How Can You Print in TypeScript? A Step-by-Step Guide
Printing in TypeScript is a fundamental skill that can enhance your development experience, whether you’re creating web applications or building server-side solutions. As a superset of JavaScript, TypeScript brings strong typing and modern features to the table, making it an attractive choice for developers looking to build robust applications. However, when it comes to outputting data, especially for debugging or displaying information to users, understanding how to effectively print in TypeScript becomes essential.
In TypeScript, printing can be accomplished through various methods, each serving different purposes and contexts. From simple console logging for debugging to rendering data in the user interface, the approach you choose can significantly impact the clarity and usability of your application. By mastering these printing techniques, you can not only improve your debugging process but also enhance the overall user experience by presenting data in a clear and engaging manner.
As we delve deeper into the nuances of printing in TypeScript, we will explore the various methods available, including console output, DOM manipulation, and more. By the end of this article, you’ll be equipped with the knowledge to effectively print and present data in your TypeScript applications, empowering you to create more interactive and user-friendly experiences.
Using Console for Output
In TypeScript, one of the most straightforward methods to print output is by using the `console` object. This object is part of the JavaScript environment and can be utilized for debugging and logging information.
- console.log(): The most commonly used method to print messages to the console.
- console.error(): Used to print error messages.
- console.warn(): Prints warning messages.
- console.table(): Displays tabular data as a table.
Here’s a brief example of how to use these methods:
“`typescript
let message: string = “Hello, TypeScript!”;
console.log(message);
console.error(“This is an error message”);
console.warn(“This is a warning message”);
console.table([{ name: “John”, age: 30 }, { name: “Jane”, age: 25 }]);
“`
Outputting to the DOM
If you want to display output directly on a web page instead of the console, you can manipulate the Document Object Model (DOM). This involves selecting an HTML element and updating its content.
Example of printing to the DOM:
“`typescript
document.getElementById(“output”).innerText = “Hello, TypeScript in the DOM!”;
“`
Make sure to have an HTML element like this in your document:
“`html
“`
Using Alert Dialogs
Another method to display output is through alert dialogs, which are a part of the browser’s window object. While not commonly used for logging, they can be effective for simple notifications.
Example of using an alert dialog:
“`typescript
alert(“This is an alert message!”);
“`
Summary of Printing Methods
The following table summarizes the different methods available for printing output in TypeScript:
Method | Description | Use Case |
---|---|---|
console.log() | Logs general information | Debugging |
console.error() | Logs error messages | Error handling |
console.warn() | Logs warning messages | Warning notifications |
console.table() | Displays tabular data | Data visualization |
document.getElementById().innerText | Updates HTML content | Displaying information on web pages |
alert() | Displays an alert dialog | Simple notifications |
Printing Objects and Arrays
When working with complex data structures like objects and arrays, you can still utilize `console.log()` to print them effectively. TypeScript preserves the structure of these data types, allowing for clear output in the console.
Example of printing an object and an array:
“`typescript
let user = { name: “Alice”, age: 28 };
let users = [{ name: “Bob”, age: 22 }, { name: “Charlie”, age: 30 }];
console.log(user);
console.log(users);
“`
This will display the object and array in a readable format in the console, enabling better debugging and understanding of the data structure.
Printing in TypeScript
In TypeScript, printing to the console is achieved using the built-in `console` object. It provides various methods to display messages, objects, and errors in the console during development.
Common Console Methods
The primary methods for printing in TypeScript include:
- console.log(): Outputs general messages and variables.
- console.error(): Displays error messages, often in red text.
- console.warn(): Shows warnings, typically in yellow.
- console.info(): Provides informational messages, similar to `log` but with a different styling.
- console.table(): Displays tabular data in a more readable format.
Usage Examples
Here are examples illustrating how to use these methods:
“`typescript
let message: string = “Hello, TypeScript!”;
console.log(message); // Outputs: Hello, TypeScript!
let error: string = “An error occurred!”;
console.error(error); // Outputs: An error occurred! (in red)
let warning: string = “This is a warning!”;
console.warn(warning); // Outputs: This is a warning! (in yellow)
let info: string = “This is some information.”;
console.info(info); // Outputs: This is some information.
let users: Array<{name: string, age: number}> = [
{name: “Alice”, age: 30},
{name: “Bob”, age: 25}
];
console.table(users); // Displays users in a table format
“`
Printing Objects and Arrays
To print complex objects or arrays, `console.log()` and `console.table()` are particularly useful. Here’s how to use them effectively:
- Using `console.log()`:
- Suitable for debugging by printing entire objects.
