How Can You Raise a Number to a Power in Python?
Raising numbers to a power is a fundamental operation in mathematics, and in the world of programming, it holds equal significance. Whether you’re working on complex algorithms, data analysis, or simply exploring the vast capabilities of Python, knowing how to efficiently raise a number to a power can enhance your coding prowess. In Python, this operation is not only straightforward but also versatile, allowing for a variety of applications from basic calculations to intricate scientific computations.
In Python, there are multiple ways to achieve exponentiation, each with its own use cases and advantages. The most common method involves using the exponentiation operator, which is both intuitive and easy to remember. Additionally, Python provides built-in functions that can handle more complex scenarios, such as raising numbers to fractional powers or even working with negative bases. Understanding these methods will empower you to manipulate numbers with ease and precision, making your coding experience smoother and more efficient.
As you delve deeper into the world of Python, mastering how to raise numbers to a power will not only bolster your mathematical toolkit but also enhance your problem-solving skills. Whether you’re a novice programmer looking to grasp the basics or an experienced coder seeking to refine your techniques, this knowledge will prove invaluable in your journey through Python programming. Get ready to unlock the full potential of exponentiation in
Using the Exponentiation Operator
In Python, the simplest way to raise a number to a power is by using the exponentiation operator, which is represented by `**`. This operator allows for straightforward calculations, enabling you to raise any number to any power with ease.
For example:
“`python
result = 2 ** 3 This raises 2 to the power of 3
print(result) Output: 8
“`
You can also raise negative numbers and fractions to a power:
“`python
negative_result = (-2) ** 3 Output: -8
fraction_result = (0.5) ** 2 Output: 0.25
“`
Using the Built-in `pow()` Function
Another method to raise a number to a power in Python is through the built-in `pow()` function. This function can take two or three arguments, where the first two arguments are the base and the exponent, respectively. If a third argument is provided, `pow()` calculates the result modulo that third argument.
Here’s how to use `pow()`:
“`python
result = pow(2, 3) Raises 2 to the power of 3
print(result) Output: 8
mod_result = pow(2, 3, 3) Raises 2 to the power of 3, then takes modulo 3
print(mod_result) Output: 2
“`
The `pow()` function is particularly useful in scenarios requiring modular arithmetic, which is often applied in cryptography.
Comparison of Methods
Both methods, `**` and `pow()`, are effective for exponentiation, but they may have different use cases and performance characteristics. Below is a comparison of their features:
Method | Syntax | Use Case | Performance |
---|---|---|---|
Exponentiation Operator | `base ** exponent` | Simple exponentiation | Fast for basic calculations |
`pow()` Function | `pow(base, exponent[, modulus])` | Exponentiation with optional modulus | Optimized for modular arithmetic |
Raising Numbers in Loops
In scenarios where you need to raise multiple numbers to powers, you can utilize loops. For instance, you might want to raise each number in a list to a specific power:
“`python
numbers = [1, 2, 3, 4, 5]
squared_numbers = [num ** 2 for num in numbers] List comprehension
print(squared_numbers) Output: [1, 4, 9, 16, 25]
“`
This technique is efficient and readable, making it a preferred choice for such operations.
Python provides multiple methods to raise numbers to powers, allowing for flexibility based on user needs. Whether using the `**` operator for straightforward calculations or the `pow()` function for more complex scenarios, both options are easily accessible and well-suited for various applications.
Using the Exponentiation Operator
In Python, the most straightforward method to raise a number to a power is by using the exponentiation operator `**`. This operator allows you to perform exponentiation directly in your expressions.
Example:
“`python
result = 2 ** 3 Raises 2 to the power of 3
print(result) Output: 8
“`
Using the Built-in pow() Function
Another method for exponentiation is the built-in `pow()` function. This function can be used for both two-parameter and three-parameter forms.
- Two-parameter form:
This form computes the result of raising the first argument to the power of the second argument.
“`python
result = pow(3, 4) Raises 3 to the power of 4
print(result) Output: 81
“`
- Three-parameter form:
This version computes the result and also takes a modulus, returning the result modulo the third argument.
“`python
result = pow(3, 4, 5) Raises 3 to the power of 4, then takes modulus 5
print(result) Output: 1
“`
Handling Negative and Fractional Exponents
Python’s exponentiation operator and `pow()` function also support negative and fractional exponents, enabling you to calculate roots and reciprocal powers easily.
