How Can You Print Parentheses in Python?
In the world of programming, even the simplest tasks can sometimes lead to unexpected challenges. One such challenge that often perplexes beginners is the art of printing parentheses in Python. While it may seem trivial at first glance, understanding how to manipulate and display these symbols can open the door to more complex coding scenarios. Whether you’re crafting a mathematical expression, formatting output for readability, or simply trying to debug your code, mastering the use of parentheses is a fundamental skill every Python programmer should possess.
Printing parentheses in Python involves more than just typing the characters; it requires an understanding of how strings and escape sequences function within the language. As you delve deeper into this topic, you’ll discover the various methods available for achieving this task, whether through straightforward print statements or more advanced techniques. Additionally, you’ll learn how to navigate the nuances of special characters and formatting options that can enhance the clarity and presentation of your output.
As you explore the intricacies of printing parentheses, you’ll gain insights into the broader principles of string manipulation and output formatting in Python. This knowledge not only empowers you to tackle similar challenges with confidence but also enriches your overall programming toolkit. So, let’s embark on this journey to demystify the seemingly simple yet essential task of printing parentheses in Python!
Using Print Statements
To print parentheses in Python, you can utilize the `print()` function directly. The parentheses can be included as part of the string that you want to output. For example, to print a simple message with parentheses, you can write:
“`python
print(“This is a message with parentheses: ()”)
“`
This will result in the output:
“`
This is a message with parentheses: ()
“`
If you want to print a single parenthesis, you can also include it in the string:
“`python
print(“(“)
print(“)”)
“`
Each of these commands will print the respective parenthesis on a new line.
Using Escape Characters
In some cases, you might want to print parentheses in a context where they could be interpreted as part of the syntax. For this, escape characters are useful. However, in Python, parentheses do not require escaping when used in strings. If needed, you can still use escape sequences for other special characters. Here’s how to print parentheses normally:
“`python
print(“Here are some parentheses: ( )”)
“`
This will work seamlessly without needing to escape the parentheses.
Printing Nested Parentheses
When dealing with nested structures or if you want to represent mathematical expressions, you might want to print multiple sets of parentheses. You can do this by simply combining strings:
“`python
print(“Nested parentheses: ((a + b) * (c – d))”)
“`
This will output:
“`
Nested parentheses: ((a + b) * (c – d))
“`
Using Formatted Strings
You can also use formatted strings (f-strings) to include variables within parentheses. This is particularly useful for dynamic content:
“`python
a = 5
b = 10
print(f”The result of the operation is: ({a} + {b})”)
“`
The output will be:
“`
The result of the operation is: (5 + 10)
“`
Table of Print Examples
The following table summarizes various methods of printing parentheses in Python:
Method | Code Example | Output |
---|---|---|
Simple Print | print(“()”) | () |
Single Parenthesis | print(“(“) | ( |
Nested Parentheses | print(“((a + b) * (c – d))”) | ((a + b) * (c – d)) |
Formatted String | print(f”({a} + {b})”) | (5 + 10) |
By understanding these methods, you can effectively print parentheses in Python across different contexts and requirements.
Printing Parentheses in Python
In Python, printing parentheses is straightforward. You can achieve this by including them directly in the print function. Here are the primary methods to print parentheses:
Using the Print Function
The simplest method is to use the `print()` function and include the parentheses as part of the string. For instance:
“`python
print(“()”) This will output: ()
“`
If you want to print parentheses without quotes, you can simply do:
“`python
print(“(“, “)”, sep=””) This will output: ()
“`
This approach utilizes the `sep` parameter of the `print` function to control the separator between multiple arguments.
Using Escape Characters
In scenarios where you need to include special characters in strings, you may want to use escape characters. However, parentheses do not require escaping in standard string literals. For example:
“`python
print(“This is a parentheses: ()”) This will output: This is a parentheses: ()
“`
For more complex cases, where parentheses are part of a formatted string, you can still include them directly.
