How Can You Program an Arduino Using Python?
In the ever-evolving world of technology, the intersection of programming languages and hardware has opened up a realm of possibilities for hobbyists and professionals alike. Among the myriad of microcontrollers available, Arduino stands out as a favorite for its versatility and ease of use. While traditional programming for Arduino is often done in C/C++, a growing number of enthusiasts are turning to Python, a language celebrated for its simplicity and readability. If you’re curious about how to program Arduino with Python, you’re in for an exciting journey that blends creativity with technical skill.
Programming Arduino with Python allows you to leverage the strengths of both platforms, making it easier to prototype and develop innovative projects. By using libraries like PySerial, you can communicate with your Arduino board seamlessly, enabling you to send and receive data without the steep learning curve of C/C++. This approach not only enhances your coding experience but also opens the door to integrating Arduino with various Python frameworks and applications, expanding the potential of your projects.
In this article, we will explore the essential tools and techniques needed to get started with programming Arduino using Python. Whether you’re a seasoned programmer looking to branch out or a newcomer eager to dive into the world of electronics, you’ll find valuable insights and practical guidance to help you harness the power of Python in your
Setting Up the Environment
To program an Arduino using Python, you need to set up your development environment properly. This involves installing the necessary software and libraries that will allow Python to communicate with the Arduino board.
- Install Python: Ensure that you have Python installed on your computer. The latest version can be downloaded from the official Python website.
- Install PySerial: This library allows Python to communicate with serial ports. You can install it using pip:
“`bash
pip install pyserial
“`
- Install Arduino IDE: While you will be programming in Python, the Arduino IDE is required for uploading sketches to the board. Download it from the Arduino website and install it.
- Set Up Your Arduino Board: Connect your Arduino board to your computer via USB. Open the Arduino IDE, select the correct board type and port, and upload a basic sketch to ensure that the board is functioning.
Basic Communication with Arduino
Once your environment is set up, the next step is to establish communication between Python and the Arduino. The Arduino needs to run a sketch that listens for commands from Python.
- Arduino Sketch: Use the following simple sketch that listens for serial input and toggles an LED based on the received command.
“`cpp
const int ledPin = 13;
void setup() {
Serial.begin(9600);
pinMode(ledPin, OUTPUT);
}
void loop() {
if (Serial.available()) {
char command = Serial.read();
if (command == ‘1’) {
digitalWrite(ledPin, HIGH); // Turn on LED
} else if (command == ‘0’) {
digitalWrite(ledPin, LOW); // Turn off LED
}
}
}
“`
- Python Code: Use the following Python script to communicate with the Arduino. This script sends commands to toggle the LED.
“`python
import serial
import time
Replace ‘COM3’ with your Arduino’s port
arduino = serial.Serial(‘COM3′, 9600)
time.sleep(2) Allow time for the connection to establish
Turn on the LED
arduino.write(b’1′)
time.sleep(1)
Turn off the LED
arduino.write(b’0’)
arduino.close()
“`
Common Libraries for Arduino and Python
There are several libraries available that facilitate interaction between Python and Arduino. Below is a table of some commonly used libraries:
Library | Description |
---|---|
PySerial | Enables serial communication between Python and Arduino. |
Firmata | Protocol for communicating with microcontrollers from software on a host computer. |
Pygame | Useful for creating interactive applications and games involving Arduino. |
Arduino-Python3 | A package designed to simplify the process of controlling Arduino boards. |
Using these libraries can enhance the functionality of your projects and make coding in Python more efficient.
Setting Up the Environment
To program an Arduino using Python, you need to set up your environment correctly. This involves installing the necessary software and libraries.
- Install Python: Ensure that Python is installed on your system. You can download it from the [official Python website](https://www.python.org/downloads/).
- Install PySerial: This library allows Python to communicate with serial ports. You can install it using pip:
“`bash
pip install pyserial
“`
- Install Arduino IDE: While you can program the Arduino using Python, the Arduino IDE is needed for uploading the initial bootloader and for basic management.
- Connect Arduino to your Computer: Use a USB cable to connect your Arduino board to your computer.
Writing Python Code for Arduino
To interact with the Arduino, you will typically write a Python script that sends commands over the serial connection. Below is an example structure for your Python script.
“`python
import serial
import time
Establish the connection to the Arduino
arduino = serial.Serial(port=’COM3′, baudrate=9600, timeout=1)
time.sleep(2) Wait for the connection to establish
Function to send data to Arduino
def send_data(data):
arduino.write(data.encode())
Example of sending data
send_data(“Hello Arduino!”)
Close the connection when done
arduino.close()
“`
Key Components
- Serial Port: Replace `’COM3’` with your Arduino’s port (e.g., `/dev/ttyUSB0` for Linux).
- Baud Rate: Ensure that the baud rate matches the Arduino’s settings (usually 9600).
- Timeout: Set an appropriate timeout for the serial connection.
Arduino Sketch for Receiving Data
While the Python code sends data, the Arduino needs a sketch to receive and process it. Below is a simple example:
“`cpp
void setup() {
Serial.begin(9600); // Initialize serial communication
}
void loop() {
if (Serial.available() > 0) {
String message = Serial.readString(); // Read incoming data
Serial.println(“Received: ” + message); // Echo the received message
}
}
“`
Explanation
- Serial.begin(9600): Initializes the serial communication.
