How Can You Print ASCII Art in Python? A Step-by-Step Guide!
In the world of programming, creativity often intertwines with functionality, and one delightful way to express this blend is through ASCII art. This unique form of visual art, crafted using the characters of the ASCII standard, has a nostalgic charm that harkens back to the early days of computing. Whether you’re looking to add a touch of whimsy to your console applications or simply want to impress your friends with your coding prowess, learning how to print ASCII art in Python can be a rewarding endeavor. In this article, we will explore the fascinating realm of ASCII art and guide you through the process of bringing your artistic visions to life using Python.
ASCII art is more than just a collection of characters; it’s a creative outlet that allows programmers to convey messages, emotions, and even intricate designs through text. With Python’s straightforward syntax and powerful libraries, creating and displaying ASCII art becomes an accessible and enjoyable task. From simple shapes to complex illustrations, the possibilities are endless, and the only limit is your imagination.
As we delve deeper into this topic, we will cover the various methods available for generating and printing ASCII art in Python. Whether you prefer crafting your own designs or utilizing existing libraries, you’ll discover the tools and techniques that can help you unleash your inner artist. So,
Methods to Print ASCII Art in Python
Printing ASCII art in Python can be accomplished through several methods, each suitable for different use cases. Below are some common approaches to achieve this.
Using Print Statements
The simplest way to display ASCII art is by using multiple print statements. Each line of the ASCII art can be printed individually or as a single multi-line string. Here’s an example:
“`python
print(” * “)
print(” *** “)
print(“*”)
“`
Alternatively, you can use triple quotes to print the ASCII art as a block:
“`python
ascii_art = “””
*
***
*
“””
print(ascii_art)
“`
This method is straightforward but may become cumbersome for large ASCII art.
Storing ASCII Art in a List
For more complex ASCII art, you can store each line in a list and use a loop to print each line. This approach enhances readability and manageability:
“`python
ascii_art = [
” * “,
” *** “,
“*”
]
for line in ascii_art:
print(line)
“`
This way, you can easily modify or extend the ASCII art by adding or removing elements from the list.
Using External Libraries
For more intricate designs or to simplify the process, consider using libraries that handle ASCII art. Libraries such as `pyfiglet` can generate ASCII art from text input. First, install the library using pip:
“`bash
pip install pyfiglet
“`
After installation, you can use it as follows:
“`python
import pyfiglet
ascii_art = pyfiglet.figlet_format(“Hello”)
print(ascii_art)
“`
This method provides a plethora of font styles and configurations, allowing for a diverse range of ASCII art styles.
Using ASCII Art Files
Another effective method is to store ASCII art in a text file and read it into your Python program. This allows for the easy reuse of large ASCII art pieces without cluttering your code:
- Create a text file named `art.txt` containing your ASCII art.
- Read and print the content in Python:
“`python
with open(‘art.txt’, ‘r’) as file:
print(file.read())
“`
This approach is particularly useful when working with large artworks or when you want to maintain a clean codebase.
ASCII Art Examples
Here is a small table showcasing various ASCII art representations:
Art Type | Example |
---|---|
Heart |
** **
|
Cat |
/\_/\ ( o.o ) > ^ < |
This table provides a glimpse into the variety of ASCII art that can be created or printed using Python, underscoring the flexibility and creativity that the medium allows.
Printing ASCII Art in Python
To print ASCII art in Python, you can utilize several methods depending on your needs and the complexity of the ASCII art. Below are the most common approaches:
Using Multi-Line Strings
One of the simplest ways to print ASCII art in Python is by using multi-line strings. You can define the ASCII art as a string with triple quotes and then print it directly.
```python
ascii_art = """
/\_/\
( o.o )
> ^ <
"""
print(ascii_art)
```
Defining a Function for Reusability
For better organization and reusability, you can encapsulate the ASCII art in a function. This allows you to print the art multiple times or in different contexts.
```python
def print_cat():
ascii_art = """
/\_/\
( o.o )
> ^ <
"""
print(ascii_art)
Call the function to display the art
print_cat()
```
Using External Libraries
For more complex ASCII art, consider using external libraries such as `pyfiglet` or `art`. These libraries can convert text into stylized ASCII art.
