How Can You Print the Keys of a Dictionary in Python?
In the world of Python programming, dictionaries are one of the most versatile and powerful data structures available. They allow you to store data in key-value pairs, making it easy to access and manipulate information efficiently. However, as you dive deeper into your coding journey, you may find yourself needing to extract just the keys from these dictionaries. Whether you’re analyzing data, building applications, or simply trying to understand how to navigate Python’s robust features, knowing how to print the keys of a dictionary is a fundamental skill that can enhance your programming toolkit.
Printing the keys of a dictionary in Python is a straightforward task, yet it opens the door to a myriad of possibilities. Understanding how to access and display these keys allows you to interact with your data more effectively, whether you’re debugging your code or generating reports. This skill is particularly useful when dealing with large datasets or when you need to iterate through the keys for further processing.
In this article, we will explore various methods to print the keys of a dictionary in Python, highlighting the simplicity and efficiency of each approach. From basic techniques to more advanced functionalities, you’ll gain insights that will empower you to manipulate dictionaries with confidence and ease. So, let’s embark on this journey to unlock the secrets of dictionary keys in Python!
Accessing Dictionary Keys
In Python, dictionaries are versatile data structures that store key-value pairs. To print the keys of a dictionary, you can utilize several built-in methods that provide different options for outputting the keys.
The most straightforward method is using the `keys()` method, which returns a view object displaying a list of all the keys in the dictionary. This can be easily printed to the console.
Here’s how to do it:
“`python
my_dict = {‘name’: ‘Alice’, ‘age’: 30, ‘city’: ‘New York’}
print(my_dict.keys())
“`
This will output:
“`
dict_keys([‘name’, ‘age’, ‘city’])
“`
To convert the keys into a list format for more conventional display, you can wrap the `keys()` method with the `list()` function:
“`python
print(list(my_dict.keys()))
“`
This results in a more familiar list format:
“`
[‘name’, ‘age’, ‘city’]
“`
Using a Loop to Print Keys
Another effective method to print dictionary keys is to iterate through the dictionary using a `for` loop. This approach can be beneficial if you need to apply additional logic during the printing process.
Example:
“`python
for key in my_dict:
print(key)
“`
This will output:
“`
name
age
city
“`
Formatting Key Output
When displaying keys, you may want to format them for better readability. This can be done by applying string formatting or using formatted output.
For example, you can print each key on a new line with a prefix:
“`python
for key in my_dict.keys():
print(f’Key: {key}’)
“`
This would produce:
“`
Key: name
Key: age
Key: city
“`
Table of Methods to Print Keys
The following table summarizes the methods available for printing dictionary keys in Python:
Method | Description | Example Output |
---|---|---|
keys() | Returns a view object of the keys. | dict_keys([‘name’, ‘age’, ‘city’]) |
list(keys()) | Converts the keys view to a list. | [‘name’, ‘age’, ‘city’] |
for loop | Iterates through keys for customized output. | name age city |
By utilizing these methods, you can effectively print and manipulate the keys of a dictionary, allowing for greater flexibility in your Python programming endeavors.
Accessing Dictionary Keys
To print the keys of a dictionary in Python, you can utilize several methods. Each method has its own syntax and advantages depending on the context in which you are working.
Using the `keys()` Method
The `keys()` method of a dictionary returns a view object that displays a list of all the keys in the dictionary. This is the most straightforward method.
“`python
my_dict = {‘a’: 1, ‘b’: 2, ‘c’: 3}
print(my_dict.keys())
“`
Output:
“`
dict_keys([‘a’, ‘b’, ‘c’])
“`
To convert this view object into a list, you can wrap it with the `list()` function:
“`python
print(list(my_dict.keys()))
“`
Output:
“`
[‘a’, ‘b’, ‘c’]
“`
Iterating Over Keys
You can also iterate over the keys of a dictionary using a for loop, which allows you to process each key individually.
“`python
for key in my_dict:
print(key)
“`
Output:
“`
a
b
c
“`
This method is particularly useful when you need to perform operations on each key.
Using List Comprehension
For more concise code, list comprehension can be employed to create a list of keys.
