How Can You Read an XML File in Python?
In a world where data is king, XML (eXtensible Markup Language) stands out as a versatile format widely used for storing and transporting structured information. Whether you’re working with web services, configuration files, or data interchange between systems, knowing how to read XML files in Python can empower you to harness the full potential of your data. This article will guide you through the essential techniques and libraries available in Python to effectively parse and manipulate XML files, opening up a world of possibilities for your projects.
Reading XML files in Python may seem daunting at first, but with the right tools and understanding, it becomes a straightforward task. Python offers several libraries, such as `xml.etree.ElementTree` and `lxml`, that simplify the process of parsing XML data. These libraries allow you to navigate through the hierarchical structure of XML documents, making it easy to extract relevant information and convert it into usable formats for your applications.
As you delve deeper into the intricacies of XML parsing, you’ll discover various methods to handle different XML structures and attributes. From simple file reading to more complex scenarios involving namespaces and validation, this article will equip you with the knowledge you need to tackle any XML-related challenge. Whether you’re a beginner or an experienced programmer, mastering XML in Python will enhance
Using the ElementTree Module
The `xml.etree.ElementTree` module is a standard library in Python that provides a simple and efficient way to parse XML files. It allows you to navigate through the XML structure and access the data easily. Here is how you can read an XML file using this module:
“`python
import xml.etree.ElementTree as ET
Load and parse the XML file
tree = ET.parse(‘file.xml’)
root = tree.getroot()
Accessing elements
for child in root:
print(child.tag, child.attrib)
“`
In this example, `ET.parse()` reads the XML file and creates an `ElementTree` object. The `getroot()` method retrieves the root element of the XML tree, allowing you to iterate through its children.
Reading XML with the minidom Module
Another option for reading XML files is the `xml.dom.minidom` module. This module provides a more flexible way to parse XML documents and can be particularly useful for handling complex XML structures. Here is a basic example:
“`python
from xml.dom import minidom
Load and parse the XML file
doc = minidom.parse(‘file.xml’)
Accessing elements
items = doc.getElementsByTagName(‘item’)
for item in items:
print(item.firstChild.nodeValue)
“`
The `getElementsByTagName` method retrieves a list of elements with the specified tag name, allowing for easy extraction of values.
Using the lxml Library
For more advanced XML processing, the `lxml` library is recommended due to its speed and additional features. It must be installed separately, as it is not included in the standard library. You can install it using pip:
“`bash
pip install lxml
“`
Here’s how to read an XML file using `lxml`:
“`python
from lxml import etree
Load and parse the XML file
tree = etree.parse(‘file.xml’)
root = tree.getroot()
Accessing elements
for elem in root.iter(‘item’):
print(elem.text)
“`
This example utilizes the `iter()` method to iterate through all occurrences of the specified tag.
Comparison of XML Parsing Methods
The choice of XML parsing method can depend on your specific needs. Below is a comparison table highlighting key differences:
Feature | ElementTree | minidom | lxml |
---|---|---|---|
Standard Library | Yes | Yes | No |
Performance | Good | Moderate | Excellent |
Ease of Use | Simple | Moderate | More Complex |
XPath Support | No | No | Yes |
Each method has its advantages and disadvantages, and your selection should be based on the complexity of the XML document and the requirements of your project.
Understanding XML Structure
XML (eXtensible Markup Language) is a markup language that defines rules for encoding documents in a format that is both human-readable and machine-readable. An XML document typically consists of:
- A prolog that defines the XML version and character encoding.
- A root element that encapsulates all other elements.
- Child elements that provide data within the structure.
- Attributes that offer additional information about elements.
Example of a basic XML structure:
“`xml
Reading XML Files with ElementTree
The `xml.etree.ElementTree` module in Python is a simple and efficient way to parse and manipulate XML data. Below are the steps to read an XML file using this module.
- Import the ElementTree module:
“`python
import xml.etree.ElementTree as ET
“`
- Load and parse the XML file:
“`python
tree = ET.parse(‘file.xml’)
root = tree.getroot()
“`
- Accessing elements:
You can access child elements using various methods:
- Use `find()` to locate a single child element.
- Use `findall()` to retrieve a list of matching child elements.
- Use `text` to get the text content of an element.
Example code to access elements:
“`python
for book in root.findall(‘book’):
title = book.get(‘title’)
author = book.get(‘author’)
year = book.find(‘year’).text
genre = book.find(‘genre’).text
print(f’Title: {title}, Author: {author}, Year: {year}, Genre: {genre}’)
“`
Reading XML Files with minidom
The `xml.dom.minidom` module is another option, providing a more DOM-compliant interface. It allows for a more detailed manipulation of the XML structure but may be less memory efficient for large files.
