How Can You Print Lists in Python Effectively?
Printing lists in Python is a fundamental skill that every programmer should master, whether you’re a beginner embarking on your coding journey or an experienced developer looking to refine your skills. Lists are one of the most versatile and widely-used data structures in Python, allowing you to store, organize, and manipulate collections of items with ease. However, knowing how to effectively display these lists can significantly enhance your ability to debug your code, present data, and communicate your findings.
In this article, we will explore various methods for printing lists in Python, from the simplest approaches to more advanced techniques that can help you format your output for clarity and effectiveness. You’ll learn how to print lists directly, customize the output using loops, and even leverage built-in functions to achieve your desired presentation. Whether you’re dealing with a small list of numbers or a complex collection of objects, understanding how to print lists will empower you to convey information more effectively and improve the readability of your code.
As we delve deeper into the topic, we’ll cover common pitfalls and best practices to ensure that your list outputs are not only accurate but also visually appealing. By the end of this article, you’ll have a comprehensive toolkit at your disposal for printing lists in Python, ready to tackle any coding challenge that comes your way. So, let’s get started and
Printing Lists Using the print() Function
The simplest way to print a list in Python is by using the built-in `print()` function. When you pass a list to `print()`, Python outputs the list as a string representation, which includes the square brackets and commas separating the elements.
“`python
my_list = [1, 2, 3, 4, 5]
print(my_list)
“`
This code will produce the following output:
“`
[1, 2, 3, 4, 5]
“`
Printing Lists with Custom Formatting
To create more aesthetically pleasing output, you might want to format the list differently. You can achieve this by converting the list elements to strings and joining them with a specified separator. The `join()` method is particularly useful for this purpose.
“`python
my_list = [‘apple’, ‘banana’, ‘cherry’]
formatted_output = ‘, ‘.join(my_list)
print(formatted_output)
“`
This will yield the following output:
“`
apple, banana, cherry
“`
Printing Lists with Indices
Sometimes, it is useful to print the indices along with the values of the list. This can be accomplished using a `for` loop that incorporates the `enumerate()` function, which provides both the index and the value of each item in the list.
“`python
my_list = [‘a’, ‘b’, ‘c’]
for index, value in enumerate(my_list):
print(f’Index {index}: {value}’)
“`
This results in:
“`
Index 0: a
Index 1: b
Index 2: c
“`
Printing Nested Lists
When dealing with nested lists (lists containing other lists), you might want to print them in a structured manner. A nested loop can be used to print each sublist on a new line.
“`python
nested_list = [[1, 2], [3, 4], [5, 6]]
for sublist in nested_list:
print(sublist)
“`
Output:
“`
[1, 2]
[3, 4]
[5, 6]
“`
Using List Comprehensions for Printing
List comprehensions can also be employed for more complex formatting. They allow for concise and readable expressions when printing or transforming lists.
“`python
my_list = [1, 2, 3, 4]
print([f’Squared: {x**2}’ for x in my_list])
“`
This would output:
“`
[‘Squared: 1’, ‘Squared: 4’, ‘Squared: 9’, ‘Squared: 16’]
“`
Printing Lists in a Tabular Format
For more complex data representation, such as printing lists in a table format, the `tabulate` library can be used. This library formats data into a readable table format.
First, install the `tabulate` library if you haven’t already:
“`bash
pip install tabulate
“`
You can then use it as follows:
“`python
from tabulate import tabulate
data = [[‘Name’, ‘Age’], [‘Alice’, 30], [‘Bob’, 25]]
print(tabulate(data, headers=’firstrow’, tablefmt=’grid’))
“`
Output:
“`
+——–+—–+
Name | Age |
---|
+——–+—–+
Alice | 30 |
---|---|
Bob | 25 |
+——–+—–+
“`
This provides a clear and structured way to present list data.
Using the print() Function
The simplest way to print a list in Python is by using the built-in `print()` function. When you pass a list to `print()`, Python will display the list in its standard format, which includes brackets and commas.
“`python
my_list = [1, 2, 3, 4, 5]
print(my_list) Output: [1, 2, 3, 4, 5]
“`
Printing List Elements Individually
If you want to print each element of a list on a separate line, you can iterate through the list using a loop. This method provides more control over formatting.
“`python
for item in my_list:
print(item)
“`
This will output:
“`
1
2
3
4
5
“`
Custom Formatting with join()
To print list elements as a single string, you can use the `join()` method. This method is particularly useful for creating a formatted string from list elements.
“`python
my_list = [‘apple’, ‘banana’, ‘cherry’]
print(“, “.join(my_list)) Output: apple, banana, cherry
“`
Be mindful that `join()` works only with strings, so ensure that all elements are of string type.
