How Can You Print the Type of a Variable in Python?
In the world of programming, understanding the types of variables you’re working with is crucial for writing efficient and error-free code. Python, known for its simplicity and readability, offers a dynamic type system that allows you to work with various data types seamlessly. However, as you dive deeper into your coding journey, you may find yourself needing to confirm the type of a variable at different stages of your program. Whether you’re debugging, optimizing performance, or simply trying to grasp the nuances of Python, knowing how to print the type of a variable can be an invaluable skill.
In this article, we will explore the straightforward yet essential method of determining a variable’s type in Python. By leveraging Python’s built-in functions, you can easily identify whether a variable is an integer, string, list, or any other type. This knowledge not only enhances your coding practices but also empowers you to write more robust and maintainable code. As we delve into the various ways to print variable types, you’ll discover practical examples and tips that will elevate your programming proficiency.
Understanding variable types is not just about curiosity; it’s about equipping yourself with the tools to handle data effectively. From simple scripts to complex applications, knowing how to check and print the type of a variable can help you avoid common pitfalls and
Understanding the `type()` Function
The primary method for determining the type of a variable in Python is the built-in `type()` function. This function returns the type of the specified object, which can be any data type such as integers, strings, lists, or even custom classes. Utilizing `type()` is straightforward and provides immediate feedback on the variable type.
To use the `type()` function, simply pass the variable as an argument:
“`python
x = 5
print(type(x)) Output:
“`
This code snippet shows that the variable `x` is of type `int`.
Examples of Using `type()`
Here are several examples demonstrating how to use the `type()` function with various data types:
“`python
Integer
a = 10
print(type(a)) Output:
Float
b = 10.5
print(type(b)) Output:
String
c = “Hello, World!”
print(type(c)) Output:
List
d = [1, 2, 3]
print(type(d)) Output:
Dictionary
e = {‘key’: ‘value’}
print(type(e)) Output:
“`
These examples illustrate how `type()` can be used to identify different data types in Python.
Using `isinstance()` for Type Checking
In addition to `type()`, the `isinstance()` function is another useful tool for checking the type of a variable. It not only checks the type but also considers inheritance, which can be beneficial in object-oriented programming.
The syntax for `isinstance()` is as follows:
“`python
isinstance(object, classinfo)
“`
Where `object` is the variable you want to check, and `classinfo` can be a type or a tuple of types.
Here is how to use `isinstance()`:
“`python
x = [1, 2, 3]
print(isinstance(x, list)) Output: True
print(isinstance(x, dict)) Output:
“`
Comparison of `type()` and `isinstance()`
The following table summarizes the differences between `type()` and `isinstance()`:
Feature | type() | isinstance() |
---|---|---|
Returns | Exact type of the object | True if the object is an instance of a class or a subclass |
Inheritance | No | Yes |
Use case | Check the exact type | Check type and subtype |
Utilizing `type()` and `isinstance()` provides developers with powerful tools for type checking in Python. Choosing between them depends on whether strict type checking or inheritance consideration is required for your application.
Methods to Determine Variable Types in Python
In Python, determining the type of a variable can be accomplished using several built-in functions. The most common method is to utilize the `type()` function.
Using the `type()` Function
The `type()` function is straightforward and returns the type of the object passed to it. Here’s how it can be employed:
“`python
x = 10
print(type(x)) Output:
y = 3.14
print(type(y)) Output:
z = “Hello, World!”
print(type(z)) Output:
“`
This function can be used with all data types, including:
- Integers
- Floats
- Strings
- Lists
- Dictionaries
- Tuples
- Sets
Using the `isinstance()` Function
The `isinstance()` function serves a dual purpose: it checks if an object is an instance of a specified class or type. This can be particularly useful for validating data types in functions or when handling user input.
“`python
a = [1, 2, 3]
print(isinstance(a, list)) Output: True
b = (1, 2)
print(isinstance(b, tuple)) Output: True
c = {“key”: “value”}
print(isinstance(c, dict)) Output: True
“`
This method is beneficial when you need to ensure that a variable is of a specific type, especially in conditional statements.
Displaying Variable Types in a Readable Format
To print the type of a variable in a more user-friendly manner, you can format the output string. This can enhance clarity when debugging or logging information.