- Can be formatted using placeholders.
Example:
“`typescript
let user = { name: “John”, age: 28 };
console.log(“User Info: “, user); // Outputs: User Info: { name: “John”, age: 28 }
“`
- Using `console.table()`:
- Ideal for displaying arrays of objects.
- Provides a clear view of data in a structured format.
Example:
“`typescript
let products = [
{ id: 1, name: “Laptop”, price: 999 },
{ id: 2, name: “Phone”, price: 499 }
];
console.table(products); // Displays products in a table
“`
Customizing Output with String Interpolation
TypeScript supports template literals, allowing for cleaner and more readable string interpolation. This can enhance your console output significantly:
“`typescript
let userName: string = “Alice”;
let userAge: number = 30;
console.log(`User: ${userName}, Age: ${userAge}`); // Outputs: User: Alice, Age: 30
“`
Using template literals not only simplifies string construction but also helps maintain clarity when printing multiple variables.
Best Practices
To ensure effective debugging and logging, consider the following best practices:
- Use specific methods for different types of messages (e.g., `console.error()` for errors).
- Avoid leaving console statements in production code; use conditional logging if necessary.
- Structure console outputs for readability, especially when dealing with complex data.
- Regularly review console outputs to ensure they provide meaningful information.
By adhering to these practices, you can optimize your development workflow and maintain clean, understandable code.
Expert Insights on Printing in TypeScript
Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To print in TypeScript, developers typically utilize the `console.log()` method, which is a straightforward way to output data to the console. This method is essential for debugging and logging purposes, especially when working with complex applications.”
Michael Thompson (Lead TypeScript Developer, CodeCraft Solutions). “In addition to `console.log()`, TypeScript allows for more structured logging through libraries like Winston or Log4js. These libraries provide advanced features such as log levels and output formatting, which can be crucial for larger applications needing robust logging capabilities.”
Sarah Kim (TypeScript Advocate and Educator, Code Academy). “When printing data in TypeScript, it’s important to remember that TypeScript is a superset of JavaScript. Therefore, any JavaScript printing methods, such as `alert()` or `document.write()`, can also be utilized, but they may not be the best practices for modern web applications.”
Frequently Asked Questions (FAQs)
How do I print to the console in TypeScript?
You can print to the console in TypeScript using the `console.log()` method. For example, `console.log(“Hello, World!”);` outputs “Hello, World!” to the console.
Can I print objects in TypeScript?
Yes, you can print objects by passing them to `console.log()`. For instance, `const obj = { name: “Alice”, age: 30 }; console.log(obj);` will display the object structure in the console.
Is there a way to format output in TypeScript?
You can format output using template literals. For example, `console.log(`Name: ${obj.name}, Age: ${obj.age}`);` allows you to create a formatted string that includes variable values.
How do I print errors in TypeScript?
To print errors, use `console.error()`. For example, `console.error(“An error occurred:”, error);` provides a clear indication of an error in the console.
Can I use third-party libraries for printing in TypeScript?
Yes, you can use libraries like `chalk` for colored console output or `winston` for more advanced logging capabilities. These can enhance the way you print messages in your applications.
How do I print to the browser console in a TypeScript web application?
In a TypeScript web application, you can use `console.log()` just as in Node.js. Ensure your TypeScript is compiled to JavaScript and run in a browser environment to see the output in the browser’s developer tools console.
In TypeScript, printing output to the console is primarily achieved using the `console.log()` method, similar to JavaScript. This method allows developers to display messages, variables, and other information for debugging and logging purposes. Additionally, TypeScript supports other console methods such as `console.error()`, `console.warn()`, and `console.info()`, which can be used to categorize the output based on severity or type.
TypeScript’s type system enhances the logging process by allowing developers to define variable types explicitly. This feature helps prevent runtime errors and improves code readability, making it easier to understand what data is being printed. Furthermore, TypeScript’s integration with modern IDEs provides features like IntelliSense, which offers suggestions and documentation as you type, facilitating a smoother coding experience when using console methods.
In summary, printing in TypeScript is straightforward and closely resembles JavaScript practices. By utilizing the various console methods available, developers can effectively debug and log information. The added benefits of TypeScript’s type system and IDE support contribute to a more robust development process, ultimately leading to higher quality code and improved maintainability.
Author Profile
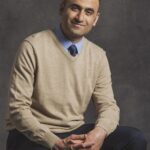
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?