How Can You Read a Text File into Python Effortlessly?
In the digital age, data is everywhere, and the ability to manipulate and analyze that data is a crucial skill for anyone looking to harness the power of programming. One of the most fundamental tasks in Python programming is reading text files, a skill that opens the door to a myriad of applications, from data analysis to web scraping. Whether you’re a seasoned developer or a curious beginner, understanding how to read a text file into Python is an essential step in your coding journey.
Reading text files in Python is not just a technical requirement; it’s an opportunity to interact with data in a meaningful way. Python provides a variety of methods to access and process text files, making it flexible and user-friendly. This capability allows programmers to extract valuable information, manipulate content, and even automate tasks that involve large datasets. By mastering this skill, you can streamline workflows, enhance your projects, and unlock new possibilities in your programming endeavors.
As we delve deeper into this topic, we will explore the various techniques and best practices for reading text files in Python. From understanding file modes to utilizing built-in functions, you will gain insights that will empower you to handle text data effectively. So, let’s embark on this journey and discover the simplicity and elegance of working with text files in Python!
Using the Built-in Open Function
The most common method for reading a text file in Python is by utilizing the built-in `open()` function. This function opens the file and returns a file object, which provides various methods to read the file’s contents. The `open()` function requires at least one argument: the path to the file. It also accepts a second optional argument that specifies the mode in which the file should be opened. The most frequently used modes are:
- `’r’`: Read (default mode)
- `’w’`: Write (overwrites the file if it exists)
- `’a’`: Append (adds to the end of the file)
- `’b’`: Binary mode
The syntax for opening a file is as follows:
“`python
file_object = open(‘file_path.txt’, ‘r’)
“`
Once the file is opened, you can read its contents using methods such as `.read()`, `.readline()`, or `.readlines()`.
Reading Entire File Contents
To read the entire contents of a file, you can use the `.read()` method. Here’s how:
“`python
with open(‘file_path.txt’, ‘r’) as file:
contents = file.read()
print(contents)
“`
Using the `with` statement ensures that the file is properly closed after its suite finishes, even if an exception is raised. This approach is highly recommended for file operations.
Reading Line by Line
If you want to read a file line by line, the `.readline()` method can be used. This method reads a single line from the file each time it is called. Alternatively, you can iterate over the file object itself, which is a more memory-efficient approach:
“`python
with open(‘file_path.txt’, ‘r’) as file:
for line in file:
print(line.strip()) Using strip() to remove any extra whitespace
“`
Reading All Lines into a List
To read all lines of a file at once and store them in a list, you can use the `.readlines()` method. This method returns a list where each element corresponds to a line in the file:
“`python
with open(‘file_path.txt’, ‘r’) as file:
lines = file.readlines()
print(lines)
“`
This method is useful when you need to process lines individually later on.
File Reading Modes Overview
Here’s a table summarizing the common file modes used when opening a file:
Mode | Description |
---|---|
‘r’ | Open for reading (default) |
‘w’ | Open for writing, truncating the file first |
‘a’ | Open for writing, appending to the end of the file |
‘b’ | Binary mode (used with other modes) |
‘x’ | Open for exclusive creation, failing if the file already exists |
Using the correct mode is essential for achieving the desired file operation.
Reading a Text File
To read a text file in Python, you can utilize the built-in `open()` function, which allows you to access and manipulate file contents effectively. Below are various methods for reading text files, along with examples for each.
Using the `open()` Function
The `open()` function opens a file and returns a file object. You can specify the mode in which the file should be opened, such as read (`’r’`), write (`’w’`), or append (`’a’`).
“`python
with open(‘example.txt’, ‘r’) as file:
content = file.read()
print(content)
“`
- The `with` statement ensures proper acquisition and release of resources.
- The file will automatically close after the block of code is executed.
Reading Line by Line
If you prefer to read a file line by line, you can iterate over the file object directly:
“`python
with open(‘example.txt’, ‘r’) as file:
for line in file:
print(line.strip())
“`
- The `strip()` method removes any leading and trailing whitespace, including newline characters.
Reading All Lines into a List
You can also read all lines of a file into a list using the `readlines()` method:
“`python
with open(‘example.txt’, ‘r’) as file:
lines = file.readlines()
for line in lines:
print(line.strip())
“`
- Each line in the list retains its newline character unless `strip()` is applied.
Using `read()` with Specific Amounts
If you need to read a specific number of characters from a file, you can pass an integer to the `read()` method:
“`python
with open(‘example.txt’, ‘r’) as file:
partial_content = file.read(100) Read first 100 characters
print(partial_content)
“`
- The number passed to `read()` determines how many characters to read.