- Installation: You can install `pyfiglet` with pip:
```bash
pip install pyfiglet
```
- Usage:
```python
import pyfiglet
ascii_art = pyfiglet.figlet_format("Hello, World!")
print(ascii_art)
```
ASCII Art from Files
If your ASCII art is saved in a text file, you can read and print it within your Python script.
- **Create an ASCII art file** (e.g., `art.txt`):
```
/\_/\
( o.o )
> ^ <
```
- Read and print the file:
```python
with open('art.txt', 'r') as file:
ascii_art = file.read()
print(ascii_art)
```
Customizing Output
You can also customize how ASCII art is displayed in the console, such as changing the text color using the `colorama` library.
- Installation:
```bash
pip install colorama
```
- Example:
```python
from colorama import init, Fore
init(autoreset=True) Initialize Colorama
print(Fore.GREEN + ascii_art)
```
This allows for visually distinct ASCII art that stands out in the console output.
Using these methods, you can effectively print and manage ASCII art in Python. Whether you choose to use simple strings, functions, libraries, or files, each method has its advantages and can be tailored to your specific requirements.
Expert Insights on Printing ASCII Art in Python
Dr. Emily Carter (Computer Science Professor, Tech University). "Printing ASCII art in Python is a straightforward process that can be accomplished using simple print statements. However, leveraging libraries like `pyfiglet` can enhance the aesthetics and complexity of the art, making it a valuable tool for educators and developers alike."
Michael Chen (Software Developer, Creative Coders Inc.). "Utilizing Python's built-in capabilities, such as the `print()` function, allows for the easy display of ASCII art. For those looking to create dynamic art, I recommend exploring string manipulation techniques to adjust the output based on user input or other variables."
Sarah Thompson (Technical Writer, Python Programming Monthly). "When it comes to printing ASCII art in Python, clarity and readability are essential. Using triple quotes for multi-line strings can simplify the process, while also ensuring that the art maintains its intended structure when displayed in the console."
Frequently Asked Questions (FAQs)
How can I create ASCII art in Python?
You can create ASCII art in Python by using string literals that represent the desired art. You can also utilize libraries such as `pyfiglet` or `art` to generate ASCII representations of text.
What libraries are available for printing ASCII art in Python?
Popular libraries for printing ASCII art in Python include `pyfiglet`, `art`, and `asciimatics`. These libraries provide various functionalities to create and display ASCII art easily.
How do I install the `pyfiglet` library?
You can install the `pyfiglet` library using pip. Run the command `pip install pyfiglet` in your terminal or command prompt to install it.
Can I print ASCII art directly to the console?
Yes, you can print ASCII art directly to the console using the `print()` function in Python. For example, `print("Your ASCII Art Here")` will display the art in the console.
Is it possible to customize the font of ASCII art?
Yes, when using libraries like `pyfiglet`, you can customize the font by specifying the font name in the function call. For example, `pyfiglet.figlet_format("Hello", font="slant")` allows you to choose a specific font style.
Are there any online tools to generate ASCII art for Python?
Yes, there are several online tools available, such as `patorjk.com/software/taag`, which allow you to generate ASCII art from text. You can then copy and paste the generated art into your Python code.
Printing ASCII art in Python is a straightforward process that can enhance the visual appeal of console applications or scripts. By utilizing simple print statements, you can display ASCII art directly in the terminal. Additionally, you can store ASCII art in multi-line strings or text files, which allows for more complex designs and easier management of the art assets.
One effective approach to printing ASCII art is to use Python's built-in print function, which can handle multi-line strings formatted with triple quotes. This method allows for easy integration of ASCII art into your code without the need for additional libraries. For more advanced applications, libraries such as `pyfiglet` can be employed to generate ASCII art from text dynamically, providing a range of styles and fonts.
whether you choose to create your own ASCII art or utilize existing libraries, Python offers flexible options for incorporating this art form into your projects. By understanding the various methods available, you can effectively enhance your Python applications with visually engaging text representations that capture attention and convey creativity.
Author Profile
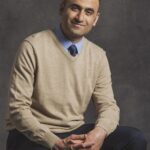
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?