How Can You Read a File in JavaScript? A Step-by-Step Guide
Introduction
In the ever-evolving world of web development, the ability to read files directly from a user’s device has become an essential skill for developers. Whether you’re building a sleek web application that needs to process user-uploaded documents or creating a dynamic webpage that interacts with local data, understanding how to read a file in JavaScript is crucial. This powerful capability not only enhances user experience but also opens up a realm of possibilities for data manipulation and analysis right in the browser. Join us as we explore the various methods and techniques for reading files in JavaScript, empowering you to take your web projects to the next level.
Reading files in JavaScript involves a few key concepts and tools that make the process both straightforward and efficient. With the advent of the File API, developers can easily access and manipulate files selected by users through input elements or drag-and-drop interfaces. This API allows for seamless integration of file reading functionalities, enabling you to handle text files, images, and other formats with ease.
Moreover, understanding how to handle asynchronous operations is vital when working with file reading in JavaScript. As files can vary in size and complexity, using Promises and async/await syntax becomes essential for ensuring smooth and responsive applications. As we delve deeper into this topic, you will discover
Using the FileReader API
The FileReader API provides a simple way to read the contents of files stored on the user’s computer. It allows web applications to asynchronously read file data selected by the user through an `` element of type `file`. To effectively utilize the FileReader API, the following steps can be taken:
- Create an `` element to allow users to select a file.
- Instantiate a FileReader object.
- Use appropriate methods to read the file data.
Here is an example of how to implement this:
In this example, when a user selects a file, the FileReader reads the file as text, and upon completion, the content is displayed in a `
` element.Reading JSON Files
Reading JSON files in JavaScript is straightforward using the FileReader API. After reading the file, it is crucial to parse the JSON data to make it usable within the application. Below is an example of how to read a JSON file:This code snippet will parse the JSON content and display it formatted in a readable way.
Using Fetch API for External Files
For scenarios where files are hosted on a server, the Fetch API can be utilized to read text files, JSON files, or any other resources. Fetch provides a more modern approach to network requests compared to older methods such as XMLHttpRequest. Here’s an example of how to fetch and display a text file:
javascript
fetch('path/to/your/file.txt')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.text();
})
.then(data => {
console.log(data);
})
.catch(error => {
console.error('There was a problem with the fetch operation:', error);
});For JSON files, the approach is similar, but you would use `response.json()` instead:
javascript
fetch('path/to/your/file.json')
.then(response => response.json())
.then(data => {
console.log(data);
})
.catch(error => {
console.error('There was a problem with the fetch operation:', error);
});File Reading Methods Comparison
When reading files in JavaScript, it is essential to choose the right method based on your application's requirements. Below is a comparison table of the methods discussed:
Method | Use Case | Asynchronous |
---|---|---|
FileReader API | Reading local files selected by the user | Yes |
Fetch API | Fetching files from a remote server | Yes |
Understanding these methods and their appropriate contexts will enhance your ability to handle file reading in JavaScript effectively.
Reading Files in JavaScript Using the File API
JavaScript provides a powerful File API that allows developers to read files from the user's system. This is particularly useful in web applications where users need to upload files.
To read a file, follow these steps:
- Create an HTML File Input Element
This allows users to select the file they wish to read.
- **Access the File Input in JavaScript**
Use JavaScript to access the file input and retrieve the file object.
javascript
const fileInput = document.getElementById('fileInput');
fileInput.addEventListener('change', (event) => {
const file = event.target.files[0]; // Get the first file
if (file) {
readFile(file);
}
});
- Read the File with FileReader
Utilize the `FileReader` object to read the contents of the file. This can be done in various formats, such as text, data URLs, or binary strings.