“`python
keys_list = [key for key in my_dict]
print(keys_list)
“`
Output:
“`
[‘a’, ‘b’, ‘c’]
“`
This approach is efficient and readable, especially when combined with other operations.
Printing Keys with Their Corresponding Values
If you want to print keys alongside their respective values, you can use the `items()` method, which returns key-value pairs.
“`python
for key, value in my_dict.items():
print(f’Key: {key}, Value: {value}’)
“`
Output:
“`
Key: a, Value: 1
Key: b, Value: 2
Key: c, Value: 3
“`
This method provides a comprehensive view of the dictionary’s contents.
Summary of Methods
Here’s a quick reference table summarizing the methods discussed:
Method | Description | Example Code |
---|---|---|
`keys()` | Returns a view of keys | `my_dict.keys()` |
Iteration | Loops through keys | `for key in my_dict: print(key)` |
List Comprehension | Creates a list of keys | `[key for key in my_dict]` |
`items()` | Returns key-value pairs | `for key, value in my_dict.items(): …` |
Each of these methods allows for flexibility in accessing and printing keys from a dictionary in Python. Choose the one that best suits your needs based on the specific task at hand.
Expert Insights on Printing Dictionary Keys in Python
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “Printing the keys of a dictionary in Python is straightforward. Utilizing the built-in `keys()` method is the most efficient approach, as it directly retrieves the keys without the need for additional loops or iterations.”
Michael Thompson (Python Developer, Tech Innovations Inc.). “For developers looking to print dictionary keys, leveraging list comprehensions can enhance readability. This method not only prints keys but also allows for easy modification of the output format, making it a versatile choice.”
Sarah Jenkins (Data Scientist, Analytics Hub). “In data-centric applications, it is essential to print dictionary keys effectively. Using the `for` loop in conjunction with the `keys()` method provides clarity and control, especially when dealing with large datasets where key manipulation is necessary.”
Frequently Asked Questions (FAQs)
How can I print all keys of a dictionary in Python?
You can print all keys of a dictionary using the `keys()` method. For example, `print(my_dict.keys())` will display all the keys.
Is there a way to print keys in a specific order?
Yes, you can print keys in a specific order by using the `sorted()` function. For instance, `print(sorted(my_dict.keys()))` will print the keys in ascending order.
Can I print keys with their corresponding values?
Yes, you can use a loop to print keys along with their values. For example:
“`python
for key, value in my_dict.items():
print(key, value)
“`
What if I want to print keys that meet a certain condition?
You can use a list comprehension or a loop with an `if` statement to filter keys. For example:
“`python
print([key for key in my_dict if my_dict[key] > 10])
“`
How do I print keys from a nested dictionary?
You can access keys from a nested dictionary by iterating through each level. For example:
“`python
for key in my_dict:
print(key)
for sub_key in my_dict[key]:
print(sub_key)
“`
Is there a way to print keys without using a loop?
Yes, you can use the `join()` method to print keys as a single string. For example:
“`python
print(‘, ‘.join(my_dict.keys()))
“`
In Python, printing the keys of a dictionary is a straightforward process that can be accomplished using several methods. The most common approach is to utilize the built-in `keys()` method, which returns a view object displaying a list of all the keys in the dictionary. This method is efficient and allows for easy iteration over the keys when combined with loops or list comprehensions.
Another effective way to print the keys is by directly converting the dictionary keys to a list using the `list()` function. This method is particularly useful when you need to manipulate the keys as a list, allowing for additional operations such as sorting or indexing. Furthermore, using a simple for loop can also achieve the desired result, providing flexibility in how the keys are processed and displayed.
Overall, understanding how to print the keys of a dictionary in Python enhances one’s ability to manipulate and interact with data structures effectively. Mastering these techniques not only improves coding efficiency but also fosters a deeper comprehension of Python’s data handling capabilities. By leveraging the various methods available, developers can tailor their approach to fit specific programming needs and scenarios.
Author Profile
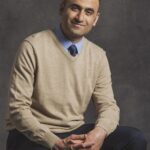
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?