Formatted Output Using f-strings
For more advanced formatting, consider using f-strings (formatted string literals), available in Python 3.6 and later. This allows for embedding expressions inside string literals.
“`python
my_list = [1, 2, 3]
print(f”My list contains: {my_list}”) Output: My list contains: [1, 2, 3]
“`
Using List Comprehensions for Custom Output
You can leverage list comprehensions for custom formatting when printing lists. This method allows you to create formatted strings based on list elements efficiently.
“`python
my_list = [1, 2, 3]
formatted_output = [f”Item: {item}” for item in my_list]
print(“\n”.join(formatted_output))
“`
This will output:
“`
Item: 1
Item: 2
Item: 3
“`
Printing Lists in Tabular Format
For lists of lists (2D lists), the `tabulate` library can be highly effective in printing data in a tabular format. First, ensure you have the library installed:
“`bash
pip install tabulate
“`
Then, use it as follows:
“`python
from tabulate import tabulate
data = [[“Name”, “Age”], [“Alice”, 30], [“Bob”, 25]]
print(tabulate(data, headers=”firstrow”))
“`
The output will resemble:
“`
Name Age
——- —–
Alice 30
Bob 25
“`
Using Pretty Print for Complex Lists
The `pprint` module is useful for printing complex data structures in a readable format. It automatically formats output to make it more understandable.
“`python
import pprint
my_list = [{‘name’: ‘Alice’, ‘age’: 30}, {‘name’: ‘Bob’, ‘age’: 25}]
pprint.pprint(my_list)
“`
This provides a formatted output that enhances readability, especially for nested structures.
These methods provide a variety of approaches for printing lists in Python, catering to different requirements and preferences for output formatting.
Expert Insights on Printing Lists in Python
Dr. Emily Chen (Senior Python Developer, Tech Innovations Inc.). “Printing lists in Python can be accomplished using the built-in `print()` function. However, for more complex data structures, utilizing the `pprint` module enhances readability by formatting the output in a more structured manner, especially for nested lists.”
James Patel (Lead Software Engineer, CodeCraft Solutions). “When printing lists, it is essential to understand the difference between printing the list as a whole and iterating through its elements. Using a loop allows for customized formatting, which can be particularly useful when dealing with large datasets or when specific output formats are required.”
Linda Garcia (Python Educator, LearnPython Academy). “For beginners, I recommend starting with the basic `print(my_list)` to familiarize themselves with list output. As they progress, they should explore list comprehensions and the `join()` method for more advanced printing techniques that can create formatted strings from list elements.”
Frequently Asked Questions (FAQs)
How can I print a simple list in Python?
You can print a simple list in Python by using the `print()` function. For example, `my_list = [1, 2, 3]` and then `print(my_list)` will output `[1, 2, 3]`.
What is the best way to format a list when printing?
To format a list for better readability, you can use a loop or the `join()` method. For instance, `print(“, “.join(map(str, my_list)))` will print the list elements separated by commas.
How do I print the elements of a list on separate lines?
You can print each element on a separate line by using a loop. For example, `for item in my_list: print(item)` will output each element on a new line.
Can I print a list of dictionaries in Python?
Yes, you can print a list of dictionaries. You can use a loop to iterate through the list and print each dictionary. For example, `for d in my_list: print(d)` will print each dictionary in the list.
How do I pretty-print a list in Python?
To pretty-print a list, you can use the `pprint` module. Import it with `from pprint import pprint` and then call `pprint(my_list)` to display the list in a more readable format.
Is there a way to print a list with its index?
Yes, you can print a list with its index using the `enumerate()` function. For example, `for index, value in enumerate(my_list): print(index, value)` will display each index and its corresponding value.
Printing lists in Python is a straightforward process that can be accomplished using various methods. The most common approach is to use the built-in `print()` function, which outputs the entire list as a string representation. This method is particularly useful for quick debugging or displaying the contents of a list in a readable format. Additionally, Python allows for the customization of the output through string formatting techniques, enabling users to present list elements in a more structured manner.
Another effective way to print lists is by utilizing loops, such as `for` loops. This method allows for more control over the output, as it enables the printing of each element on a new line or in a specific format. Furthermore, list comprehensions can be employed to format the output dynamically, providing a powerful tool for more complex printing requirements. The use of the `join()` method also allows for the concatenation of list elements into a single string, which can then be printed in a user-defined format.
In summary, Python offers multiple techniques for printing lists, each suited to different scenarios and requirements. Understanding these methods enhances a programmer’s ability to effectively communicate data and improve code readability. By leveraging the built-in functionalities and customizing the output, users can ensure that their printed lists meet
Author Profile
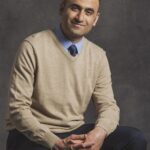
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?