How Can You Determine the Type of a Variable in Python?
In the world of programming, understanding the type of a variable is crucial for effective coding and debugging. Whether you’re a novice just starting your journey into Python or an experienced developer looking to refine your skills, knowing how to print the type of a variable can significantly enhance your coding efficiency. Python, with its dynamic typing system, allows for flexibility in variable assignments, but this very feature can sometimes lead to confusion. This article will guide you through the simple yet essential process of determining and printing the type of any variable in Python, empowering you to write clearer and more robust code.
At its core, Python provides built-in functions that make it easy to identify the type of a variable. This functionality not only helps in ensuring that your code behaves as expected but also aids in debugging when things go awry. By leveraging these tools, you can gain insights into the data structures you’re working with, whether they are integers, strings, lists, or more complex objects. Understanding variable types is a foundational skill that lays the groundwork for more advanced programming concepts.
As we delve deeper into this topic, we will explore various methods to print variable types, the significance of type checking, and practical examples that illustrate these concepts in action. By the end of this article, you will have a solid grasp of how
Using the `type()` Function
To determine the type of a variable in Python, the most straightforward method is to use the built-in `type()` function. This function returns the type of the specified object, which can be particularly useful for debugging or understanding the data being handled in your code.
For example:
“`python
variable = 42
print(type(variable)) Output:
“`
In this example, `type(variable)` returns `
Identifying Multiple Variable Types
When working with multiple variables, you can use the `type()` function in a loop or list comprehension to quickly identify their types. Here’s how you can do this:
“`python
variables = [42, 3.14, ‘hello’, True]
types = [type(var) for var in variables]
print(types) Output: [
“`
This code snippet creates a list of variables and then generates a list of their types.
Using the `isinstance()` Function
While `type()` is useful, the `isinstance()` function provides a more flexible way to check the type of a variable, especially when dealing with subclasses. This function checks if an object is an instance of a specified class or tuple of classes.
For instance:
“`python
variable = ‘hello’
if isinstance(variable, str):
print(“This variable is a string.”)
“`
The `isinstance()` function can also check for multiple types at once:
“`python
if isinstance(variable, (int, float)):
print(“This variable is either an integer or a float.”)
“`
Table of Common Types in Python
Below is a table summarizing some common built-in types in Python:
Type | Description |
---|---|
int | Integer type, representing whole numbers. |
float | Floating-point number type, representing real numbers. |
str | String type, representing sequences of characters. |
list | Mutable sequence type, representing ordered collections. |
tuple | Immutable sequence type, representing ordered collections. |
dict | Dictionary type, representing key-value pairs. |
bool | Boolean type, representing True or values. |
In summary, using `type()` and `isinstance()` provides a robust approach for identifying and validating the types of variables in Python. Understanding these functions can significantly enhance your programming proficiency and help avoid type-related errors.
Methods to Determine Variable Type
In Python, determining the type of a variable can be accomplished using several built-in functions. The primary method is the `type()` function, which returns the type of an object.
Using the `type()` Function
The `type()` function is straightforward and can be applied to any variable. Here’s how it works:
“`python
variable = 42
print(type(variable)) Output:
“`
- Syntax: `type(object)`
- Returns: The type of the specified object.
Using the `isinstance()` Function
Another useful approach is the `isinstance()` function, which checks if an object is an instance of a specific class or a tuple of classes.
“`python
variable = “Hello, World!”
print(isinstance(variable, str)) Output: True
“`
- Syntax: `isinstance(object, classinfo)`
- Returns: `True` if the object is an instance of the class or classes specified; otherwise, “.
Example of Type Checking
Here is a comprehensive example demonstrating both `type()` and `isinstance()`:
“`python
variables = [10, 3.14, “Python”, [1, 2, 3], {‘key’: ‘value’}, None]
for var in variables:
print(f’Type of {var} using type(): {type(var)}’)
print(f’Is {var} an int? {isinstance(var, int)}’)
print(f’Is {var} a float? {isinstance(var, float)}’)
print(f’Is {var} a str? {isinstance(var, str)}’)
print(f’Is {var} a list? {isinstance(var, list)}’)
print(f’Is {var} a dict? {isinstance(var, dict)}’)
print(f’Is {var} NoneType? {isinstance(var, type(None))}’)
print(‘—‘)
“`
This code will output the type of each variable and determine whether it belongs to specified types.
