How Can You Print an Array in Python? A Step-by-Step Guide
In the world of programming, arrays are fundamental data structures that allow us to store collections of items efficiently. Whether you’re working with numbers, strings, or even complex objects, understanding how to manipulate and display these arrays is crucial for effective coding in Python. If you’ve ever found yourself wondering how to print an array in Python, you’re not alone. This simple yet essential skill can unlock a plethora of possibilities, enabling you to visualize data, debug your code, and present information in a user-friendly manner.
Printing an array in Python may seem straightforward, but it encompasses various methods and techniques that cater to different needs and scenarios. From basic print statements to more advanced formatting options, Python offers a rich set of tools to help you display your data clearly and concisely. In this article, we will explore the various ways to print arrays, highlighting the nuances that can enhance your coding experience and improve the readability of your output.
As we delve into the topic, you’ll discover not only the syntax and functions available for printing arrays but also best practices that can elevate your programming skills. Whether you’re a beginner looking to grasp the basics or an experienced coder seeking to refine your techniques, this guide will provide you with the knowledge you need to print arrays effectively in Python. Get ready to
Using the Print Function
To print an array in Python, the most straightforward method is to utilize the built-in `print()` function. When printing a list or array, Python will automatically format the output to show the contents in a readable manner.
“`python
Example of printing a list
my_array = [1, 2, 3, 4, 5]
print(my_array)
“`
This code will output:
“`
[1, 2, 3, 4, 5]
“`
Iterating Through an Array
For more control over the formatting of the output, you can iterate through the elements of the array using a loop. This method allows you to customize how each element is printed.
“`python
Using a for loop to print each element
for element in my_array:
print(element)
“`
This will print each element on a new line:
“`
1
2
3
4
5
“`
Printing Arrays with Custom Formatting
If you wish to format the output in a specific way, you can use string formatting techniques. For instance, you may want to separate elements with commas or display them in a tabular format.
“`python
Joining elements with a comma
print(“, “.join(str(x) for x in my_array))
“`
This will produce:
“`
1, 2, 3, 4, 5
“`
Using the NumPy Library
When dealing with numerical data, the NumPy library offers an efficient way to handle arrays. If you are working with NumPy arrays, the `print()` function can also be used, but NumPy has its own methods for enhanced output formatting.
“`python
import numpy as np
Creating a NumPy array
np_array = np.array([[1, 2, 3], [4, 5, 6]])
Printing the NumPy array
print(np_array)
“`
The output will be:
“`
[[1 2 3]
[4 5 6]]
“`
Table of Array Printing Methods
Below is a summary of various methods for printing arrays in Python:
Method | Description | Example |
---|---|---|
print() | Basic output of the array | print(my_array) |
for loop | Iterate for custom output | for element in my_array: print(element) |
join() | Output with specific separators | print(“, “.join(str(x) for x in my_array)) |
NumPy print | Output with NumPy arrays | print(np_array) |
Methods to Print an Array in Python
In Python, arrays can be represented using lists or the `array` module. Below are various methods to print an array.
Using the print() Function
The simplest way to print an array is by using the built-in `print()` function. This method works for both lists and arrays created with the `array` module.
“`python
Example with a list
my_list = [1, 2, 3, 4, 5]
print(my_list)
Example with an array
import array as arr
my_array = arr.array(‘i’, [1, 2, 3, 4, 5])
print(my_array)
“`
Formatting Output with Loops
For more controlled formatting, especially for multi-dimensional arrays, you can utilize loops. This method allows for customization of how elements are presented.
“`python
Example with a 2D list
my_2d_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
for row in my_2d_list:
for item in row:
print(item, end=’ ‘)
print() New line after each row
“`
Using the join() Method
To print a one-dimensional array as a string, `join()` can be employed, converting each element to a string format.
“`python
Example with a list
my_list = [1, 2, 3, 4, 5]
print(” “.join(map(str, my_list)))
“`
Utilizing NumPy for Arrays
When working with numerical data, NumPy is a powerful library. Arrays created with NumPy can be printed in a more structured way.