“`python
var = {1, 2, 3}
print(f”The type of var is: {type(var)}”) Output: The type of var is:
“`
Table of Common Data Types
Data Type | Description | Example |
---|---|---|
`int` | Integer number | `5` |
`float` | Floating-point number | `3.14` |
`str` | Sequence of characters | `”Hello”` |
`list` | Ordered, mutable collection | `[1, 2, 3]` |
`tuple` | Ordered, immutable collection | `(1, 2, 3)` |
`dict` | Key-value pairs | `{“key”: “value”}` |
`set` | Unordered collection of unique items | `{1, 2, 3}` |
Type Checking in Conditional Statements
In scenarios where type checking is essential, combining `type()` or `isinstance()` with conditional statements can be effective. This approach is particularly useful in functions that require specific input types.
“`python
def process_data(data):
if isinstance(data, list):
print(“Processing a list.”)
elif isinstance(data, dict):
print(“Processing a dictionary.”)
else:
print(“Unsupported data type.”)
process_data([1, 2, 3]) Output: Processing a list.
process_data({“key”: “value”}) Output: Processing a dictionary.
“`
By leveraging these methods, Python developers can effectively manage and validate variable types throughout their code.
Understanding Variable Types in Python: Expert Insights
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “To print the type of a variable in Python, one can utilize the built-in `type()` function. This function returns the type of an object, allowing developers to confirm the variable’s data type during runtime, which is crucial for debugging and ensuring code reliability.”
Michael Chen (Lead Software Engineer, CodeCrafters). “Using the `print()` function in conjunction with `type()` is a straightforward approach to display a variable’s type. For instance, `print(type(variable_name))` will output the type, which is particularly useful when working with dynamic typing in Python.”
Sarah Johnson (Python Educator, LearnPython.org). “Understanding how to print the type of a variable is fundamental for beginners. It not only aids in grasping the concept of data types but also enhances one’s ability to write efficient and error-free code. I always encourage students to experiment with `type()` to deepen their understanding.”
Frequently Asked Questions (FAQs)
How can I print the type of a variable in Python?
You can print the type of a variable in Python using the `type()` function. For example, `print(type(variable_name))` will display the type of `variable_name`.
What types of variables can I check in Python?
You can check any variable type in Python, including built-in types like integers, floats, strings, lists, tuples, dictionaries, and user-defined classes.
Is there a way to get the type of a variable without printing it?
Yes, you can assign the result of the `type()` function to a variable. For example, `var_type = type(variable_name)` stores the type of `variable_name` in `var_type` without printing it.
Can I check the type of multiple variables at once?
You can check the type of multiple variables by using a loop. For instance, you can iterate over a list of variables and print their types using a for loop:
“`python
for var in variables_list:
print(type(var))
“`
What will happen if I use `type()` on a variable that is not defined?
If you use `type()` on an variable, Python will raise a `NameError`, indicating that the variable is not recognized in the current scope.
Does the `type()` function return a string representation of the type?
No, the `type()` function returns a type object, not a string. To obtain a string representation, you can use `str(type(variable_name))`.
In Python, determining the type of a variable is a fundamental aspect of programming that aids in understanding how data is structured and manipulated. The built-in function `type()` is the primary tool used for this purpose. By passing a variable as an argument to `type()`, developers can easily retrieve and display the type of that variable, whether it be an integer, string, list, or any other data type defined in Python. This function is essential for debugging and ensuring that operations performed on variables are appropriate for their respective types.
Moreover, the output of the `type()` function can be utilized in various contexts, such as conditional statements, to enforce type checks or to implement type-specific logic within a program. This functionality enhances code robustness and helps prevent type-related errors that could lead to runtime exceptions. Understanding the type of a variable also plays a crucial role in type hinting and annotations, which are increasingly important in modern Python programming for improving code clarity and maintainability.
In summary, the ability to print the type of a variable in Python is a straightforward yet powerful feature that supports effective coding practices. By leveraging the `type()` function, developers can gain insights into their data, optimize their code, and ensure that their programs function as
Author Profile
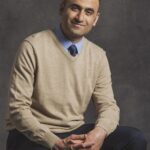
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?