How Can You Print on the Same Line in Python?
In the world of programming, the ability to control output is a vital skill that can enhance the clarity and effectiveness of your code. Python, known for its simplicity and readability, offers various ways to manipulate how information is displayed. One common requirement among developers is the need to print multiple items on the same line, a task that can seem straightforward but often requires a deeper understanding of Python’s print functionality. Whether you’re crafting a user interface, logging data, or simply trying to present information in a more organized manner, mastering this technique can elevate your coding prowess.
When working with Python, the default behavior of the `print()` function is to output each call on a new line. However, there are several methods to modify this behavior, allowing you to print on the same line. Understanding these methods not only improves your coding efficiency but also enhances the user experience by providing more cohesive outputs. From using the `end` parameter to leveraging string concatenation, there are various approaches that can be tailored to fit different scenarios and preferences.
As we delve deeper into this topic, you’ll discover practical examples and best practices for printing on the same line in Python. Whether you’re a beginner looking to grasp the fundamentals or an experienced programmer seeking to refine your skills, this guide will equip you with the knowledge needed
Using the Print Function in Python
In Python, the `print()` function is versatile and allows for printing output in various formats. To print on the same line, you can utilize the `end` parameter, which specifies what to print at the end of the output. By default, the `print()` function ends with a newline character (`\n`), but you can change this behavior.
To print multiple items on the same line, specify `end=”` in your print statements:
python
print(“Hello”, end=’ ‘)
print(“World!”)
This code will output:
Hello World!
Using Commas in Print Statements
When printing multiple items, you can also separate them using commas. This automatically adds a space between the items:
python
print(“Hello”, “World!”)
Output will be:
Hello World!
However, if you want to customize the separator, you can use the `sep` parameter:
python
print(“Hello”, “World!”, sep=’, ‘)
This will yield:
Hello, World!
Printing in Loops
When dealing with loops, printing on the same line can be quite useful. Here is an example using a `for` loop:
python
for i in range(5):
print(i, end=’ ‘)
This will produce the output:
0 1 2 3 4
If you want to add a delay between prints, you can use the `time` module:
python
import time
for i in range(5):
print(i, end=’ ‘, flush=True)
time.sleep(1)
This will print each number with a one-second pause in between.
Using String Formatting
String formatting can also be used to control how output is displayed on the same line. For example, using f-strings (available in Python 3.6 and later):
python
name = “Alice”
age = 30
print(f”{name} is {age}”, end=’ years old.’)
This will output:
Alice is 30 years old.
Example Table of Print Options
The following table summarizes various ways to use the `print()` function to control output formatting:
Method | Description | Example |
---|---|---|
Default | Prints with newline | print(“Hello”) |
End Parameter | Prints on the same line | print(“Hello”, end=’ ‘) |
Sep Parameter | Custom separator for multiple items | print(“Hello”, “World”, sep=’, ‘) |
String Formatting | Formatted output | print(f”{name} is {age}”) |
By understanding these techniques, you can effectively control how output is displayed in your Python programs, enhancing readability and user experience.
Using the `print()` Function with `end` Parameter
In Python, the default behavior of the `print()` function is to end with a newline character (`\n`). To print on the same line, you can modify this behavior using the `end` parameter. By setting `end` to an empty string or a different character, you can control what is printed at the end of the output.
python
print(“Hello”, end=’ ‘) # Prints “Hello” without a newline
print(“World!”) # Prints “World!” on the same line
In this example, the output will be:
Hello World!
Using a Comma in Python 2.x
For those using Python 2.x, a trailing comma at the end of a `print` statement can achieve similar functionality. However, this method is not recommended for new projects since Python 2 is no longer supported.
python
print “Hello”,
print “World!”
This will produce:
Hello World!
Using String Concatenation
Another approach is to concatenate strings before printing. This method allows for more control over formatting.
python
output = “Hello” + ” ” + “World!”
print(output)
This will yield the same result:
Hello World!