Printing Nested Parentheses
If you want to print nested parentheses, you can do so by including them directly within the string:
“`python
print(“( ( ) )”) This will output: ( ( ) )
“`
Alternatively, you can create a more structured output using formatted strings:
“`python
a = “(”
b = “)”
print(f”{a} {b}”) This will output: ( )
“`
Using String Formatting
Python supports various string formatting methods, including f-strings, `str.format()`, and the percentage operator. Here’s how to print parentheses using these methods:
- F-Strings (Python 3.6+):
“`python
value = “test”
print(f”({value})”) This will output: (test)
“`
- str.format():
“`python
value = “test”
print(“({})”.format(value)) This will output: (test)
“`
- Percentage Operator:
“`python
value = “test”
print(“(%s)” % value) This will output: (test)
“`
Printing Parentheses in Loops
When printing parentheses in a loop, you can concatenate strings or use formatted output. Here’s an example:
“`python
for i in range(3):
print(f”({i})”) This will output: (0), (1), (2)
“`
Alternatively, using a list comprehension:
“`python
print(” “.join(f”({i})” for i in range(3))) This will output: (0) (1) (2)
“`
Printing parentheses in Python can be accomplished through various methods, depending on the context and requirements. Whether using basic string literals, string formatting, or loops, Python provides flexibility for effectively displaying parentheses in your output.
Expert Insights on Printing Parentheses in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, printing parentheses can be done simply by using the print function. For instance, to print the string ‘(Hello, World!)’, you should encapsulate it in quotes within the print statement: print(‘(Hello, World!)’). This approach ensures that the parentheses are treated as part of the string rather than as a grouping operator.”
Michael Thompson (Lead Software Engineer, CodeCraft Solutions). “When printing parentheses in Python, it is crucial to remember that they can also be used to denote tuples. To avoid confusion, always use quotes around the parentheses when you intend to print them as characters. For example, print(‘()’) will correctly display the parentheses on the console.”
Sarah Johnson (Python Programming Instructor, LearnCode Academy). “For beginners, understanding how to print parentheses is a foundational skill. I often advise my students to experiment with the print function using various string formats. For instance, using formatted strings like f'({value})’ can be particularly useful when embedding variables within parentheses.”
Frequently Asked Questions (FAQs)
How do I print parentheses in Python?
To print parentheses in Python, you can simply include them in a string. For example, use `print(“()”)` to display parentheses.
Can I print parentheses without using quotes?
No, you cannot print parentheses without using quotes. Parentheses need to be part of a string or a character to be displayed.
What if I want to print parentheses with other characters?
You can concatenate parentheses with other characters in a string. For instance, `print(“Hello (World)”)` will print “Hello (World)”.
Is there a way to format strings that include parentheses?
Yes, you can use formatted strings (f-strings) to include parentheses. For example, `name = “Alice”; print(f”Hello ({name})”)` will output “Hello (Alice)”.
Can I escape parentheses in Python when printing?
Escaping parentheses is not necessary when printing, as they do not have special meaning in strings. You can print them directly without escaping.
What happens if I try to print parentheses without a string?
If you attempt to print parentheses without wrapping them in a string, Python will raise a `SyntaxError` because it expects an expression or a valid statement.
In Python, printing parentheses is a straightforward task. When using the print function, parentheses are treated as part of the syntax for function calls. To print actual parentheses as characters, they should be included within the string that is passed to the print function. This can be achieved by enclosing the parentheses within single or double quotes, allowing them to be displayed as part of the output.
Additionally, it is important to note that if you need to include parentheses in a formatted string, you can do so by using f-strings or the format method. Both approaches allow for dynamic content to be inserted into strings while maintaining the ability to include parentheses. This flexibility is particularly useful when constructing output that requires both textual and numerical data.
In summary, printing parentheses in Python is easily accomplished by including them in strings passed to the print function. Understanding how to format strings effectively enhances the ability to create clear and informative outputs, making it a valuable skill for any Python programmer.
Author Profile
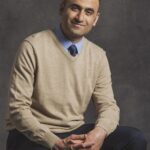
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?