- Serial.available(): Checks if data is available to read.
- Serial.readString(): Reads the incoming data from the serial buffer.
Common Libraries and Tools
Using libraries can significantly enhance your interaction with Arduino. Here are some recommended libraries:
Library | Description |
---|---|
PyFirmata | Control Arduino using the Firmata protocol. |
Arduino-CLI | Command-line interface for Arduino project management. |
Pygame | Useful for creating graphical interfaces to control Arduino. |
Using PyFirmata
To use the PyFirmata library, first install it:
“`bash
pip install pyfirmata
“`
Then, you can utilize it as follows:
“`python
from pyfirmata import Arduino, util
board = Arduino(‘COM3’) Connect to Arduino
iterator = util.Iterator(board)
iterator.start()
Example: Blink an LED connected to pin 13
while True:
board.digital[13].write(1) Turn LED on
time.sleep(1)
board.digital[13].write(0) Turn LED off
time.sleep(1)
“`
This approach provides a more abstract way to interact with the Arduino without direct serial communication.
Troubleshooting Tips
When programming Arduino with Python, you may encounter some common issues:
- Port Not Found: Ensure the correct port is specified and that the Arduino is connected.
- Permission Denied: On Linux, you may need to add your user to the `dialout` group or run the script with `sudo`.
- Data Not Received: Check the baud rate and ensure the Arduino sketch is running.
By following these steps and utilizing the provided code examples, you can successfully program your Arduino using Python.
Programming Arduino with Python: Expert Insights
Dr. Emily Chen (Embedded Systems Specialist, Tech Innovations Inc.). “Utilizing Python to program Arduino boards opens up a world of possibilities for rapid prototyping and ease of use. Libraries like PyMata and Firmata allow developers to leverage Python’s simplicity while still accessing the powerful capabilities of Arduino hardware.”
Michael Thompson (Lead Software Engineer, Robotics Research Group). “Integrating Python with Arduino can significantly enhance the development process, especially for complex projects. The ability to write scripts in Python allows for quick iterations and testing, which is invaluable in robotics and automation.”
Sarah Patel (IoT Solutions Architect, Future Tech Labs). “While Arduino is traditionally programmed in C/C++, using Python can simplify the learning curve for beginners. This approach encourages more people to explore hardware programming and fosters innovation in IoT applications.”
Frequently Asked Questions (FAQs)
How can I set up my Arduino to be programmed with Python?
To program your Arduino with Python, you need to install the `pySerial` library, which allows communication between Python and the Arduino. Additionally, you may need to upload a compatible sketch to the Arduino board that listens for commands from Python.
What libraries are available for programming Arduino with Python?
Several libraries facilitate programming Arduino with Python, including `pySerial`, `Arduino-Python3`, and `Firmata`. The Firmata protocol is particularly useful as it allows Python to control the Arduino board in real time.
Can I use Python to upload sketches to my Arduino?
Typically, Python is not used to upload sketches directly to Arduino. However, you can use Python scripts to send commands to an already uploaded sketch that communicates with the board, or you can use tools like `Arduino-cli` to manage uploads through Python.
What is the role of the Firmata protocol when programming Arduino with Python?
The Firmata protocol acts as a communication protocol between the Arduino and Python. By uploading the Firmata sketch to the Arduino, you enable Python scripts to control the board’s pins and read sensor data without the need for constant reprogramming.
Are there any limitations to programming Arduino with Python?
Yes, there are limitations. Python is generally slower than C/C++, which is the native language for Arduino sketches. Additionally, not all libraries and functionalities available in C/C++ are accessible in Python, which may restrict certain advanced features.
What IDEs or tools can I use to program Arduino with Python?
You can use various IDEs and text editors to write Python scripts for Arduino, such as PyCharm, VSCode, or even simple text editors like Notepad++. Additionally, you can use the Arduino IDE for uploading sketches and managing your Arduino board.
Programming an Arduino with Python offers a flexible and powerful alternative to the traditional C/C++ programming environment. By utilizing libraries such as PySerial, users can establish serial communication between their Python scripts and the Arduino board. This allows for a seamless integration of Python’s extensive capabilities, such as data analysis and machine learning, with the hardware control features of Arduino. The process typically involves writing a sketch in the Arduino IDE to handle incoming data and commands, while the Python script manages the logic and user interface.
One of the key takeaways from this approach is the ability to leverage Python’s rich ecosystem of libraries, which can significantly enhance the functionality of Arduino projects. For instance, users can easily implement data visualization or connect to web services using Python, broadening the scope of what can be achieved with Arduino hardware. Furthermore, the ease of use of Python makes it accessible for beginners, while still providing depth for advanced users looking to create complex applications.
Additionally, the community support around both Arduino and Python is robust, with numerous tutorials, forums, and resources available to assist users in troubleshooting and expanding their projects. This collaborative environment fosters innovation and encourages experimentation, making it an ideal choice for hobbyists and professionals alike. Ultimately, programming Arduino with Python
Author Profile
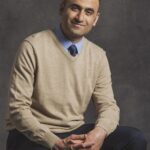
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?