How Can You Plot a Function in Python Effortlessly?
### Introduction
In the world of data science and programming, visualizing functions is a powerful tool that can transform abstract concepts into tangible insights. Whether you’re a student grappling with calculus, a researcher analyzing complex data sets, or a hobbyist exploring the realms of mathematics and programming, the ability to plot functions in Python opens up a universe of possibilities. With its rich ecosystem of libraries and tools, Python has become a go-to language for anyone looking to create stunning visual representations of mathematical functions.
Plotting a function in Python is not just about drawing graphs; it’s about enhancing understanding and communication. By visualizing relationships between variables, you can uncover patterns, identify trends, and make informed decisions based on data. From simple linear equations to intricate multi-dimensional surfaces, Python provides a variety of methods to bring your mathematical ideas to life. The flexibility of libraries like Matplotlib, NumPy, and Seaborn allows users to customize their plots, making them as informative and aesthetically pleasing as needed.
As we delve deeper into the process of plotting functions in Python, you’ll discover the essential tools and techniques that will empower you to create your own visualizations. Whether you’re interested in static plots or interactive graphs, this guide will equip you with the knowledge to effectively represent mathematical functions and enhance your analytical capabilities
Importing Necessary Libraries
To plot a function in Python, you typically need to use libraries such as `Matplotlib` and `NumPy`. These libraries provide extensive functionalities for creating a wide range of plots and handling numerical data.
- Matplotlib: A plotting library that offers an object-oriented API for embedding plots into applications.
- NumPy: A library for numerical operations that allows for efficient array computations.
To get started, ensure that you have these libraries installed. You can install them using pip if they are not already available in your environment:
bash
pip install matplotlib numpy
Once installed, you can import them in your Python script as follows:
python
import numpy as np
import matplotlib.pyplot as plt
Defining the Function
Before plotting, you need to define the mathematical function you wish to visualize. For example, let’s consider a simple quadratic function:
python
def f(x):
return x**2
This function takes an input `x` and returns its square.
Generating Data Points
To visualize the function, you’ll need to generate a range of x-values and compute the corresponding y-values using the defined function. You can use NumPy’s `linspace` to create an array of x-values.
python
x_values = np.linspace(-10, 10, 100) # Generates 100 points from -10 to 10
y_values = f(x_values) # Compute y-values using the function f
This approach ensures a smooth curve in the plot by generating a sufficient number of data points.
Plotting the Function
With the x and y values prepared, you can now create the plot using Matplotlib. Here’s how you can do that:
python
plt.plot(x_values, y_values, label=’f(x) = x^2′, color=’blue’)
plt.title(‘Plot of f(x) = x^2’)
plt.xlabel(‘x-axis’)
plt.ylabel(‘y-axis’)
plt.axhline(0, color=’black’,linewidth=0.5, ls=’–‘) # Horizontal line at y=0
plt.axvline(0, color=’black’,linewidth=0.5, ls=’–‘) # Vertical line at x=0
plt.grid(color = ‘gray’, linestyle = ‘–‘, linewidth = 0.5)
plt.legend()
plt.show()
This code snippet will generate a plot of the function \( f(x) = x^2 \) with labeled axes and a grid for better readability.
Customizing the Plot
Matplotlib allows for extensive customization options for your plot. You can modify colors, line styles, markers, and other attributes to enhance the visual appeal of your plots. Here are some common customization options:
- Line Styles: Change the line style using `linestyle` parameter (e.g., `linestyle=’–‘` for dashed lines).
- Colors: Specify colors using color names or HEX codes.
- Markers: Add markers to points in the plot (e.g., `marker=’o’`).
For example, to customize the line style and add markers, you can modify the `plot` function as follows:
python
plt.plot(x_values, y_values, label=’f(x) = x^2′, color=’red’, linestyle=’–‘, marker=’o’)
Attribute | Description |
---|---|
color | Sets the color of the line. |
linestyle | Defines the style of the line (solid, dashed, etc.). |
marker | Specifies the shape of the markers at data points. |
Utilizing these options will help create visually informative plots tailored to your preferences.
Utilizing Matplotlib for Function Plotting
Matplotlib is a versatile and widely-used library in Python for creating static, animated, and interactive visualizations. To plot a function using Matplotlib, you can follow these steps:
- Install Matplotlib: If not already installed, you can do this via pip. Open your terminal or command prompt and execute:
bash
pip install matplotlib
- Import Necessary Libraries: You need to import both Matplotlib and NumPy, which is useful for numerical operations.
python
import matplotlib.pyplot as plt
import numpy as np
- Define the Function: Create a Python function that you wish to plot. For example, to plot \( f(x) = x^2 \):
python
def f(x):
return x ** 2
- Generate Data Points: Use NumPy to generate an array of x values and compute the corresponding y values using your function.
python
x = np.linspace(-10, 10, 100) # 100 points from -10 to 10
y = f(x)
- Create the Plot: Use Matplotlib to create the plot.
python
plt.plot(x, y, label=’f(x) = x^2′)
- Customize the Plot: Add titles, labels, and legends for better understanding.
