How Can You Effectively Pass Arguments to a Python Script?
In the world of programming, the ability to pass arguments to scripts is a fundamental skill that can significantly enhance the functionality and flexibility of your code. Whether you’re automating tasks, processing data, or building applications, knowing how to effectively pass arguments to your Python scripts can streamline your workflow and make your programs more dynamic. This capability allows you to customize the behavior of your scripts without altering the code itself, empowering you to create more versatile and reusable solutions.
When you run a Python script, you often want to provide it with specific inputs to dictate its behavior. Understanding how to pass these arguments can open up a world of possibilities, allowing you to interact with your scripts in real-time. Python provides several methods to handle command-line arguments, each suited for different scenarios and levels of complexity. From simple positional arguments to more advanced options using libraries like `argparse`, mastering these techniques can elevate your programming prowess.
As we delve deeper into this topic, we will explore the various methods for passing arguments to Python scripts, highlighting their unique features and use cases. By the end of this article, you will have a solid grasp of how to effectively utilize arguments in your Python scripts, enabling you to write more robust and user-friendly code. Whether you’re a beginner or looking to refine your skills, this
Using `sys.argv` to Pass Arguments
One of the most straightforward ways to pass arguments to a Python script is by using the `sys.argv` list from the `sys` module. This list contains the command-line arguments passed to the script, where the first element is always the script name itself.
To utilize `sys.argv`, follow these steps:
- Import the `sys` module at the beginning of your script.
- Access the arguments using `sys.argv`, which is a list where:
- `sys.argv[0]` is the name of the script.
- `sys.argv[1:]` contains all the additional arguments passed.
Here’s a basic example:
“`python
import sys
Print all arguments passed
print(“Script name:”, sys.argv[0])
print(“Arguments:”, sys.argv[1:])
“`
If you run this script with the command `python script.py arg1 arg2`, the output will be:
“`
Script name: script.py
Arguments: [‘arg1’, ‘arg2’]
“`
Using `argparse` for More Complex Scenarios
For more sophisticated argument parsing, the `argparse` module is highly recommended. This module allows for more control over the command-line interface, including types, default values, and help messages. Here’s how to set it up:
- Import the `argparse` module.
- Create an `ArgumentParser` object.
- Define the expected arguments using the `add_argument` method.
- Call `parse_args()` to convert the arguments into an object.
Here’s an example implementation:
“`python
import argparse
Create the parser
parser = argparse.ArgumentParser(description=’Process some integers.’)
Add arguments
parser.add_argument(‘integers’, metavar=’N’, type=int, nargs=’+’,
help=’an integer for the accumulator’)
parser.add_argument(‘–sum’, dest=’accumulate’, action=’store_const’,
const=sum, default=max,
help=’sum the integers (default: find the max)’)
Parse the arguments
args = parser.parse_args()
Display the result
print(args.accumulate(args.integers))
“`
In this example, if the script is executed as `python script.py 1 2 3 –sum`, it will output `6` (the sum of the integers). If run without `–sum`, it will output `3` (the maximum).
Handling Optional Arguments
Using `argparse`, you can also define optional arguments. These are typically denoted with a prefix (like `–`) and can have default values. Below is a comparison of how required and optional arguments can be defined:
Argument Type | Syntax | Description |
---|---|---|
Required | `parser.add_argument(‘name’)` | Must be provided by the user. |
Optional | `parser.add_argument(‘–name’, default=’value’)` | Can be omitted; if not provided, a default value is used. |
This flexibility allows for designing user-friendly command-line interfaces that can accommodate various input scenarios without overwhelming users with mandatory arguments.
Using Command Line Arguments
Python scripts can accept command line arguments, which are parameters provided when executing the script from the command line. These arguments can be accessed using the `sys` module.
To utilize command line arguments:
- Import the `sys` module.
- Access `sys.argv`, which is a list containing the command line arguments.
- The first element, `sys.argv[0]`, is the script name, while subsequent elements are the arguments passed.
Example:
“`python
import sys
Access command line arguments
script_name = sys.argv[0]
arguments = sys.argv[1:]
print(f”Script Name: {script_name}”)
print(f”Arguments: {arguments}”)
“`
Using argparse Module
For more complex argument parsing, Python provides the `argparse` module, which offers a more powerful way to handle arguments.
To implement `argparse`:
- Import the `argparse` module.
- Create a parser object.
- Define the expected arguments using `add_argument()`.
- Call `parse_args()` to retrieve the arguments.
Example:
“`python
import argparse
Create parser
parser = argparse.ArgumentParser(description=”Process some integers.”)