- Import the minidom module:
“`python
from xml.dom import minidom
“`
- Parse the XML file:
“`python
doc = minidom.parse(‘file.xml’)
“`
- Accessing elements:
You can access elements using methods like `getElementsByTagName()`.
Example code:
“`python
books = doc.getElementsByTagName(‘book’)
for book in books:
title = book.getAttribute(‘title’)
author = book.getAttribute(‘author’)
year = book.getElementsByTagName(‘year’)[0].firstChild.nodeValue
genre = book.getElementsByTagName(‘genre’)[0].firstChild.nodeValue
print(f’Title: {title}, Author: {author}, Year: {year}, Genre: {genre}’)
“`
Performance Considerations
When reading XML files, consider the following:
Method | Pros | Cons |
---|---|---|
`ElementTree` | Lightweight, easy to use | Limited to simple XML structures |
`minidom` | More powerful, supports complex structures | Higher memory usage, slower parsing |
Choosing the right method depends on the size and complexity of the XML data you are working with. For simple and moderately sized XML files, `ElementTree` is often sufficient, while `minidom` may be preferred for more complex data manipulation tasks.
Expert Insights on Reading XML Files in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “When reading XML files in Python, I recommend using the ElementTree module, which is part of the standard library. It provides a simple and efficient way to parse and navigate XML data, making it ideal for data extraction tasks.”
James Liu (Software Engineer, Data Solutions Group). “For complex XML structures, I often utilize the lxml library due to its speed and ease of use. It allows for XPath queries, which can be extremely beneficial for extracting specific data points from large XML documents.”
Maria Gonzalez (Lead Python Developer, Open Source Projects). “Using the xmltodict library can significantly simplify the process of reading XML files in Python. It converts XML data into Python dictionaries, which makes it easier to manipulate and access the data programmatically.”
Frequently Asked Questions (FAQs)
How can I read an XML file in Python?
You can read an XML file in Python using libraries such as `xml.etree.ElementTree`, `lxml`, or `xml.dom.minidom`. The `ElementTree` module is commonly used for its simplicity and ease of use.
What is the basic syntax for using ElementTree to read an XML file?
To read an XML file using `ElementTree`, you can use the following syntax:
“`python
import xml.etree.ElementTree as ET
tree = ET.parse(‘file.xml’)
root = tree.getroot()
“`
How do I access specific elements in an XML file using Python?
You can access specific elements by using the `find()` or `findall()` methods on the root or any element. For example:
“`python
element = root.find(‘tag_name’)
elements = root.findall(‘tag_name’)
“`
Can I read XML files with namespaces in Python?
Yes, you can read XML files with namespaces by using the `find()` method with the namespace defined in a dictionary. For example:
“`python
namespaces = {‘ns’: ‘http://example.com/ns’}
element = root.find(‘ns:tag_name’, namespaces)
“`
What are some common errors when reading XML files in Python?
Common errors include `FileNotFoundError` if the file path is incorrect, `ET.ParseError` if the XML is malformed, and `AttributeError` when trying to access non-existent elements.
Is there a way to read large XML files efficiently in Python?
Yes, for large XML files, consider using the `iterparse()` method from `xml.etree.ElementTree`, which allows you to parse the file incrementally and manage memory usage effectively.
Reading XML files in Python is a straightforward process that can be accomplished using various libraries, with the most commonly used being `xml.etree.ElementTree`, `lxml`, and `minidom`. Each of these libraries offers distinct advantages depending on the complexity of the XML data and the specific requirements of the task at hand. The `xml.etree.ElementTree` module is part of the standard library and is ideal for simple XML parsing, while `lxml` provides extensive features for handling large XML files and offers better performance. The `minidom` module, also part of the standard library, is useful for working with XML documents in a more DOM-like manner.
When reading an XML file, it is essential to first parse the file to create an ElementTree object, which allows for easy navigation through the XML structure. Once parsed, elements can be accessed using methods such as `find()`, `findall()`, and `iter()`, enabling users to extract the necessary data efficiently. Additionally, handling namespaces and attributes can be crucial when working with XML files that contain complex structures.
understanding how to read XML files in Python is a valuable skill for developers working with data interchange formats. By leveraging the appropriate
Author Profile
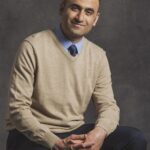
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?