python
plt.title(‘Plot of f(x) = x^2’)
plt.xlabel(‘x-axis’)
plt.ylabel(‘y-axis’)
plt.axhline(0, color=’black’,linewidth=0.5, ls=’–‘)
plt.axvline(0, color=’black’,linewidth=0.5, ls=’–‘)
plt.grid(color = ‘gray’, linestyle = ‘–‘, linewidth = 0.5)
plt.legend()
- Display the Plot: Finally, use `plt.show()` to display the plot.
python
plt.show()
Example Code
Here is a complete example for plotting the function \( f(x) = x^2 \):
python
import matplotlib.pyplot as plt
import numpy as np
def f(x):
return x ** 2
x = np.linspace(-10, 10, 100)
y = f(x)
plt.plot(x, y, label=’f(x) = x^2′)
plt.title(‘Plot of f(x) = x^2’)
plt.xlabel(‘x-axis’)
plt.ylabel(‘y-axis’)
plt.axhline(0, color=’black’,linewidth=0.5, ls=’–‘)
plt.axvline(0, color=’black’,linewidth=0.5, ls=’–‘)
plt.grid(color = ‘gray’, linestyle = ‘–‘, linewidth = 0.5)
plt.legend()
plt.show()
Advanced Plotting Techniques
For more advanced plotting capabilities, consider the following techniques:
- Multiple Functions: Plot multiple functions on the same graph by calling `plt.plot()` for each function.
- Subplots: Use `plt.subplot()` to create multiple plots in a single figure.
- Styling: Customize colors, line styles, and markers using parameters in the `plt.plot()` function.
Here is an example of plotting two functions in a single figure with subplots:
python
x = np.linspace(-10, 10, 100)
y1 = f(x)
y2 = np.sin(x)
plt.subplot(2, 1, 1) # 2 rows, 1 column, 1st subplot
plt.plot(x, y1, label=’f(x) = x^2′, color=’blue’)
plt.title(‘Plot of f(x) = x^2′)
plt.grid()
plt.subplot(2, 1, 2) # 2nd subplot
plt.plot(x, y2, label=’f(x) = sin(x)’, color=’red’)
plt.title(‘Plot of f(x) = sin(x)’)
plt.grid()
plt.tight_layout() # Adjust spacing
plt.show()
Using these techniques will allow you to create comprehensive and informative visualizations of mathematical functions in Python.
Expert Insights on Plotting Functions in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “When plotting functions in Python, utilizing libraries such as Matplotlib and Seaborn is essential. They provide extensive customization options and are widely accepted in the data science community for their versatility and ease of use.”
Michael Chen (Software Engineer, Open Source Advocate). “For beginners, I recommend starting with Matplotlib due to its straightforward syntax. Once comfortable, transitioning to libraries like Plotly can enhance interactivity in visualizations, which is crucial for dynamic data presentations.”
Lisa Morales (Professor of Computer Science, University of Tech). “Understanding the fundamentals of plotting in Python is vital. I suggest exploring the documentation of each library thoroughly, as well as engaging with community forums. This will not only improve your skills but also expose you to best practices in data visualization.”
Frequently Asked Questions (FAQs)
How do I install the necessary libraries to plot a function in Python?
To plot a function in Python, you typically need to install libraries such as Matplotlib and NumPy. You can install them using pip with the following command: `pip install matplotlib numpy`.
What is the basic syntax for plotting a function using Matplotlib?
The basic syntax involves importing the Matplotlib library, defining the function, generating x values, computing the corresponding y values, and then using `plt.plot()` to create the plot. Finally, use `plt.show()` to display the plot.
Can I customize the appearance of my plot in Python?
Yes, Matplotlib allows extensive customization of plots. You can modify colors, line styles, markers, labels, and titles using various parameters and functions such as `plt.title()`, `plt.xlabel()`, and `plt.ylabel()`.
How can I plot multiple functions on the same graph?
To plot multiple functions on the same graph, simply call `plt.plot()` for each function before calling `plt.show()`. Ensure to differentiate each function using labels and legends for clarity.
Is it possible to save my plot to a file in Python?
Yes, you can save your plot to a file using the `plt.savefig()` function. Specify the filename and format (e.g., PNG, PDF) as arguments to this function before calling `plt.show()`.
What should I do if my plot does not display correctly?
If your plot does not display correctly, check for common issues such as incorrect data ranges, missing `plt.show()` call, or conflicts with other libraries. Ensure that your environment supports graphical output.
Plotting a function in Python is a straightforward process that can be accomplished using various libraries, with Matplotlib being the most popular choice. To begin, one must first install the Matplotlib library if it is not already available in the Python environment. This can be done using package managers like pip. Once installed, users can import the library and utilize its functions to create visual representations of mathematical functions.
The typical workflow for plotting a function involves defining the function itself, generating a range of x-values, and then computing the corresponding y-values. The plotting functions provided by Matplotlib allow for customization of the plot, including setting titles, labels, and legends, which enhance the clarity and presentation of the data. Additionally, users can save their plots in various formats, such as PNG or PDF, for further use or sharing.
Key takeaways from this discussion include the importance of understanding the basic structure of a plot, which consists of axes, data points, and labels. Familiarity with other libraries such as NumPy can also be beneficial, as it provides powerful tools for numerical operations that can simplify the process of generating data for plots. Overall, mastering the art of plotting functions in Python opens up numerous possibilities for data visualization and analysis.
Author Profile
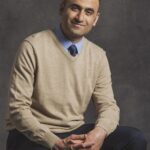
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?