Using Formatted Strings
Formatted strings offer a modern and versatile way to construct strings. With f-strings (available in Python 3.6 and later), you can include variables directly in the string.
python
name = “World”
print(f”Hello {name}”, end=’!’)
The output will be:
Hello World!
Creating a Loop for Continuous Output
When printing multiple items in a loop, you can still apply the `end` parameter effectively to maintain output on the same line.
python
for i in range(5):
print(i, end=’ ‘) # Prints numbers on the same line
The output will be:
0 1 2 3 4
Using the `flush` Parameter for Immediate Output
In scenarios where buffering might delay output, the `flush` parameter ensures that the output is written immediately to the console.
python
import time
for i in range(5):
print(i, end=’ ‘, flush=True)
time.sleep(1) # Simulates a delay
This will print numbers one by one on the same line, with a one-second interval between each.
Handling Multiple Outputs with Separator
The `print()` function also has a `sep` parameter that allows you to specify a string that separates multiple arguments. This can be useful when you want to format the output neatly.
python
print(“Hello”, “World”, sep=’, ‘)
This will result in:
Hello, World
These methods provide a range of options for printing on the same line in Python, allowing for flexibility in formatting and output control.
Expert Insights on Printing in Python
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “To print on the same line in Python, one can utilize the `end` parameter of the `print()` function. By default, `print()` ends with a newline character, but setting `end=”` allows for continuous output on the same line.”
Michael Chen (Python Developer, Tech Innovations Inc.). “Using the `flush` parameter alongside `end` can be particularly useful in scenarios where immediate output is necessary, such as in real-time applications. This ensures that the output is displayed without buffering delays.”
Lisa Patel (Data Scientist, Analytics Hub). “For more complex scenarios where formatted output is required, consider using formatted strings or f-strings in conjunction with the `print()` function. This allows for a clean and readable way to output data on the same line.”
Frequently Asked Questions (FAQs)
How can I print multiple values on the same line in Python?
You can print multiple values on the same line by using the `print()` function with the `end` parameter set to an empty string or a space. For example, `print(“Hello”, end=” “)` will print “Hello” and keep the cursor on the same line.
What is the default behavior of the print function in Python?
By default, the `print()` function in Python ends with a newline character, which means each call to `print()` will start on a new line unless specified otherwise.
Can I use the `sep` parameter in the print function to control output formatting?
Yes, the `sep` parameter allows you to specify a string that separates the values printed by the `print()` function. For example, `print(“Hello”, “World”, sep=”, “)` will output “Hello, World”.
How do I print a list on the same line in Python?
You can print a list on the same line by unpacking it with the `*` operator in the `print()` function. For example, `print(*my_list)` will print all elements of `my_list` on the same line, separated by spaces.
Is there a way to overwrite the previous output in the same line?
Yes, you can overwrite the previous output by using the carriage return character `\r`. For example, `print(“Hello”, end=”\r”)` will move the cursor back to the start of the line, allowing you to print new content over the existing text.
What are some common use cases for printing on the same line in Python?
Common use cases include displaying progress indicators, creating dynamic command-line interfaces, and formatting output for better readability without cluttering the console with multiple lines.
In Python, printing on the same line can be achieved using the `print()` function with specific parameters. By default, the `print()` function ends with a newline character, which causes subsequent prints to appear on new lines. To override this behavior, the `end` parameter of the `print()` function can be set to an empty string or any other desired string, allowing multiple outputs to be displayed consecutively on the same line.
Another method to print on the same line involves using the `sys.stdout.write()` function from the `sys` module. This function does not automatically append a newline character, providing more control over the output format. However, it is essential to manually manage the output by including line breaks when necessary. Both methods are useful depending on the context and the specific requirements of the output format.
In summary, understanding how to print on the same line in Python enhances the ability to format output effectively. By utilizing the `end` parameter in the `print()` function or employing `sys.stdout.write()`, developers can create more dynamic and visually appealing console outputs. Mastery of these techniques is beneficial for improving user interaction and readability in Python applications.
Author Profile
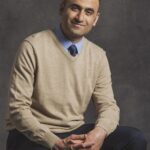
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?