Define arguments
parser.add_argument(‘integers’, metavar=’N’, type=int, nargs=’+’, help=’an integer for the accumulator’)
parser.add_argument(‘–sum’, dest=’accumulate’, action=’store_const’, const=sum, default=max,
help=’sum the integers (default: find the max)’)
Parse arguments
args = parser.parse_args()
print(args.accumulate(args.integers))
“`
Passing Arguments in Scripts
When executing a Python script, you can pass arguments directly in the command line as follows:
“`bash
python script.py arg1 arg2 arg3
“`
- `arg1`, `arg2`, and `arg3` are the arguments that can be accessed in the script.
- Ensure to handle different data types properly according to your needs.
Using Environment Variables
Another method of passing data to a Python script is through environment variables. This can be useful for configuration settings.
To access environment variables:
- Use the `os` module.
- Use `os.environ` to retrieve the value of an environment variable.
Example:
“`python
import os
Access environment variable
value = os.environ.get(‘MY_ENV_VAR’, ‘default_value’)
print(f’The value of MY_ENV_VAR is: {value}’)
“`
Example of a Complete Script
Here’s a complete example that combines command line arguments and environment variables:
“`python
import argparse
import os
Set up argument parsing
parser = argparse.ArgumentParser(description=”Example script with args and env vars.”)
parser.add_argument(‘–name’, type=str, help=’Name of the user’)
Parse arguments
args = parser.parse_args()
Access environment variable
greeting = os.environ.get(‘GREETING’, ‘Hello’)
Print the output
print(f”{greeting}, {args.name}!”)
“`
To run this script, you can use:
“`bash
GREETING=”Hi” python script.py –name John
“`
This will output: `Hi, John!` if the environment variable is set correctly.
Expert Insights on Passing Arguments to Python Scripts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). In Python, passing arguments to scripts can be efficiently accomplished using the `argparse` module. This built-in library allows developers to define expected arguments and automatically generates help and usage messages, making it easier for users to understand how to interact with the script.
Michael Thompson (Python Developer Advocate, CodeCraft). Utilizing command-line arguments is a fundamental skill for Python developers. By employing the `sys.argv` list, one can access arguments directly from the command line. However, for more complex scripts, I recommend `argparse` for its flexibility and user-friendly interface.
Sarah Kim (Lead Data Scientist, Data Insights Group). When designing Python scripts that require user input, it’s crucial to implement robust argument parsing. Not only does `argparse` enhance user experience, but it also allows for type checking and default values, which can significantly reduce errors during execution.
Frequently Asked Questions (FAQs)
How can I pass command-line arguments to a Python script?
You can pass command-line arguments to a Python script by using the `sys` module. Import `sys` and access the arguments via `sys.argv`, where `sys.argv[0]` is the script name and subsequent indices contain the arguments.
What is the difference between `sys.argv` and `argparse`?
`sys.argv` is a simple list of command-line arguments, while `argparse` is a module that provides a more powerful and flexible way to handle command-line arguments, including type checking, help messages, and default values.
Can I pass multiple arguments to a Python script?
Yes, you can pass multiple arguments by separating them with spaces in the command line. Each argument will be captured as a separate element in the `sys.argv` list or parsed using `argparse`.
How do I handle optional arguments in a Python script?
You can handle optional arguments using the `argparse` module by defining them with the `add_argument` method, specifying the `–` prefix for optional arguments. This allows users to provide these arguments without requiring them.
Is it possible to pass arguments of different types to a Python script?
Yes, you can pass arguments of different types. When using `argparse`, you can specify the type of each argument using the `type` parameter in `add_argument`, allowing automatic conversion to the desired type.
How do I access the passed arguments in the script?
In a script using `sys.argv`, access the arguments using indexing, such as `sys.argv[1]` for the first argument. In a script using `argparse`, access the arguments through the namespace returned by `parser.parse_args()`.
Passing arguments to a Python script is a fundamental skill that enhances the functionality and flexibility of your code. The most common method for achieving this is through the use of the `sys` module, which allows you to access command-line arguments via the `sys.argv` list. This list contains the script name as the first element, followed by any additional arguments provided when the script is executed. Understanding how to effectively utilize this feature enables developers to create more dynamic and user-friendly applications.
Another powerful approach for handling command-line arguments is through the `argparse` module. This module provides a more structured way to define expected arguments, including options for types, default values, and help messages. By leveraging `argparse`, developers can create scripts that are not only easier to use but also more robust, as it automatically generates help and usage messages, thereby improving user experience and reducing errors.
In summary, mastering the techniques for passing arguments to Python scripts is essential for any programmer looking to enhance their coding practices. Whether using `sys.argv` for straightforward needs or `argparse` for more complex requirements, understanding these methods allows for greater control over script execution and user interaction. By incorporating these practices, developers can significantly improve the usability and functionality of
Author Profile
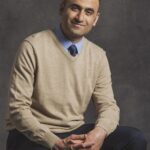
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?