How Can You Print Quotation Marks in Python?
In the world of programming, even the simplest tasks can sometimes lead to unexpected challenges, especially when it comes to handling strings. One common hurdle that many Python newcomers encounter is the correct way to print quotation marks within a string. While it may seem trivial, mastering this skill is essential for effective communication in your code, whether you’re crafting user-friendly messages or debugging your output. In this article, we’ll explore the various methods to achieve this, ensuring you can confidently include quotation marks in your Python strings without a hitch.
Understanding how to manipulate strings in Python is a foundational skill that opens the door to more complex programming concepts. Quotation marks, both single and double, serve as delimiters for string literals, but when you want to include them as part of the output, things can get a bit tricky. Fortunately, Python offers several straightforward techniques to help you seamlessly integrate quotation marks into your printed strings, allowing you to enhance the clarity and functionality of your code.
From using escape characters to leveraging different types of quotes, there are multiple approaches to printing quotation marks that cater to various coding styles and preferences. Whether you’re creating a simple script or working on a larger project, knowing how to effectively include quotation marks will not only improve your coding efficiency but also elevate the readability of your output.
Using Escape Characters
In Python, one of the most straightforward methods to print quotation marks is by using escape characters. An escape character allows you to include special characters in strings. The backslash (`\`) is used as an escape character in Python. To print a quotation mark, you simply precede it with a backslash.
For example:
“`python
print(“He said, \”Hello!\””)
“`
This code snippet will output:
“`
He said, “Hello!”
“`
Here, the backslashes before the quotation marks tell Python to treat them as literal characters rather than string delimiters.
Using Single Quotes to Enclose Double Quotes
Another method involves using single quotes to enclose a string that contains double quotes. This approach avoids the need for escape characters entirely.
Example:
“`python
print(‘He said, “Hello!”‘)
“`
The output will be the same:
“`
He said, “Hello!”
“`
This technique can be particularly useful for maintaining readability in code, especially when dealing with longer strings that require quotation marks.
Using Triple Quotes
Python also supports triple quotes, which can be either three single quotes or three double quotes. This method allows you to include both single and double quotes within the string without escaping them.
Example:
“`python
print(“””He said, “It’s a beautiful day!” “””)
“`
The output will be:
“`
He said, “It’s a beautiful day!”
“`
This method is particularly beneficial for strings that span multiple lines or contain complex formatting.
Comparison of Methods
Here’s a comparative overview of the different methods for printing quotation marks in Python:
Method | Example | Output |
---|---|---|
Escape Characters | print(“He said, \”Hello!\””) | He said, “Hello!” |
Single Quotes | print(‘He said, “Hello!”‘) | He said, “Hello!” |
Triple Quotes | print(“””He said, “It’s a beautiful day!” “””) | He said, “It’s a beautiful day!” |
Each method has its advantages, and the choice of which to use can depend on personal preference, readability, and the specific requirements of the string being printed.
Printing Quotation Marks in Python
In Python, printing quotation marks can be achieved through various methods, depending on the context in which you want to include them. Here are the primary techniques:
Using Escape Characters
One of the most straightforward methods to print quotation marks is by using escape characters. An escape character allows you to insert special characters into strings.
- Single Quotation Marks: Use a backslash `\` before the quotation mark.
“`python
print(‘He said, \”Hello!\”‘)
“`
- Double Quotation Marks: Similarly, you can escape double quotes within a string.
“`python
print(“She replied, ‘Goodbye!'”)
“`
The output for these examples will be:
- `He said, “Hello!”`
- `She replied, ‘Goodbye!’`
Using Different Quote Types
Another method involves using different types of quotes to avoid the need for escape characters. If your string is enclosed in single quotes, you can include double quotes without escaping, and vice versa.
- Example with Single Quotes:
“`python
print(‘He said, “Hello!”‘)
“`
- Example with Double Quotes:
“`python
print(“It’s a sunny day.”)