Handling Exceptions
It’s important to handle exceptions that may arise during file operations. The most common exceptions include `FileNotFoundError` and `IOError`. You can manage these using a try-except block:
“`python
try:
with open(‘example.txt’, ‘r’) as file:
content = file.read()
print(content)
except FileNotFoundError:
print(“The file was not found.”)
except IOError:
print(“An error occurred while reading the file.”)
“`
- This ensures your program can gracefully handle errors without crashing.
Working with CSV Files
For reading CSV files, Python provides the `csv` module, which simplifies the process:
“`python
import csv
with open(‘example.csv’, ‘r’) as file:
reader = csv.reader(file)
for row in reader:
print(row)
“`
- Each row is read as a list, allowing for easy access to individual elements.
Reading Files in Different Modes
When opening files, you can also specify different modes:
Mode | Description |
---|---|
‘r’ | Read (default) |
‘w’ | Write (overwrites existing files) |
‘a’ | Append (adds to existing files) |
‘rb’ | Read in binary mode |
‘wb’ | Write in binary mode |
- Choose the mode according to your needs to ensure proper handling of file operations.
These methods provide a robust framework for reading text files in Python, allowing for various approaches depending on the specific requirements of your application.
Expert Insights on Reading Text Files in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Reading a text file in Python can be accomplished efficiently using the built-in `open()` function. This function allows developers to specify the mode in which the file is opened, such as read (‘r’) or write (‘w’), making it versatile for various applications.”
James Liu (Data Scientist, Analytics Hub). “For those looking to read large text files, I recommend using the `with` statement in Python. This ensures that the file is properly closed after its suite finishes, which is crucial for resource management and preventing memory leaks.”
Sarah Thompson (Python Developer, CodeCrafters). “When reading text files, it is essential to handle exceptions properly. Utilizing try-except blocks will help manage potential errors, such as file not found or permission issues, ensuring that your program remains robust and user-friendly.”
Frequently Asked Questions (FAQs)
How can I read a text file in Python?
You can read a text file in Python using the built-in `open()` function along with methods like `.read()`, `.readline()`, or `.readlines()`. For example, `with open(‘file.txt’, ‘r’) as file: content = file.read()` reads the entire file into a string.
What is the difference between `read()`, `readline()`, and `readlines()`?
The `read()` method reads the entire file as a single string, `readline()` reads one line at a time, and `readlines()` reads all lines into a list, where each element is a line from the file.
How do I handle file exceptions when reading a text file?
You can handle exceptions by using a `try` and `except` block. For example:
“`python
try:
with open(‘file.txt’, ‘r’) as file:
content = file.read()
except FileNotFoundError:
print(“File not found.”)
“`
Can I read a text file line by line in a loop?
Yes, you can read a text file line by line using a `for` loop. For example:
“`python
with open(‘file.txt’, ‘r’) as file:
for line in file:
print(line)
“`
What encoding should I use when reading a text file?
The default encoding in Python is usually UTF-8. However, if you are working with files in different encodings, specify the encoding parameter in the `open()` function, such as `open(‘file.txt’, ‘r’, encoding=’utf-16′)`.
Is it necessary to close a file after reading it in Python?
While using the `with` statement automatically closes the file when the block is exited, if you use `open()` without it, you should explicitly call `file.close()` to free up system resources.
Reading a text file into Python is a fundamental skill that enables users to access and manipulate data stored in external files. The most common method involves using the built-in `open()` function, which allows for various modes of file access, such as reading (‘r’), writing (‘w’), or appending (‘a’). After opening the file, users can read its contents using methods like `read()`, `readline()`, or `readlines()`, depending on their specific needs for data retrieval.
It is essential to handle files properly to avoid resource leaks. This is typically achieved by using a context manager, implemented with the `with` statement, which automatically closes the file once the block of code is exited. This approach not only simplifies the code but also enhances its reliability by ensuring that files are closed appropriately after their contents have been processed.
Moreover, understanding how to handle exceptions is crucial when dealing with file operations. Implementing error handling using try-except blocks can help manage potential issues, such as file not found errors or permission issues, making the code more robust and user-friendly. By mastering these techniques, users can effectively read and manipulate text files in Python, paving the way for more complex data processing tasks.
Author Profile
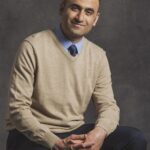
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?