How Can You Effectively Parse XML in Python?
In an era where data is the lifeblood of innovation and decision-making, the ability to efficiently parse and manipulate XML files is a crucial skill for developers and data analysts alike. XML, or eXtensible Markup Language, serves as a versatile format for data interchange, powering everything from web services to configuration files. However, diving into the intricacies of XML can feel daunting, especially for those new to programming or data handling. Fear not! This article will illuminate the path to mastering XML parsing in Python, a language renowned for its simplicity and robust libraries.
Parsing XML in Python opens up a world of possibilities for extracting, transforming, and utilizing data. With a variety of libraries at your disposal, including the built-in `xml.etree.ElementTree` and the more powerful `lxml`, Python provides the tools you need to navigate and manipulate XML structures with ease. Understanding how to leverage these libraries will not only streamline your workflow but also enhance your ability to integrate diverse data sources into your projects.
As we delve deeper into the nuances of XML parsing, you’ll discover practical techniques for reading XML files, accessing specific elements, and handling attributes and namespaces. Whether you’re looking to process configuration files, scrape web data, or interact with APIs, mastering XML parsing
Using ElementTree for XML Parsing
ElementTree is a built-in Python library that provides a simple and efficient way to parse XML data. It allows you to navigate through the elements of an XML document, manipulate them, and extract information easily.
To parse an XML file using ElementTree, follow these steps:
- Import the library:
“`python
import xml.etree.ElementTree as ET
“`
- Load the XML file:
You can load an XML file from your filesystem or parse a string containing XML data.
“`python
tree = ET.parse(‘file.xml’) For a file
root = tree.getroot() Get the root element
“`
- Accessing elements:
Use methods like `find()`, `findall()`, and `text` to access elements and their data.
“`python
for child in root:
print(child.tag, child.attrib)
“`
- Navigating the XML tree:
You can navigate through the XML structure using the tree’s methods.
- `root` refers to the root element.
- `child` refers to the direct children of the root.
Here’s a simple example of parsing an XML string:
“`python
xml_data = ”’
”’
root = ET.fromstring(xml_data)
for item in root.findall(‘item’):
name = item.find(‘name’).text
value = item.find(‘value’).text
print(f’Name: {name}, Value: {value}’)
“`
Working with XML Attributes
XML elements can also have attributes, which are additional information stored in the element tags. To access these attributes, use the `attrib` property.
Example of accessing attributes:
“`xml
“`
To retrieve the `id` attribute in Python:
“`python
for item in root.findall(‘item’):
item_id = item.attrib[‘id’]
print(f’Item ID: {item_id}’)
“`
Handling Namespaces in XML
XML namespaces help avoid naming conflicts. When working with XML that uses namespaces, you will need to include the namespace when searching for elements.
Example:
“`xml
“`
To parse this XML:
“`python
namespaces = {‘ns’: ‘http://example.com/ns’}
item_name = root.find(‘ns:item/ns:name’, namespaces).text
print(item_name)
“`
Common XML Parsing Methods
Here’s a quick reference table of commonly used methods in ElementTree:
Method | Description |
---|---|
parse(file) | Parses an XML file and returns an ElementTree object. |
fromstring(string) | Parses an XML string and returns the root element. |
find(path) | Finds the first matching subelement. |
findall(path) | Finds all matching subelements. |
iter(tag) | Iterates over all matching elements. |
Utilizing these methods effectively can significantly simplify XML data handling in Python, providing a robust toolset for developers.
Parsing XML with ElementTree
ElementTree is a built-in module in Python that provides a simple and efficient way to parse and manipulate XML data. It is part of the `xml` library and is suitable for most basic XML parsing tasks.
To use ElementTree for XML parsing, follow these steps:
- Import the ElementTree module:
“`python
import xml.etree.ElementTree as ET
“`
- Load the XML data: You can either parse from a string or load from a file.
- From a string:
“`python
xml_data = ”’
root = ET.fromstring(xml_data)
“`
- From a file:
“`python
tree = ET.parse(‘file.xml’)
root = tree.getroot()
“`
- Access elements and attributes: You can navigate the XML tree using methods provided by ElementTree.
- To find elements:
“`python
items = root.findall(‘item’)
for item in items:
print(item.text)
“`
- To access attributes:
“`xml
“`
“`python
item_id = item.get(‘id’)
“`
- Iterate through elements: You can also iterate through the XML elements directly.
“`python
for child in root:
print(child.tag, child.attrib)
“`
Parsing XML with lxml
For more complex XML parsing tasks, the `lxml` library is an excellent choice. It is faster than ElementTree and supports XPath and XSLT.
- Install lxml:
“`bash
pip install lxml
“`
- Import lxml:
“`python
from lxml import etree
“`
- Load XML data: Similar to ElementTree, you can load XML from a string or a file.
