How Can You Print Quotes in Python?
In the world of programming, the ability to effectively communicate with both the computer and fellow developers is paramount. One of the fundamental skills every Python programmer must master is the art of printing quotes. Whether you’re crafting a simple message, displaying a user prompt, or debugging your code, understanding how to handle quotes in Python is essential. This seemingly straightforward task can sometimes lead to confusion, especially for beginners. In this article, we will delve into the nuances of printing quotes in Python, equipping you with the knowledge to enhance your coding experience.
When it comes to printing quotes in Python, there are several methods and techniques that can be employed to ensure your strings are formatted correctly. Python provides flexibility in how you can encapsulate your text, allowing for single quotes, double quotes, and even triple quotes. Each option has its own advantages, particularly when it comes to including quotes within your strings without causing syntax errors.
Furthermore, understanding how to escape characters and utilize raw strings will empower you to create more complex outputs and handle special cases with ease. As we explore this topic, you’ll discover not only the mechanics of printing quotes but also best practices that can elevate your coding skills. Whether you’re a novice eager to learn or an experienced coder looking to refresh your knowledge, mastering this
Using Single and Double Quotes
In Python, you can print quotes by enclosing them in either single quotes (`’`) or double quotes (`”`). This flexibility allows you to include quotes within quotes without needing escape characters. For example:
“`python
print(“He said, ‘Hello, World!'”)
print(‘She replied, “Goodbye, World!”‘)
“`
Both lines will correctly print the respective strings, showcasing the use of different types of quotes.
Escaping Quotes
When you need to include the same type of quote within a string, Python requires you to escape the inner quotes using a backslash (`\`). This ensures that the interpreter recognizes the quotes as part of the string rather than as string delimiters. For instance:
“`python
print(“He said, \”Hello, World!\””)
print(‘It\’s a beautiful day!’)
“`
In the above examples, the backslashes allow the quotes to be included within the strings.
Triple Quotes for Multi-line Strings
Python also supports triple quotes, either `”’` or `”””`, which are particularly useful for multi-line strings and preserving formatting. This feature is advantageous when you want to include both single and double quotes without escaping them. For example:
“`python
print(“””This is a string that can include ‘single’ and “double” quotes
without any issues.”””)
“`
This method enables a cleaner and more readable code structure for long strings.
Printing Quotes with the `repr()` Function
Another method for printing quotes is by using the `repr()` function, which returns a string representation of an object. This function can be particularly useful for debugging, as it shows the string with quotes included. For example:
“`python
quote = “Python is fun!”
print(repr(quote))
“`
This will output:
“`
‘Python is fun!’
“`
Formatting Strings with f-strings
Python’s f-strings (formatted string literals) provide a way to embed expressions inside string literals while also allowing for the inclusion of quotes. For example:
“`python
name = “Alice”
print(f”{name} said, ‘Hello!'”)
“`
This results in:
“`
Alice said, ‘Hello!’
“`
Table of Quote Usage
Quote Type | Example | Usage |
---|---|---|
Single Quotes | ‘Hello’ | Used for simple strings without needing escape characters. |
Double Quotes | “Hello” | Useful when the string contains single quotes. |
Triple Single Quotes | ”’Hello”’ | Ideal for multi-line strings. |
Triple Double Quotes | “””Hello””” | Also for multi-line strings, allowing both single and double quotes. |
Printing Strings with Quotes in Python
To print strings that contain quotes in Python, you have several methods at your disposal. Each method allows you to include quotes without causing syntax errors. Below are the most common techniques:
Using Different Quote Types
You can use single quotes (`’`) to enclose a string that contains double quotes (`”`), and vice versa. This is a straightforward approach.
“`python
print(“He said, ‘Hello, World!'”)
print(‘She replied, “Goodbye!”‘)
“`
Escaping Quotes
If you want to use the same type of quotes for both the string and the content, you can escape the inner quotes using a backslash (`\`). This tells Python to treat the quote as a literal character.
“`python
print(“He said, \”Hello, World!\””)
print(‘It\’s a beautiful day.’)
“`
Using Triple Quotes
Triple quotes (`”’` or `”””`) can also be utilized, allowing you to include both single and double quotes without escaping them. This is particularly useful for multi-line strings.