“`
The outputs will be:
- `He said, “Hello!”`
- `It’s a sunny day.`
Using Triple Quotes
For multi-line strings or to include both single and double quotes without escaping, triple quotes can be used. Triple quotes can be either single (`”’`) or double (`”””`).
- Example:
“`python
print(“””He said, “It’s a beautiful day!” “””)
“`
This will output:
- `He said, “It’s a beautiful day!”`
Summary of Methods
Method | Description | Example |
---|---|---|
Escape Characters | Use `\` to escape quotes. | `print(‘He said, \”Hello!\”‘)` |
Different Quote Types | Use single quotes for double quotes and vice versa. | `print(‘He said, “Hello!”‘)` |
Triple Quotes | Use for multi-line or mixed quotes. | `print(“””He said, “It’s sunny.”””`) |
These methods provide flexibility in how you can include quotation marks in your Python strings, allowing you to choose the best approach for your specific scenario.
Expert Insights on Printing Quotation Marks in Python
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “In Python, printing quotation marks can be achieved using escape characters. For instance, using a backslash before the quotation mark allows you to include it within a string without causing syntax errors.”
Michael Chen (Python Developer, Tech Innovations Inc.). “To print quotation marks in Python, one can also use single quotes to wrap a string containing double quotes, or vice versa. This flexibility simplifies the process and enhances code readability.”
Sarah Thompson (Programming Instructor, LearnPython Academy). “Understanding how to print quotation marks is fundamental for beginners. Utilizing triple quotes can also be beneficial, as they allow for multi-line strings while including both single and double quotes seamlessly.”
Frequently Asked Questions (FAQs)
How do I print double quotation marks in Python?
To print double quotation marks in Python, you can use the escape character `\`. For example, `print(“He said, \”Hello!\””)` will output: He said, “Hello!”.
How can I print single quotation marks in Python?
To print single quotation marks, you can also use the escape character. For instance, `print(‘It\’s a sunny day.’)` will display: It’s a sunny day.
Is there a way to print both single and double quotation marks?
Yes, you can print both by using triple quotes or by escaping them. For example, `print(‘He said, “It\’s a sunny day.”‘)` or `print(“””He said, “It’s a sunny day.”””`) will work.
What is the difference between using single and double quotes in Python?
There is no functional difference between single and double quotes in Python; both can be used interchangeably for string literals. The choice often depends on the need to include quotes within the string.
Can I use triple quotes to include quotation marks in my string?
Yes, triple quotes allow for multi-line strings and can include both single and double quotation marks without the need for escaping. For example, `print(“””He said, “It’s a sunny day.”””`) will work seamlessly.
What happens if I forget to escape quotation marks?
If you forget to escape quotation marks, Python will raise a `SyntaxError` because it will misinterpret the end of the string. Always ensure proper escaping or use different types of quotes to avoid this issue.
In Python, printing quotation marks can be achieved through several methods, allowing for flexibility depending on the context of the string. The most common approaches include using escape characters, utilizing different types of quotation marks, or employing triple quotes. Each method serves to ensure that the quotation marks are interpreted correctly by the Python interpreter, thus preventing syntax errors.
One effective method is to use the backslash escape character (\\) before the quotation marks within a string. For instance, to print double quotes, one can write: `print(“He said, \”Hello!\””)`. Alternatively, using single quotes to enclose a string that contains double quotes eliminates the need for escape characters, as in `print(‘He said, “Hello!”‘)`. This versatility allows developers to choose the most readable option based on their specific needs.
Furthermore, triple quotes (either triple single or triple double quotes) can be employed to include both types of quotation marks without the need for escaping. For example, `print(“””He said, “It’s a great day!” “””)` effectively incorporates both double and single quotes seamlessly. Understanding these techniques is essential for anyone working with strings in Python, as it enhances code clarity and functionality.
Author Profile
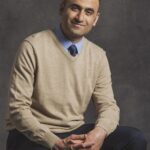
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?