- From a string:
“`python
xml_data = ”’
root = etree.fromstring(xml_data)
“`
- From a file:
“`python
with open(‘file.xml’, ‘rb’) as f:
tree = etree.parse(f)
root = tree.getroot()
“`
- Use XPath for querying: lxml allows you to query XML using XPath expressions.
“`python
items = root.xpath(‘//item’)
for item in items:
print(item.text)
“`
- Modify XML: You can also modify XML documents easily.
“`python
new_item = etree.Element(“item”)
new_item.text = “3”
root.append(new_item)
“`
Parsing XML with minidom
The `xml.dom.minidom` module provides a DOM implementation that allows for more complex XML manipulations.
- Import minidom:
“`python
from xml.dom import minidom
“`
- Load XML data:
“`python
doc = minidom.parse(‘file.xml’)
“`
- Access elements:
“`python
items = doc.getElementsByTagName(‘item’)
for item in items:
print(item.firstChild.nodeValue)
“`
- Create and append new elements:
“`python
new_item = doc.createElement(‘item’)
new_item.appendChild(doc.createTextNode(‘3’))
doc.documentElement.appendChild(new_item)
“`
Choosing the right XML parsing library depends on your specific needs: ElementTree for simplicity, lxml for performance and advanced features, or minidom for a DOM-based approach. Each method provides robust functionalities to effectively parse and manipulate XML data in Python.
Expert Insights on Parsing XML in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “When parsing XML in Python, utilizing libraries such as `xml.etree.ElementTree` and `lxml` can significantly streamline the process. These libraries offer robust methods for navigating and manipulating XML data, making it easier to extract the necessary information efficiently.”
Michael Chen (Software Engineer, Open Source Projects). “I recommend starting with `xmltodict` for its simplicity and ease of use. This library converts XML data into a Python dictionary, allowing for straightforward access and manipulation. For more complex XML structures, `lxml` provides advanced features like XPath support, which can be invaluable.”
Sarah Patel (Technical Writer, Python Programming Magazine). “Understanding the structure of the XML you are working with is crucial. Before parsing, familiarize yourself with the document’s schema. This knowledge will guide you in selecting the appropriate parsing method and help avoid common pitfalls, such as handling namespaces and attributes.”
Frequently Asked Questions (FAQs)
What libraries can be used to parse XML in Python?
Python offers several libraries for XML parsing, including `xml.etree.ElementTree`, `lxml`, and `minidom`. Each library has its strengths, with `ElementTree` being part of the standard library, making it widely accessible.
How do I parse an XML file using ElementTree?
To parse an XML file with ElementTree, import the library, use `ElementTree.parse()` to load the XML file, and then navigate the tree structure using methods like `find()`, `findall()`, and `iter()` to access specific elements.
Can I parse XML strings directly in Python?
Yes, you can parse XML strings directly using `xml.etree.ElementTree.fromstring()`. This method allows you to convert a string containing XML data into an Element object for further manipulation.
What is the difference between SAX and DOM parsing in Python?
SAX (Simple API for XML) is an event-driven, streaming approach that reads XML data sequentially, while DOM (Document Object Model) loads the entire XML document into memory, allowing for random access. SAX is more memory efficient, whereas DOM is easier to work with for complex structures.
How can I handle namespaces while parsing XML in Python?
To handle namespaces in XML, you can use the `find()` and `findall()` methods with a namespace dictionary. Define the namespaces in a dictionary and include them in the search queries to correctly locate the elements.
Is it possible to convert XML data to other formats in Python?
Yes, you can convert XML data to various formats such as JSON, CSV, or dictionaries using libraries like `xmltodict` or by manually iterating through the XML structure and formatting it as needed.
Parsing XML in Python can be efficiently accomplished using several libraries, with the most notable being ElementTree, lxml, and minidom. Each of these libraries offers unique features and capabilities, catering to different needs and preferences. ElementTree is part of the standard library, making it easily accessible for basic XML parsing tasks. It provides a simple and intuitive API for navigating and modifying XML trees.
For more complex XML processing, lxml is a powerful alternative that supports XPath and XSLT, making it suitable for advanced users who require more functionality. Additionally, lxml is known for its performance and ability to handle large XML files efficiently. On the other hand, minidom is a lightweight option that is also included in the standard library, offering a more DOM-like interface for those who prefer that style of XML manipulation.
When choosing a library for XML parsing in Python, it is essential to consider the specific requirements of your project, such as performance needs, ease of use, and the complexity of the XML data. Understanding the strengths and weaknesses of each library will help developers make informed decisions that align with their goals. Overall, Python provides robust tools for XML parsing, allowing for flexible and effective data handling in various applications.
Author Profile
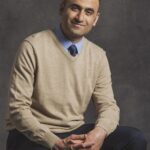
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?