javascript
function readFile(file) {
const reader = new FileReader();
reader.onload = function(event) {
const fileContent = event.target.result; // File content as string
console.log(fileContent); // Process the file content
};
reader.onerror = function(event) {
console.error("Error reading file: ", event.target.error);
};
reader.readAsText(file); // Use the desired method to read
}
Different Methods of Reading Files
The `FileReader` API provides several methods to read files, each suitable for different use cases:
Method | Description | Use Case |
---|---|---|
`readAsText(file)` | Reads the file as a text string. | Ideal for text files such as .txt or .csv. |
`readAsDataURL(file)` | Reads the file and encodes it as a Base64 URL. | Useful for images or media files to display directly in the browser. |
`readAsArrayBuffer(file)` | Reads the file as a binary buffer. | Suitable for binary data processing, like audio or video files. |
`readAsBinaryString(file)` | Reads the file as a binary string (deprecated). | Previously used for binary data, but now generally replaced by `readAsArrayBuffer`. |
Handling File Read Errors
Error handling is crucial when dealing with file input. The `FileReader` object emits an error event when an issue arises. Implement error handling as shown:
javascript
reader.onerror = function(event) {
switch(event.target.error.code) {
case event.target.error.NOT_FOUND_ERR:
console.error("File not found!");
break;
case event.target.error.NOT_READABLE_ERR:
console.error("File not readable!");
break;
case event.target.error.ABORT_ERR:
console.error("File read aborted!");
break;
default:
console.error("An unknown error occurred.");
break;
}
};
Using Fetch API to Read Files from the Server
If you need to read files from a server rather than from user input, you can leverage the Fetch API. This is suitable for obtaining files stored on a server.
javascript
fetch('path/to/your/file.txt')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.text(); // or response.blob() for binary files
})
.then(data => {
console.log(data); // Process the file content
})
.catch(error => {
console.error('Error fetching the file: ', error);
});
This approach allows seamless integration of file reading capabilities, whether from local user input or remote resources.
Expert Insights on Reading Files in JavaScript
Jessica Lin (Senior JavaScript Developer, Tech Innovations Inc.). "Reading files in JavaScript can be efficiently accomplished using the File API in conjunction with the FileReader object. This allows developers to handle file inputs seamlessly in web applications, enhancing user experience."
Mark Thompson (Lead Software Engineer, CodeCrafters). "For server-side JavaScript, utilizing the 'fs' module in Node.js is essential. This module provides a robust set of functions for reading files asynchronously, which is crucial for maintaining performance in a non-blocking environment."
Sofia Patel (Web Development Instructor, Digital Learning Academy). "Understanding the differences between synchronous and asynchronous file reading methods in JavaScript is vital. While synchronous methods are simpler, they can lead to performance issues, especially in larger applications."
Frequently Asked Questions (FAQs)
How can I read a text file in JavaScript?
You can read a text file in JavaScript using the File API in conjunction with an `` element. By using the `FileReader` object, you can read the contents of the file as text.
What is the FileReader API in JavaScript?
The FileReader API allows web applications to asynchronously read the contents of files stored on the user's computer. It provides methods to read files as text, data URLs, or binary strings.
Can I read files from a server using JavaScript?
Yes, you can read files from a server using JavaScript by making HTTP requests. You can use the `fetch` API or `XMLHttpRequest` to retrieve file data from a specified URL.
Is it possible to read JSON files in JavaScript?
Yes, you can read JSON files in JavaScript using the `fetch` API. You can retrieve the file, then parse the JSON data using `response.json()` to convert it into a JavaScript object.
What are the limitations of reading files in JavaScript?
JavaScript running in the browser has limitations regarding file access. It can only read files selected by the user via an input element and cannot access the local file system directly due to security restrictions.
How do I handle errors when reading files in JavaScript?
You can handle errors when reading files by using the `onerror` event handler of the `FileReader` object. This allows you to catch and respond to any issues that occur during the file reading process.
Reading a file in JavaScript can be accomplished through various methods, depending on the environment in which the code is executed. In a browser context, the File API and the FileReader object are commonly used to read files selected by users through an input element. This allows developers to handle files in a user-friendly manner, enabling functionalities such as displaying images or processing text files directly within the web application.
In contrast, when working in a Node.js environment, the built-in 'fs' (file system) module is utilized to read files from the server's file system. This module provides asynchronous and synchronous methods to read files, allowing for efficient handling of file operations without blocking the execution of other code. Understanding the differences between these approaches is crucial for developers to effectively manage file reading tasks in their respective environments.
Overall, mastering file reading in JavaScript involves recognizing the appropriate methods and APIs for the specific context, whether it be client-side or server-side. By leveraging these tools, developers can enhance their applications with functionalities that require file manipulation, ultimately improving user experience and application performance.
Author Profile
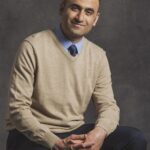
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?