How Can You Effectively Pass Arguments to a Python Script?
In the world of programming, the ability to pass arguments to scripts is a fundamental skill that empowers developers to create flexible and dynamic applications. Whether you’re automating tasks, processing data, or building complex systems, understanding how to effectively pass arguments to a Python script can significantly enhance your coding prowess. This seemingly simple concept opens the door to a multitude of possibilities, allowing your scripts to adapt based on user input or external data sources.
Passing arguments to a Python script enables you to customize its behavior without altering the code itself. By leveraging command-line arguments, you can provide input values that influence the execution of your program in real-time. This not only makes your scripts more versatile but also improves user experience by allowing for greater interactivity. As you delve deeper into this topic, you’ll discover various methods to handle these arguments, from basic positional parameters to more complex options that enhance functionality.
Moreover, understanding how to manage arguments effectively is crucial for developing robust applications. It allows for better error handling, user feedback, and overall code maintainability. As we explore the intricacies of argument passing in Python, you’ll learn about the tools and libraries available to simplify this process, ensuring that your scripts are not only powerful but also user-friendly. Get ready to unlock the full potential of your Python scripts by
Using the sys Module
The most common way to pass arguments to a Python script is by utilizing the `sys` module. This approach allows access to command-line arguments through the `sys.argv` list. The first element in this list, `sys.argv[0]`, is the name of the script itself, while subsequent elements correspond to the arguments passed.
For example, consider a script named `example.py` that is executed as follows:
“`bash
python example.py arg1 arg2 arg3
“`
In this case, `sys.argv` will be:
“`python
import sys
print(sys.argv)
“`
Output:
“`
[‘example.py’, ‘arg1’, ‘arg2’, ‘arg3’]
“`
To extract individual arguments, you can reference them by their index:
“`python
arg1 = sys.argv[1]
arg2 = sys.argv[2]
“`
Using the argparse Module
For more complex command-line interfaces, the `argparse` module is highly recommended. It provides a more robust way to handle command-line arguments, including type checking, default values, and help messages.
Here’s a brief overview of how to use `argparse`:
- Import the `argparse` module.
- Create a parser object.
- Add expected arguments.
- Parse the arguments.
Example:
“`python
import argparse
Create a parser object
parser = argparse.ArgumentParser(description=’Process some integers.’)
Add arguments
parser.add_argument(‘integers’, metavar=’N’, type=int, nargs=’+’, help=’an integer for the accumulator’)
parser.add_argument(‘–sum’, dest=’accumulate’, action=’store_const’,
const=sum, default=max,
help=’sum the integers (default: find the max)’)
Parse the arguments
args = parser.parse_args()
print(args.accumulate(args.integers))
“`
In this example, the script can be executed with:
“`bash
python example.py 1 2 3 –sum
“`
Output:
“`
6
“`
Using the click Module
The `click` module is another excellent library for building command-line interfaces. It allows for more flexible command structures and is user-friendly.
Here’s how to use `click`:
- Install `click` using pip:
“`bash
pip install click
“`
- Create a script using `click` decorators.
Example:
“`python
import click
@click.command()
@click.argument(‘name’)
@click.option(‘–greet’, is_flag=True, help=’Greet the user.’)
def hello(name, greet):
if greet:
click.echo(f’Hello, {name}!’)
else:
click.echo(f’Goodbye, {name}!’)
if __name__ == ‘__main__’:
hello()
“`
You can run this script as follows:
“`bash
python example.py Alice –greet
“`
Output:
“`
Hello, Alice!
“`
Comparison of Argument Handling Methods
Method | Use Case | Pros | Cons |
---|---|---|---|
sys | Simple scripts | Lightweight, built-in | Limited error handling, no types |
argparse | More complex scripts | Robust, built-in help, type checking | Slightly more verbose |
click | User-friendly CLI applications | Intuitive syntax, flexible commands | Requires external installation |
Each method has its advantages and is suitable for different scenarios, allowing developers to choose the one that best fits their needs.
Passing Arguments via Command Line
In Python, the most common way to pass arguments to a script is through the command line using the `sys` module or the `argparse` library. Each method serves different needs based on complexity and usability.
Using the sys Module
The `sys` module provides access to command-line arguments via the `sys.argv` list. This list contains the script name as the first element, followed by any additional arguments.
Example:
“`python
import sys
Accessing command line arguments
script_name = sys.argv[0]
arguments = sys.argv[1:]
print(“Script Name:”, script_name)
print(“Arguments:”, arguments)
“`
- sys.argv: A list where:
- `sys.argv[0]`: Name of the script
- `sys.argv[1]`: First argument passed to the script
- `sys.argv[2]`: Second argument, and so forth.
Using the argparse Module
For more complex command-line parsing, the `argparse` module is recommended. It allows for easier handling of positional and optional arguments, along with automatic help message generation.