Example of negative exponent:
“`python
result = 2 -2 Equivalent to 1/(22)
print(result) Output: 0.25
“`
Example of fractional exponent:
“`python
result = 16 ** 0.5 Square root of 16
print(result) Output: 4.0
“`
Performance Considerations
When dealing with large numbers or high powers, Python’s `pow()` function can be more efficient than using the `**` operator, especially when the modulus is also provided. The three-parameter `pow()` utilizes an optimized algorithm for modular exponentiation.
Method | Description | When to Use |
---|---|---|
`**` Operator | Simple exponentiation | General use and clarity |
`pow(base, exp)` | Exponentiation with an optional modulus | Performance with large numbers |
`pow(base, exp, mod)` | Efficient modular exponentiation | When working with large integers |
Raising Elements in a List
To raise each element in a list to a specific power, you can use list comprehension or the `map()` function.
Example using list comprehension:
“`python
numbers = [1, 2, 3, 4]
squared = [x ** 2 for x in numbers] Raises each number to the power of 2
print(squared) Output: [1, 4, 9, 16]
“`
Example using `map()`:
“`python
numbers = [1, 2, 3, 4]
squared = list(map(lambda x: x ** 2, numbers)) Raises each number to the power of 2
print(squared) Output: [1, 4, 9, 16]
“`
Utilizing these methods allows for efficient and effective exponentiation in Python, whether working with single values or collections.
Expert Insights on Raising Powers in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Python, raising a number to a power can be efficiently accomplished using the exponentiation operator ‘**’. This operator allows for both integer and floating-point calculations, making it versatile for various applications in scientific computing.”
Michael Chen (Python Developer Advocate, CodeMaster Academy). “For those looking to enhance readability in their code, utilizing the built-in `pow()` function is a great alternative. It not only raises a number to a power but also includes an optional modulus argument, which can be particularly useful in cryptographic applications.”
Sarah Patel (Data Scientist, Analytics Solutions Group). “When working with large datasets, leveraging NumPy’s `numpy.power()` function can significantly improve performance. This function is optimized for array operations, allowing for efficient calculations across entire datasets in a single command.”
Frequently Asked Questions (FAQs)
How do I raise a number to a power in Python?
You can raise a number to a power in Python using the exponentiation operator ``. For example, `result = base exponent` computes the value of `base` raised to the power of `exponent`.
Is there a built-in function for exponentiation in Python?
Yes, Python provides a built-in function called `pow()`. It can be used as `result = pow(base, exponent)` to achieve the same result as the `**` operator.
Can I use negative exponents in Python?
Yes, Python supports negative exponents. For example, `result = 2 ** -3` will compute the reciprocal, yielding `0.125` (which is `1/8`).
What happens if I raise a number to the power of zero in Python?
In Python, any non-zero number raised to the power of zero equals `1`. For example, `5 ** 0` will return `1`.
How can I raise a number to a fractional power in Python?
You can raise a number to a fractional power using the `` operator or the `pow()` function. For instance, `result = 9 0.5` computes the square root of `9`, which is `3.0`.
What is the difference between using `**` and `pow()` in Python?
The `` operator is a shorthand for exponentiation, while `pow()` is a function that can also accept a third argument for modulus. For example, `pow(base, exponent, modulus)` computes `(base exponent) % modulus`.
In Python, raising a number to a power can be accomplished using several methods, each with its own advantages. The most common approach is to use the exponentiation operator ``, which allows for straightforward syntax and readability. For example, `result = base exponent` effectively computes the power of the base raised to the specified exponent. This method is intuitive and widely used in various applications, from simple calculations to complex mathematical modeling.
Another method to raise a number to a power in Python is by utilizing the built-in `pow()` function. This function can take two or three arguments, where the first two represent the base and exponent, respectively, and the optional third argument allows for modular exponentiation. The syntax `result = pow(base, exponent)` or `result = pow(base, exponent, modulus)` provides flexibility depending on the specific needs of the computation, particularly in scenarios involving large numbers or cryptography.
Additionally, the `math` module in Python offers the `math.pow()` function, which is specifically designed for floating-point exponentiation. It is important to note that `math.pow()` always returns a float, which can be beneficial in certain contexts. However, for integer exponentiation, the `**` operator or `
Author Profile
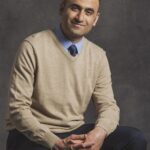
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?