“`python
import numpy as np
Creating a NumPy array
my_numpy_array = np.array([[1, 2, 3], [4, 5, 6]])
Printing the NumPy array
print(my_numpy_array)
“`
Custom Formatting with f-Strings
For personalized formatting, f-strings can be used. This feature is particularly useful when you need to display each element with specific formatting.
“`python
my_list = [1, 2, 3, 4, 5]
for item in my_list:
print(f’Item: {item}’)
“`
Using PrettyPrint for Enhanced Output
The `pprint` module allows for more readable outputs, especially for nested structures.
“`python
from pprint import pprint
my_complex_structure = {‘key1’: [1, 2, 3], ‘key2’: [[4, 5], [6, 7]]}
pprint(my_complex_structure)
“`
Summary of Methods
Method | Description |
---|---|
`print()` | Basic printing for lists and arrays. |
Loops | Custom formatting for multi-dimensional arrays. |
`join()` | Convert and print list elements as a string. |
NumPy | Structured printing for numerical arrays. |
f-Strings | Personalized formatting for output. |
`pprint` | Enhanced readability for complex structures. |
Utilizing these methods allows for flexibility and control over how arrays are printed in Python, catering to various needs in data representation and readability.
Expert Insights on Printing Arrays in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Printing an array in Python can be straightforward using the built-in print function. However, for more complex data structures, utilizing libraries like NumPy can provide enhanced functionality and readability.”
Michael Chen (Data Scientist, Analytics Hub). “When working with large datasets, it’s crucial to format the output properly. Using the pprint module can help with printing nested arrays in a more readable format, which is essential for data analysis.”
Sarah Johnson (Software Engineer, CodeCraft Solutions). “For beginners, starting with simple lists and understanding the use of loops to print elements can build a strong foundation. As you progress, exploring list comprehensions can also enhance your coding efficiency.”
Frequently Asked Questions (FAQs)
How do I print a simple array in Python?
You can print a simple array in Python using the built-in `print()` function. For example, if you have an array defined as `arr = [1, 2, 3]`, you can print it by executing `print(arr)`.
What is the difference between printing a list and an array in Python?
In Python, a list is a built-in data structure, while an array requires importing the `array` module or using libraries like NumPy. The `print()` function works similarly for both, but arrays may have additional formatting options depending on their type.
Can I format the output when printing an array in Python?
Yes, you can format the output using string formatting methods such as f-strings or the `format()` method. For example, `print(f’Array elements: {arr}’)` allows for customized output.
How can I print each element of an array on a new line?
You can achieve this by using a loop. For example:
“`python
for element in arr:
print(element)
“`
This will print each element of the array on a separate line.
Is there a way to print the array with indices?
Yes, you can use the `enumerate()` function to print the array with indices. For example:
“`python
for index, value in enumerate(arr):
print(f’Index {index}: {value}’)
“`
What libraries can help with printing arrays in a more structured way?
Libraries like NumPy provide enhanced functionality for printing arrays. For instance, using `numpy.array(arr)` and then `print(np.array(arr))` can display the array in a more structured format, especially for multi-dimensional arrays.
Printing an array in Python can be accomplished using various methods, depending on the specific requirements and the type of array being utilized. The most common approach is to use the built-in `print()` function, which can display the contents of a list or an array directly to the console. For more complex data structures, such as NumPy arrays, the `print()` function can still be employed, but additional formatting options may enhance readability and presentation.
When working with lists, one can simply pass the list to the `print()` function. For instance, `print(my_list)` will output the entire list. In contrast, if the goal is to print each element on a new line, a loop can be implemented, such as `for item in my_list: print(item)`. This flexibility allows users to tailor the output to their needs, whether for debugging or displaying results to end-users.
For those using NumPy, the library offers its own array structure, which can be printed using the same `print()` function. However, NumPy arrays come with additional methods, such as `numpy.array_str()` and `numpy.array_repr()`, that provide more control over the formatting of the output. This is particularly useful when dealing with large
Author Profile
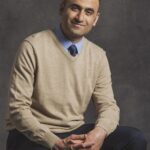
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?