Example:
“`python
import argparse
Create the parser
parser = argparse.ArgumentParser(description=’Process some integers.’)
Add arguments
parser.add_argument(‘integers’, metavar=’N’, type=int, nargs=’+’, help=’an integer for the accumulator’)
parser.add_argument(‘–sum’, dest=’accumulate’, action=’store_const’, const=sum, default=max, help=’sum the integers (default: find the max)’)
Parse arguments
args = parser.parse_args()
print(args.accumulate(args.integers))
“`
- Positional arguments: Required inputs that must be provided.
- Optional arguments: Additional inputs that modify the behavior of the script, often prefixed with `–`.
Argument Types and Validation
When defining arguments in `argparse`, you can specify types and validation rules:
Argument Type | Description |
---|---|
`int` | Accepts integer values |
`float` | Accepts floating-point numbers |
`str` | Accepts string values |
`choices` | Restricts input to a set of predefined values |
Example of using choices:
“`python
parser.add_argument(‘–mode’, choices=[‘test’, ‘train’], required=True, help=’Mode of operation’)
“`
Accessing Help and Usage Information
The `argparse` module automatically generates help messages. Users can access this by running the script with the `-h` or `–help` flag. For example:
“`bash
python script.py –help
“`
This will display the usage information and descriptions of all arguments, aiding in user understanding.
Best Practices for Argument Handling
- Keep it Simple: Use `sys.argv` for straightforward scripts with minimal argument requirements.
- Use argparse for Complexity: Opt for `argparse` when dealing with multiple arguments, especially if you need validation, help messages, or default values.
- Provide Clear Help Descriptions: Ensure that all arguments have descriptive help messages to guide users.
- Use Type Checking: Always define the type of each argument to prevent runtime errors and improve user experience.
Expert Insights on Passing Arguments to Python Scripts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Passing arguments to a Python script can be accomplished using the `sys` module, which allows you to access command-line arguments via `sys.argv`. This method enables scripts to accept input dynamically, enhancing their versatility.”
Michael Chen (Data Scientist, Analytics Hub). “Utilizing the `argparse` library is a best practice for passing arguments to Python scripts. It provides a user-friendly interface for defining expected arguments, generating help messages, and ensuring that input is validated, which is crucial for robust applications.”
Sarah Johnson (Python Developer, CodeCraft Solutions). “For more complex applications, consider using `click` or `fire` libraries. These tools simplify argument parsing and enhance user experience by allowing for more intuitive command-line interfaces, making your scripts easier to use and maintain.”
Frequently Asked Questions (FAQs)
How can I pass command-line arguments to a Python script?
You can pass command-line arguments to a Python script using the `sys` module. Import `sys` and access the arguments via `sys.argv`, where `sys.argv[0]` is the script name and subsequent indices are the arguments.
What is the difference between positional and keyword arguments in Python?
Positional arguments are passed to a function in the order they are defined, while keyword arguments are specified by name, allowing you to pass them in any order. This distinction is crucial for clarity and flexibility in function calls.
How do I handle multiple arguments in a Python script?
You can handle multiple arguments by using the `argparse` module, which allows you to define expected arguments and automatically generates help messages. This approach enhances usability and error handling.
Can I pass default values for arguments in a Python script?
Yes, you can set default values for arguments by defining them in the function signature. If an argument is not provided during the function call, the default value will be used.
What is the purpose of the `*args` and `**kwargs` syntax in Python?
The `*args` syntax allows you to pass a variable number of positional arguments to a function, while `**kwargs` allows you to pass a variable number of keyword arguments. This flexibility is useful for functions that need to accept a varying number of inputs.
How can I read arguments from a configuration file instead of command-line arguments?
You can read arguments from a configuration file using the `configparser` module. This allows you to define parameters in a structured format and access them programmatically within your script.
Passing arguments to a Python script is a fundamental skill that enhances the flexibility and functionality of your programs. The most common method to achieve this is by utilizing the `sys` module, specifically the `sys.argv` list, which contains the command-line arguments passed to the script. This method allows you to access arguments as strings, enabling your script to accept user input directly from the command line when it is executed. Additionally, the `argparse` module provides a more advanced and user-friendly approach for handling command-line arguments, allowing for the definition of expected arguments, types, and help messages.
Another important aspect of passing arguments is understanding the distinction between positional and optional arguments. Positional arguments must be provided in a specific order, while optional arguments can be specified in any order and often come with default values. This flexibility allows developers to create more robust scripts that can accommodate various use cases and user preferences.
In summary, mastering the techniques for passing arguments to a Python script is essential for developing interactive and dynamic applications. By leveraging the `sys` and `argparse` modules, programmers can create scripts that not only accept input from users but also provide clear instructions and error handling. This enhances the overall user experience and makes scripts more versatile
Author Profile
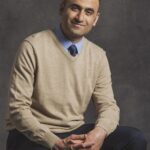
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?