` or `rsync` to copy files to the server:
scp -r /path/to/local/folder username@server_ip_address:/path/to/remote/folder
- Alternatively, use Git to clone the repository directly on the server.
- Set Up a Virtual Environment:
- Create a virtual environment to manage dependencies:
python3 -m venv myenv
source myenv/bin/activate
- Install Dependencies:
- Install the required packages:
pip install -r requirements.txt
- Run the Application:
- Depending on the application type (web, script, etc.), use the appropriate command. For a Flask application:
export FLASK_APP=app.py
flask run –host=0.0.0.0
Using a Web Server Gateway Interface (WSGI)
For web applications, a WSGI server is essential. Popular choices include:
- Gunicorn: A Python WSGI HTTP server.
- uWSGI: Another versatile option for deploying Python applications.
Example Configuration with Gunicorn:
gunicorn -w 4 -b 0.0.0.0:8000 app:app
- `-w 4` specifies the number of worker processes.
- `-b` binds the server to the given address.
Setting Up a Reverse Proxy
To improve performance and security, set up a reverse proxy using Nginx or Apache.
Basic Nginx Configuration:
nginx
server {
listen 80;
server_name your_domain.com;
location / {
proxy_pass http://127.0.0.1:8000;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
}
}
Monitoring and Logging
Implement monitoring and logging to ensure application health and performance:
- Logging: Use Python’s `logging` module for application logs.
- Monitoring Tools: Consider tools like Prometheus, Grafana, or New Relic to monitor application metrics.
Security Considerations
Finally, ensure security best practices are followed:
- Firewall: Configure firewall rules to restrict access.
- SSL/TLS: Use Let’s Encrypt for free SSL certificates to secure connections.
- Regular Updates: Keep the server and dependencies updated to mitigate vulnerabilities.
Expert Insights on Deploying Python Code to Servers
Dr. Emily Chen (Senior Software Engineer, Cloud Solutions Inc.). “When deploying Python code to a server, it is crucial to utilize a robust deployment framework such as Docker or Kubernetes. These tools not only streamline the deployment process but also enhance scalability and maintainability of your applications.”
Michael Torres (DevOps Specialist, Tech Innovations Group). “To effectively post Python code to a server, one should prioritize setting up a continuous integration/continuous deployment (CI/CD) pipeline. This approach automates testing and deployment, ensuring that your code is consistently delivered to the server with minimal errors.”
Lisa Patel (Lead Python Developer, CodeCraft Solutions). “Understanding the server environment is essential before posting Python code. Ensure that the server has the correct Python version and necessary dependencies installed. Utilizing virtual environments can help manage these dependencies effectively and avoid conflicts.”
Frequently Asked Questions (FAQs)
How do I upload Python code to a server?
You can upload Python code to a server using various methods, such as Secure Copy Protocol (SCP), File Transfer Protocol (FTP), or using a version control system like Git. Choose the method that best suits your server configuration and access permissions.
What tools can I use to transfer Python files to a server?
Common tools for transferring Python files include WinSCP, FileZilla for FTP/SFTP, and command-line tools like SCP or Rsync. Additionally, you can use Git to clone repositories directly onto the server.
Can I run Python code directly on the server after uploading?
Yes, once you upload your Python code to the server, you can execute it using the command line, provided that Python is installed on the server and you have the necessary permissions to run scripts.
What are the security considerations when uploading Python code to a server?
Ensure that you use secure transfer protocols like SFTP or SCP to protect your code during transmission. Additionally, validate the server’s security settings and permissions to prevent unauthorized access to your files.
How do I set up a virtual environment on the server for my Python code?
To set up a virtual environment, SSH into your server, navigate to your project directory, and run `python3 -m venv env_name`. Activate it using `source env_name/bin/activate` to manage dependencies without affecting the system Python installation.
What should I do if my Python code requires specific libraries on the server?
You should create a `requirements.txt` file listing all required libraries and their versions. After uploading this file to the server, you can install the libraries using `pip install -r requirements.txt` within your virtual environment.
In summary, deploying Python code to a server involves several essential steps that ensure the application runs smoothly in a production environment. Initially, it is crucial to choose the right server, which could be a cloud-based solution like AWS, Google Cloud, or a traditional VPS. After selecting a server, the next step is to set up the environment, which includes installing the necessary software, libraries, and dependencies required by the Python application.
Furthermore, it is important to configure the server for security and performance. This may involve setting up firewalls, managing user permissions, and optimizing the server settings to handle the expected load. Additionally, utilizing version control systems such as Git can streamline the deployment process and facilitate collaboration among developers. Finally, continuous integration and continuous deployment (CI/CD) practices can automate the deployment process, ensuring that updates are efficiently rolled out with minimal downtime.
successfully posting Python code to a server requires careful planning and execution. By selecting the appropriate server, setting up a secure and optimized environment, and employing best practices in version control and deployment, developers can ensure that their applications are robust and reliable. These considerations not only enhance the performance of the application but also contribute to a better user experience.
Author Profile
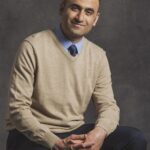
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?