Additional Considerations
- Custom Classes: For user-defined classes, `type()` will return the class type.
“`python
class MyClass:
pass
obj = MyClass()
print(type(obj)) Output:
“`
- Module Types: You can also check types from imported modules, such as `datetime` or `numpy`.
Type Hinting and Annotations
Python supports type hinting, which allows you to indicate the expected type of variables. This is not enforced but serves as documentation and can be checked using static analysis tools.
“`python
def greet(name: str) -> str:
return f”Hello, {name}!”
print(type(greet)) Output:
“`
- Syntax: `variable: Type`
- Usage: Enhances code readability and can help in debugging.
Determining the type of a variable in Python is essential for effective programming and debugging. The `type()` and `isinstance()` functions provide a robust mechanism for type checking, while type hinting enhances code clarity and maintainability.
Understanding Variable Types in Python: Expert Insights
Dr. Emily Chen (Senior Python Developer, Tech Innovations Inc.). “To print the type of a variable in Python, one can utilize the built-in `type()` function. This function is straightforward and returns the type of the specified object, making it an essential tool for debugging and understanding data structures.”
Michael Patel (Lead Software Engineer, CodeCraft Solutions). “Using `print(type(variable))` is a common practice among developers. It not only helps in identifying the variable’s type but also aids in ensuring that the code behaves as expected, especially when working with dynamic typing in Python.”
Sarah Johnson (Data Scientist, Analytics Hub). “In data analysis, knowing the type of your variables is crucial. The `type()` function allows data scientists to quickly ascertain whether they are dealing with integers, floats, strings, or other data types, thus facilitating appropriate data handling and manipulation.”
Frequently Asked Questions (FAQs)
How can I determine the type of a variable in Python?
You can determine the type of a variable in Python by using the built-in `type()` function. For example, `type(variable_name)` will return the type of the specified variable.
What is the output of the `type()` function?
The `type()` function returns a type object that represents the type of the variable passed to it. For instance, if the variable is an integer, it will return `
Can I print the type of a variable directly?
Yes, you can print the type of a variable directly by using the `print()` function in conjunction with `type()`. For example, `print(type(variable_name))` will display the type in the console.
Is there a way to check the type of multiple variables at once?
You can check the type of multiple variables by using a loop or a list comprehension. For example, you can use `print([type(var) for var in [var1, var2, var3]])` to print the types of multiple variables.
What types of variables can I check in Python?
In Python, you can check various types of variables, including integers, floats, strings, lists, tuples, dictionaries, sets, and custom objects. The `type()` function will accurately identify each of these types.
Are there alternative methods to check variable types in Python?
Yes, you can also use the `isinstance()` function to check if a variable is of a specific type. For example, `isinstance(variable_name, int)` will return `True` if the variable is an integer.
In Python, determining the type of a variable is a straightforward process that can be accomplished using the built-in `type()` function. This function returns the type of the specified object, which can be essential for debugging or understanding the data being manipulated in a program. For example, calling `type(variable_name)` will yield the data type of `variable_name`, whether it be an integer, string, list, or any other type defined in Python.
Another useful method for checking the type of a variable is the `isinstance()` function. This function not only checks the type of a variable but also allows for checking against multiple types. Utilizing `isinstance(variable_name, type)` can help ensure that the variable is of the expected type, which is particularly beneficial in scenarios involving function parameters or complex data structures.
In summary, understanding how to print the type of a variable in Python is crucial for effective programming. The `type()` function provides a quick way to identify variable types, while `isinstance()` offers a more flexible approach for type checking. Mastery of these functions can enhance code reliability and facilitate better debugging practices.
Author Profile
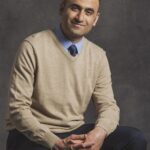
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?