“`python
print(“””He said, “It’s a beautiful day!” “””)
print(”’She replied, “Indeed, it is!””’)
“`
Printing Quotes from Variables
If you have strings stored in variables that contain quotes, you can print them directly without any additional formatting.
“`python
quote1 = ‘To be or not to be.’
quote2 = “That’s the question.”
print(quote1)
print(quote2)
“`
Using f-Strings for Dynamic Content
In Python 3.6 and later, f-strings offer a convenient way to embed expressions inside string literals. They can also include quotes seamlessly.
“`python
name = “Alice”
print(f”{name} said, ‘Hello!'”)
“`
Summary of Techniques
Technique | Example |
---|---|
Different Quote Types | `print(“He said, ‘Hello!'”)` |
Escaping Quotes | `print(“He said, \”Hello!\””)` |
Triple Quotes | `print(“””He said, “It’s a beautiful day!” “””)` |
Variables | `quote = “That’s interesting.”` |
f-Strings | `name = “Bob”; print(f”{name} said, ‘Hi!'”)` |
These methods provide flexibility and clarity when printing strings with quotes in Python, ensuring you can handle various scenarios effectively.
Expert Insights on Printing Quotes in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Institute). “Printing quotes in Python is a fundamental skill for any programmer. Utilizing the print function with either single or double quotes allows for flexibility in handling strings, especially when dealing with quotes within quotes.”
Michael Chen (Lead Python Instructor, Code Academy). “Understanding how to print quotes in Python not only enhances your coding capabilities but also improves your ability to format output effectively. Mastering escape characters can help you include quotes in your strings without syntax errors.”
Jessica Liu (Data Scientist, Tech Innovations Corp). “In data visualization and reporting, printing quotes correctly in Python can significantly impact readability. It is essential to know the difference between raw strings and formatted strings to ensure accurate representation of data.”
Frequently Asked Questions (FAQs)
How do I print a string that contains quotes in Python?
You can print a string containing quotes by using escape characters. For example, to print a string with double quotes, use `print(“He said, \”Hello!\””)`. For single quotes, use `print(‘It\’s a sunny day.’)`.
Can I use triple quotes to print strings with quotes in Python?
Yes, triple quotes allow you to include both single and double quotes without escaping them. For example, `print(“””He said, “It’s a sunny day!” “””)` will print the string as intended.
What is the difference between single and double quotes in Python?
In Python, both single (`’`) and double (`”`) quotes can be used interchangeably for strings. The choice depends on personal preference or the need to include one type of quote within the string without escaping.
How do I print a quote character itself in Python?
To print a quote character, you can escape it using a backslash. For example, to print a double quote, use `print(“\””)`, and for a single quote, use `print(“\'”)`.
Is there a way to format strings with quotes in Python?
Yes, you can use formatted string literals (f-strings) to include quotes. For example, `name = “Alice”; print(f”{name} said, ‘Hello!'”)` will format the string correctly with quotes.
Can I use the `repr()` function to print strings with quotes?
Yes, the `repr()` function returns a string representation of an object, including quotes. For example, `print(repr(“Hello, World!”))` will output `”Hello, World!”` with quotes included.
Printing quotes in Python is a fundamental task that can be accomplished using various methods. The most straightforward approach involves using the print function along with either single quotes, double quotes, or triple quotes. Each of these options allows for flexibility in how strings are defined and displayed, making it easy to include quotes within strings without causing syntax errors.
When using single or double quotes, it is essential to ensure that the quotes used to define the string do not conflict with those included within the string itself. For example, if a string contains a single quote, it should be enclosed in double quotes, and vice versa. Alternatively, escape characters can be employed to denote quotes within the same type of quotes, providing another layer of flexibility in string formatting.
Additionally, triple quotes are particularly useful for multi-line strings or when quotes are needed within the string without the need for escaping. This feature enhances readability and allows for more complex string representations, such as including quotations from other sources directly in the code. Understanding these various methods equips programmers with the tools necessary to handle strings effectively in Python.
Author Profile
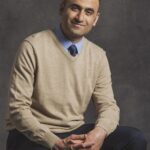
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?